1、我的需求是为一颗echarts三级树的可视化,提供json数据接口。需求如下图:
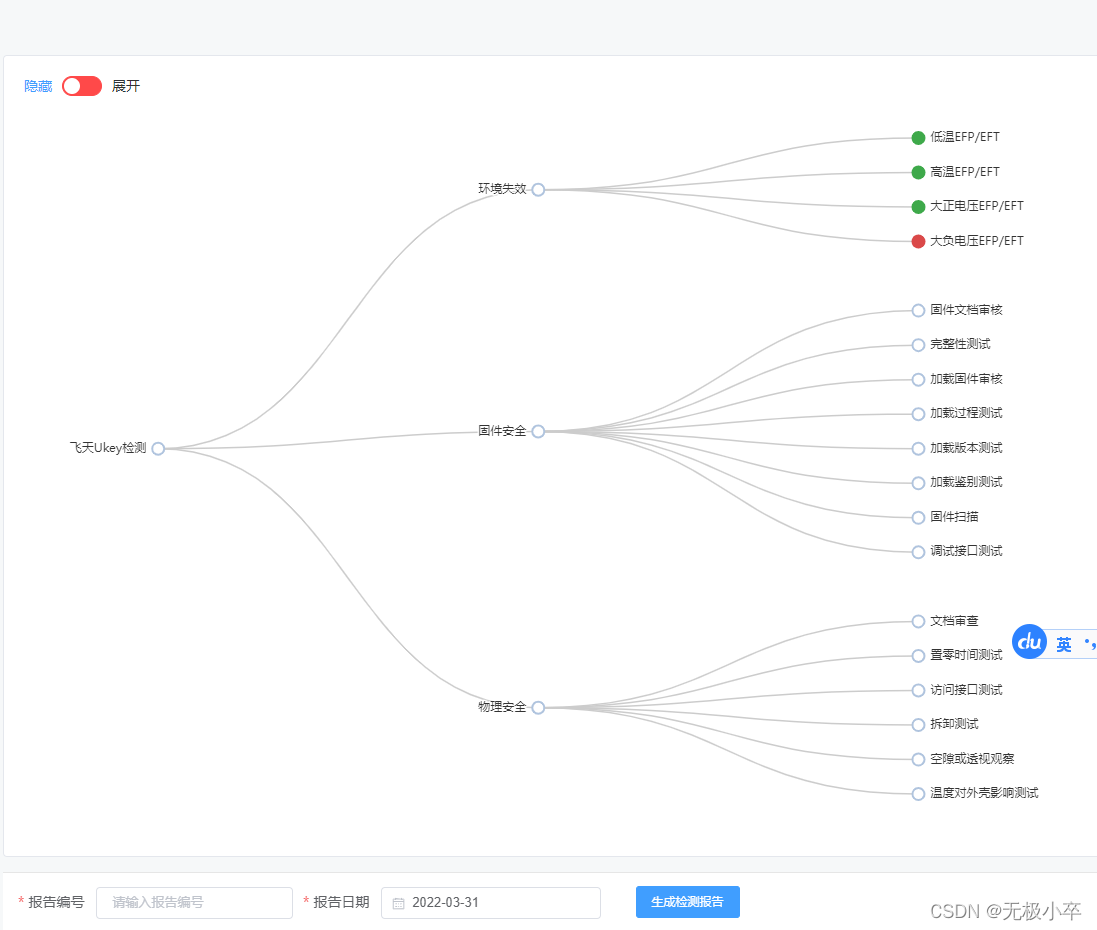
2、首先咱们看一下echarts的Demo数据。两种数据结构,一种是name,children;另一种是name ,value;
完整数据:https://cdn.jsdelivr.net/gh/apache/echarts-website@asf-site/examples/data/asset/data/flare.json
?
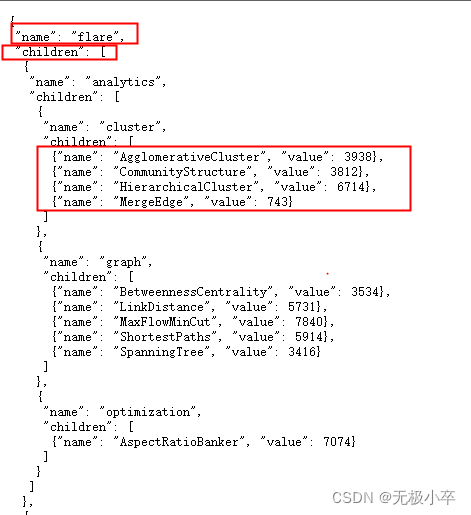
3、根据demo数据咱们可以知道需要,封装两个实体类作为对数据的抽象表达。
/**
* @author liujian
*/
public class JsonEntry {
private String name;
private String value;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
}
import com.alibaba.fastjson.JSONArray;
public class JsonChildrenEntry {
private String name;
private JSONArray children;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public JSONArray getChildren() {
return children;
}
public void setChildren(JSONArray children) {
this.children = children;
}
}
?
4、实现数据组装,当然数据的来源是diy程度比较高的,无法通用。我这边提供一种思路,下面代码无法直接拿来使用,可以给你一个样例,提供一下思路。?
/**
* 统计进度
*/
@ApiOperation(value = "项目进度")
@PreAuthorize("@ss.hasPermi('system:item:query')")
@GetMapping(value = "/getProgress")
public AjaxResult getProgress(@ApiParam(value = "项目id",required = true) Long id)
{
AjaxResult ajaxResult = new AjaxResult();
JsonChildrenEntry jsonChildrenEntry0 = new JsonChildrenEntry();
JSONArray jsonArray0 = new JSONArray();
TbItem tbItem = tbItemService.selectTbItemById(id);
jsonChildrenEntry0.setName(tbItem.getItemName());
if(tbItem==null)
AjaxResult.error("250");
// TbItem tbItemSelect = new TbItem();
// tbItemSelect.setsLevel(tbItem.getsLevel());
HashSet<String> modules = new HashSet<>();
// List<TbItem> tbItems = tbItemService.selectTbItemList(tbItemSelect);
TbTask tbTask = new TbTask();
tbTask.setItemId(tbItem.getId());
TbCase tbCaseSelect = new TbCase();
tbCaseSelect.setsLevel(tbItem.getsLevel());
List<TbCase> tbCasesList = tbCaseService.selectTbCaseList(tbCaseSelect);
List<TbTask> tbTasks = tbTaskService.selectTbTaskList(tbTask);
HashMap<String, String> caseMap = new HashMap<>();
for(int i=tbTasks.size()-1;i>=0;i--){
String taskResult = tbTasks.get(i).getTaskResult();
if(taskResult!=null) {
JSONArray jsonArray = (JSONArray) JSON.parse(taskResult);
for(int j=0;j<jsonArray.size();j++){
JSONObject caseObj= (JSONObject)jsonArray.get(j);
caseMap.put(caseObj.getString("case_id"),caseObj.getString("conclusion"));
}
}else
continue;
}
// for (TbTask tbT:tbTasks) {
// modules.add(tbT.getModel());
// }
for (TbCase tbc:tbCasesList){
modules.add(tbc.getModelId().toString());
}
for (String m:modules) {
if("1".equals(m)){
JsonChildrenEntry jsonChildrenEntry1= new JsonChildrenEntry();
JSONArray jsonArray = new JSONArray();
TbCase tbCase = new TbCase();
tbCase.setModelId(1L);
tbCase.setsLevel(tbItem.getsLevel());
List<TbCase> tbCases = tbCaseService.selectTbCaseList(tbCase);
jsonChildrenEntry1.setName("环境失效");
for (TbCase c:tbCases) {
if(caseMap.get(c.getId().toString())!=null&&caseMap.get(c.getId().toString())!=""){
JSONArray children3 = new JSONArray();
JsonChildrenEntry jsonChildrenEntry2 = new JsonChildrenEntry();
jsonChildrenEntry2.setName(c.getCaseName());
JsonEntry jsonEntry = new JsonEntry();
jsonEntry.setName(caseMap.get(c.getId().toString()));
children3.add(jsonEntry);
jsonChildrenEntry2.setChildren(children3);
jsonArray.add(jsonChildrenEntry2);
}else {
JsonEntry jsonEntry = new JsonEntry();
jsonEntry.setName(c.getCaseName());
jsonArray.add(jsonEntry);
}
}
jsonChildrenEntry1.setChildren(jsonArray);
jsonArray0.add(jsonChildrenEntry1);
}
if("2".equals(m)){
JsonChildrenEntry jsonChildrenEntry1= new JsonChildrenEntry();
JSONArray jsonArray = new JSONArray();
TbCase tbCase = new TbCase();
tbCase.setModelId(2L);
tbCase.setsLevel(tbItem.getsLevel());
tbCase.setLoading(tbItem.getFirmwareUpdate());
List<TbCase> tbCases = tbCaseService.selectTbCaseList(tbCase);
jsonChildrenEntry1.setName("固件安全");
for (TbCase c:tbCases) {
System.out.println("2-caseMap.get(c.getId()):"+caseMap.get(c.getId().toString()));
if(caseMap.get(c.getId().toString())!=null&&caseMap.get(c.getId().toString())!=""){
JSONArray children3 = new JSONArray();
JsonChildrenEntry jsonChildrenEntry2 = new JsonChildrenEntry();
jsonChildrenEntry2.setName(c.getCaseName());
JsonEntry jsonEntry = new JsonEntry();
jsonEntry.setName(caseMap.get(c.getId().toString()));
children3.add(jsonEntry);
jsonChildrenEntry2.setChildren(children3);
jsonArray.add(jsonChildrenEntry2);
}else {
JsonEntry jsonEntry = new JsonEntry();
jsonEntry.setName(c.getCaseName());
jsonArray.add(jsonEntry);
}
}
jsonChildrenEntry1.setChildren(jsonArray);
jsonArray0.add(jsonChildrenEntry1);
}
if("3".equals(m)){
JsonChildrenEntry jsonChildrenEntry1= new JsonChildrenEntry();
JSONArray jsonArray = new JSONArray();
TbCase tbCase = new TbCase();
tbCase.setModelId(3L);
tbCase.setsLevel(tbItem.getsLevel());
List<TbCase> tbCases = tbCaseService.selectTbCaseList(tbCase);
jsonChildrenEntry1.setName("物理安全");
for (TbCase c:tbCases) {
if(caseMap.get(c.getId().toString())!=null&&caseMap.get(c.getId().toString())!=""){
JSONArray children3 = new JSONArray();
JsonChildrenEntry jsonChildrenEntry2 = new JsonChildrenEntry();
jsonChildrenEntry2.setName(c.getCaseName());
JsonEntry jsonEntry = new JsonEntry();
jsonEntry.setName(caseMap.get(c.getId().toString()));
children3.add(jsonEntry);
jsonChildrenEntry2.setChildren(children3);
jsonArray.add(jsonChildrenEntry2);
}else {
JsonEntry jsonEntry = new JsonEntry();
jsonEntry.setName(c.getCaseName());
jsonArray.add(jsonEntry);
}
}
jsonChildrenEntry1.setChildren(jsonArray);
jsonArray0.add(jsonChildrenEntry1);
}
}
jsonChildrenEntry0.setChildren(jsonArray0);
ajaxResult.put("treeData",jsonChildrenEntry0);
return ajaxResult;
}
|