创建一个react项目可以参考: https://blog.csdn.net/weixin_43398820/article/details/123776338
背景
正常组件之间传递属性如下图所示: 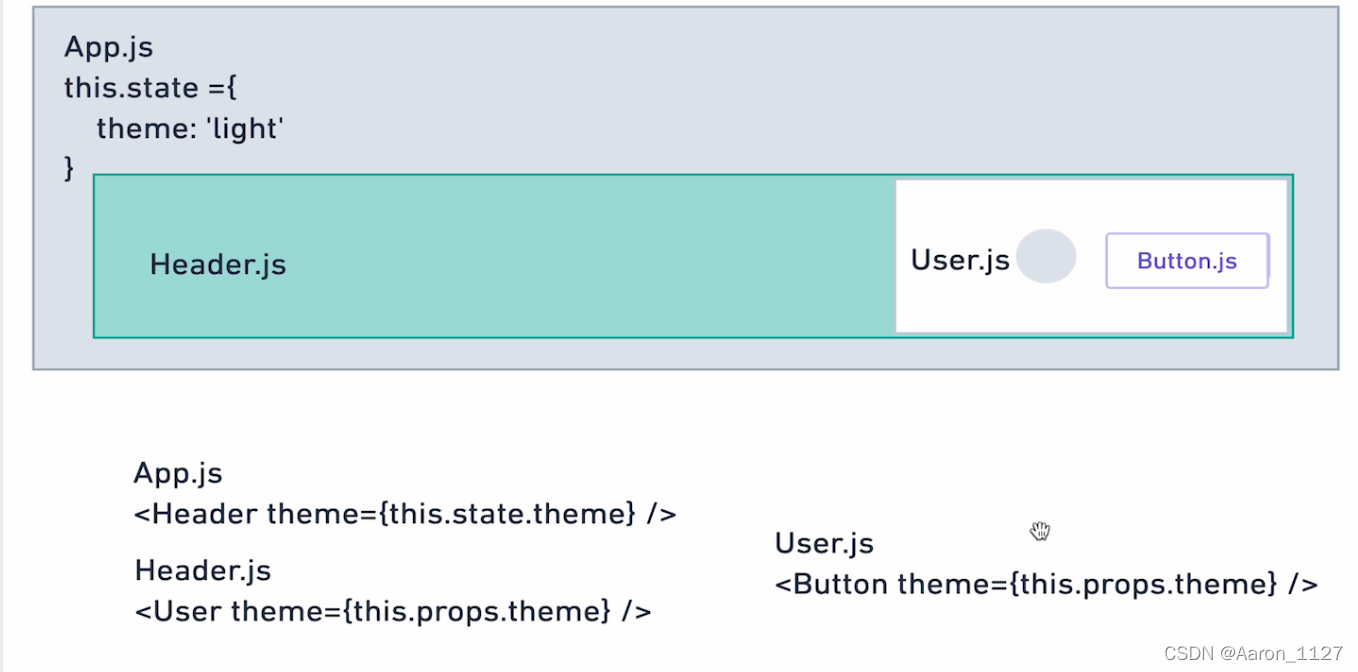 app.js中有个全局属性theme,如果想把theme对象作为属性传递到其它子组件中: Header->User->Button如果不用hooks可以像上面那样一层一层的传递下去。
useContext的使用
useContext主要解决组件多层传递属性的问题,使用useContext就可以在子组件中直接使用theme值。
- app.tsx
import React, { useState } from 'react';
import logo from './logo.svg';
import './App.css';
import LikeButton from './components/LikeButton';
import Hello from './components/Hello';
interface IThemeProps{
[key:string]:{color:string,background:string}
}
const themes:IThemeProps = {
'common':{
color:'#99969A',
background:'#F0EDF1'
},
'active':{
color:'#e70c',
background:'#FFF1E6'
}
}
export const ThemeContext = React.createContext(themes.common);
function App() {
const [status,setStatus] = useState('common');
return (
<div className="App">
<ThemeContext.Provider value={themes[status]}>
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<LikeButton/>
<Hello/>
<button onClick={()=>{setStatus(status === 'common'?'active':'common')}}>toggle color</button>
</header>
</ThemeContext.Provider>
</div>
);
}
export default App;
- 组件1 :LikeButton.tsx
import react,{useContext} from 'react';
import { ThemeContext } from '../App';
const LikeButton: react.FC = ()=>{
const theme = useContext(ThemeContext);
console.log(theme,'theme');
const style = {
color:theme.color,
background:theme.background
}
return (
<div>
<button style={style}>发布</button>
</div>
)
}
export default LikeButton;
组件2:Hello.tsx
import React,{useContext} from "react";
import { ThemeContext } from '../App';
interface IHelloProps{
message?:string
}
const Hello :React.FC<IHelloProps> = (props) =>{
const style = useContext(ThemeContext);
return (
<h2 style={style}>{props.message}</h2>
)
}
Hello.defaultProps={
message:'hello world'
}
export default Hello;
- 实现效果:
初始状态: 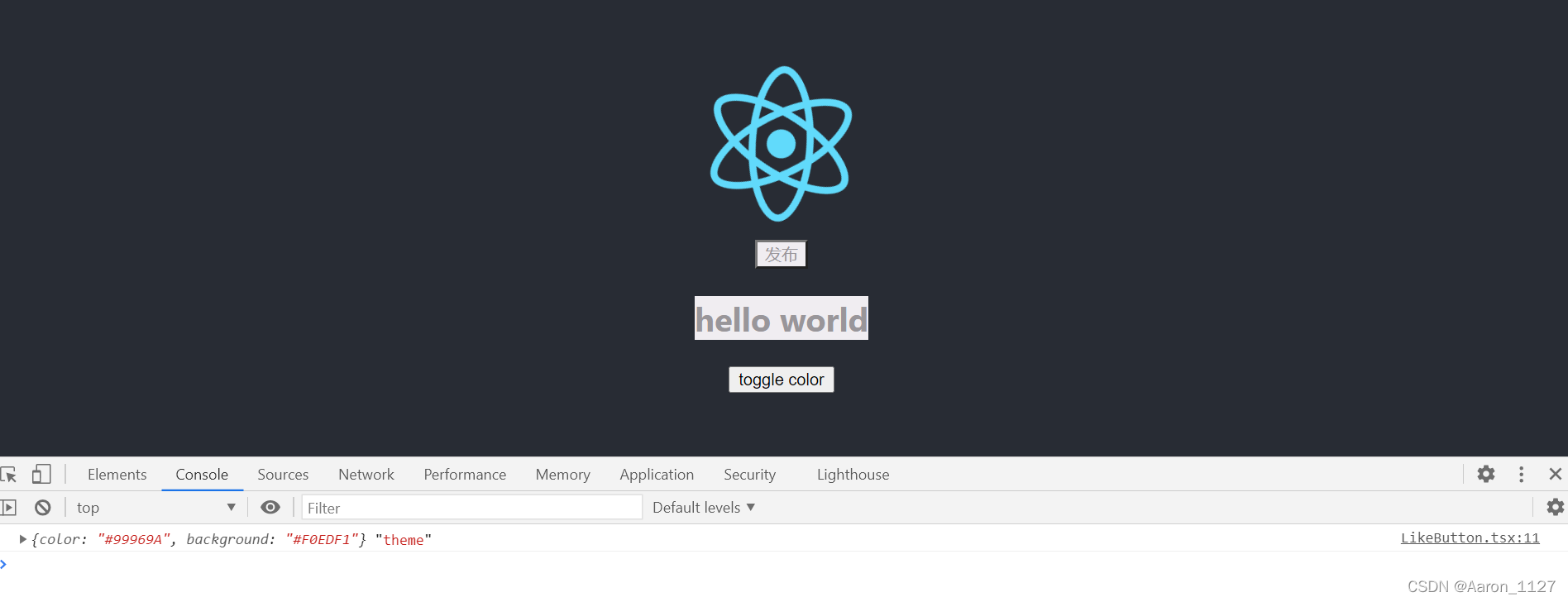 点击切换按钮:
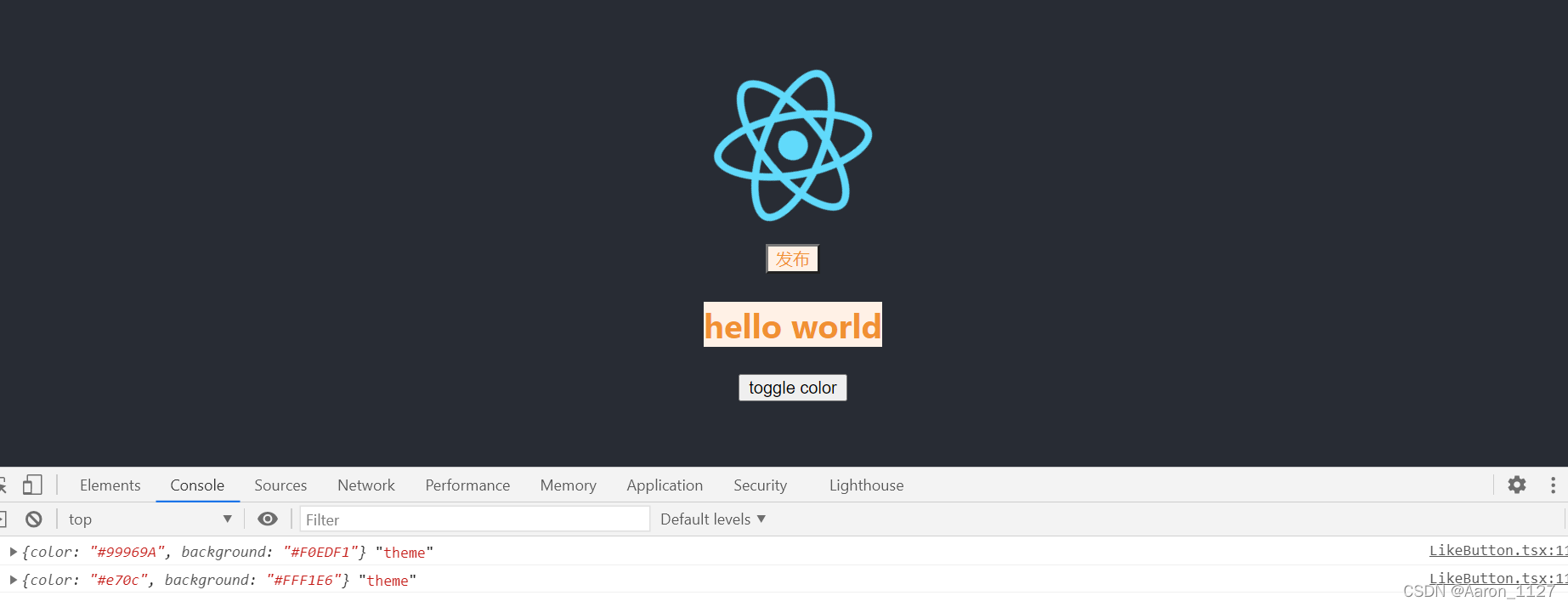
源码地址:https://gitee.com/jlzhanglong/hooks.git
|