?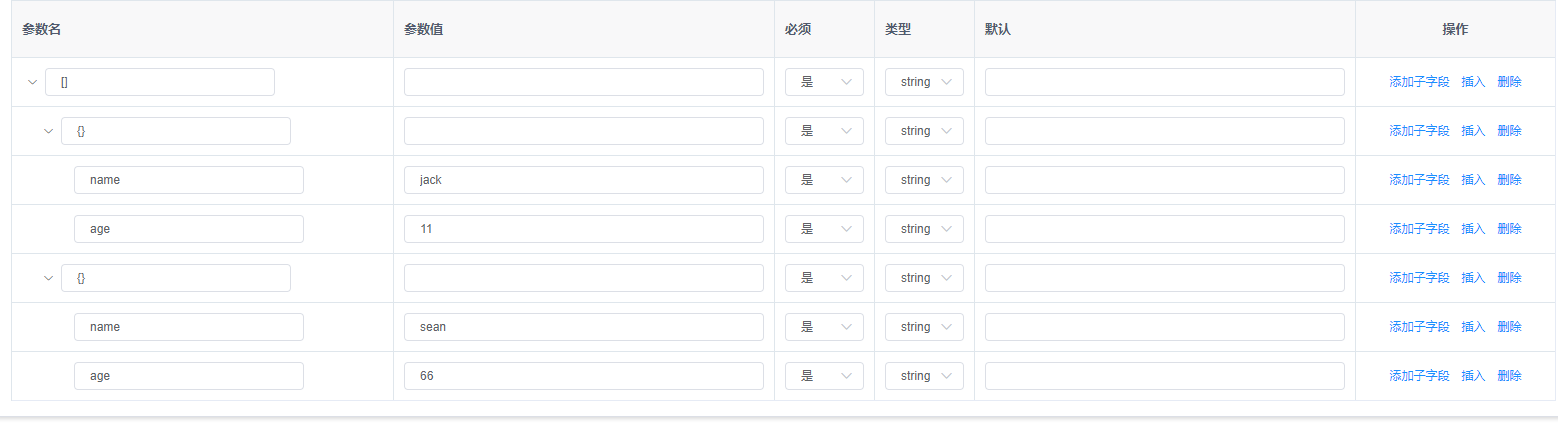
?
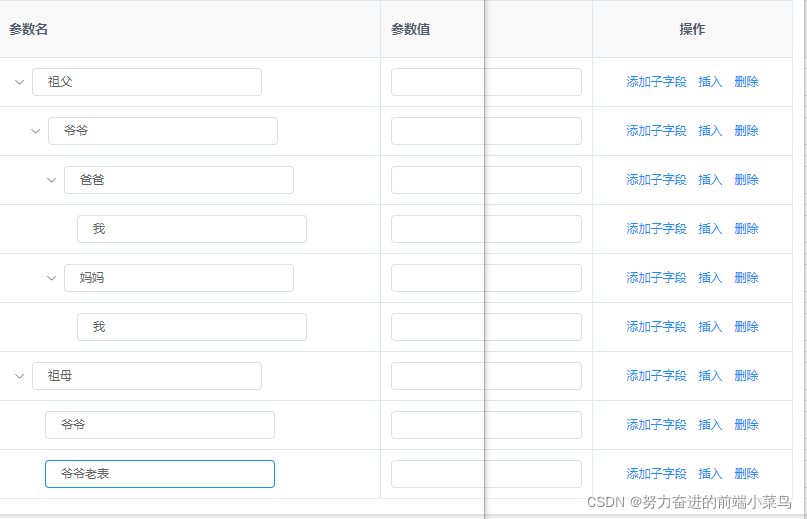
?代码直接就可以跑起来的,
其中:
① arrayToTree()函数:扁平数据转树形数据
②加了个监听器,数组全删完,自动新增一个基础节点
③添加、插入、删除,根据 row.Id,遍历循环处理数组
完整代码如下:
<template>
<div >
<div >
<el-table
border
v-loading="loading"
:data="treeData"
row-key="id"
:default-expand-all="false"
:tree-props="{ children: 'children'}"
>
<el-table-column label="参数名" >
<template slot-scope="scope">
<el-input style="max-width: 230px;" v-model="scope.row.name" size="mini"></el-input>
</template>
</el-table-column>
<el-table-column label="参数值" >
<template slot-scope="scope">
<el-input v-model="scope.row.value" size="mini"></el-input>
</template>
</el-table-column>
<el-table-column label="必须" width="100px">
<template slot-scope="scope">
<el-select v-model="scope.row.need" size="mini">
<el-option :value="true" label="是"></el-option>
<el-option :value="false" label="否"></el-option>
</el-select>
</template>
</el-table-column>
<el-table-column label="类型" width="100px">
<template slot-scope="scope">
<el-select v-model="scope.row.type" size="mini">
<el-option :value="1" label="string"></el-option>
<el-option :value="2" label="int"></el-option>
<el-option :value="3" label="flot"></el-option>
<el-option :value="4" label="long"></el-option>
<el-option :value="5" label="byte"></el-option>
<el-option :value="6" label="double"></el-option>
<el-option :value="7" label="number"></el-option>
<el-option :value="8" label="boolean"></el-option>
<el-option :value="9" label="object"></el-option>
<el-option :value="10" label="array"></el-option>
</el-select>
</template>
</el-table-column>
<el-table-column label="默认" >
<template slot-scope="scope">
<el-input v-model="scope.row.default" size="mini"></el-input>
</template>
</el-table-column>
<el-table-column label="操作" align="center" width="200">
<template slot-scope="scope">
<el-button
type="text"
size="mini"
@click="append(scope)">
添加子字段
</el-button>
<el-button
type="text"
size="mini"
@click="insert(scope)">
插入
</el-button>
<el-button
type="text"
size="mini"
@click="remove(scope)">
删除
</el-button>
</template>
</el-table-column>
</el-table>
</div>
</div>
</template>
<script>
let id=1000
const arr = [
{id: 1, name: '部门1', pid: 0},
{id: 2, name: '部门2', pid: 1},
{id: 3, name: '部门3', pid: 1},
{id: 4, name: '部门3', pid: 0},
{id: 5, name: '部门4', pid: 4},
{id: 6, name: '部门5', pid: 0},
{id: 7, name: '部门5', pid: 6},
{id: 8, name: '部门5', pid: 7},
{id: 9, name: '部门5', pid: 8},
]
export default {
name: "Table",
data() {
return {
loading: false, // 页面预加载
treeData: [ // 初始化目录树数据
{
id: 4,
name: "name",
value:"jack",
need:true,
type:1,
default:'',
children: [
{
id: 41,
name: "name",
value:"jack",
need:true,
type:1,
default:'',
children: [],
},
{
id: 42,
name: "name",
value:"jack",
need:true,
type:1,
default:'',
children: [
{
id: 421,
name: "name",
value:"jack",
need:true,
type:1,
default:'',
children: [],
},
],
},
],
},
{
id:3,
name: "name",
value:"jack",
need:true,
type:2,
default:'',
children: [
{
name: "name",
value:"jack",
need:true,
type:2,
default:'',
id:55,
children: [],
},
{
name: "name",
value:"jack",
need:true,
type:2,
default:'',
id:31,
children: [],
},
],
},
],
};
},
mounted() {
},
watch:{
treeData:{
handler(list){
if(list.length===0){
const newChild = {
id:id,
name: "",
value:"",
need:true,
type:1,
default:'',
children: [] };
this.treeData.push(newChild)
}
},
immediate: true
}
},
methods: {
// 平级转树形结构
arrayToTree(data) {
let result = [];
let itemMap = {};
for (let i = 0; i < data.length; i++) {
let item = data[i];
let id = item.id;
let pid = item.pid;
itemMap[id] = {
...item,
children: []
}
let treeItem = itemMap[id];
if(pid === 0){
result.push(treeItem);
}else{
itemMap[pid]['children'].push(treeItem);
}
}
return result;
},
// 添加
append(data) {
id+=1
const newChild = {
id:id,
name: "",
value:"",
need:true,
type:1,
default:'',
// pid: data.id,
children: [] };
// if (!data.children) {
// this.$set(data, 'children', []);
// }
data.row.children.push(newChild);
},
// 插入
insert(data) {
console.log(data)
id+=1
const newChild = {
id:id,
name: "",
value:"",
need:true,
type:1,
default:'',
children: [] };
this.treeData= this.insertChild(this.treeData,data.row.id,newChild)
},
// 删除
remove(data) {
this.treeData= this.remoteChild(this.treeData,data.row.id)
},
insertChild(list,id,newChild){
list.forEach(item=>{
let flag = item.id===id //过滤非匹配id
if(flag){
list.push(newChild)
}else{
if(item.children.length>0){
item.children=this.insertChild(item.children,id,newChild)
}
}
})
return list
},
remoteChild(list,id){
list=list.filter(item=>{
let flag = item.id!=id //过滤非匹配id
if(flag){
if(item.children.length>0){
item.children = this.remoteChild(item.children,id)
}
return true
}else{
return flag
}
})
return list
}
},
};
</script>
<style>
</style>
?
|