?
目录
代码:
?
效果:
代码:
<div class="box">
<i class="tl"></i>
<i class="tr"></i>
<i class="bl"></i>
<i class="br"></i>
</div>
<script>
function Box(el) {
this.el = el
let that = this
let dirs = {
tl: {ox: 1,oy: 1,x: -1,y: -1},
tr: {ox: 0,oy: 1,x: 1,y: -1},
bl: {ox: 1,oy: 0,x: -1,y: 1},
br: {ox: 0,oy: 0,x: 1,y: 1}
}
let startX = null, startY = null, left = 0, top = 0, w = 0, h = 0
let action = '', _w, _h, _l, _t, ox, oy, curDir
function move(e) {
if (startX == null) {
startX = e.pageX
startY = e.pageY
return
}
let offsetX = e.pageX - startX
let offsetY = e.pageY - startY
if (action == 'resize') {
let sx = 1 + curDir.x * offsetX / w
let sy = 1 + curDir.y * offsetY / h
ox = curDir.ox * w + left
oy = curDir.oy * h + top
ox-=ox*sx
oy-=oy*sy
let px = sx * left + ox
let py = sy * top + oy
let px2 = sx * (left + w) + ox
let py2 = sy * (top+h) + oy
_w = px2 - px
_h = py2 - py
_l = px
_t = py
if (_w > 1e-2 && _h > 1e-2) {
that.el.style.width = _w + 'px'
that.el.style.height = _h + 'px'
that.el.style.left = _l + 'px'
that.el.style.top = _t + 'px'
}
} else {
that.el.style.left = (offsetX + left) + 'px'
that.el.style.top = (offsetY + top) + 'px'
}
}
function up(e) {
startX = null
startY = null
document.body.style.cursor = ''
document.removeEventListener('mousemove', move, false)
document.removeEventListener('mouseup', up, false)
}
this.el.addEventListener('mousedown', (e) => {
e.preventDefault();
let rect = that.el.getBoundingClientRect()
left = rect.left
top = rect.top
w = rect.width
h = rect.height
let target = e.target
if (target.nodeType == 1 && target.closest('i')) {
action = 'resize'
document.body.style.cursor = window.getComputedStyle(target).cursor
curDir = dirs[target.className]
} else {
action = 'move'
document.body.style.cursor = 'move'
}
document.addEventListener('mousemove', move, false)
document.addEventListener('mouseup', up, false)
})
}
new Box(document.querySelector('.box'))
</script>
<style>
.box {
border: solid 1 #000;
height: 100px;
width: 100px;
position: absolute;
border: solid 1px #000;
}
.box>i {
position: absolute;
border: solid 1px #000;
height: 10px;
width: 10px;
transform: translate(-50%, -50%);
background-color: transparent;
}
.tl {
top: 0;
left: 0;
cursor: se-resize;
}
.tr {
top: 0;
left: 100%;
cursor: sw-resize;
}
.bl {
top: 100%;
left: 0;
cursor: ne-resize;
}
.br {
top: 100%;
left: 100%;
cursor: nw-resize;
}
</style>
?
效果:
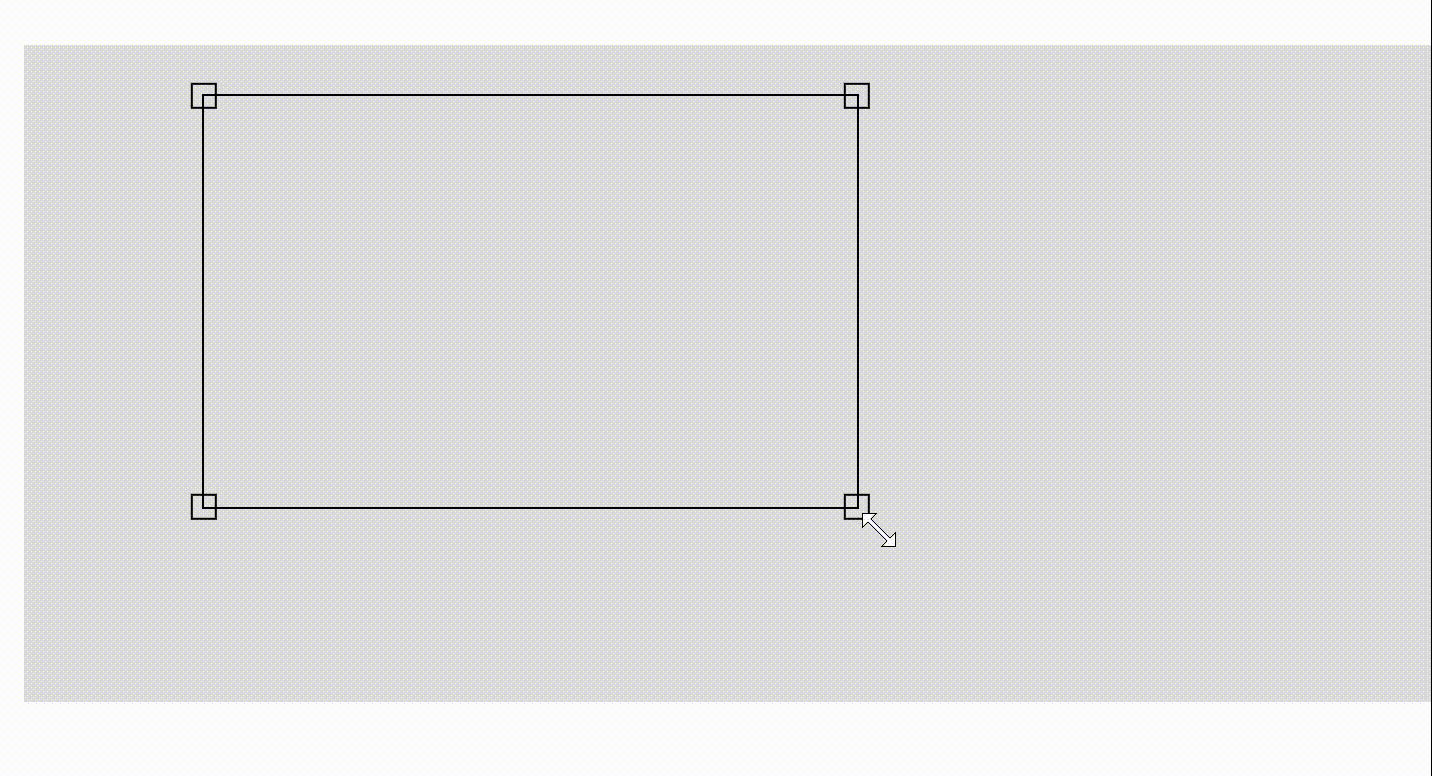
?
|