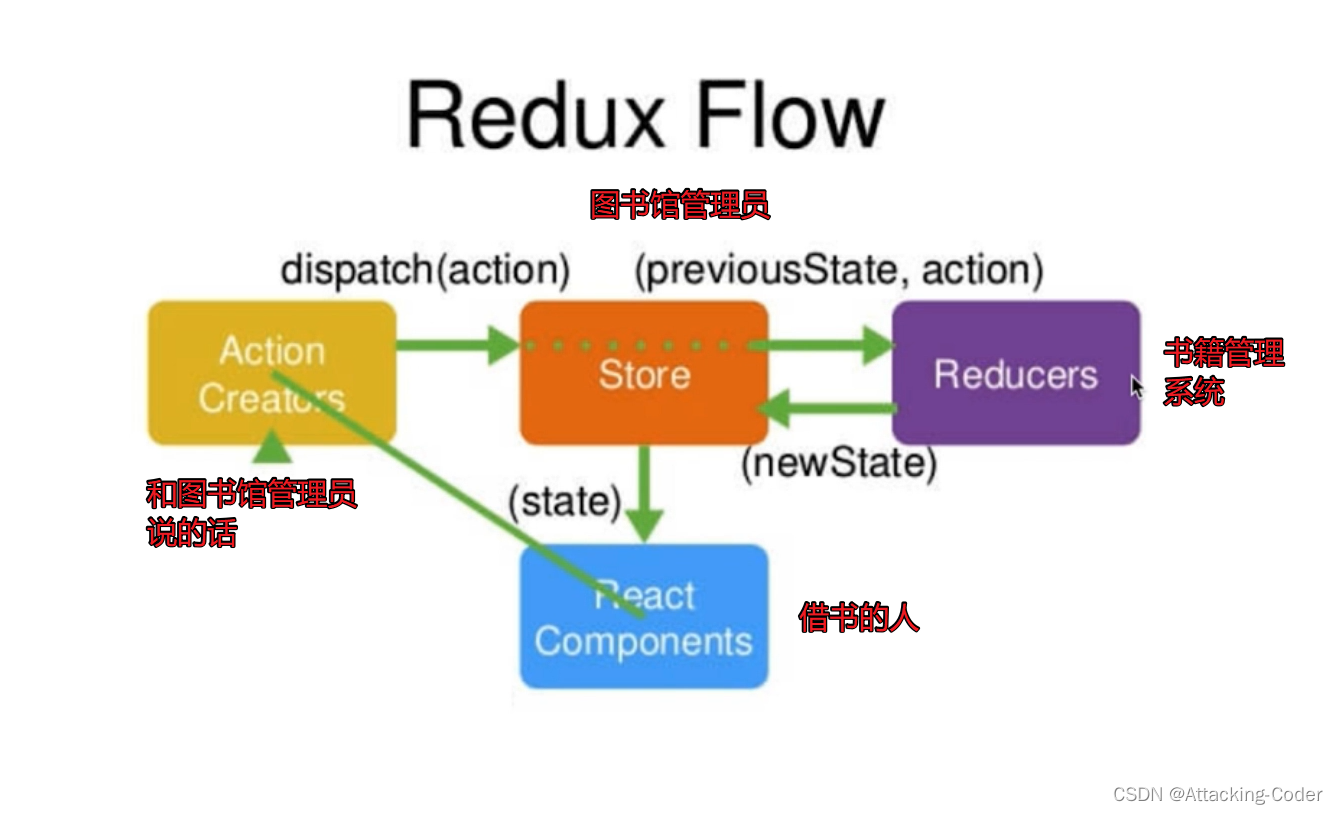
工作流程
更改store中的数据
组件通过dispatch发布action命令
const action = {
type:"del_item"
}
store.dispatch(action)
当store拿到命令后它会把当前的state数据和action命令一起传给reducer reducer深拷贝一份state数据,然后将新数据按照命令修改,并将新数据返给store
export default (state, action) => {
if (action.type === "del_item") {
let newState = JSON.parse(JSON.stringify(state))
newState.list.splice(action.index, 1)
return newState
}
return state
}
store对state数据进行更新
为什么reducer要深拷贝一下:reducer可以接受state 但是绝对不能修改state 所以要深拷贝一下
组件获取store的数据
store.getState()
组件订阅store数据
store.subscribe(this.storeChanged)
当store中的数据发生变更,则会触发store.subscribe中的this.storeChanged函数(这个函数名随便起)
action如果是函数(需要发送异步请求)应该怎么写
首先需要安装“redux-thunk” 配置store/index.js文件
import { createStore, applyMiddleware, compose } from 'redux'
import thunk from 'redux-thunk'
import reducer from "./reducer";
const composeEnhancers = window.__REDUX_DEVTOOLS_EXTENSION_COMPOSE__ ? window.__REDUX_DEVTOOLS_EXTENSION_COMPOSE__({}) : compose;
const enhancer = composeEnhancers(
applyMiddleware(thunk),
);
const store = createStore(reducer, enhancer)
export default store
配置store/actionCreators.js文件 getTodoList(函数名随便)返回不是对象而是函数(如果没有“redux-thunk”,则会报错,正常action只能是对象) 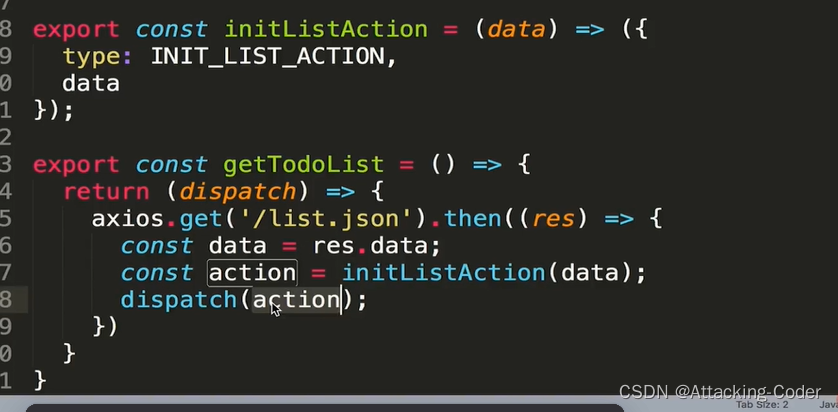 在组件中发送action命令,thunk会自动调用函数 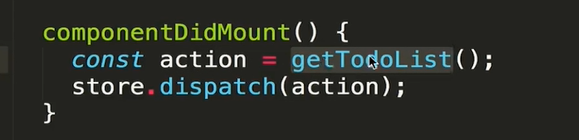
什么是redux中间件
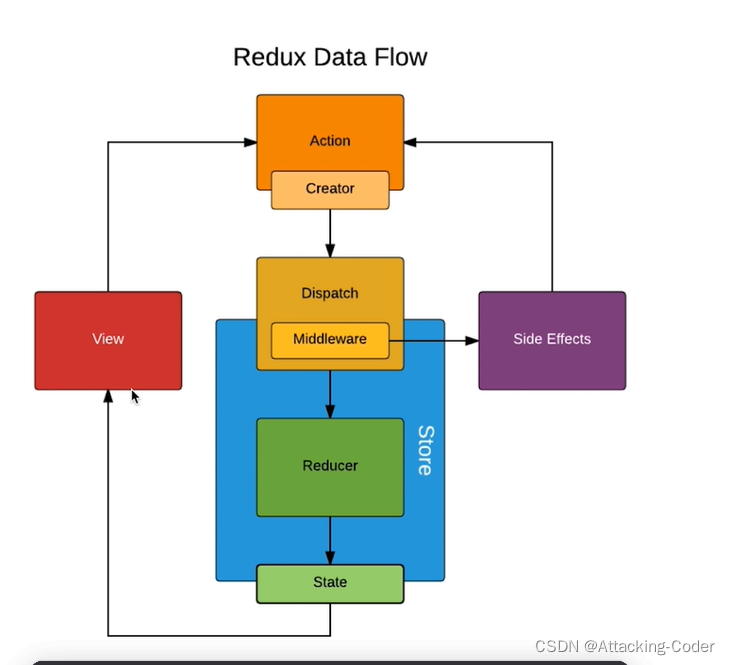
中间件是Action和Store中间执行的东西。 例如“redux-thunk”
假如action传过来的是个对象则正常传给Store然后给reducer处理 但是如果action是个函数则中间件先回把函数执行完,然后再把有用的数据dispatch给store
react-redux的使用
安装
npm install react-redux
在src/index.js如下配置
主要就是用Provider将store和子组件联系起来
import React from 'react';
import ReactDOM from 'react-dom';
import Todolist from './Todolist'
import { Provider } from 'react-redux'
import store from './store';
const App = (
<Provider store={store}>
<Todolist></Todolist>
</Provider>
)
ReactDOM.render(
App,
document.getElementById('root')
);
组件进行如下配置
主要就是通过 connect将组件和store链接起来
import React, { Component } from 'react'
import { connect } from 'react-redux'
export class Todolist extends Component {
render() {
return (
<div>
<div>
<input value={this.props.inputValue} onChange={this.props.inputChange}></input>
<button>提交</button>
</div>
<ul>
<li>12345646</li>
</ul>
</div>
)
}
}
const mapStateToProps = (state) => {
return {
inputValue: state.inputValue
}
}
const mapDispatchToProps = (dispatch) => {
return {
inputChange(e) {
const action = {
type: "input_change",
value: e.target.value
}
dispatch(action)
}
}
}
export default connect(mapStateToProps, mapDispatchToProps)(Todolist)
store文件夹下index和reducer如下配置
store/index.js
import { createStore } from "redux";
import reducer from "./reducer"
const store = createStore(reducer)
export default store
store/reducer.js
const defaultState = {
inputValue: 'hello',
list: []
}
const reducer = (state = defaultState, action) => {
if (action.type == 'input_change') {
const newState = JSON.parse(JSON.stringify(state))
newState.inputValue = action.value
return newState
}
return state
}
export default reducer
|