一、组件
组件是可复用的Vue实例,说白了就是一组可以重复使用的模板
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div id="app">
<study></study>
</div>
<!--导入Vue.js-->
<script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script>
<script>
//定义一个Vue组件component
Vue.component("study",{
template:'<li>Hello</li>'
});
var vm = new Vue({
el:'#app',
data:{
}
})
</script>
</body>
</html>
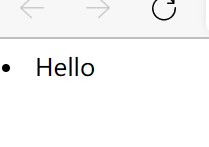
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div id="app">
<!--组件:传递给组件中的值:props-->
<study v-for="item in items" v-bind:can="item"></study>
</div>
<!--导入Vue.js-->
<script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script>
<script>
//定义一个Vue组件component
Vue.component("study",{
props:['can'],
template:'<li>{{can}}</li>'
});
var vm = new Vue({
el:'#app',
data:{
items:["java","python","Linux"]
}
})
</script>
</body>
</html>
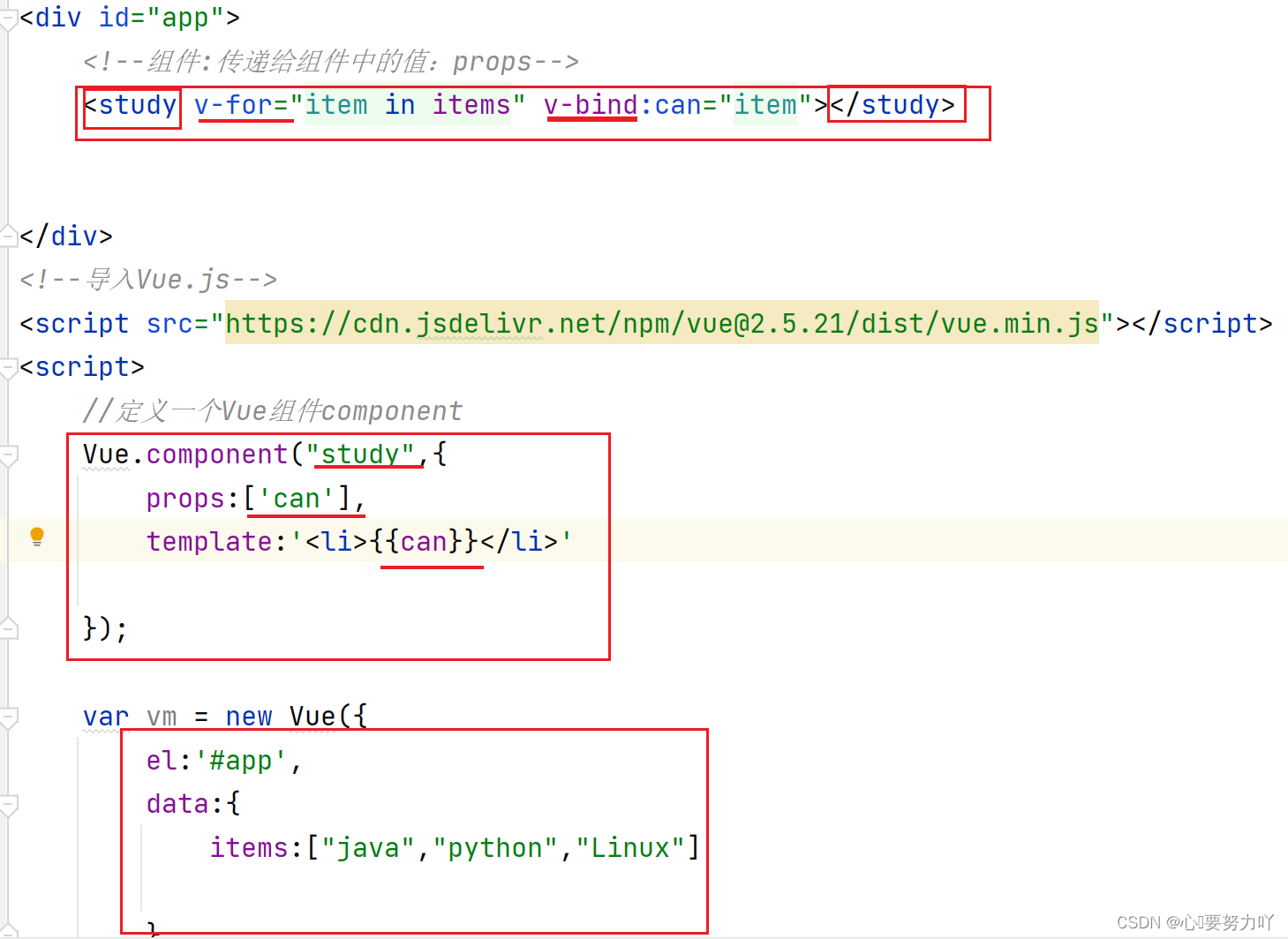
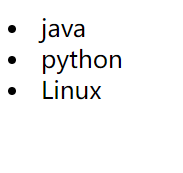
二、Axios异步通信
2.1Axios访问基本数据
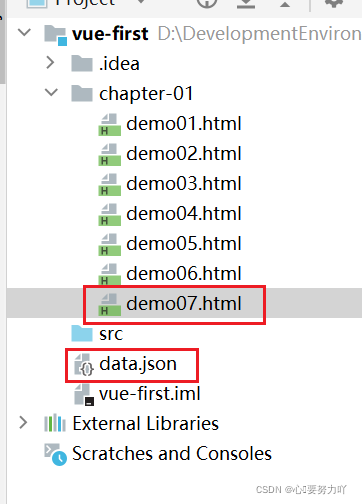
data.json 代码
{
"name": "vue",
"url": "https://www.bilibili.com/",
"page": 1,
"isNonProfit": true,
"address": {
"street": "xxx",
"city": "北京",
"country": "中国"
},
"links": [
{
"name": "bilbili",
"url": "https://space.bilbili.com/95256449"
},
{
"name": "狂胜说java",
"url": "https://blog.kuangstudy.com"
},
{
"name": "百度",
"url": "https://www.baidu.com/"
}
]
}
demo07代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<!--v-clock:解决闪烁问题-->
<style>
[v-clock]{
display: none;
}
</style>
</head>
<body>
<div id="vue" v-clock>
<div>{{info.address.city}}</div>
<div>{{info.name}}</div>
</div>
<!--导入Vue.js-->
<script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script>
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script type="text/javascript">
var vm = new Vue({
el:'#vue',
//data:属性:vm
data(){
return{
//请求的返回参数合适,必须和json字符串一样
info:{
name:null,
address:{
street:null,
city:null,
country:null
}
}
}
},
mounted(){ //钩子函数 链式编程 ES6新特性
axios.get('../data.json').then(response=>(this.info=response.data));
}
})
</script>
</body>
</html>
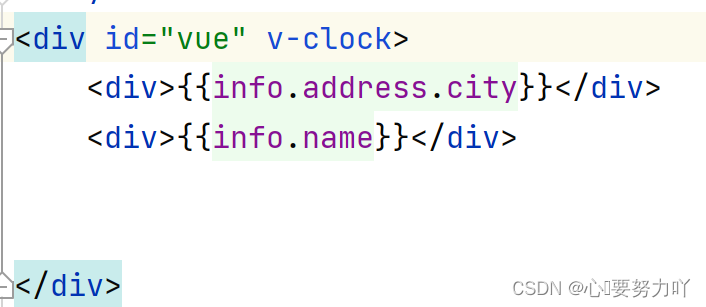 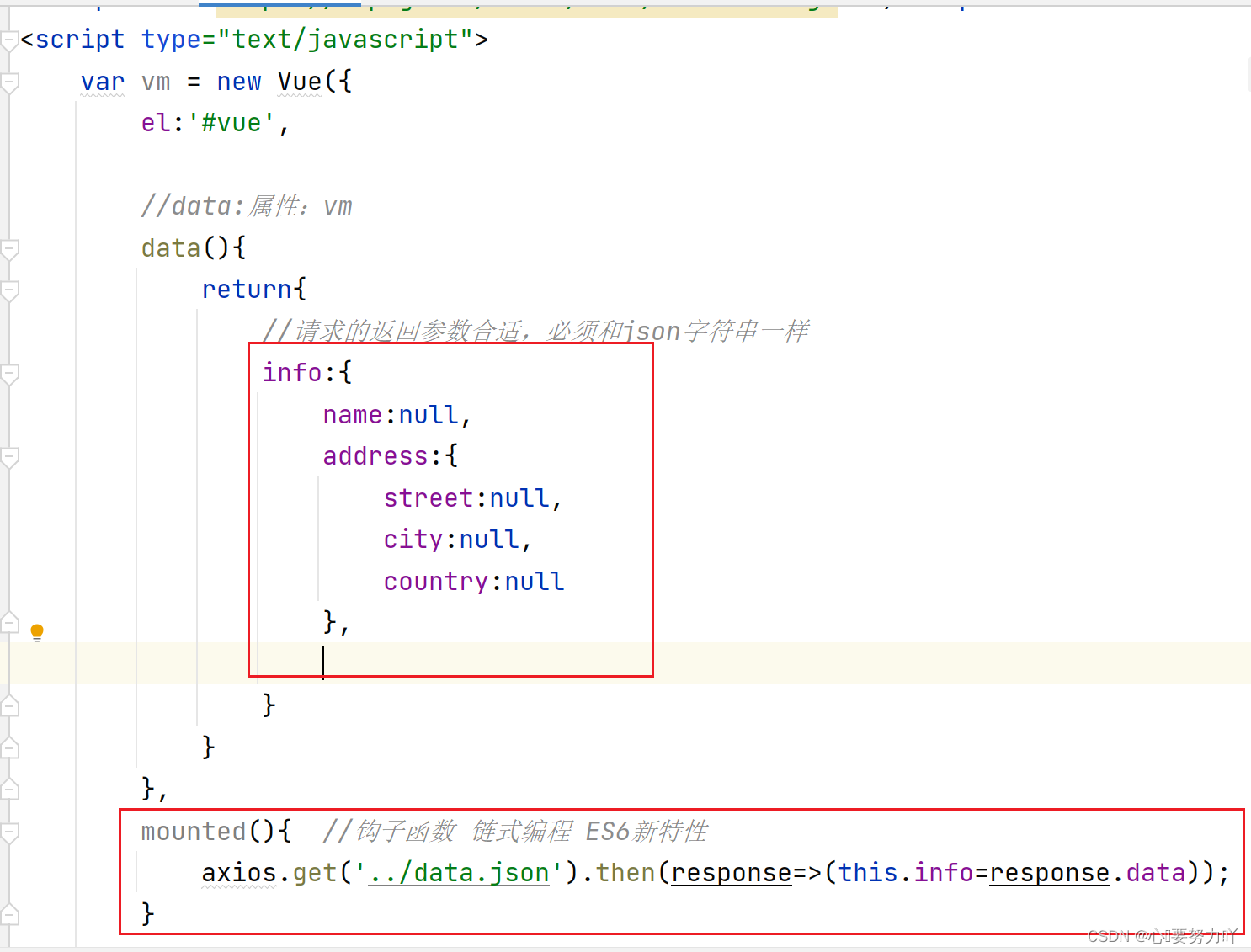
结果: 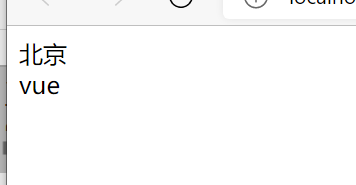 解决网页闪烁问题
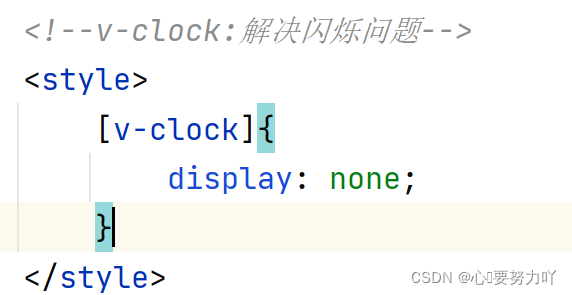
2.2 Axios访问链接
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<!--v-clock:解决闪烁问题-->
<style>
[v-clock]{
display: none;
}
</style>
</head>
<body>
<div id="vue" v-clock>
<div>{{info.address.city}}</div>
<div>{{info.name}}</div>
<a v-bind:href="info.url">点我</a>
</div>
<!--导入Vue.js-->
<script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script>
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script type="text/javascript">
var vm = new Vue({
el:'#vue',
//data:属性:vm
data(){
return{
//请求的返回参数合适,必须和json字符串一样
info:{
name:null,
address:{
street:null,
city:null,
country:null
},
url:null
}
}
},
mounted(){ //钩子函数 链式编程 ES6新特性
axios.get('../data.json').then(response=>(this.info=response.data));
}
})
</script>
</body>
</html>
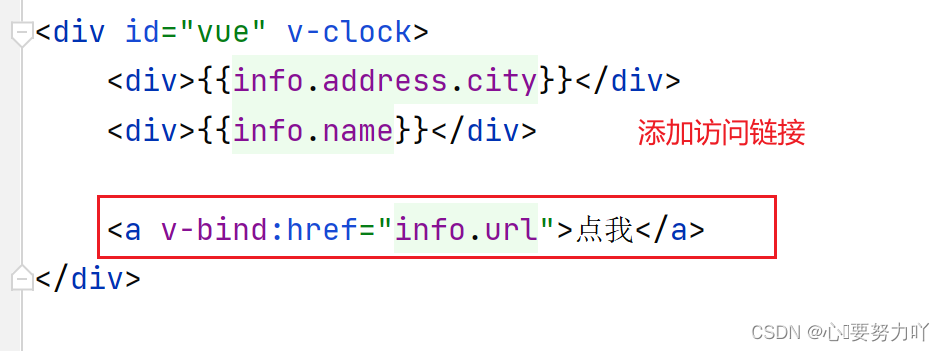 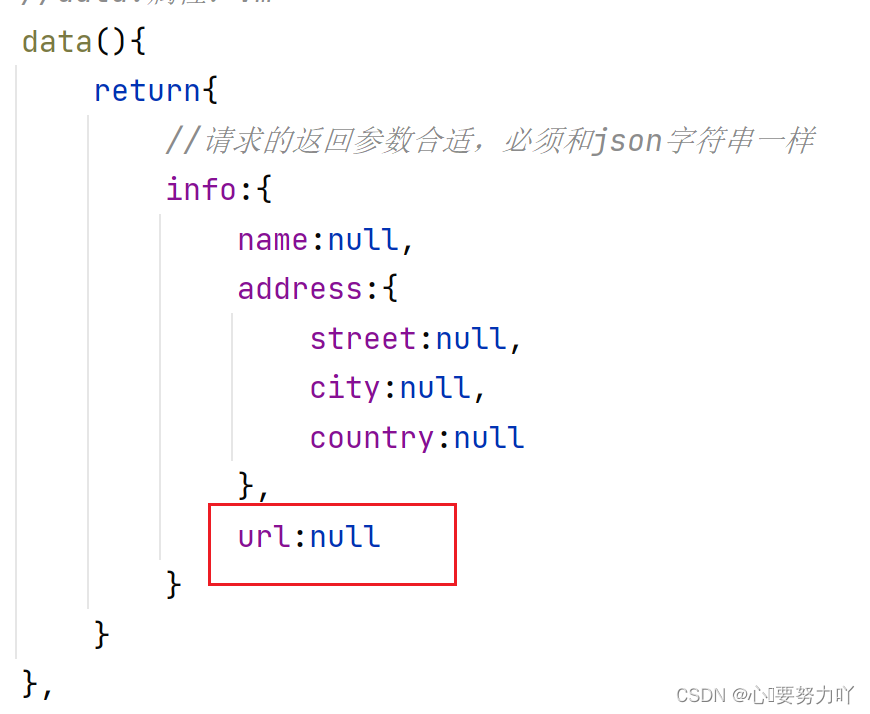 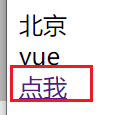
|