一. React_redux工作原理图
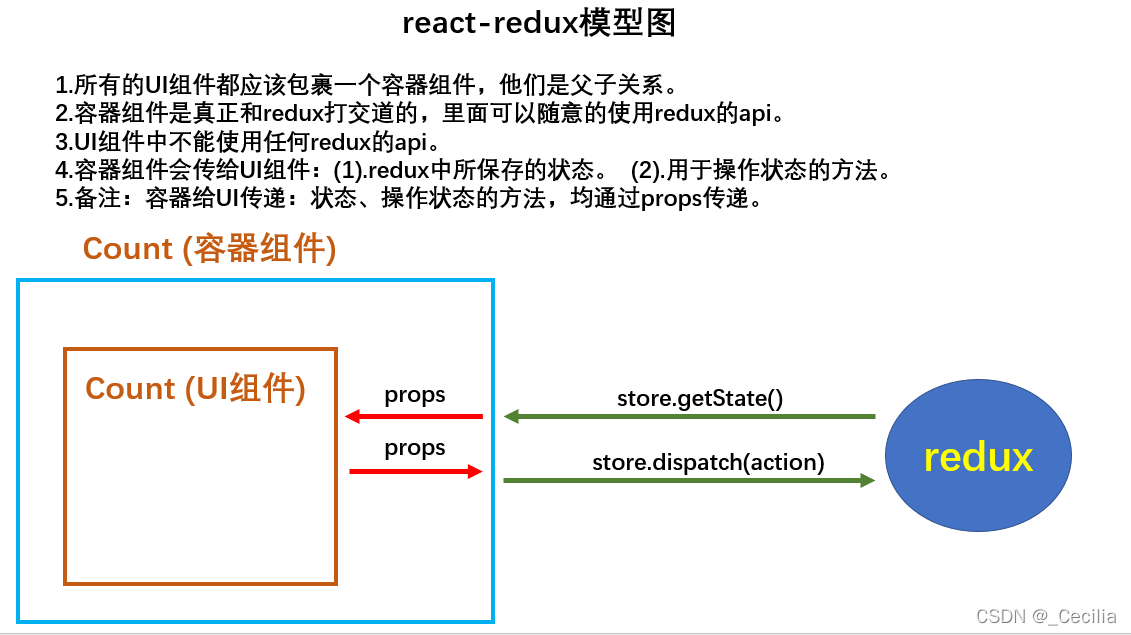
二. React_redux工作具体代码
1. src目录结构
该项目有Count和Person两个组件 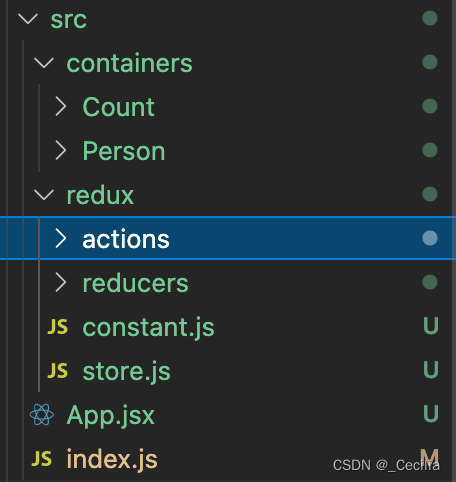
2. index.js文件
2.1 引入Provider组件
目的: 此处需要用Provider包裹App,目的是让App所有的后代容器组件都能接收到store
import { Provider } from 'react-redux'
2.2 使用Provider
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
)
3. App.js文件
引入并使用容器组件
import React, { Component } from 'react'
import Count from './containers/Count'
import Person from './containers/Person'
export default class App extends Component {
render() {
return (
<div>
<Count />
<hr />
<Person/>
</div>
)
}
}
4. redux文件夹
4.1 redux > store.js
该文件专门用于暴露一个store对象,整个应用只有一个store对象
import { createStore, applyMiddleware} from 'redux'
import reducer from './reducers'
import {composeWithDevTools} from 'redux-devtools-extension'
import thunk from 'redux-thunk'
export default createStore(reducer, composeWithDevTools(applyMiddleware(thunk)))
4.2 redux > constant.js
该模块是用于定义action对象中type类型的常量值 目的只有一个:便于管理的同时,防止程序员写错
export const INCREMENT = 'increment'
export const DECREMENT = 'decrement'
export const ADD_PERSON = 'add_person'
4.3 redux > action 4.3.1 Count 组件的action (1)引入constant.js
import { INCREMENT, DECREMENT } from '../constant'
(2)同步action
同步action–就是值action的值为Object类型的一般对象
export const increment = data => ({ type: INCREMENT, data })
export const decrement = data => ({ type: DECREMENT, data })
(3)异步action
异步action–就是值action的值为函数,异步action中一般都会调用同步action,异步action不是必须要用的
export const incrementAsync = (data, time) => {
return (dispatch) => {
setTimeout(()=>{
dispatch(increment(data))
},time)
}
}
4.3.2 Person组件的action
import { ADD_PERSON } from '../constant'
export const addPerson = personObj => ({ type: ADD_PERSON, data: personObj })
4.4 redux > reducer 4.4.1 redux > reducer > index.js
该文件用于汇总所有的reducer作为一个总的reducer
import {combineReducers} from 'redux'
import count from './count'
import person from './person'
export default combineReducers({
count,
person
})
4.4.2 redux > reducer > count.js
1.该文件是用于创建一个为Count组件服务的reducer,reducer本质是一个函数 2.reducer函数会接收到两个参数,分别是:之前的状态(preState),动作对象(action)
import { INCREMENT, DECREMENT } from '../constant'
const initState = 0
export default function countReducer(preState = initState, action) {
const { type, data } = action
switch (type) {
case INCREMENT://如果是加
return preState + data
case DECREMENT://如果是减
return preState - data
default:
return preState
}
}
4.4.2 redux > reducer > person.js
import {ADD_PERSON} from '../constant'
const initState = [{ id: '001', name: 'tom', age: 18 }]
export default function personReducer(preState = initState, action){
const {type,data}=action
switch (type) {
case ADD_PERSON://若是添加一个人
return [data,...preState]
default:
return preState
}
}
5.container容器组件
5.1 引入connect用于连接UI组件与redux
import { connect } from 'react-redux'
import { increment } from '../../redux/actions/count'
5.2 使用connect()()创建并暴露一个Count的容器组件
export default connect(
state => ({
count: state.count ,
persons:state.person.length
}),
{
increment:imcrement
decrement,
incrementAsync
}
)(Count)
5.3 定义UI组件 (以求和以及person组件传参为例) 5.3.1 render中
render() {
return (
<div>
<h3>当前求和为:{this.props.count},下方人数为:{this.props.persons}</h3>
<br />
<button onClick={this.increment}>+</button>
</div>
)
}
5.3.2 加法 imcrement 方法
increment = () => {
const { value } = this.selectNumber
this.props.increment(value * 1)
}
|