一、计算属性
1.1定义
重点在属性两个字上面,这里的计算就是个函数,它就是一个能够将计算结果缓存起来的属性,可以想象为缓存
1.2主要特性
为了将不进厂发生变化的计算结果进行缓存,以节约我们的系统开销
计算属性 methods computed方法名不能重名,重名之后只会调用methods中的方法,所以不建议方法重名
methods在调用的时候需要调用方法名加(),而computed在调用的时候直接调用方法名
1.3例子
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>计算属性</title>
</head>
<body>
<div id="app">
<p>currencyTime1={{currencyTime1()}}</p>
<p>currencyTime2={{currencyTime2}}</p>
</div>
<!--导入Vue.js-->
<script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script>
<script>
let ww = new Vue({
el: "#app",
data: {
message:"hello,vue!!"
},
methods: {
currencyTime1: function () {
return Date.now();//返回一个时间戳
}
},
computed: { //计算属性 methods computed方法名不能重名,重名之后只会调用methods中的方法
currencyTime2:function (){
return Date.now();//返回一个时间戳
}
}
})
</script>
</body>
</html>
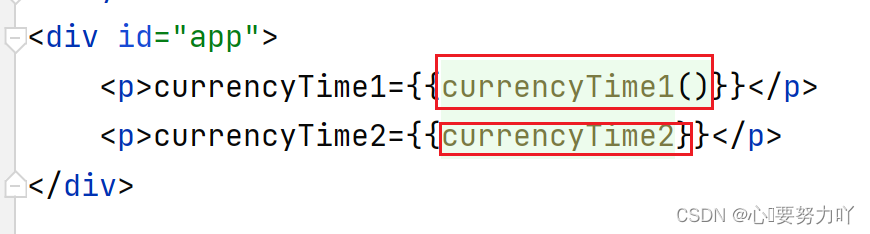 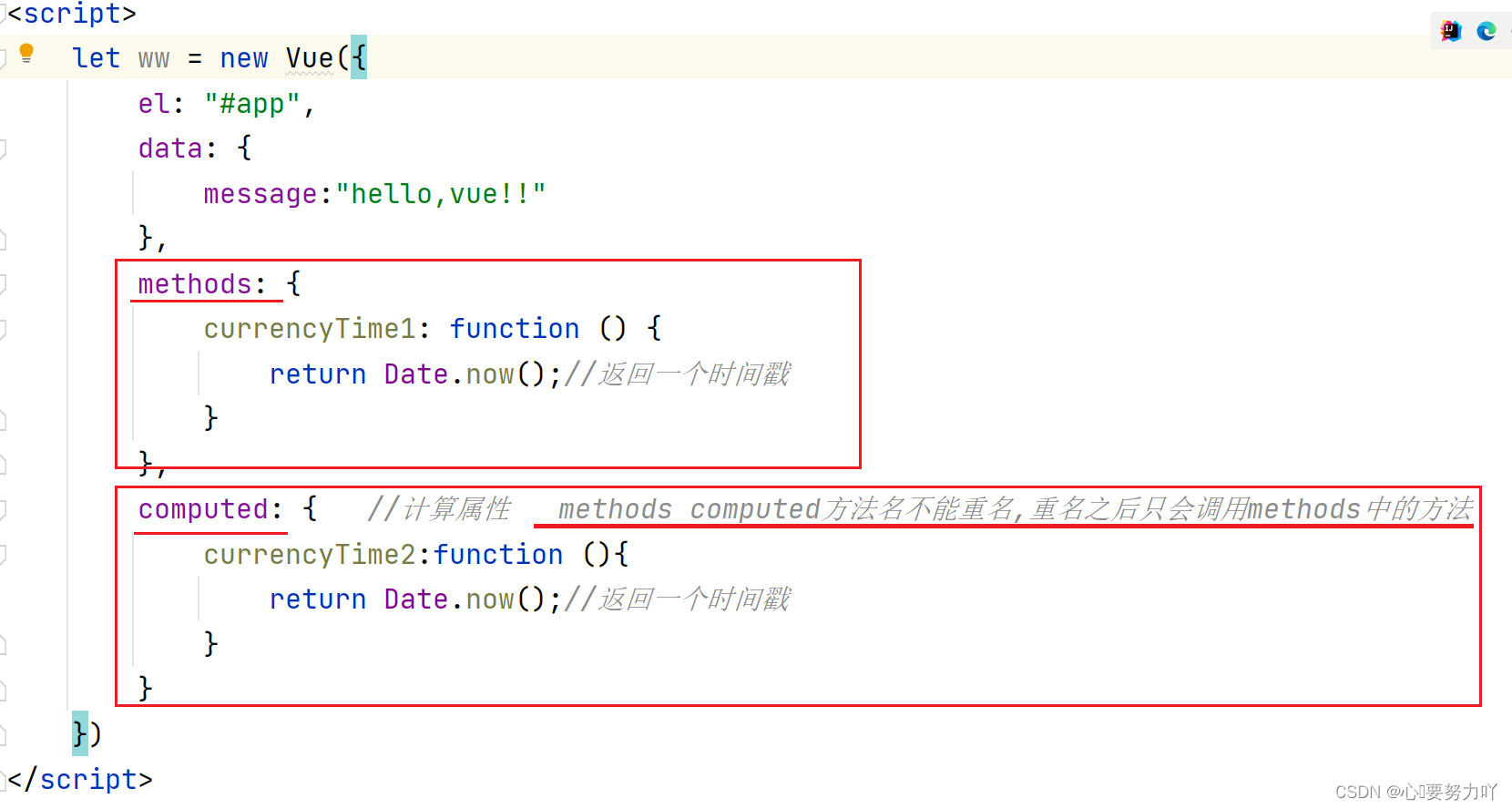
结果: 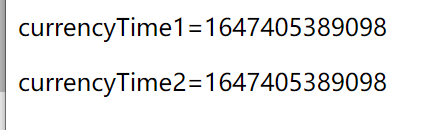
二、插槽(slot)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>插槽</title>
</head>
<body>
<div id="app">
<todo-title slot="todo-title" :title="title"></todo-title>
<todo-items slot="todo-items" v-for="item in todoItems" :item="item"></todo-items>
</div>
<!--导入Vue.js-->
<script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script>
<script>
Vue.component("todo",{
template: '<div>\
<slot name="todo-title"></slot>\
<ul>\
<slot name="todo-items"></slot>\
</ul>\
</div>'
})
Vue.component("todo-title",{
props: ['title'],
template: '<div>{{title}}</div>'
});
Vue.component("todo-items",{
props: ['item'],
template: '<li>{{item}}</li>'
});
var vm = new Vue({
el:"#app",
data:{
title:"课程",
todoItems:['java','前端','python']
}
})
</script>
</body>
</html>
 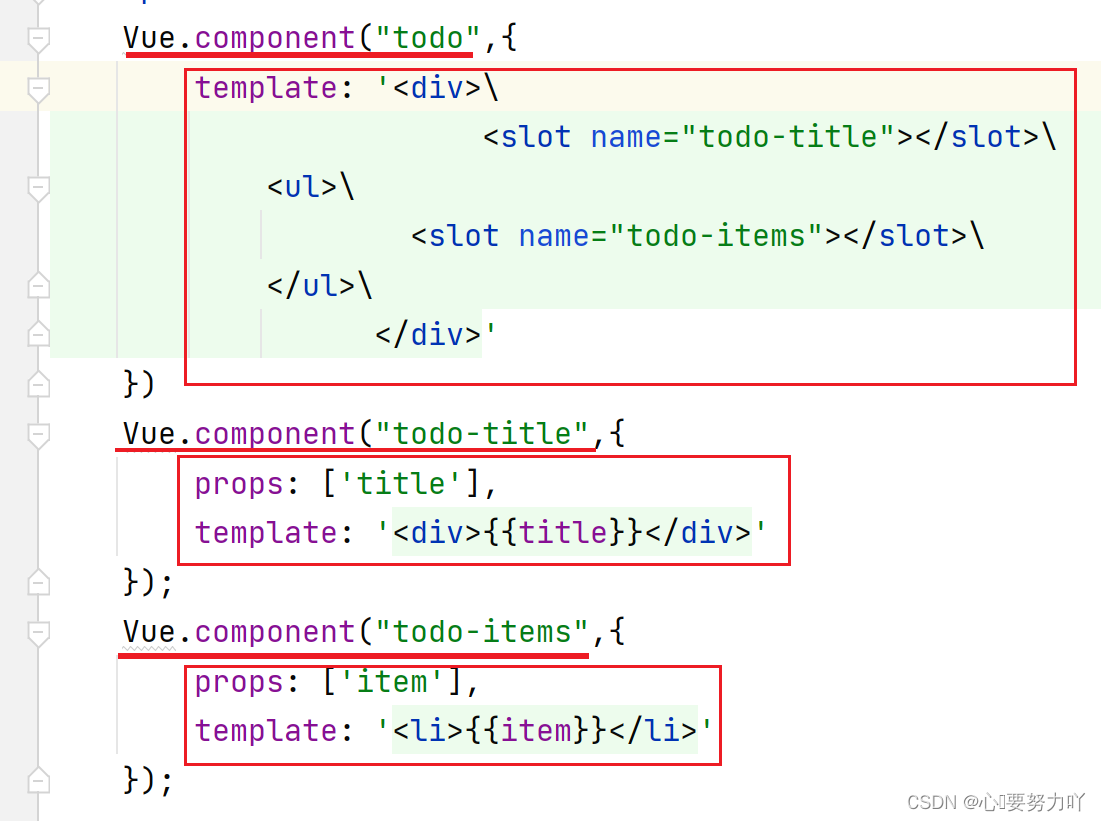
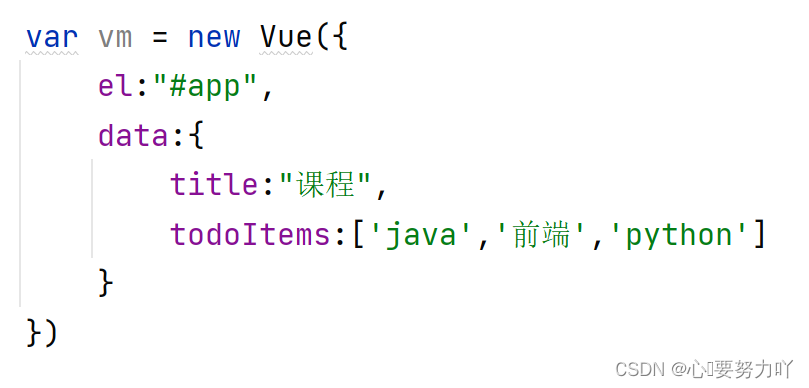
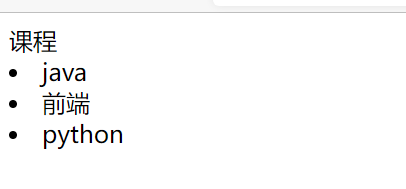
三、自定义事件内容分发
3.1在Vue实例里面删除
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>插槽</title>
</head>
<body>
<div id="app">
<todo-title slot="todo-title" :title="title"></todo-title>
<todo-items slot="todo-items" v-for="item in todoItems" :item="item"></todo-items>
</div>
<!--导入Vue.js-->
<script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script>
<script>
Vue.component("todo",{
template: '<div>\
<slot name="todo-title"></slot>\
<ul>\
<slot name="todo-items"></slot>\
</ul>\
</div>'
})
Vue.component("todo-title",{
props: ['title'],
template: '<div>{{title}}</div>'
});
Vue.component("todo-items",{
props: ['item'],
template: '<li>{{item}}<button>删除</button></li>',
methods: {
remove:function (){
alert('OK')
}
}
});
var vm = new Vue({
el:"#app",
data:{
title:"课程",
todoItems:['java','前端','python']
},
methods: {
removeItem: function (index){
console.log("删除了"+this.todoItems[index]+"OK");
this.todoItems.splice(index,1); //一次删除一个元素
}
}
});
</script>
</body>
</html>
主要代码: 
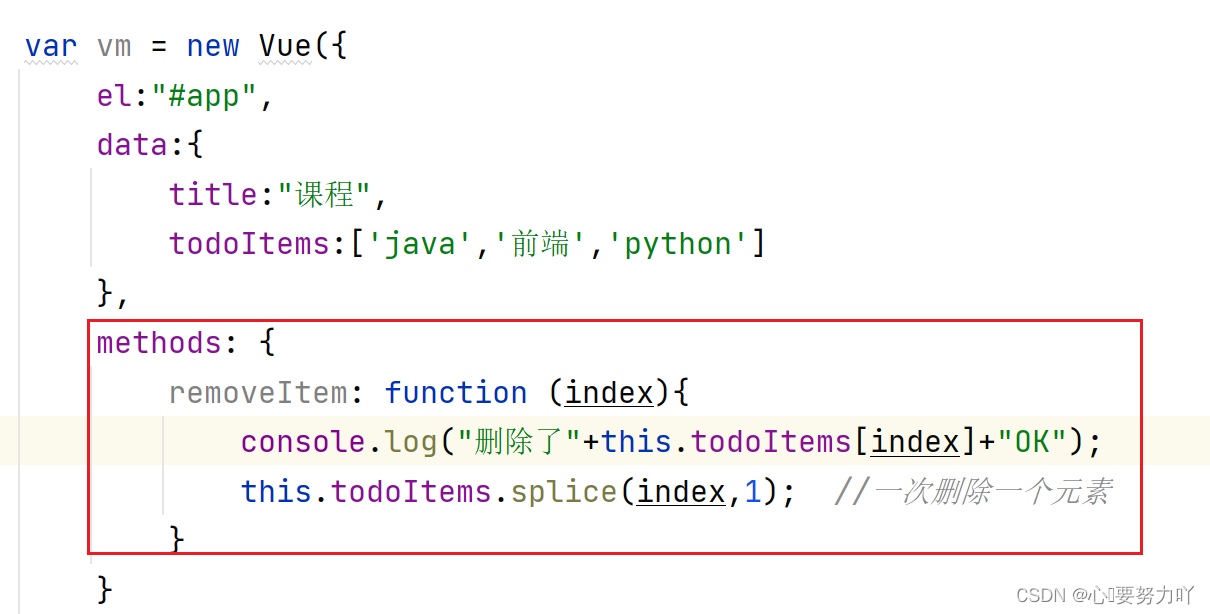
结果;在控制台手动删除 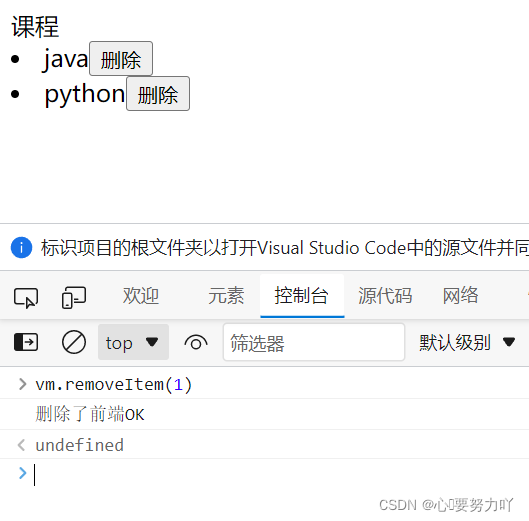
3.2在Vue组件里面删除,组件如何删除Vue实例中的数据?
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>插槽</title>
</head>
<body>
<div id="app">
<todo>
<todo-title slot="todo-title" :title="title"></todo-title>
<todo-items slot="todo-items" v-for="(item,index) in todoItems"
:item="item" v-bind:index="index" v-on:remove="removeItems(index)" :key="index"></todo-items>
</todo>
</div>
<!--导入Vue.js-->
<script src="https://cdn.jsdelivr.net/npm/vue@2.5.21/dist/vue.min.js"></script>
<script>
//slot:插槽
Vue.component("todo",{
template: '<div>\
<slot name="todo-title"></slot>\
<ul>\
<slot name="todo-items"></slot>\
</ul>\
</div>'
})
Vue.component("todo-title",{
props: ['title'],
template: '<div>{{title}}</div>'
});
Vue.component("todo-items",{
props: ['item','index'],
//只能绑定当前组件的方法
template: '<li>{{index}}----{{item}} <button @click="remove">删除</button></li>',
methods: {
remove:function (index){
//this.$emit 自定义事件分发
this.$emit('remove',index);
}
}
});
var vm = new Vue({
el:"#app",
data:{
title:"课程",
todoItems:['java','前端','python']
},
methods: {
removeItems: function (index){
console.log("删除了"+this.todoItems[index]+"OK");
this.todoItems.splice(index,1); //一次删除一个元素
}
}
});
</script>
</body>
</html>
 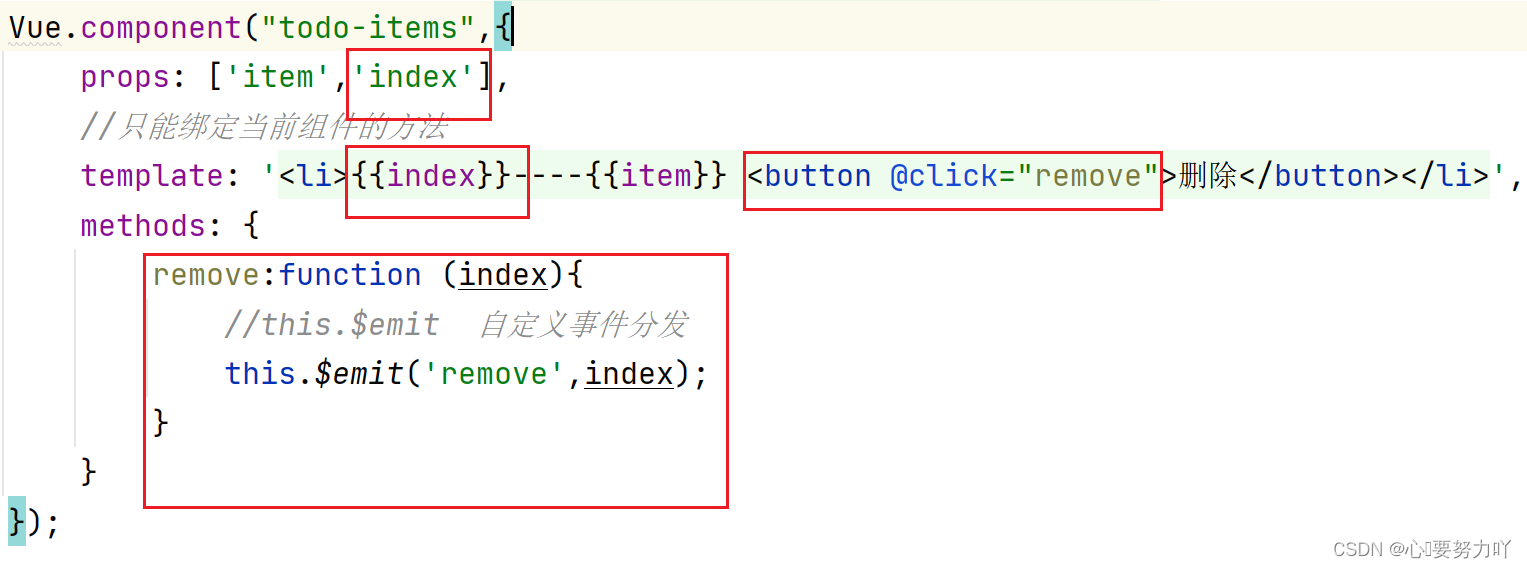
结果: 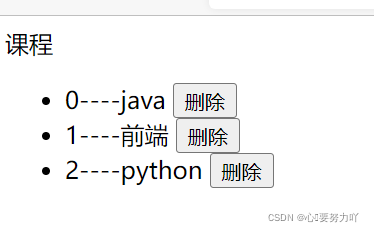 点击删除: 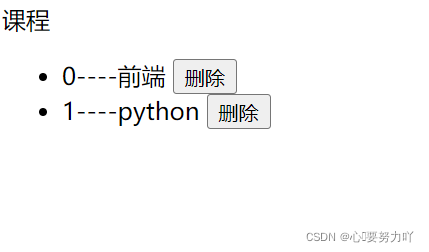
四、总结
常用属性: v-if v-else v-else-if v-for v-on 绑定事件,简写@ v-model 数据双向绑定 v-bind 给组件绑定参数 ,简写:(冒号)
组件化: 组合组件slot插槽 组件内部绑定事件需要使用到this.$emit(“事件名”,参数); 计算属性的特色,缓存计算数据
|