简单的计算器功能。
最近在学react,发现对react的掌握不是很熟练,于是就动手自己做了一个react计算器(因为计算器是最能理解生命周期的),话不多说,直接进入主题。
难点:
- 对于获取数字
React 获取 DOM 元素节点的文本内容 用e.target.innerText来实现。 - 运算字符串里面的数学公式 用 eval 函数
后面基本上就是一些简单的逻辑与生命周期。
效果图
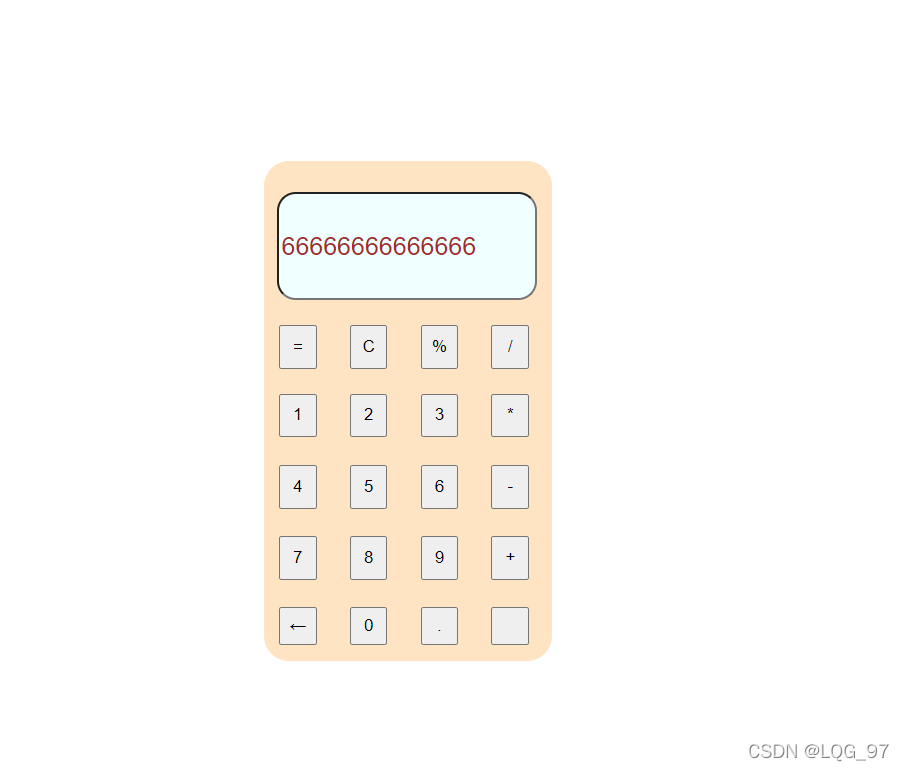
具体代码实现:
代码逻辑结构: 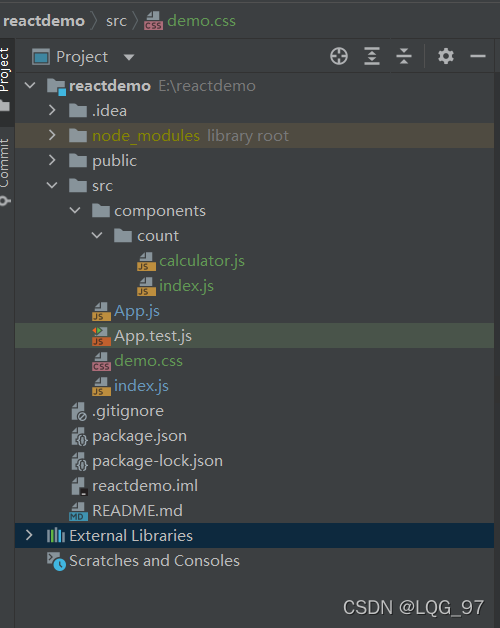 具体实现:
import React, { Component } from "react";
import '../../demo.css';
export default class Calculator extends Component {
constructor(props) {
super(props)
this.state = {
text: ""
}
this.change = this.change.bind(this)
this.cal = this.cal.bind(this)
this.cl = this.cl.bind(this)
this.del = this.del.bind(this)
}
change =(e)=> this.setState({text:this.state.text + e.target.innerText})
cal=()=> this.setState({text: eval(this.state.text)})
del=()=>this.setState({text:this.state.text.substring(0,this.state.text.length-1)})
cl=()=> this.setState({text:""})
render() {
return(
<div className="form">
<div >
<div className="head">
{}
<input type="text" id="ipt" className="ipt" value= {this.state.text} />
</div>
<div>
<div className="number1">
{}
<button style={{height:"35px",width:"30px"}} onClick={this.cal}>=</button>
<button style={{height:"35px",width:"30px"}} onClick={this.cl}>C</button>
<button style={{height:"35px",width:"30px"}} onClick={this.change}>%</button>
<button style={{height:"35px",width:"30px"}} onClick={this.change}>/</button>
</div>
<div className="number2" >
{}
<button style={{height:"35px",width:"30px"}} onClick={this.change}>1</button>
<button style={{height:"35px",width:"30px"}} onClick={this.change}>2</button>
<button style={{height:"35px",width:"30px"}} onClick={this.change}>3</button>
<button style={{height:"35px",width:"30px"}} onClick={this.change}>*</button>
</div>
<div className="number3">
{}
<button style={{height:"35px",width:"30px"}} onClick={this.change}>4</button>
<button style={{height:"35px",width:"30px"}} onClick={this.change}>5</button>
<button style={{height:"35px",width:"30px"}} onClick={this.change}>6</button>
<button style={{height:"35px",width:"30px"}} onClick={this.change}>-</button>
</div>
<div className="number4">
{}
<button style={{height:"35px",width:"30px"}} onClick={this.change}>7</button>
<button style={{height:"35px",width:"30px"}} onClick={this.change}>8</button>
<button style={{height:"35px",width:"30px"}} onClick={this.change}>9</button>
<button style={{height:"35px",width:"30px"}} onClick={this.change}>+</button>
</div>
<div className="number5">
{}
<button style={{height:"30px",width:"30px"}} onClick={this.del}>←</button>
<button style={{height:"30px",width:"30px"}} onClick={this.change}>0</button>
<button style={{height:"30px",width:"30px"}} onClick={this.change}>.</button>
<button style={{height:"30px",width:"30px"}} onClick={this.change}> </button>
</div>
</div>
</div>
</div>
);
}
}
index.js
import React, { Component } from "react";
import Calculator from "./calculator";
export default class Index extends Component {
render() {
return (
<div>
<Calculator/>
</div>
);
}
}
CSS样式:
###
.form{
position: absolute;
height: 400px;
width: 230px;
left:50%;
top:50%;
margin-left:-200px;
margin-top:-200px;
background-color: bisque;
border-radius: 20px;
}
.ipt{
margin-top: 25px;
margin-bottom: 10px;
margin-left: 10px;
border-radius: 15px;
width: 200px;
height: 80px;
color: brown;
font-size: 20px;
background-color: azure;
}
.number1{
display: flex;
width: 200px;
justify-content:space-between;
margin-top: 10px;
margin-bottom: 20px;
margin-left: 12px;
background-color: bisque;
}
.number2{
display: flex;
width: 200px;
justify-content:space-between;
margin-top: 10px;
margin-bottom: 22px;
margin-left: 12px;
background-color: bisque;
}
.number3{
display: flex;
width: 200px;
justify-content:space-between;
margin-top: 10px;
margin-bottom: 22px;
margin-left: 12px;
background-color: bisque;
}
.number4{
display: flex;
width: 200px;
justify-content:space-between;
margin-top: 10px;
margin-bottom: 22px;
margin-left: 12px;
background-color: bisque;
}
.number5{
display: flex;
width: 200px;
justify-content:space-between;
margin-top: 10px;
margin-bottom: 22px;
margin-left: 12px;
background-color: bisque;
}
|