vue项目纯前端配置跨域实践
首次配置伪装请求以达到跨域的目的
vue.config.js文件
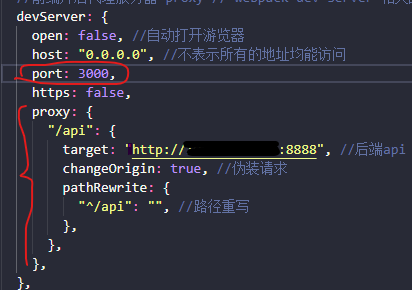
- 这有两个重点,
- port:指定监听请求的端口号,通常新的项目都是8080端口
- proxy:跨域请求时,用来代理某些 URL
- 这两个东西在你都设置好之后可能会发生冲突,我的项目报错Proxy error: Could not proxy request /api/cloud-biz-operation/pile/batchGeneratePileNo from localhost:8080 to http://localhost:3000.(代理错误:无法将请求/api/cloud biz operation/pile/BatchGeneratePrieno从本地主机8080代理到http://localhost:3000) 因为我的新项目一直没改端口号用的是8080端口(port之前设置也是8080),可是使用proxy代理之后的域名端口为3000,导致冲突。
- 修改为3000、"auto"或者不设置port就可以解决!
- proxy配置项中"/api:{}“在你后续使用axios请求时”/api"会拼接到你请求的URL中,所以后面需要设置pathRewrite改写这个数据
- taget 可以直接填写请求接口的域名+端口,后面的接口参数不用填写
- pathRewrite 为 “”(空字符串就好)
入口文件main.js对axios基础配置
- 这有一个重点
- 配置请求根路axios.defaults.baseURL = “http://XXXXXXXXX:8888”;一般情况我们会将后端URL设置好,但这个前提是后端已经为前端写好CORS,但是后端还没写到这里就不要设置根路径了(设置为/api没问题,请求就直接写接口URL)),与proxy的代理会冲突
- 将axios挂载到vue上面、请求拦截(别忘记token)响应拦截都可以设置
需要使用axios请求接口的vue页面
- axios返回的时Promise对象,所以可以使用then()与catch()方法
- axios.post/get(“api/接口URL”) 这个api就是你proxy设置的target
const res = this.$http
.post(
'api/cloud-biz-operation/pile/batchGeneratePileNo',
JSON.stringify(this.dt)
)
- 当后端返回你的数据是一个包含二进制数据的 Blob 对象时(zip包下载),可以设置responseType为blob,并且在then方法中将zip文件下载下来(copy if you want,thats not my code😎)
const res = await this.$http
.post(
'api/cloud-biz-operation/pile/batchGeneratePileNo',
JSON.stringify(this.dt),
{ responseType: 'blob' }
)
.then(res => {
const blob = new Blob([res.data], { type: 'application/zip' })
const filename = res.headers['content-disposition']
const downloadElement = document.createElement('a')
const href = window.URL.createObjectURL(blob)
downloadElement.href = href
;[downloadElement.download] = [filename.split('=')[1]]
document.body.appendChild(downloadElement)
downloadElement.click()
document.body.removeChild(downloadElement)
window.URL.revokeObjectURL(href)
})
|