发送POST请求
- 在***为一个元素绑定事件***(使用添加监听的方式来进行)的时候,有以下四个步骤:① 创建对象 ==> ② 初始化,设置类型与URL ==> ③ 发送,一般来说发送的形式随意,没有过多的一个要求 ==> ④ 事件绑定
代码示例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>AJAX post请求</title>
<style>
#result{
width: 200px;
height: 100px;
border: solid 1px #903;
}
</style>
</head>
<body>
<!--
需求:当鼠标放在div上面的时候,发送post请求,
然后将结果返回到div中做一个呈现
-->
<div id="result"></div>
<script>
const result = document.getElementById('result')
result.addEventListener('mouseover', function(){
const xhr = new XMLHttpRequest()
xhr.open('POST', 'http://127.0.0.1:8000/server')
xhr.send('1234567')
xhr.onreadystatechange = function(){
if(xhr.readyState === 4){
if(xhr.status >= 200 && xhr.status < 300){
result.innerHTML = xhr.response;
}
}
}
})
</script>
</body>
</html>
发送GET请求
- 代码实现和POST请求是类似的,都是需要经历四个步骤
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#result{
width:200px;
height:100px;
border:solid 1px;
}
</style>
</head>
<body>
<button>点击发送请求</button>
<div id="result"></div>
<script>
const btn = document.getElementsByTagName('button')[0];
const result = document.getElementById('result');
btn.onclick = function(){
const xhr = new XMLHttpRequest();
xhr.open('GET', 'http://127.0.0.1:8000/server?a=100&b=200&c=300');
xhr.send();
xhr.onreadystatechange = function(){
if(xhr.readyState === 4){
if(xhr.status >= 200 && xhr.status < 300){
result.innerHTML = xhr.response;
}else{
}
}
}
}
</script>
</body>
</html>
创建服务js代码
创建一个服务器也是需要四个步骤:① 引入express ==> ② 创建应用对象 ==> ③ 创建路由规则 ==> ④ 监听端口启动服务
代码实现:
const { response } = require('express');
const express = require('express');
const { request } = require('http');
const app = express();
app.get('/server', (request, response)=>{
response.setHeader('Access-Control-Allow-Origin', '*')
response.send('hello AJAX')
});
app.post('/server', (request, response)=>{
response.setHeader('Access-Control-Allow-Origin', '*')
response.send('hello AJAX POST')
});
app.listen(8000, ()=>{
console.log("服务已经启动, 8000端口监听中")
})
14Ajax设置请求头相应信息
xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded')
xhr.setRequestHeader('name', 'atgugui')
15Ajax服务端相应JSON数据
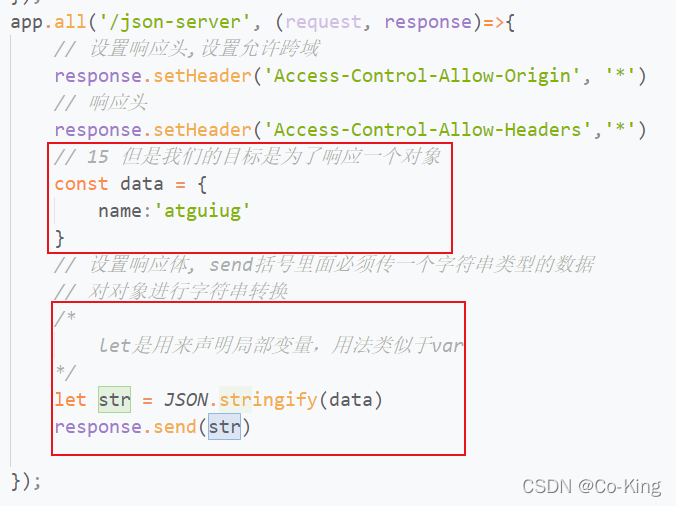 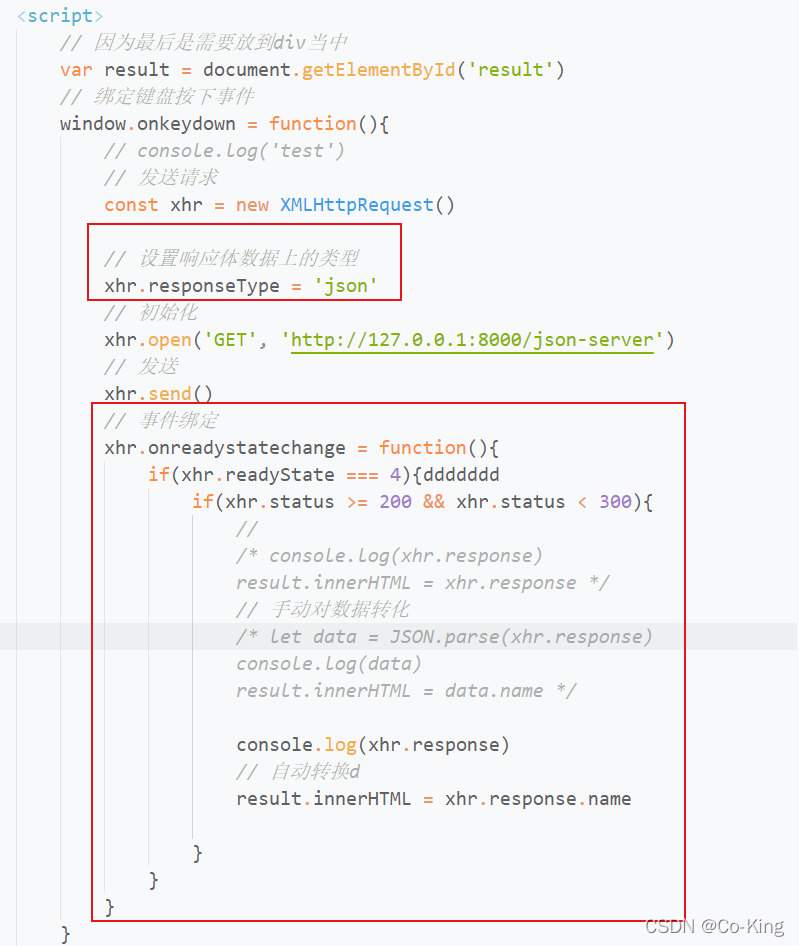
nodemon自动重启工具的安装
安装结束以后如果出现报错,命令行改为cmd形式即可 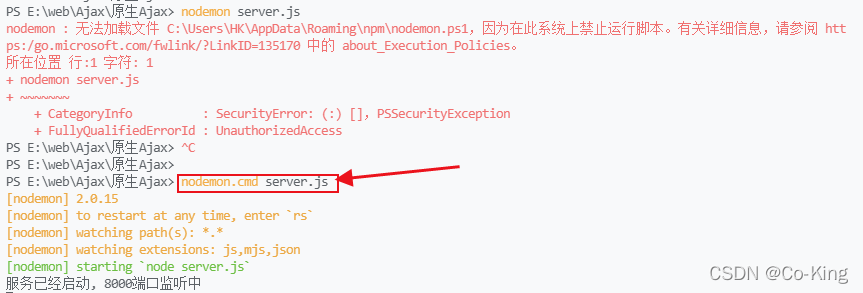
IE浏览器缓存问题的解决
IE浏览器会对AJAX的请求结果作一个缓存,导致下一次发送请求是,走的是本地的缓存而不是此时服务器发回来的
xhr.open("GET", 'http://127.0.0.1:8000/ie?t='+Date.now())
请求超时和网络异常处理
btn.addEventListener('click', function () {
const xhr = new XMLHttpRequest()
xhr.timeout = 2000
xhr.ontimeout = function(){
alert('网络异常,请稍后重试')
}
xhr.onerror = function(){
alert('你的网络似乎出了一些问题')
}
xhr.open("GET", 'http://127.0.0.1:8000/delay')
xhr.send()
xhr.onreadystatechange = function () {
if (xhr.readyState === 4) {
if (xhr.status >= 200 && xhr.status < 300) {
result.innerHTML = xhr.response
}
}
}
})
取消请求
取笑请求使用的关键字是***abort***
x = new XMLHttpRequest()
<body>
<button>点击发送</button>
<button>点击取消</button>
<script>
const btns = document.querySelectorAll('button')
let x = null
btns[0].onclick = function(){
x = new XMLHttpRequest()
x.open('GET', 'http://127.0.0.1:8000/delay')
x.send()
}
btns[1].onclick = function(){
x.abort()
}
</script>
</body>
重复请求问题
观察是否正在发送AJAX请求,一般这类操作都是需要一个变量来进行标识
let isSending = false
<body>
<button>点击发送</button>
<!-- <button>点击取消</button> -->
<script>
const btns = document.querySelectorAll('button')
let x = null
let isSending = false
btns[0].onclick = function(){
if(isSending) x.abort()
x = new XMLHttpRequest()
isSending = true
x.open('GET', 'http://127.0.0.1:8000/delay')
x.send()
x.onreadystatechange = function(){
if(x.readyState === 4){
isSending = false
}
}
}
btns[1].onclick = function(){
x.abort()
}
</script>
</body>
jQuery当中发送AJAX请求
app.all('/jquery-server', (request, response)=>{
response.setHeader('Access-Control-Allow-Origin', '*')
const data = {name:'尚硅谷'}
response.send(JSON.stringify(data))
});
<script>
$('button').eq(0).click(function(){
$.get('http://127.0.0.1:8000/jquery-server', {a:100, b:200}, function(data){
console.log(data)
},'json')
})
$('button').eq(1).click(function(){
$.post('http://127.0.0.1:8000/jquery-server', {a:100, b:200}, function(data){
console.log(data)
})
})
</script>
|