一、父子组件之间的通信
1、父传子——props
父组件在使用子组件标签中通过字面量来传递值
<template>
<Childrean :name="name"/>
</template>
<script>
import Children from "引用子组件的路径"
data(){
return{
name:"zyy"
}
}
</script>
子组件设置props属性,定义接收父组件传递过来的参数
<script>
props:{
name:{
type:String,
default:"",
required: true
}
}
</script>
2、子传父——$emit 触发自定义事件/ref
- $emit 触发自定义事件
1、子组件通过
e
m
i
t
触
发
自
定
义
事
件
,
emit触发自定义事件,
emit触发自定义事件,emit第一个参数为触发的事件,第二个参数为传递的数值。 (1)子组件通过事件触发$emit
<template>
<button @click="passPar"></button>
</template>
<script>
data(){
name:"zyy"
},
methods:{
passPar(){
this.$emit('add', this.name);
}
}
</script>
(2)子组件通过生命周期触发$emit
<template>
</template>
<script>
data(){
name:"zyy"
},
created(){
this.passPar();
},
methods:{
passPar(){
this.$emit('add', name);
}
}
</script>
2、父组件绑定监听器获取到子组件传递过来的参数,父组件触发的事件是子组件$emit事件定义的第一个参数,如例子是add
<template>
<Childrean @add="update"/>
</template>
<script>
import Children from "引用子组件的路径"
data(){
return{
name:""
}
},
methods:{
update(data){
this.name=data;
}
}
</script>
- ref
子组件:
<template>
<div class=“children”>
</div>
</template>
<script>
data(){
name:"zyy"
},
methods:{
passPar(){
}
}
</script>
父组件:
<template>
<Childrean ref="foo"/>
</template>
<script>
import Children from "引用子组件的路径"
data(){
return{
name:""
}
},
mounted(){
this.getData();
},
methods:{
getData(){
this.$refs.foo;
this.name=this.$refs.foo.name;
}
}
</script>
二、兄弟组件之间的通信——EventBus
- 创建一个中央事件总线EventBus
- 兄弟组件通过$
e
m
i
t
触
发
自
定
义
事
件
,
emit触发自定义事件,
emit触发自定义事件,emit第二个参数为传递的数值
- 另一个兄弟组件通过$on监听自定义事件
Bus.js
class Bus {
constructor() {
this.callbacks = {};
}
$on(name, fn) {
this.callbacks[name] = this.callbacks[name] || [];
this.callbacks[name].push(fn);
}
$emit(name, args) {
if (this.callbacks[name]) {
this.callbacks[name].forEach((cb) => cb(args));
}
}
}
Vue.prototype.$bus = new Bus()
Vue.prototype.$bus = new Vue()
Children1.vue
this.$bus.$emit('foo')
Children2.vue
this.$bus.$on('foo', this.handle)
三、祖孙与后代组件之间的通信——$ parent 或$ root
1、通过共同祖辈$ parent或者$ root搭建通信桥连
兄弟组件
this.$parent.on('add',this.add)
另一个兄弟组件
this.$parent.emit('add')
2、祖先传递数据给子孙:$ attrs 与$ listeners
设置批量向下传属性$attrs和 $ listeners 包含了父级作用域中不作为 prop 被识别 (且获取) 的特性绑定 ( class 和 style 除外)。 可以通过 v-bind="$attrs" 传?内部组件
<p>{{$attrs.foo}}</p>
<HelloWorld foo="foo"/>
<Child2 msg="lalala" @some-event="onSomeEvent"></Child2>
<Grandson v-bind="$attrs" v-on="$listeners"></Grandson>
<div @click="$emit('some-event', 'msg from grandson')">
{{msg}}
</div>
3、provide 与 inject
在祖先组件定义provide属性,返回传递的值 祖先组件:
provide(){
return {
foo:'foo'
}
}
在后代组件通过inject接收组件传递过来的值 后代组件:
inject:['foo']
四、vuex
- 适用场景: 复杂关系的组件数据传递
- Vuex作用相当于一个用来存储共享变量的容器
- state用来存放共享变量的地方
- getter,可以增加一个getter派生状态,(相当于store中的计算属性),用来获得共享变量的值
- mutations用来存放修改state的方法。
- actions也是用来存放修改state的方法,不过action是在mutations的基础上进行。常用来做一些异步操作
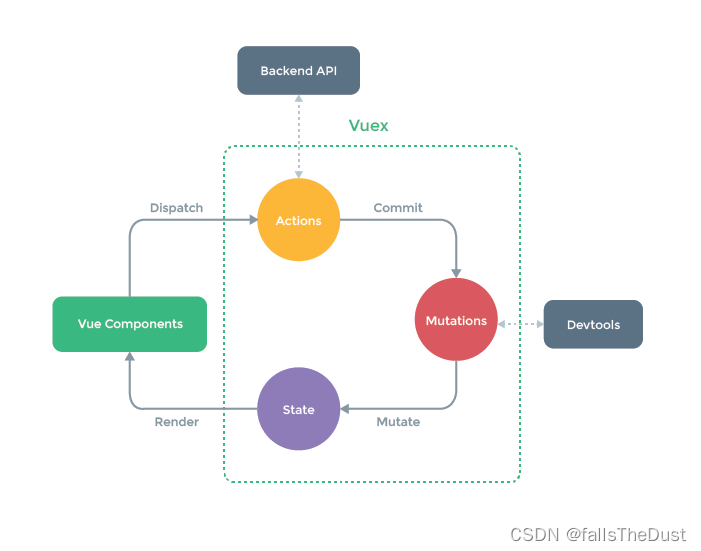
|