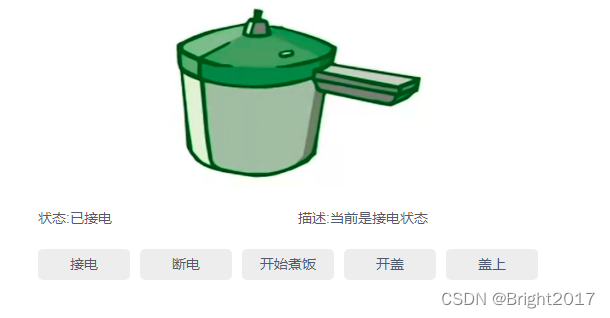
?
<template>
<div class="cooker">
<img src="../assets/gaoyaguo.png" alt="." />
<div class="flex typeName">
<div>状态:{{ typeName }}</div>
<div>描述:{{ desction }}</div>
</div>
<div class="flex buttonSubmit">
<div @click="powerOn">接电</div>
<div @click="powerOff">断电</div>
<div @click="startCook">开始煮饭</div>
<div @click="open">开盖</div>
<div @click="close">盖上</div>
</div>
</div>
</template>
<script>
import Cooker from "./cookerData.js";
const CookerCent = new Cooker();
export default {
data() {
return {
typeName: "~",
desction: "~",
};
},
mounted() {},
methods: {
powerOn() {
CookerCent.changeSwitch('powerOn');
this.desction = CookerCent.getTypeName();
this.typeName = CookerCent.getDesction();
},
powerOff() {
CookerCent.changeSwitch('powerOff');
this.desction = CookerCent.getTypeName();
this.typeName = CookerCent.getDesction();
},
startCook() {
CookerCent.changeSwitch('startCook');
this.desction = CookerCent.getTypeName();
this.typeName = CookerCent.getDesction();
},
open() {
CookerCent.changeSwitch('open');
this.desction = CookerCent.getTypeName();
this.typeName = CookerCent.getDesction();
},
close() {
CookerCent.changeSwitch('close');
this.desction = CookerCent.getTypeName();
this.typeName = CookerCent.getDesction();
},
},
};
</script>
<style>
.flex {
display: flex;
}
button {
cursor: pointer;
}
.cooker{
width: 500px;
margin: 0 auto;
}
img{
display: block;
width: 300px;
margin: 0 auto;
}
.typeName{
width: 100%;
justify-content: center;
margin: 20px 0;
}
.typeName>div{
width: 50%;
}
.typeName>div:nth-child(1){
margin-right: 20px;
}
.buttonSubmit{
width: 100%;
justify-content: center;
}
.buttonSubmit>div{
width:calc((100% - 30px)/4);
margin-right: 10px;
background-color: #ededed;
border-radius: 5px;
cursor: pointer;
padding: 5px 0;
text-align: center;
}
.buttonSubmit>div:last-child{
margin-right: 0;
}
</style>
js
class Cooker {
constructor() { // constructor 是状态变量、固定写法
this.cookerType = new PowerOn(this) // 默认状态 已接电 此时的 this 承接上下文
}
changeSwitch(cookerType) { // 外部调用下面5个类中的方法 默认当前是 已接电 PowerOn、所以点击外部任何按钮、都是调用这个类下面的函数、
this.cookerType[cookerType]()
}
getTypeName() {
return this.cookerType.typeName // 这里的typeName 是下面5个类中的共有属性 this.cookerType 此时代表的就是当前点击时的类、比如默认实例化的类PowerOn、点击的任何函数都是这个类里面的
}
getDesction() {
return this.cookerType.desction // 同上
}
}
class PowerOn { // 当前类的状态是已接电、里面的5个函数分别做了处理、无法执行下一步的会提示文字、可以执行下一步的、实例化下一步的类
constructor(cooker) { // 构造函数传入的参数就是上面的this、可以理解为固定写法
this.cooker = cooker // this.cooker 相当于 Cooker 里面的this
}
powerOn() {
this.typeName = '当前是接电状态'
this.desction = '已接电'
}
powerOff() {
this.cooker.cookerType = new PowerOff(this.cooker) // 当前状态是已接电、这个函数是断电、点击后指向断电的类、之后操作和前面一样、在格子的类中处理各自的状态
}
startCook() {
this.cooker.cookerType = new StartCook(this.cooker)
}
open() {
this.typeName = '当前是接电状态'
this.desction = '不能开盖、会爆炸'
}
close() {
this.typeName = '当前状态-已接电'
this.desction = '已经盖上了'
}
}
class PowerOff {
constructor(cooker) {
this.cooker = cooker
this.typeName = '当前状态-断电'
this.desction = '已断电'
}
powerOn() {
this.cooker.cookerType = new PowerOn(this.cooker)
}
powerOff() {
this.typeName = '当前状态-断电'
this.desction = '已断电'
}
startCook() {
this.typeName = '当前状态-断电'
this.desction = '不能煮饭'
}
open() {
this.typeName = '当前状态-断电'
this.desction = '可以开盖'
}
close() {
this.cooker.cookerType = new Close(this.cooker)
}
}
class StartCook {
constructor(cooker) {
this.cooker = cooker
this.typeName = '当前状态-煮饭'
this.desction = '开始煮饭222'
}
powerOn() {
this.typeName = '当前状态-煮饭'
this.desction = '已接电'
}
powerOff() {
this.cooker.cookerType = new PowerOff(this.cooker)
}
startCook() {
this.typeName = '当前状态-煮饭'
this.desction = '正在煮饭'
}
open() {
this.typeName = '当前状态-煮饭'
this.desction = '不能开盖、会爆炸'
}
close() {
this.typeName = '当前状态-煮饭'
this.desction = '已经盖上了'
}
}
class Open {
constructor(cooker) {
this.cooker = cooker
this.typeName = '当前状态-开盖'
this.desction = ''
}
powerOn() {
this.typeName = '当前状态-开盖'
this.desction = '接电中、不能打开'
}
powerOff() {
this.cooker.cookerType = new PowerOff(this.cooker)
}
startCook() {
this.typeName = '当前状态-开盖'
this.desction = '开盖中、不能煮饭'
}
open() {
this.typeName = '当前状态-开盖'
this.desction = '已开盖'
}
close() {
this.cookerType = new Close(this.cooker)
}
}
class Close {
constructor(cooker) {
this.cooker = cooker
this.typeName = '当前状态-关盖'
this.desction = ''
}
powerOn() {
this.cooker.cookerType = new PowerOn(this.cooker)
}
powerOff() {
this.cooker.cookerType = new PowerOff(this.cooker)
}
startCook() {
this.cooker.cookerType = new StartCook(this.cooker)
}
open() {
this.cooker.cookerType = new Open(this.cooker)
}
close() {
this.typeName = '当前状态-关盖'
this.desction = '已关盖'
}
}
export default Cooker
|