创建前后端框架
前端
vue版本
查看版本,vue -V
? npm uninstall vue-cli -g //卸载vue2
? npm install -g @vue/cli-init //安装vue3
创建项目
采用可视化创建,勾选router和vuex
? vue ui
安装插件
一、安装Element UI 先执行
npm i element-ui -S
然后在main.js中加入以下代码
import ElementUI from 'element-ui';
import 'element-ui/lib/theme-chalk/index.css';
Vue.use(ElementUI);
二、安装Axios 先执行
npm install axios -S
然后在main.js中加入以下代码
import axios from 'axios'
Vue.prototype.$axios = axios
配置全局的Axios
- 新建axios.js文件,配置全局的baseURL,后面需要的拦截也在此配置
import router from './router'
import ElementUI from 'element-ui';
import axios from 'axios'
axios.defaults.baseURL = 'http://106.14.219.106:8081'
axios.interceptors.request.use(config => {
return config
})
axios.interceptors.response.use(res => {
let data = res.data
if (data.code == 200) {
return res;
} else if (data.code == 401) {
ElementUI.Message.error("用户名错误")
router.push('/login')
return Promise.reject(data.message)
} else {
ElementUI.Message.error("密码错误")
router.push('/login')
return Promise.reject(data.message)
}
})
-
并在main.js中引用此配置
import './axios'
后端
pom文件
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.5.0</version>
<relativePath/>
</parent>
<groupId>com.jiao</groupId>
<artifactId>ee</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>ee</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.junit.platform</groupId>
<artifactId>junit-platform-launcher</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.2</version>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-generator</artifactId>
<version>3.4.1</version>
</dependency>
<dependency>
<groupId>org.freemarker</groupId>
<artifactId>freemarker</artifactId>
<version>2.3.31</version>
</dependency>
<dependency>
<groupId>org.apache.shiro</groupId>
<artifactId>shiro-spring-boot-starter</artifactId>
<version>1.5.3</version>
</dependency>
<dependency>
<groupId>org.apache.shiro</groupId>
<artifactId>shiro-ehcache</artifactId>
<version>1.5.3</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<version>0.9.1</version>
</dependency>
<dependency>
<groupId>com.auth0</groupId>
<artifactId>java-jwt</artifactId>
<version>3.4.0</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.75</version>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.9</version>
</dependency>
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper-spring-boot-starter</artifactId>
<version>1.3.0</version>
</dependency>
<dependency>
<groupId>com.github.jeffreyning</groupId>
<artifactId>mybatisplus-plus</artifactId>
<version>1.3.1-RELEASE</version>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
yml文件
application.yml
spring:
profiles:
active: local
application-local.yml
server:
port: 8081
spring:
datasource:
url: jdbc:mysql://localhost:3306/springbootvueshiro? useUnicode=true&useSSL=false&characterEncoding=utf8&serverTimezone=Asia/Shanghai
driver-class-name: com.mysql.cj.jdbc.Driver
username: root
password: 123456
mvc:
pathmatch:
matching-strategy: ANT_PATH_MATCHER
mybatis-plus:
mapper-locations: classpath*:/mapper/**Mapper.xml
type-aliases-package: com.jiao.entity
configuration:
map-underscore-to-camel-case: true
use-generated-keys: true
application-server.yml
生成代码
CodeGenerator.java 执行此代码,输入要生成的表对象即可
package com.jiao;
import com.baomidou.mybatisplus.core.exceptions.MybatisPlusException;
import com.baomidou.mybatisplus.core.toolkit.StringPool;
import com.baomidou.mybatisplus.core.toolkit.StringUtils;
import com.baomidou.mybatisplus.generator.AutoGenerator;
import com.baomidou.mybatisplus.generator.InjectionConfig;
import com.baomidou.mybatisplus.generator.config.*;
import com.baomidou.mybatisplus.generator.config.po.TableInfo;
import com.baomidou.mybatisplus.generator.config.rules.NamingStrategy;
import com.baomidou.mybatisplus.generator.engine.FreemarkerTemplateEngine;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class CodeGenerator {
public static String scanner(String tip) {
Scanner scanner = new Scanner(System.in);
StringBuilder help = new StringBuilder();
help.append("请输入" + tip + ":");
System.out.println(help.toString());
if (scanner.hasNext()) {
String ipt = scanner.next();
if (StringUtils.isNotBlank(ipt)) {
return ipt;
}
}
throw new MybatisPlusException("请输入正确的" + tip + "!");
}
public static void main(String[] args) {
AutoGenerator mpg = new AutoGenerator();
GlobalConfig gc = new GlobalConfig();
String projectPath = System.getProperty("user.dir");
gc.setOutputDir(projectPath + "/src/main/java");
gc.setAuthor("jiao");
gc.setOpen(true);
gc.setServiceName("%sService");
mpg.setGlobalConfig(gc);
DataSourceConfig dsc = new DataSourceConfig();
dsc.setUrl("jdbc:mysql://localhost:3306/springbootvueshiro?useUnicode=true&useSSL=false&characterEncoding=utf8&serverTimezone=UTC");
dsc.setDriverName("com.mysql.cj.jdbc.Driver");
dsc.setUsername("root");
dsc.setPassword("123456");
mpg.setDataSource(dsc);
PackageConfig pc = new PackageConfig();
pc.setModuleName(null);
pc.setParent("com.jiao");
mpg.setPackageInfo(pc);
InjectionConfig cfg = new InjectionConfig() {
@Override
public void initMap() {
}
};
String templatePath = "/templates/mapper.xml.ftl";
List<FileOutConfig> focList = new ArrayList<>();
focList.add(new FileOutConfig(templatePath) {
@Override
public String outputFile(TableInfo tableInfo) {
return projectPath + "/src/main/resources/mapper/"
+ "/" + tableInfo.getEntityName() + "Mapper" + StringPool.DOT_XML;
}
});
cfg.setFileOutConfigList(focList);
mpg.setCfg(cfg);
TemplateConfig templateConfig = new TemplateConfig();
templateConfig.setXml(null);
mpg.setTemplate(templateConfig);
StrategyConfig strategy = new StrategyConfig();
strategy.setNaming(NamingStrategy.underline_to_camel);
strategy.setColumnNaming(NamingStrategy.underline_to_camel);
strategy.setEntityLombokModel(true);
strategy.setRestControllerStyle(true);
strategy.setInclude(scanner("表名,多个英文逗号分割").split(","));
strategy.setControllerMappingHyphenStyle(true);
strategy.setTablePrefix("m_");
mpg.setStrategy(strategy);
mpg.setTemplateEngine(new FreemarkerTemplateEngine());
mpg.execute();
}
}
Mapscan注解
在主启动application.java上添加@MapScan 注解
@SpringBootApplication
@MapperScan("com.jiao.mapper")
public class EeApplication {
public static void main(String[] args) {
SpringApplication.run(EeApplication.class, args);
}
}
测试
? 至此可以进行数据库的连接测试,看是否可以取出数据库中的数据
@Autowired
UserService userService;
@Test
void connectTest() {
List<User> users = userService.list();
for (User user : users) {
System.out.println(user);
}
}
编写Result统一响应类
package com.jiao.common;
@Data
@AllArgsConstructor
@NoArgsConstructor
@Accessors(chain = true)
public class Result {
private int status;
private String msg;
private Object data;
static public Result success(String msg, Object data) {
Result result = new Result();
result.setData(data).setMsg(msg).setStatus(200);
return result;
}
static public Result success(Object data) {
Result result = new Result();
result.setData(data).setStatus(200);
return result;
}
static public Result fail(String msg, int status) {
Result result = new Result();
result.setMsg(msg).setStatus(status);
return result;
}
static public Result fail(String msg) {
Result result = new Result();
result.setMsg(msg).setStatus(401);
return result;
}
}
全局异常处理
? 创建全局异常处理类
package com.jiao.common;
@RestControllerAdvice
@Slf4j
public class GlobalException {
@ExceptionHandler(value = UnauthorizedException.class)
public Result handler(UnauthorizedException e) {
log.error("无权限操作---》", e.getMessage());
return Result.fail("无权限操作", 402);
}
@ExceptionHandler(value = ExpiredCredentialsException.class)
public Result handler(ExpiredCredentialsException e) {
log.error("登录过期---》", e.getMessage());
return Result.fail("登录过期", 401);
}
@ExceptionHandler(value = UnauthenticatedException.class)
public Result handler(UnauthenticatedException e) {
log.error("未认证---》", e.getMessage());
return Result.fail("未认证(登录)", 401);
}
@ExceptionHandler(value = UnknownAccountException.class)
public Result handler(UnknownAccountException e) {
log.error("未知账户异常---》", e.getMessage());
return Result.fail("未知账户异常", 401);
}
}
前后端交互
Springboot接收参数注解
https://blog.csdn.net/qq_37236286/article/details/108850732
跨域问题
先用@CrossOrigin 注解解决跨域
测试后端controller
@RestController
@RequestMapping("/user")
@CrossOrigin
public class UserController {
@Autowired
UserService userService;
@RequestMapping(value = "/test",method = RequestMethod.GET)
public Result test( @RequestParam("userId")String userId){
User user = userService.getOne(new QueryWrapper<User>().eq("username", userId));
return Result.success("获取用户信息成功",user);
}
}
-
访问
? http://localhost:8081/user/test?userId=20201001
-
结果
{
-
“status”: 200, -
“msg”: “获取用户信息成功”, -
“data” {
- “userId”: 1,
- “username”: “20201001”,
- “password”: “4d3d7008836ee74feebc32a43ed24181”,
- “salt”: “wcwad12”,
- “nickname”: “张三”,
- “schoolId”: 1,
- “sex”: “男”,
- “tel”: “12135”,
- “studentClass”: “计算机2018-01班”
}
}
前后端交互
<template>
<div>
{{user.nickname}}
</div>
</template>
<script>
export default {
name: "LoginView",
data() {
return {
user: ''
}
},
methods: {
getUserById() {
this.$axios.get("/user/test?userId=20201001").then(
res => {
this.user = res.data.data
console.log(this.user)
}
)
}
},
created() {
this.getUserById()
}
}
</script>
<style scoped>
</style>
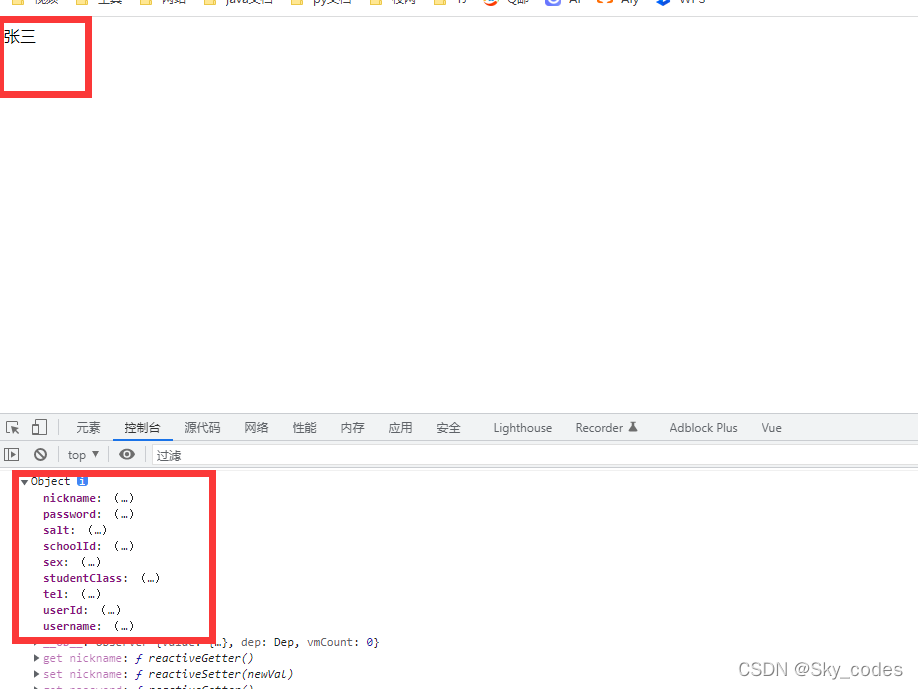
集成shiro
工具类
package com.jiao.util;
import java.util.Random;
public class SaltUtil {
public static String getSalt(int n) {
char[] chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz01234567890!@#$%^&*()".toCharArray();
StringBuilder sb = new StringBuilder();
for (int i = 0; i < n; i++) {
char aChar = chars[new Random().nextInt(chars.length)];
sb.append(aChar);
}
return sb.toString();
}
}
-
新建一个用户
@Test
void createUser() {
String salt = SaltUtil.getSalt(6);
User user = new User();
Md5Hash password = new Md5Hash("1234", salt, 1024);
user.setUsername("20220404")
.setPassword(password.toString())
.setSalt(salt)
.setNickname("娇娇")
.setSchoolId(1)
.setSex("女")
.setTel("12312312312");
boolean save = userService.save(user);
System.out.println(save);
}
自定义Realm
package com.jiao.Realm;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.jiao.entity.User;
import com.jiao.service.UserService;
import org.apache.shiro.authc.AuthenticationException;
import org.apache.shiro.authc.AuthenticationInfo;
import org.apache.shiro.authc.AuthenticationToken;
import org.apache.shiro.authc.SimpleAuthenticationInfo;
import org.apache.shiro.authz.AuthorizationInfo;
import org.apache.shiro.realm.AuthorizingRealm;
import org.apache.shiro.subject.PrincipalCollection;
import org.apache.shiro.util.ByteSource;
import org.springframework.beans.factory.annotation.Autowired;
public class MyRealm extends AuthorizingRealm {
@Autowired
UserService userService;
@Override
protected AuthorizationInfo doGetAuthorizationInfo(PrincipalCollection principalCollection) {
return null;
}
@Override
protected AuthenticationInfo doGetAuthenticationInfo(AuthenticationToken token) throws AuthenticationException {
String username = (String) token.getPrincipal();
User user = userService.getOne(new QueryWrapper<User>().eq("username", username));
if (user != null) {
if (user.getUsername().equals(username)) {
return new SimpleAuthenticationInfo(
user.getUsername(),
user.getPassword(),
ByteSource.Util.bytes(user.getSalt()),
getName()
);
}
}
return null;
}
}
shiroConfig配置类
package com.jiao.config;
import com.jiao.Realm.MyRealm;
import org.apache.shiro.authc.credential.HashedCredentialsMatcher;
import org.apache.shiro.realm.Realm;
import org.apache.shiro.spring.security.interceptor.AuthorizationAttributeSourceAdvisor;
import org.apache.shiro.spring.web.ShiroFilterFactoryBean;
import org.apache.shiro.web.mgt.DefaultWebSecurityManager;
import org.apache.shiro.mgt.SecurityManager;
import org.apache.shiro.web.mgt.WebSecurityManager;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import java.util.LinkedHashMap;
@Configuration
public class ShiroConfig {
@Bean
public ShiroFilterFactoryBean getShiroFilterFactoryBean(DefaultWebSecurityManager defaultWebSecurityManager) {
ShiroFilterFactoryBean shiroFilter = new ShiroFilterFactoryBean();
shiroFilter.setSecurityManager(defaultWebSecurityManager);
LinkedHashMap<String, String> filterMap = new LinkedHashMap<>();
filterMap.put("/user/test", "anon");
filterMap.put("/user/test2", "authc");
shiroFilter.setFilterChainDefinitionMap(filterMap);
shiroFilter.setLoginUrl("/user/login");
shiroFilter.setUnauthorizedUrl("/user/login");
return shiroFilter;
}
@Bean
public DefaultWebSecurityManager getDefaultWebSecurityManager(@Qualifier("myRealm") Realm realm) {
DefaultWebSecurityManager webSecurityManager = new DefaultWebSecurityManager();
webSecurityManager.setRealm(realm);
return webSecurityManager;
}
@Bean(value = "myRealm")
public Realm getRealm() {
MyRealm myRealm = new MyRealm();
HashedCredentialsMatcher credentialsMatcher = new HashedCredentialsMatcher();
credentialsMatcher.setHashAlgorithmName("md5");
credentialsMatcher.setHashIterations(1024);
myRealm.setCredentialsMatcher(credentialsMatcher);
return myRealm;
}
@Bean
public AuthorizationAttributeSourceAdvisor authorizationAttributeSourceAdvisor(SecurityManager securityManager) {
AuthorizationAttributeSourceAdvisor authorizationAttributeSourceAdvisor = new AuthorizationAttributeSourceAdvisor();
authorizationAttributeSourceAdvisor.setSecurityManager(securityManager);
return authorizationAttributeSourceAdvisor;
}
}
PostMan测试
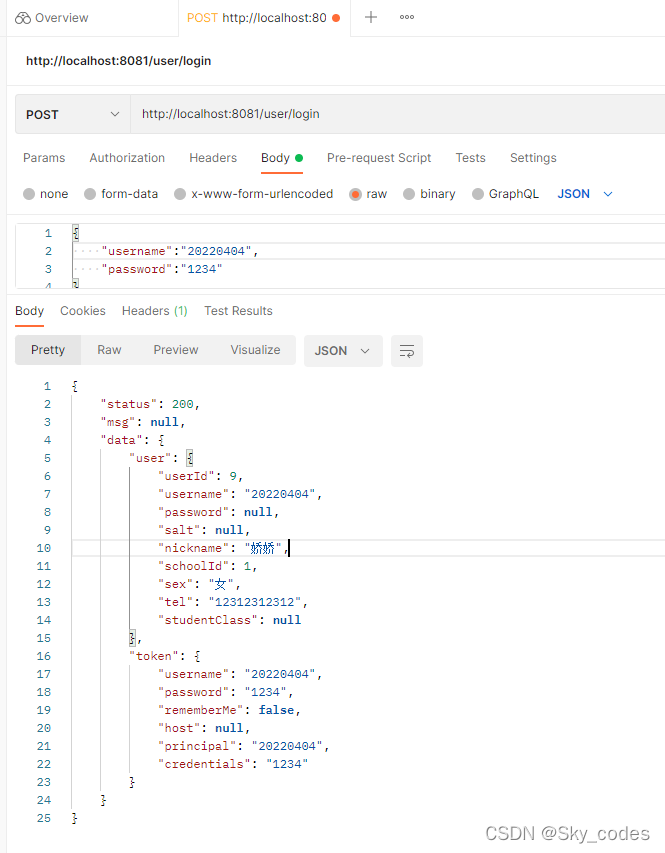
开启Redis缓存
https://blog.csdn.net/A233666/article/details/113436813
-
MybyteSource package com.jiao.cache;
import org.apache.shiro.codec.Base64;
import org.apache.shiro.codec.CodecSupport;
import org.apache.shiro.codec.Hex;
import org.apache.shiro.util.ByteSource;
import java.io.File;
import java.io.InputStream;
import java.io.Serializable;
import java.util.Arrays;
public class MyByteSource implements ByteSource, Serializable {
private byte[] bytes;
private String cachedHex;
private String cachedBase64;
public MyByteSource() {
}
public MyByteSource(byte[] bytes) {
this.bytes = bytes;
}
public MyByteSource(char[] chars) {
this.bytes = CodecSupport.toBytes(chars);
}
public MyByteSource(String string) {
this.bytes = CodecSupport.toBytes(string);
}
public MyByteSource(ByteSource source) {
this.bytes = source.getBytes();
}
public MyByteSource(File file) {
this.bytes = (new com.jiao.cache.MyByteSource.BytesHelper()).getBytes(file);
}
public MyByteSource(InputStream stream) {
this.bytes = (new com.jiao.cache.MyByteSource.BytesHelper()).getBytes(stream);
}
public static boolean isCompatible(Object o) {
return o instanceof byte[] || o instanceof char[] || o instanceof String || o instanceof ByteSource || o instanceof File || o instanceof InputStream;
}
public byte[] getBytes() {
return this.bytes;
}
public boolean isEmpty() {
return this.bytes == null || this.bytes.length == 0;
}
public String toHex() {
if (this.cachedHex == null) {
this.cachedHex = Hex.encodeToString(this.getBytes());
}
return this.cachedHex;
}
public String toBase64() {
if (this.cachedBase64 == null) {
this.cachedBase64 = Base64.encodeToString(this.getBytes());
}
return this.cachedBase64;
}
public String toString() {
return this.toBase64();
}
public int hashCode() {
return this.bytes != null && this.bytes.length != 0 ? Arrays.hashCode(this.bytes) : 0;
}
public boolean equals(Object o) {
if (o == this) {
return true;
} else if (o instanceof ByteSource) {
ByteSource bs = (ByteSource) o;
return Arrays.equals(this.getBytes(), bs.getBytes());
} else {
return false;
}
}
private static final class BytesHelper extends CodecSupport {
private BytesHelper() {
}
public byte[] getBytes(File file) {
return this.toBytes(file);
}
public byte[] getBytes(InputStream stream) {
return this.toBytes(stream);
}
}
}
-
RedisCache package com.jiao.cache;
import com.jiao.util.ApplicationContextUtils;
import org.apache.shiro.cache.Cache;
import org.apache.shiro.cache.CacheException;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.StringRedisSerializer;
import java.util.Collection;
import java.util.Set;
public class RedisCache<k, v> implements Cache<k, v> {
private String cacheName;
public RedisCache() {
}
public RedisCache(String cacheName) {
this.cacheName = cacheName;
}
@Override
public v get(k k) throws CacheException {
System.out.println("get key:" + k);
return (v) getRedisTemplate().opsForHash().get(this.cacheName, k.toString());
}
@Override
public v put(k k, v v) throws CacheException {
System.out.println("put key: " + k);
System.out.println("put value:" + v);
getRedisTemplate().opsForHash().put(this.cacheName, k.toString(), v);
return null;
}
@Override
public v remove(k k) throws CacheException {
System.out.println("=============remove=============");
return (v) getRedisTemplate().opsForHash().delete(this.cacheName, k.toString());
}
@Override
public void clear() throws CacheException {
System.out.println("=============clear==============");
getRedisTemplate().delete(this.cacheName);
}
@Override
public int size() {
return getRedisTemplate().opsForHash().size(this.cacheName).intValue();
}
@Override
public Set<k> keys() {
return getRedisTemplate().opsForHash().keys(this.cacheName);
}
@Override
public Collection<v> values() {
return getRedisTemplate().opsForHash().values(this.cacheName);
}
private RedisTemplate getRedisTemplate() {
RedisTemplate redisTemplate = (RedisTemplate) ApplicationContextUtils.getBean("redisTemplate");
redisTemplate.setKeySerializer(new StringRedisSerializer());
redisTemplate.setHashKeySerializer(new StringRedisSerializer());
return redisTemplate;
}
}
-
RedisCacheManager package com.jiao.cache;
import org.apache.shiro.cache.Cache;
import org.apache.shiro.cache.CacheException;
import org.apache.shiro.cache.CacheManager;
public class RedisCacheManager implements CacheManager {
@Override
public <K, V> Cache<K, V> getCache(String cacheName) throws CacheException {
System.out.println(cacheName);
return new RedisCache<K,V>(cacheName);
}
}
-
工具类ApplicationContextUtils
自动注入失效,需要手动获取
package com.jiao.util;
import org.springframework.beans.BeansException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.stereotype.Component;
@Component
public class ApplicationContextUtils implements ApplicationContextAware {
public static ApplicationContext context;
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
context = applicationContext;
}
public static Object getBean(String beanName) {
return context.getBean(beanName);
}
}
-
修改MyRealm 的盐,序列化,使用自定义的MyByteSource @Override
protected AuthenticationInfo doGetAuthenticationInfo(AuthenticationToken token) throws AuthenticationException {
String username = (String) token.getPrincipal();
User user = userService.getOne(new QueryWrapper<User>().eq("username", username));
if (user != null) {
if (user.getUsername().equals(username)) {
return new SimpleAuthenticationInfo(
user.getUsername(),
user.getPassword(),
new MyByteSource(user.getSalt()),
getName()
);
}
}
return null;
}
-
shiroConfig 中,在realm注入时开启注解 @Bean(value = "myRealm")
public Realm getRealm() {
MyRealm myRealm = new MyRealm();
HashedCredentialsMatcher credentialsMatcher = new HashedCredentialsMatcher();
credentialsMatcher.setHashAlgorithmName("md5");
credentialsMatcher.setHashIterations(1024);
myRealm.setCredentialsMatcher(credentialsMatcher);
myRealm.setCachingEnabled(true);
myRealm.setAuthorizationCacheName("authorizationCacheName");
myRealm.setAuthorizationCachingEnabled(true);
myRealm.setAuthenticationCacheName("authenticationCacheName");
myRealm.setAuthenticationCachingEnabled(true);
myRealm.setCacheManager(new RedisCacheManager());
return myRealm;
}
-
当前local-yml server:
port: 8081
spring:
datasource:
url: jdbc:mysql://localhost:3306/springbootvueshiro? useUnicode=true&useSSL=false&characterEncoding=utf8&serverTimezone=Asia/Shanghai
driver-class-name: com.mysql.cj.jdbc.Driver
username: root
password: 123456
mvc:
pathmatch:
matching-strategy: ANT_PATH_MATCHER
redis:
port: 6379
host: localhost
database: 0
mybatis-plus:
mapper-locations: classpath*:/mapper/**Mapper.xml
type-aliases-package: com.jiao.entity
configuration:
map-underscore-to-camel-case: true
use-generated-keys: true
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
-
开启redis
-
查询key
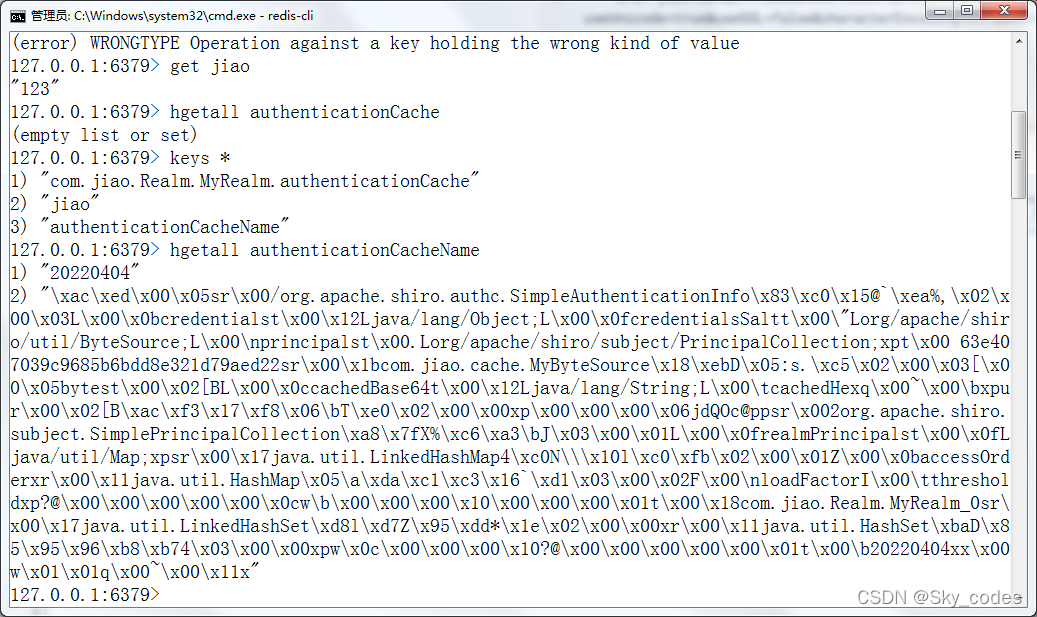
当前项目结构
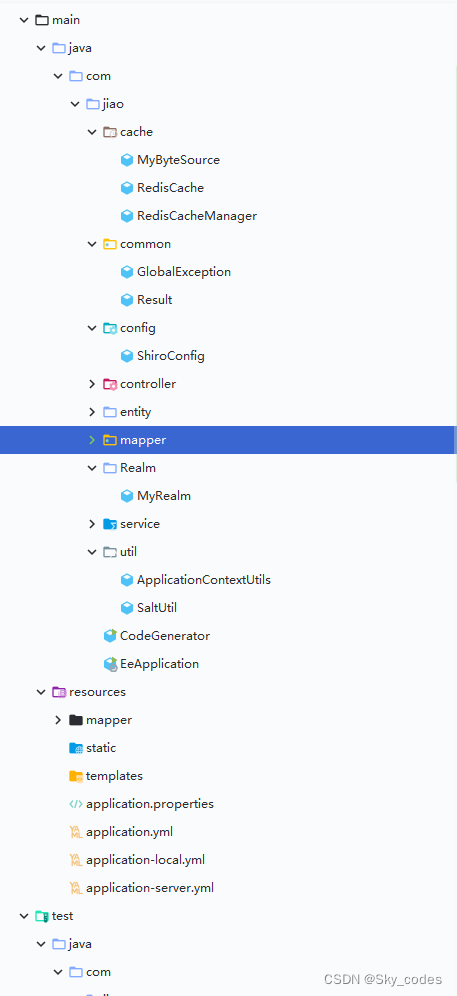
集成JWT
https://blog.csdn.net/qq_38366063/article/details/103993117
server:
port: 8081
spring:
datasource:
url: jdbc:mysql://localhost:3306/springbootvueshiro? useUnicode=true&useSSL=false&characterEncoding=utf8&serverTimezone=Asia/Shanghai
driver-class-name: com.mysql.cj.jdbc.Driver
username: root
password: 123456
mvc:
pathmatch:
matching-strategy: ANT_PATH_MATCHER
redis:
port: 6379
host: localhost
database: 0
mybatis-plus:
mapper-locations: classpath*:/mapper/**Mapper.xml
type-aliases-package: com.jiao.entity
configuration:
map-underscore-to-camel-case: true
use-generated-keys: true
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
~~~
- 开启redis
- > ? redis-server
- 查询key
- > ? redis-cli
>
![在这里插入图片描述](https://img-blog.csdnimg.cn/b3c5bf6f10dd495bb8d2342094b6d6fd.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBAU2t5X2NvZGVz,size_20,color_FFFFFF,t_70,g_se,x_16
https://blog.csdn.net/qq_38366063/article/details/103993117
https://blog.csdn.net/Gorho/article/details/115921028
|