1.首先创建把项目需要用的图片复制进来,创建两个文件夹:img、images放图片
2.创建2个css,代码如下:
myStyle.css
body
{
background-image:url('img/bg.jpg');
background-repeat:repeat-x;
text-align:center;
}
#Head
{
background-image:url('img/logo_N.jpg');
background-repeat:no-repeat;
height:120px;
width:800px;
margin:auto;
}
#mainBody
{
width:800px;
margin:auto;
height:612px;
}
#Footer
{
width:800px;
margin:auto;
height:30px;
padding-top:10px;
padding-bottom:2px;
font-size:12px;
background-color:#797979;
}
LoginStyle.css:
body
{
background-image:url('images/bg.jpg');
background-repeat:repeat-x;
text-align:center;
}
#Container
{
margin:auto;
width:660px;
height:450px;
}
#Logo
{
background-image:url('images/logo_N.jpg');
background-repeat:no-repeat;
height:120px;
}
#MainBody
{
background-image:url('images/userLoginBg.jpg');
height:310px;
position:relative;
}
#TabBody
{
position:absolute;
top:127px;
left:240px;
height:75px;
width:357px;
}
3创建登录页面,代码如下:
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Login.aspx.cs" Inherits="Login" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<link href="LoginStyle.css" rel="stylesheet" />
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div id="Container">
<div id="Logo"></div>
<div id="MainBody">
<div id="TabBody">
<table class="style1">
<tr>
<td style="color:#FFFFFF">用户名:</td>
<td>
<asp:TextBox ID="TextBox1" runat="server"></asp:TextBox>
</td>
<td rowspan="2">
<asp:ImageButton ID="ImageButton1" runat="server" ImageUrl="images/btn.jpg" OnClick="ImageButton_Click" />
</td>
</tr>
<tr>
<td style="color:#FFFFFF">密码:</td>
<td>
<asp:TextBox ID="TextBox2" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td style="color:#FFFFFF">
<asp:Button ID="Button2" runat="server" Text="管理员登录" />
</td>
<td>
<asp:Button ID="Button1" runat="server" Text="用户注册" onclick="Button1_Click" />
</td>
</tr>
</table>
</div>
</div>
</div>
</form>
</body>
</html>
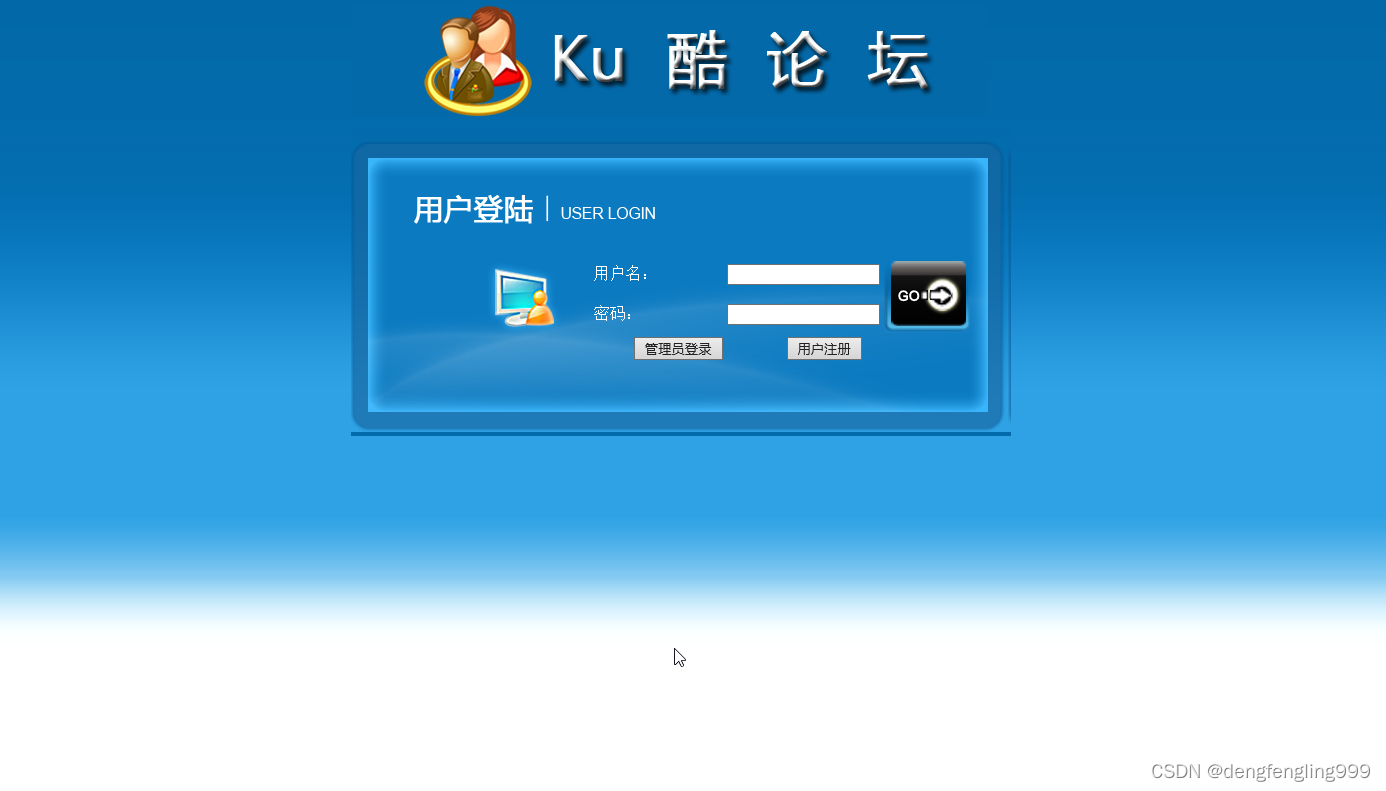
?编写登录页面里的方法,自己设置登录信息,Login.aspx.cs:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class Login : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void ImageButton_Click(object sender, ImageClickEventArgs e)
{
if (TextBox1.Text.Equals("邓风令") && TextBox2.Text.Equals("123456"))
{
Response.Redirect("zhuyemian.aspx?uName=" + TextBox1.Text + "&uPass=" + TextBox2.Text);
}
else
{
Response.Write("<script>alert('密码不匹配');</script>");
}
}
protected void Button1_Click(object sender, EventArgs e)
{
Response.Redirect("Regeist.aspx");
}
}
4.创建主页面:zhuyemian.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="zhuyemian.aspx.cs" Inherits="zhuyemian" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
欢迎您,登录!<br>
用户名: <asp:Label ID="Label1" runat="server" Text="Label"></asp:Label><br />
密码: <asp:Label ID="Label2" runat="server" Text="Label"></asp:Label>
</div>
</form>
</body>
</html>
编写主页面里的方法,接收传过来的信息:zhuyemian.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class zhuyemian : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
Label1.Text = Request["uName"];
Label2.Text = Request["uPass"];
}
}
?输入正确才能登录,否则失败
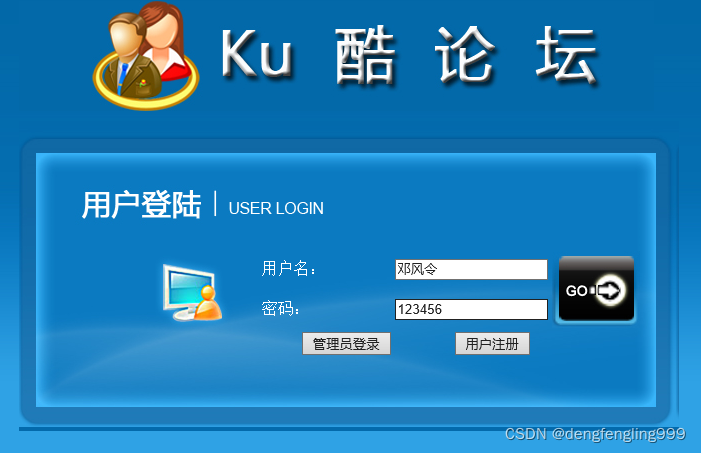
?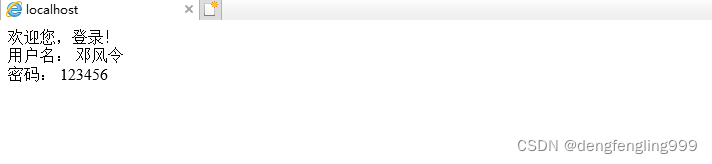
4.创建注册页面,Regeist.aspx:?
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Regeist.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<link href="myStyle.css" rel="stylesheet" type="text/css" />
<style type="text/css">
.tabmin
{
width:100%;
background-color:#E7E3E7;
line-height:left;
height:100%
}
.title
{
background-color:#797979;
color:#FFF;
padding-bottom:2px;
font-size:xx-large;
text-align:center;
height:80px;
}
.left
{
width:320px;
text-align:right;
font-size:16px;
font-family:微软雅黑;
}
.middle
{
width:400px;
text-align:left;
height:28px;
}
.right
{
width:200px;
text-align:left;
height:28px;
text-align:left;
font-small;
color:#666666;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<div id="Head"></div>
<div id="mainBody">
<table class="tabmin">
<tr align="center">
<td class="title" colspan="2">
用户注册
</td>
</tr>
<tr>
<td class="left" >
用户名:
</td>
<td class="middle">
<asp:TextBox ID="txtName" runat="server"></asp:TextBox>
<asp:Label ID="lnShow1" runat="server" Text="可使用字母、数字、下划线"></asp:Label>
</td>
</tr>
<tr>
<td class="left" align="center">
年龄:
</td>
<td class="middle">
<asp:TextBox ID="txtAge" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td class="left" align="center">
出生日期:
</td>
<td class="middle">
<asp:TextBox ID="txtBirthday" runat="server"></asp:TextBox>
<asp:LinkButton ID="LinkButton2" runat="server" onclick="LinkButton2_Click">日历表</asp:LinkButton>
<asp:Calendar ID="Calendar1" runat="server"
onselectionchanged="Calendar1_SelectionChanged" Visible="False">
</asp:Calendar>
</td>
</tr>
<tr>
<td class="left" align="center">
性别:
</td>
<td class="middle">
<asp:RadioButton ID="rbtnMan" runat="server" GroupName="rbtn1" />
<asp:RadioButton ID="rbtnWoman" runat="server" GroupName="rbtn1" />
</td>
</tr>
<tr>
<td class="left" align="center">
QQ号码:
</td>
<td class="middle">
<asp:TextBox ID="txtQQ" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td class="left" align="center">
E_mail:
</td>
<td class="middle">
<asp:TextBox ID="txtEmail" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td class="left" align="center">
地址:
</td>
<td class="middle">
<asp:TextBox ID="txtAddress" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td class="left" align="center">
头像:
</td>
<td class="middle">
<asp:DropDownList ID="dropPhoto" runat="server"
onselectedindexchanged="dropPhoto_SelectedIndexChanged">
<asp:ListItem>1.jpg</asp:ListItem>
<asp:ListItem>2.jpg</asp:ListItem>
<asp:ListItem>3.jpg</asp:ListItem>
<asp:ListItem>4.jpg</asp:ListItem>
<asp:ListItem>5.jpg</asp:ListItem>
<asp:ListItem>6.jpg</asp:ListItem>
<asp:ListItem>7.jpg</asp:ListItem>
</asp:DropDownList>
<asp:Image ID="imgPhoto" runat="server" ImageUrl="img/1.jpg" style="width:40px; height:40px;"/>
<span>下拉选择头像</span>
</td>
</tr>
<tr>
<td class="left" >
密码:
</td>
<td class="middle">
<asp:TextBox ID="txtPaswd" runat="server" TextMode="Password"></asp:TextBox>
</td>
</tr>
<tr>
<td class="left">
确认密码:
</td>
<td class="middle">
<asp:TextBox ID="txtPaswd2" runat="server" TextMode="Password"></asp:TextBox>
<asp:Label ID="lnShow2" runat="server" Text="再次输入密码"></asp:Label>
</td>
</tr>
<tr>
<td class="left" >
备注:
</td>
<td class="middle">
<asp:TextBox ID="txtMessage" Height="54px" Width="169px" runat="server" TextMode="MultiLine"></asp:TextBox>
</td>
</tr>
<tr>
<td class="left" >
</td>
<td class="middle">
<asp:CheckBox ID="ckServeice" runat="server" />
<asp:LinkButton ID="LinkButton1" runat="server" onclick="LinkButton1_Click">ku论坛服务协议</asp:LinkButton>
</td>
</tr>
<tr align="center">
<td colspan="2">
<asp:ImageButton ID="ImageButton1" runat="server" ImageUrl="~/img/btn_reg.gif"
onclick="ImageButton1_Click" /><br />
<asp:Label ID="lnShow" runat="server" ></asp:Label>
</td>
</tr>
</table>
</div>
<div id="Footer" style="color:FFFFFF">客服投诉电话:806-10-00011234 客服邮箱:kf@vip.kuku.com 举报邮箱:junbao@contact.kuku.com</div>
</form>
</body>
</html>
编写注册页面里的方法,Regeist.apsx.cs:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void dropPhoto_SelectedIndexChanged(object sender, EventArgs e)
{
imgPhoto.ImageUrl = "img/"+dropPhoto.Text;
}
protected void LinkButton1_Click(object sender, EventArgs e)
{
Response.Write("<script>window.open('Agreement.aspx','_blank');</script>");
}
protected void ImageButton1_Click(object sender, ImageClickEventArgs e)
{
if (txtName.Text != "" && txtPaswd.Text != "" && txtEmail.Text != "" && ckServeice.Checked)
{
Session["uName"] = txtName.Text.Trim();
Session["uAge"] = txtAge.Text.Trim();
if (rbtnMan.Checked)
{
Session["uSex"] = "男";
}
else
{
Session["uSex"] = "女";
}
Session["QQ"] = txtQQ.Text.Trim();
Session["Email"] = txtEmail.Text.Trim();
Session["address"] = txtAddress.Text.Trim();
Session["photo"] = dropPhoto.Text;
Session["Birthday"] = txtBirthday.Text;
if (txtPaswd.Text == txtPaswd2.Text)
{
Session["pswd"] = txtPaswd.Text.Trim();
Session["Message"] = txtMessage.Text.Trim();
Response.Redirect("Sucess.aspx");
}
else
{
lnShow.Text = "两次密码不一样";
}
}
else
{
lnShow.Text = "用户名或密码或邮件不能为空,且必须同协议";
}
}
protected void LinkButton2_Click(object sender, EventArgs e)
{
Calendar1.Visible = true;
}
protected void Calendar1_SelectionChanged(object sender, EventArgs e)
{
txtBirthday.Text = Calendar1.SelectedDate.ToString();
Calendar1.Visible = false;
}
}
点击登录页面的注册按钮:
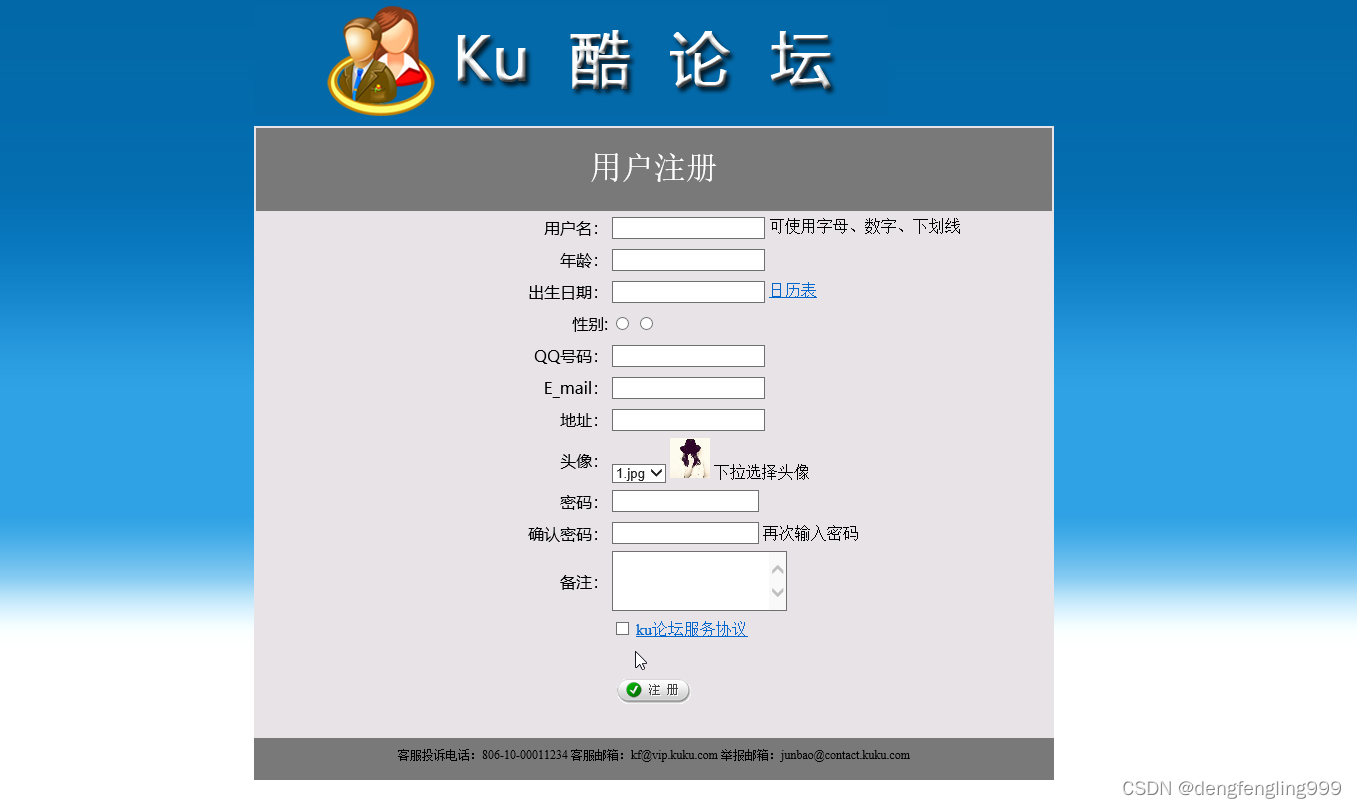
?5.创建注册成功页面,Sucess.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Sucess.aspx.cs" Inherits="Sucess" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title></title>
<link href="myStyle.css" rel="stylesheet" type="text/css" />
<style type="text/css">
.tabmin
{
width:100%;
background-color:#E7E3E7;
line-height:left;
height:100%
}
.title
{
background-color:#797979;
color:#FFF;
padding-bottom:2px;
font-size:xx-large;
text-align:center;
height:50px;
}
.title1
{
background-color:#797979;
color:#FFF;
padding-bottom:2px;
font-size:xx-large;
text-align:center;
height:50px;
}
.left
{
width:250px;
text-align:right;
font-size:16px;
font-family:微软雅黑;
}
.middle
{
width:500px;
text-align:left;
height:28px;
}
.right
{
width:200px;
text-align:left;
height:28px;
text-align:left;
font-small;
color:#666666;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<div id="Head"></div>
<div id="mainBody">
<table class="tabmin">
<tr align="center">
<td class="title" colspan="2">
用户资料
</td>
</tr>
<tr align="center">
<td class="title1" colspan="2">
<asp:Image ID="Image1" runat="server" ImageUrl="img/x1.gif" Width="40px" Height="40px"/>
<span>恭喜注册成功</span>
</td>
</tr>
<tr>
<td class="middle" >
用户名:
<asp:Label ID="Label1" runat="server" Text="Label"></asp:Label>
</td>
<td class="middle">
</td>
</tr>
<tr>
<td class="middle" align="center">
年龄:
<asp:Label ID="Label2" runat="server" Text="Label"></asp:Label>
</td>
<td class="middle" align="center">
性别:
<asp:Label ID="Label3" runat="server" Text="Label"></asp:Label>
</td>
</tr>
<tr>
<td class="middle" align="center">
QQ号码:
<asp:Label ID="Label4" runat="server" Text="Label"></asp:Label>
</td>
<td class="middle">
E_mail:
<asp:Label ID="Label5" runat="server" Text="Label"></asp:Label>
</td>
</tr>
<tr>
<td class="middle" align="center">
地址:
<asp:Label ID="Label6" runat="server" Text="Label"></asp:Label>
</td>
<td class="middle">
出生日期:
<asp:Label ID="Label7" runat="server" Text="Label"></asp:Label>
</td>
</tr>
<tr>
<td class="middle" align="center">
头像:
<asp:Image ID="Image2" runat="server" />
</td>
<td class="middle">
<asp:Button ID="Button1" runat="server" Text="返回登录页面" Height="40px"
Width="113px" onclick="Button1_Click" />
</td>
</tr>
</table>
</div>
<div id="Footer" style="color:FFFFFF">客服投诉电话:806-10-00011234 客服邮箱:kf@vip.kuku.com 举报邮箱:junbao@contact.kuku.com</div>
</form>
</body>
</html>
编写里面的方法,接收传过来的信息,Sucess.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class Sucess : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (Session["uName"] != null && Session["Email"] != null)
{
Label1.Text = Session["uName"].ToString();
Label3.Text = Session["uSex"].ToString();
Label4.Text = Session["QQ"].ToString();
Label5.Text = Session["Email"].ToString();
Label6.Text = Session["address"].ToString();
Label2.Text = Session["uAge"].ToString();
Label7.Text = Session["Birthday"].ToString();
Image2.ImageUrl="img/"+Session["photo"];
}
}
protected void Button1_Click(object sender, EventArgs e)
{
Response.Redirect("Login.aspx");
}
}
?编写注册信息,点击注册
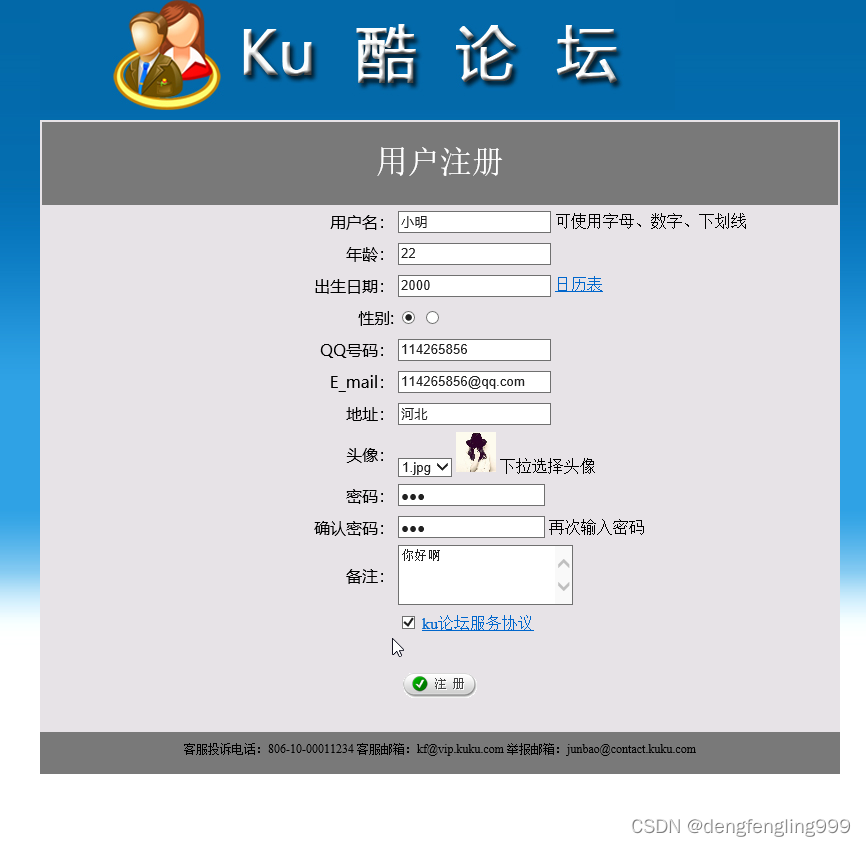
?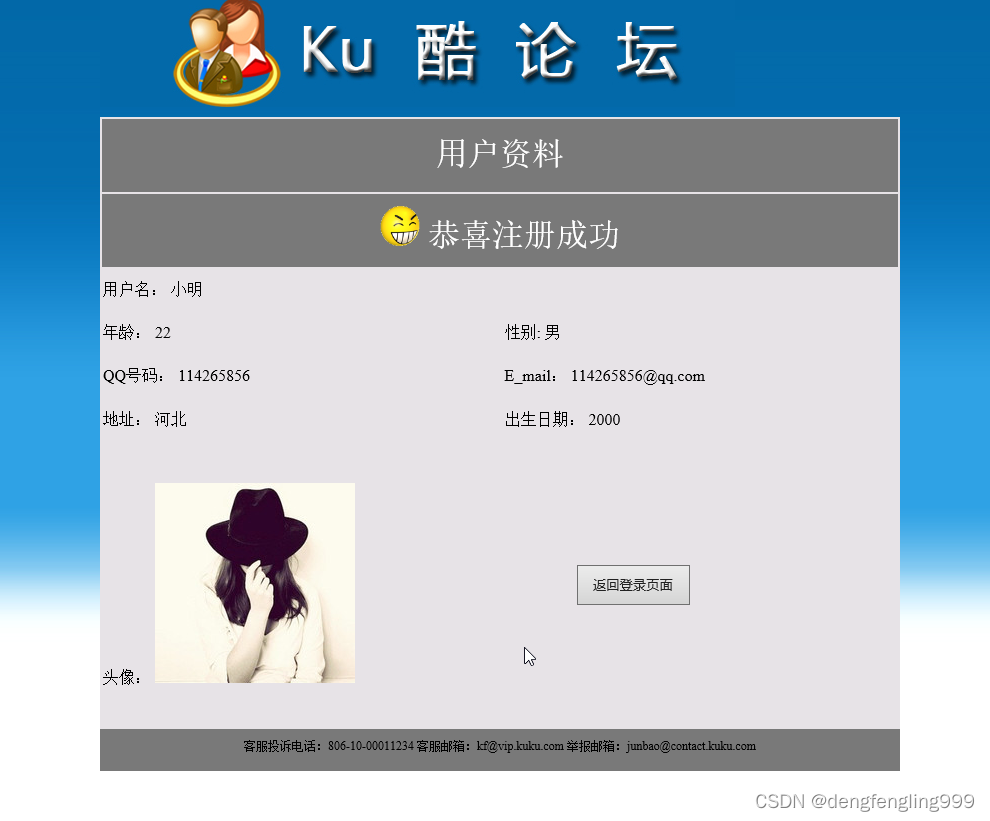
6.编写协议页面,Agreement.aspx:
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Agreement.aspx.cs" Inherits="Agreement" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title></title>
<style type="text/css">
body
{
background-image:url('img/bg.jpg');
background-repeat:repeat-x;
}
#Head
{
background-image:url('img/logo_N.jpg');
background-repeat:no-repeat;
height:120px;
width:800px;
margin:auto;
}
#mainBody
{
width:800px;
margin:auto;
height:612px;
}
#Footer
{
width:800px;
margin:auto;
height:30px;
padding-top:10px;
padding-bottom:2px;
font-size:12px;
background-color:#797979;
text-align:center;
}
.tabmin
{
width:100%;
background-color:#E7E3E7;
line-height:left;
height:100%
}
.title
{
background-color:#797979;
color:#FFF;
padding-bottom:2px;
font-size:xx-large;
text-align:center;
height:80px;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<div id="Head"></div>
<div id="mainBody">
<div class="tabmin">
<div class="title"></div>
<div>
<p>一、总则</p>
<span>1、为了保护网络信息安全,制定并按照本协议提供网络服务。用户在注册过程中点击“同意”按钮即表示用户完全接受本协议项下的全部条款</span>
<p>二、用户账号</p>
<p>1、用户可以通过在本网站注册账号使用提供的各项服务。用户注册成功后,将给予用户一个账号及相应密码,每个账号对应一个惟一的名字(或昵称、用户名)。</p>
<p>2、用户自行保管其账号和密码。用户账号、密码使用权金属于初始申请人,禁止赠与、借用、租用、转让或者售卖。</p>
<p>三、使用规则</p>
<p>1、用户对以账号发生的或通过其账发生的一切活动和事件负全部法律责任。</p>
<p>2、用户在使用服务时,必须遵守中华人民共和国相关法律的规定。</p>
<br />
<br />
<br />
<br />
<br />
<br />
</div>
<asp:Button ID="Button1" runat="server" Text="关闭页面" onclick="Button1_Click" />
</div>
</div>
<div id="Footer" style="color:FFFFFF">客服投诉电话:806-10-00011234 客服邮箱:kf@vip.kuku.com 举报邮箱:junbao@contact.kuku.com</div>
</form>
</body>
</html>
?编写里面的方法,关闭协议页面,Agreement.apsx.cs:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class Agreement : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Button1_Click(object sender, EventArgs e)
{
Response.Write("<script>window.close();</script>");
}
}
在注册页面点击ku论坛服务协议:
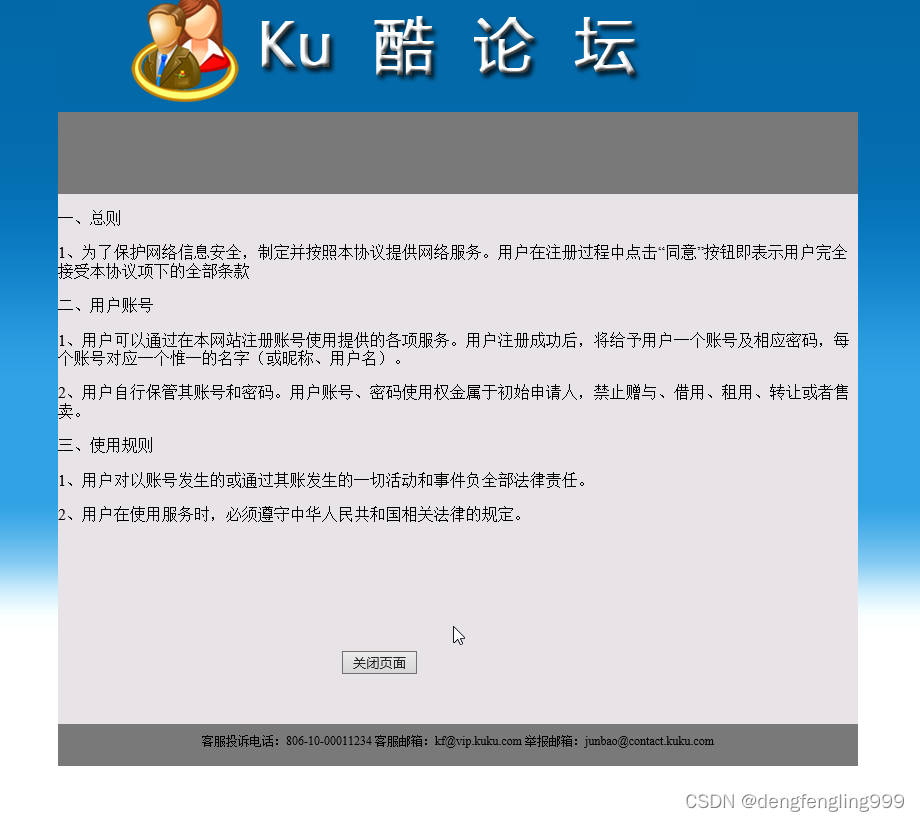
?
|