xterm.js 作为终端面板,以 socket.js + stompjs 实现消息通信。
效果如下: 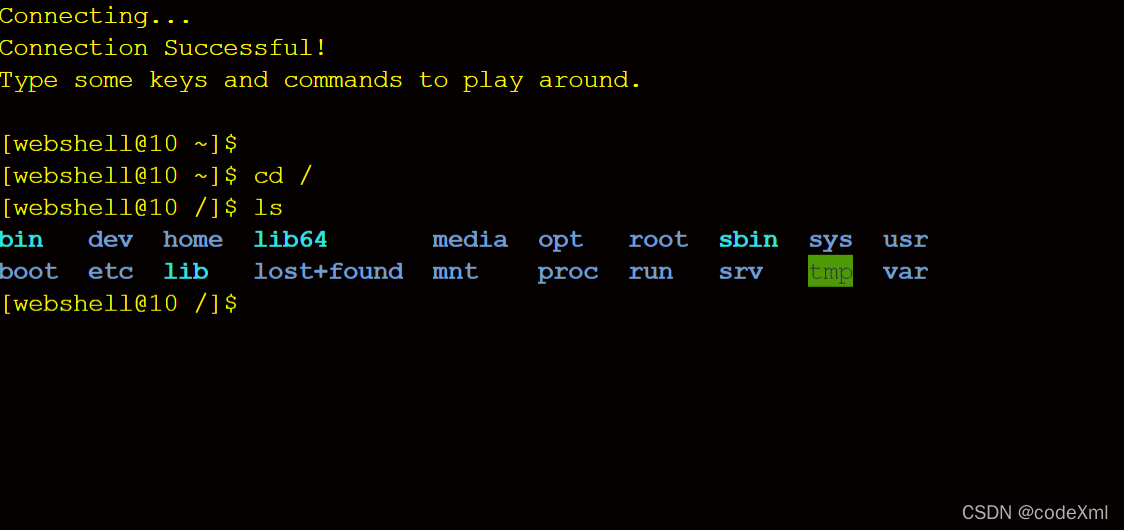 代码:
<template>
<div>
<div ref="terminalContainer"></div>
</div>
</template>
<script lang="ts">
import {Vue, Component, Prop } from "vue-property-decorator";
import { Terminal } from "xterm";
import "xterm/css/xterm.css";
import SockJS from "sockjs-client";
import Stomp from "stompjs";
import { cloneDeep } from "lodash-es";
@Component({
name: "TerminalDrawer"
})
export default class TerminalDrawer extends Vue {
socket = null;
term = null;
stompClient = null;
socketOptions = {
url: "/amo/amo-socket",
subscribes: ["/amo/"],
sendUrl: "/amo/webssh/recever",
}
mounted() {
this.initWebSSH();
}
initWebSSH () {
this.$nextTick(() => {
this.openTerminal();
});
}
disconnect() {
this.stompClient.disconnect();
this.term.dispose();
console.log("Disconnected!");
}
onSendMsg (data) {
this.stompClient.send(this.socketOptions.sendUrl, {}, JSON.stringify(data));
}
createSocket (term) {
const that = this;
let msgObj = {
ip: "xx.xx.xx.xx",
port: 8080,
msg: "",
status: "CONNECT"
};
const onSubscribes = () => {
const subscribes = this.socketOptions.subscribes;
subscribes.forEach(url => {
const link = url + "自定义参数,比如主机ip,需与后台协商";
this.stompClient.subscribe(
link,
msg => {
let { body } = msg;
body = JSON.parse(body);
const { bizCode, val } = body;
console.log(bizCode + "_received", val);
term.write(val);
},
{}
);
});
};
const onKeyBoard = ()=> {
term.onData(str => {
msgObj.msg = str;
msgObj.status= "COMMAND";
this.onSendMsg(msgObj);
});
};
this.socket = new SockJS(this.socketOptions.url, null, {
"transports": ["websocket"],
timeout: 15000
});
this.stompClient = Stomp.over(this.socket);
this.stompClient.connect(
{},frame => {
onKeyBoard();
that.onSendMsg(msgObj);
onSubscribes();
term.writeln("Connection Successful!");
term.writeln("Type some keys and commands to play around.");
term.focus();
},() => {
term.writeln("");
term.writeln("Connection Fail!");
term.writeln("");
console.log("***连接失败***");
}
);
}
runFakeTerminal(term) {
if (term._initialized) {
return;
}
term._initialized = true;
term.writeln("Connecting...");
}
openTerminal(){
const terminalDom = this.$refs.terminalContainer as any;
const term = new Terminal({
rows: 40,
cursorBlink: true,
cursorStyle: "block",
scrollback:10,
tabStopWidth: 8,
disableStdin: false,
rendererType: "canvas",
theme: {
foreground: "yellow",
background: "#060101",
cursor: "help",
}
});
term.open(terminalDom);
this.term = term;
this.runFakeTerminal(term);
this.createSocket(term);
}
}
</script>
|