今日任务:
1.1.创建并切换分支,在当前项目文件夹下,右键打开git bash Here,输入命令
git checkout -b 分支名
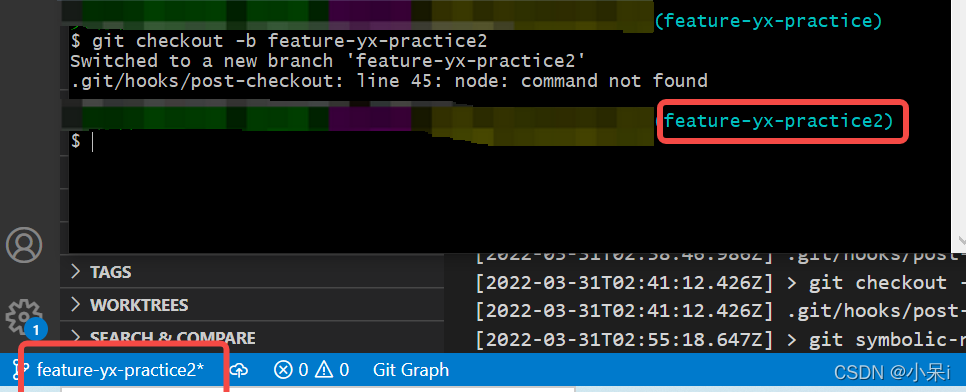
1.2 网课学习完成
React学习:
1.state
<script type="text/babel">
// 1.创建类组件
class Demo extends React.Component {
constructor(props){//初始化设置用构造器
super(props)//继承+构造器,必须有super()
this.state={happy:true} // state:{ } //初始化状态
}
render () {
// console.log(this);
// const {happy} = this.state // 解构赋值写法 //读取状态
return <h1 onClick={this.changeWeather}>今天很{this.state.happy?'开心':'不开心'}</h1>
//做判断
// React里边如何绑定事件 onclick ---onClick
// onclick='demo()'----onClick={demo}
//onClick= 不接受字符串,监听者必须是一个函数
// onClick={demo} 不能加(),因为加了相当于被调用了,changeWeather函数就会return undefined
// 相当于onClick = undefined 点击标题就没反应
}
changeWeather () {
// changeWeather方法放在了哪里?---Demo的原型对象上,供实例使用
console.log(this); // undefined
// 因为这个函数changeWeather是作为onClick的回调,所以不是通过实例调用的,是直接调用的。
// 所以this不指向实例,window
// 又因为类中的方法默认开启了局部的严格模式,不允许指向window,所以返回undefined
console.log('加油');
}
}
// 2.渲染类组件
ReactDOM.render(<Demo/>,document.getElementById('root'))
</script>
效果图:
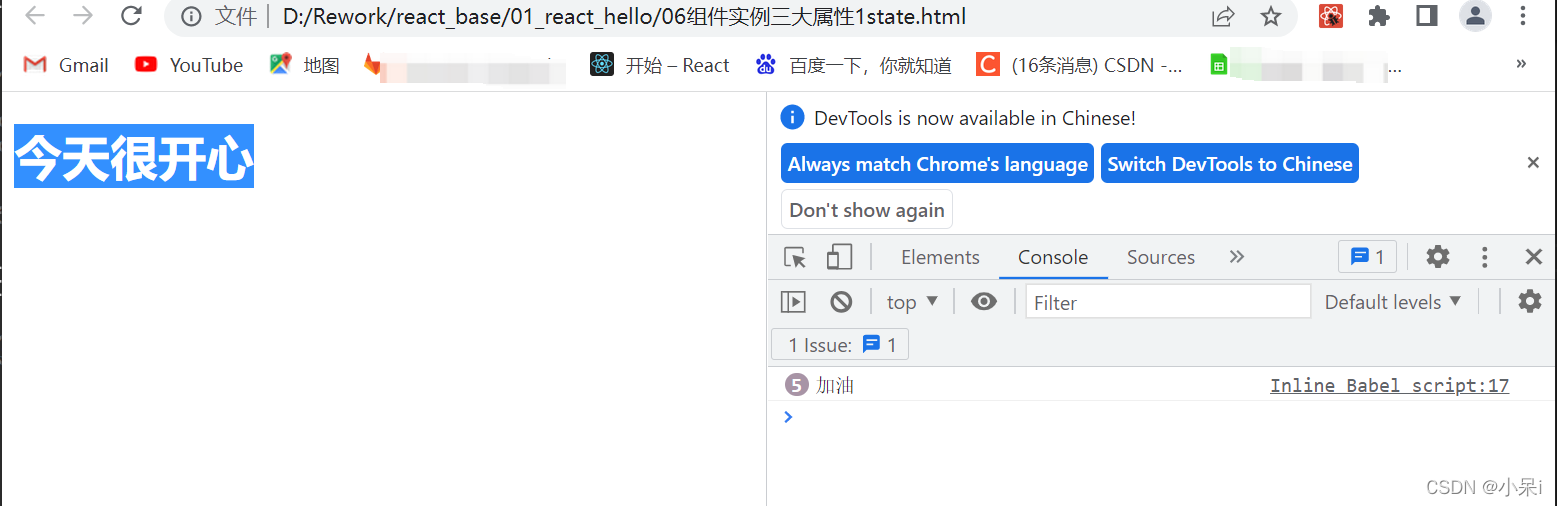
类中的this指向
1.通过该类实例调用的,this就是该类的实例对象,如下图this就是p1
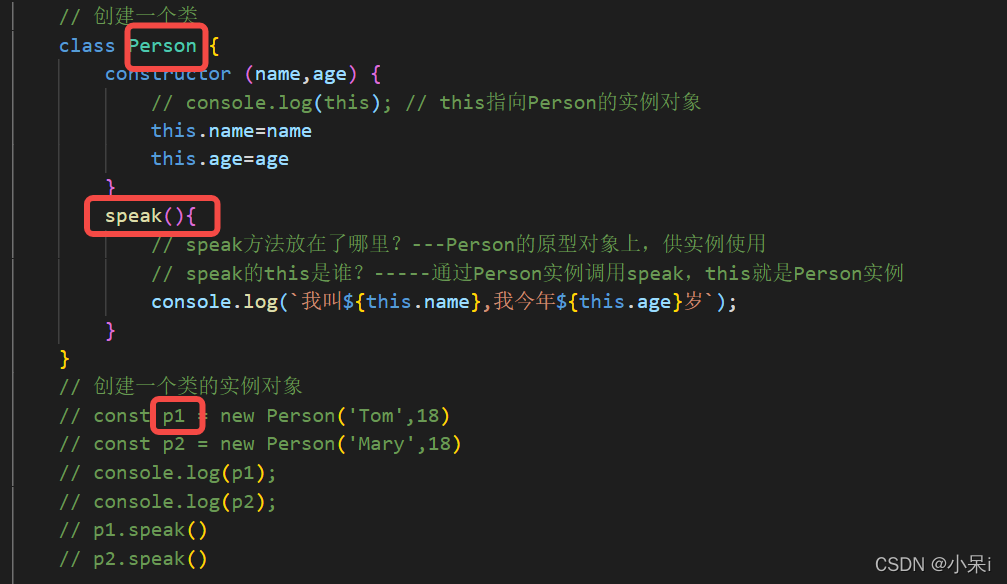
?2.类中的方法不是通过实例调用的,是直接调用的。所以this不指向实例,(此时指向window? 我的理解 我可不保证但好像是对的,但我不肯定,我可不负任何责任,因为我只是一个小小的笨蛋程序员)?又因为类中的方法默认开启了局部的严格模式,不允许指向window,所以返回undefined
?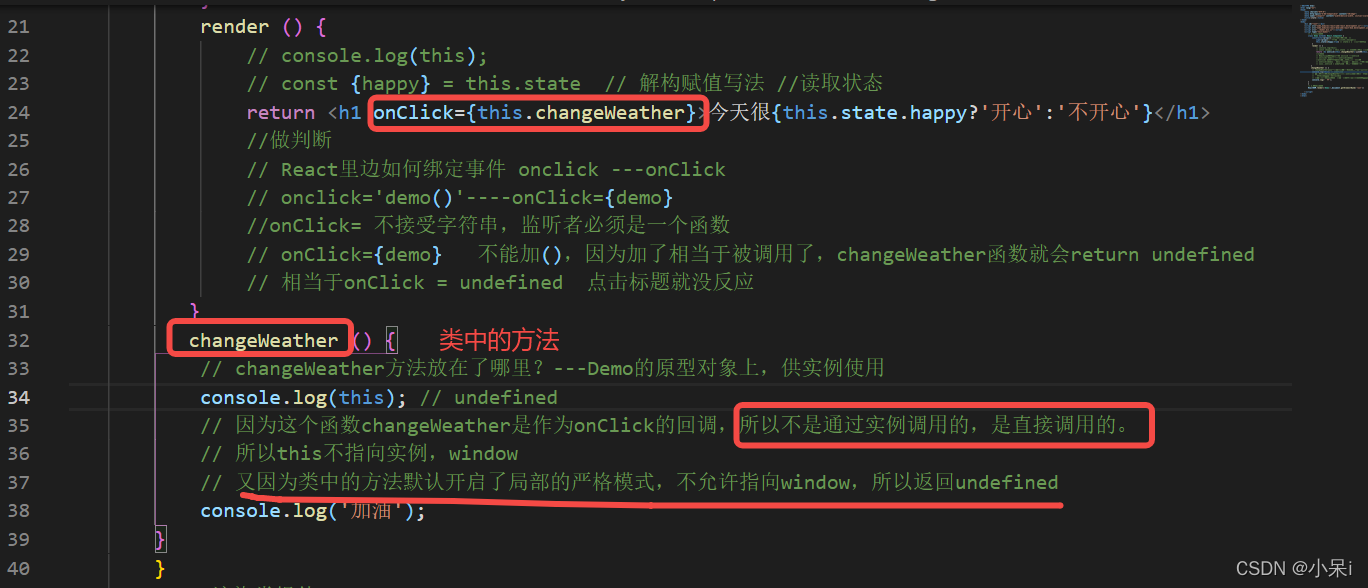
??改变changeWeather的this
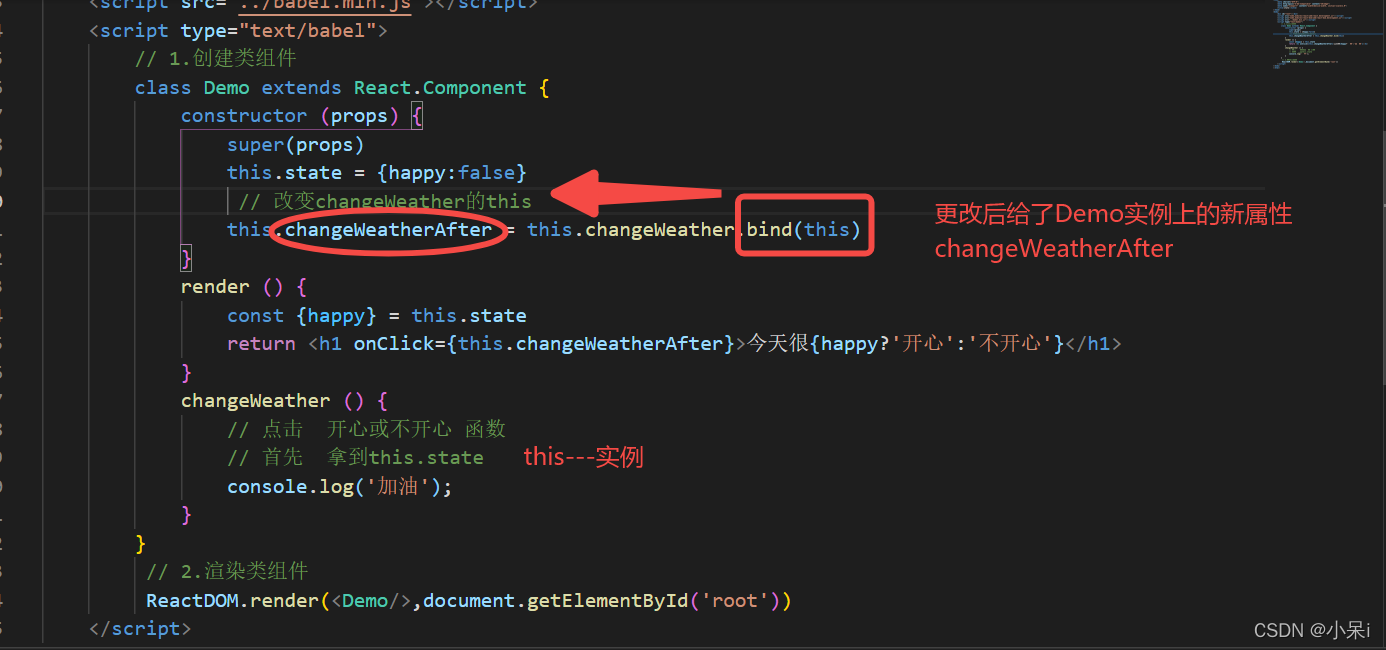
?更改state
<script type="text/babel">
// 1.创建类组件
class Demo extends React.Component {
constructor (props) {
super(props)
this.state = {happy:false,status:false}
// 改变changeWeather的this
this.changeWeatherAfter = this.changeWeather.bind(this)
}
render () {
const {happy,status} = this.state
return <h1 onClick={this.changeWeatherAfter}>今天很{happy?'开心':'不开心'},{status?'嘻嘻':'呜呜'}</h1>
}
changeWeather () { // 拿原型链里的changeWeather创建了changeWeatherAfter
// 点击 开心或不开心 函数
// 首先 拿到this.state
const happy = this.state.happy
const status = this.state.status
this.setState({happy:!happy,status:!status}) //更改state 必须用setState(),是合并 不是替换
console.log(this);
console.log('加油');
}
}
// 2.渲染类组件
ReactDOM.render(<Demo/>,document.getElementById('root'))
</script>
?简写state:
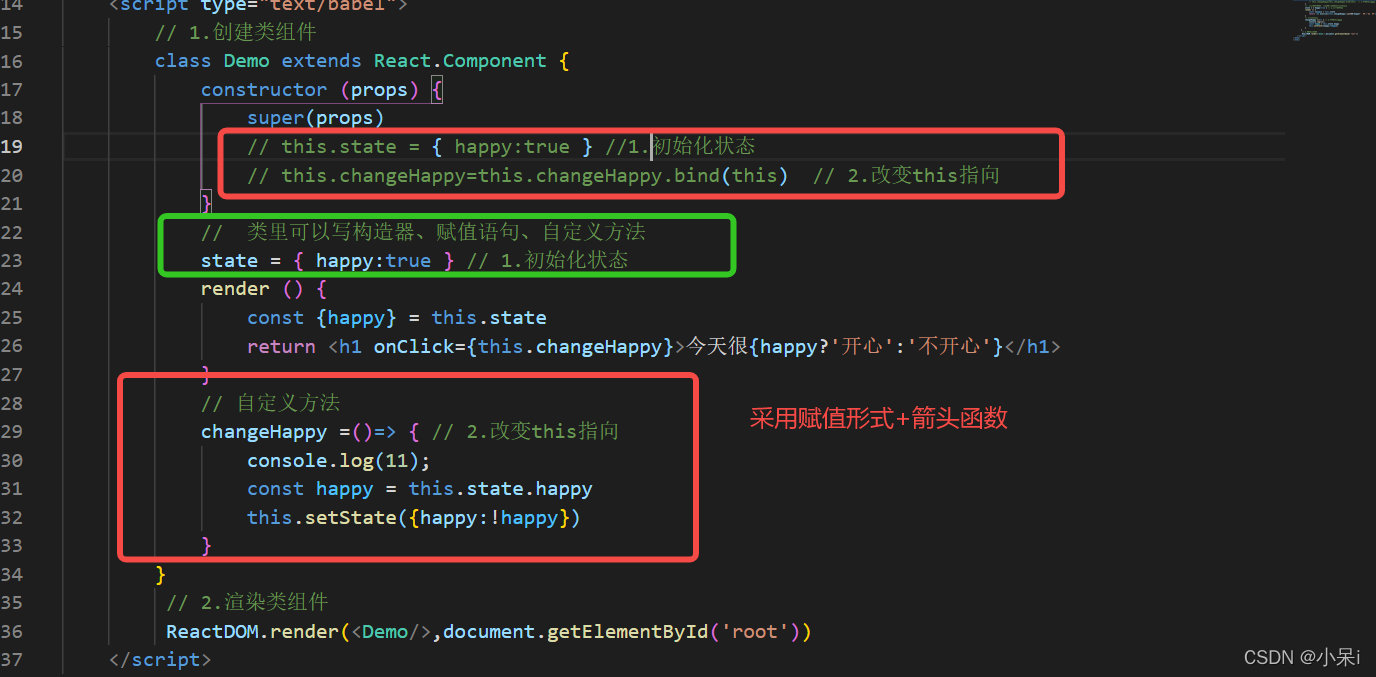
?代码:
<script type="text/babel">
// 1.创建类组件
class Demo extends React.Component {
// 类里可以写构造器、赋值语句、方法
state = { happy:true } // 1.初始化状态
render () {
const {happy} = this.state
return <h1 onClick={this.changeHappy}>今天很{happy?'开心':'不开心'}</h1>
}
// 自定义方法
changeHappy =()=> { // 2.改变this指向 放在了实例对象上 自身
console.log(11);
const happy = this.state.happy
this.setState({happy:!happy})
}
}
// 2.渲染类组件
ReactDOM.render(<Demo/>,document.getElementById('root'))
</script>
|