对网页不同尺寸进行截图,检查在不同尺寸下网页ui是否有错误。 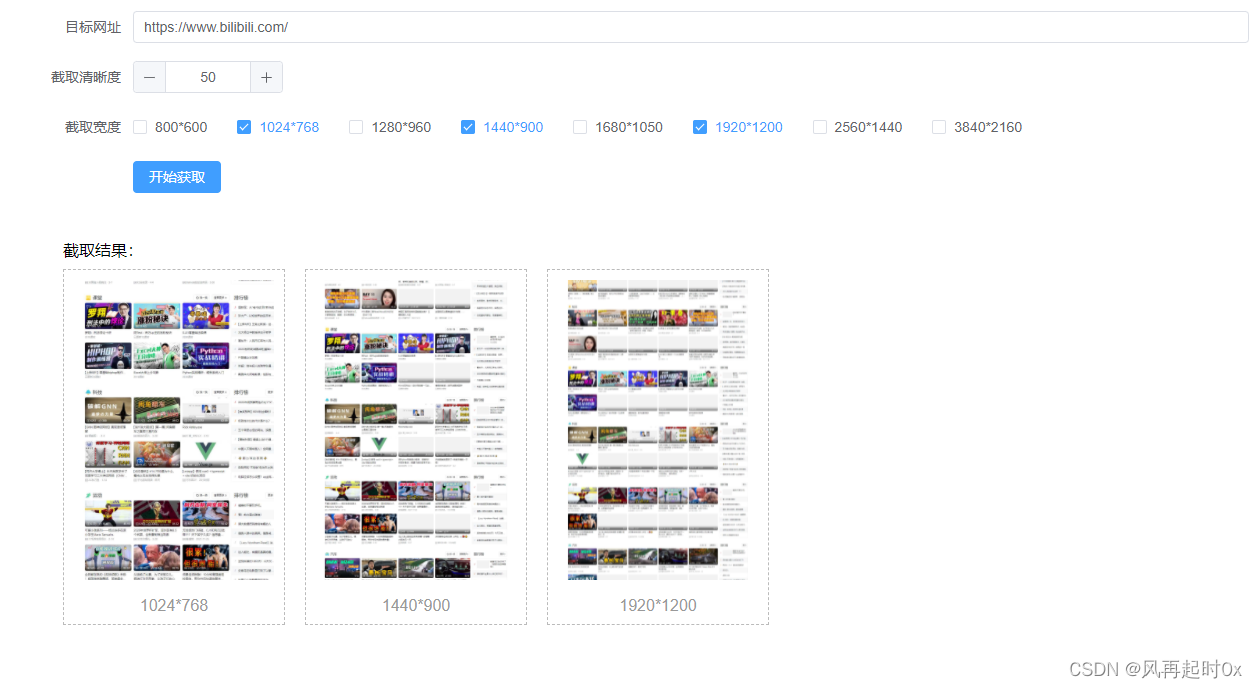 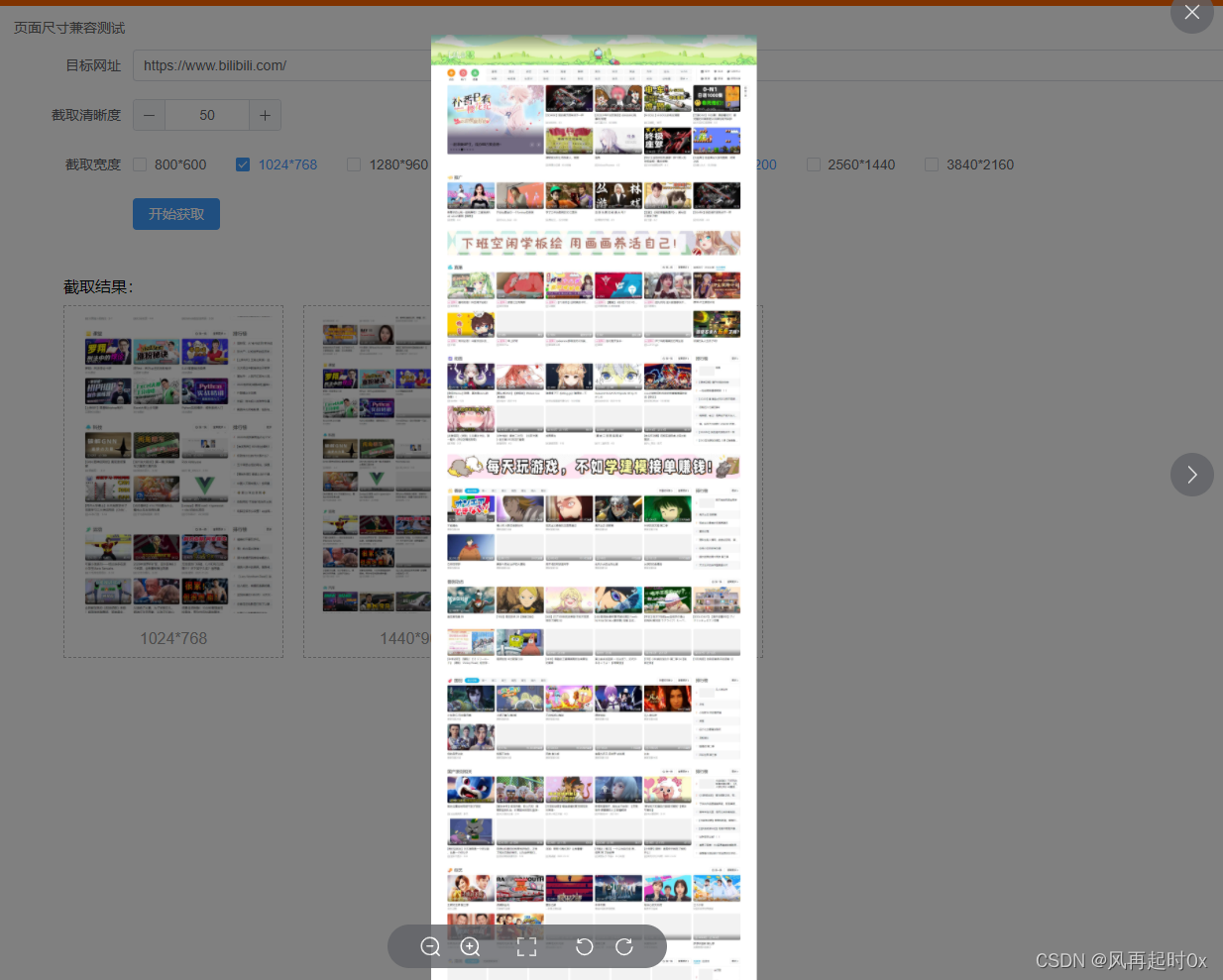 该demo基于koa.js和puppeteer.js 接口实现:
index.js
const Koa = require('koa');
const app = new Koa;
const cors = require('koa2-cors');
app.use(cors());
const path = require('path')
const koaStatic = require('koa-static')
app.use(koaStatic(path.join(__dirname, './src/assets/')))
const userRoute = require('./src/router');
app.use(userRoute.routes()).use(userRoute.allowedMethods());
app.listen(3000, () => {
console.log('Service running: http://127.0.0.1:3000');
})
module.exports = app;
/router/index.js:
const Router = require('koa-router');
const router = new Router();
const KoaBody = require('koa-body');
router.get('/getJpeg', auth, async (ctx) => {
const getPdf = require('../views/getJpeg');
ctx.body = JSON.stringify(await getJpeg(ctx));
})
module.exports = router;
/views/getJpeg.js:
const puppeteer = require('puppeteer');
function delay(time) {
return new Promise(res => setTimeout(res, time))
}
function autoScroll(page) {
return page.evaluate(() => {
return new Promise((resolve, reject) => {
var totalHeight = 0;
var distance = 500;
var timer = setInterval(() => {
var scrollHeight = document.body.scrollHeight;
window.scrollBy(0, distance);
totalHeight += distance;
if (totalHeight >= scrollHeight) {
clearInterval(timer);
resolve(totalHeight);
}
}, 17);
})
});
}
const sc = [
{ width: 800, height: 600 },
{ width: 1024, height: 768 },
{ width: 1280, height: 960 },
{ width: 1440, height: 900 },
{ width: 1680, height: 1050 },
{ width: 1920, height: 1200 },
{ width: 2560, height: 1440 },
{ width: 3840, height: 2160 },
]
async function getJpegStart(url, scarr, quality = 50) {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto(url, {
waitUntil: 'networkidle0'
});
await delay(3000)
let pathArr = [];
async function sh() {
if (!scarr.length) return
await page.setViewport(sc[scarr[0]]);
let height = await autoScroll(page);
let u = url.replace(':', '_').replace(/\./g, '_').replace(/\//g, '');
await page.screenshot({
path: 'src/assets/' + sc[scarr[0]].width + '_' + sc[scarr[0]].height + '(' + u + ')' + '.jpeg',
clip: {
x: 0,
y: 0,
width: sc[scarr[0]].width,
height: height,
},
quality
});
pathArr.push({
src: sc[scarr[0]].width + '_' + sc[scarr[0]].height + '(' + u + ')' + '.jpeg',
size: sc[scarr[0]].width + '*' + sc[scarr[0]].height,
name: sc[scarr[0]].width + '_' + sc[scarr[0]].height + '(' + u + ')'
});
scarr.shift();
await sh();
}
await sh()
await browser.close()
return pathArr
}
async function getJpeg(ctx, id) {
let res;
let url = ctx.query.url;
let quality = Number(ctx.query.quality);
let sc = [];
ctx.query.sc.split(',').forEach(function (v) { sc.push(Number(v)) })
let pathArr = await getJpegStart(url, sc, quality);
if (pathArr.length) {
pathArr.forEach(function (v) {
v.src = 'http://' + ctx.host + '/' + v.src;
})
res = {
status: 1,
data: pathArr
}
} else {
res = {
status: 0,
data: null,
msg: '目标链接访问失败'
}
}
return res
}
module.exports = getJpeg;
|