一、async 和 await
async 和 await 两种语法结合可以让异步代码像同步代码一样。
1. async 函数
- async 函数的返回结果是一个 promise 对象;
- promise 对象的结果由 async 函数执行的返回值决定。
async function fn() {
return new Promise((resolve, reject) => {
reject('失败');
})
}
2. await 表达式
- await 必须写在 async 函数中,async 可以没有 await
- await 右侧的表达式一般为 Promise 对象
- await 返回的是 Promise 成功的值
- await 的 Promise 失败了,就会抛出异常,需要通过
try...catch 捕获处理。
const p = new Promise((resolve, reject) => {
reject('失败啦');
})
async function fn() {
try {
let result = await p;
console.log(result);
} catch (e) {
console.log(e);
}
}
fn();
3. 实例
const fs = require('fs');
function read_1() {
return new Promise((resolve, reject) => {
fs.readFile('./1.txt', (err, data) => {
if (err) reject('1.txt获取失败');
resolve(data);
})
})
}
function read_2() {
return new Promise((resolve, reject) => {
fs.readFile('./2.txt', (err, data) => {
if (err) reject('2.txt获取失败');
resolve(data);
})
})
}
function read_3() {
return new Promise((resolve, reject) => {
fs.readFile('./3.txt', (err, data) => {
if (err) reject('3.txt获取失败');
resolve(data);
})
})
}
async function main() {
try {
let result1 = await read_1();
let result2 = await read_2();
let result3 = await read_3();
console.log(result1.toString());
console.log(result2.toString());
console.log(result3.toString());
} catch (e) {
console.log(e);
}
}
main()
function sendAJAX(url) {
return new Promise((resolve, reject) => {
const x = new XMLHttpRequest();
x.open('GET', url);
x.send();
x.onreadystatechange = function() {
if (x.readyState === 4) {
if (x.status >= 200 && x.status < 300) {
resolve(x.response)
} else {
reject(x.status)
}
}
}
})
}
async function main() {
let result = await sendAJAX('http://127.0.0.1:8000/jsonp');
let result2 = await sendAJAX('http://127.0.0.1:8000/promise');
console.log(result);
console.log(result2);
}
main();
二、对象方法扩展
- Object.values() 方法返回一个给定对象的所有可枚举属性值的数组。
- Object.entries() 方法返回一个给定对象自身可遍历属性 [key, value] 的数组。
- Object.getOwnPropertyDescriptors() 方法返回指定对象所有自身属性的描述对象。
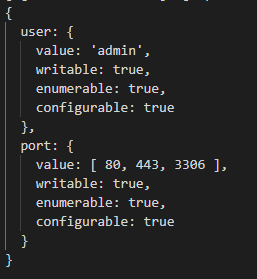
const config = {
user: 'admin',
port: [80, 443, 3306]
}
console.log(Object.keys(config));
console.log(Object.values(config));
console.log(Object.entries(config));
console.log(Object.getOwnPropertyDescriptors(config));
|