目录
1 对象的简化写法
ES6允许在大括号里面,直接写入变量和函数,作为对象的属性和方法,这样的书写更加简洁
let name = 'aaa';
let change = function(){
console.log('change()执行了...');
}
const school = {
name: name,
change: change,
improve: function(){
consolg.log('improve()执行了...');
}
}
const school1 = {
name,
change,
improve(){
consolg.log('improve()执行了...');
}
}
2 结构赋值
什么是解构赋值: ES6 允许按照一定模式,从数组和对象中提取值,对变量进行赋值,这被称为解构赋值
演示代码:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>解构赋值</title>
</head>
<body>
<script>
const F4 = ["大哥", "二哥", "三哥", "四哥"];
let [a, b, c, d] = F4;
console.log(a + b + c + d);
const F3 = {
name: "大哥",
age: 22,
sex: "男",
xiaopin: function () {
}
}
let { name, age, sex, xiaopin } = F3;
console.log(name + age + sex + xiaopin);
xiaopin();
</script>
</body>
</html>
3 模板字符串
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<script>
let string = `我也一个字符串哦!`;
console.log(string);
let str = `<ul>
<li>大哥</li>
<li>二哥</li>
<li>三哥</li>
<li>四哥</li>
</ul>`;
console.log(str);
let s = "大哥";
let out = `${s}是我最大的榜样!`;
console.log(out);
</script>
</body>
</html>
4 箭头函数
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>箭头函数</title>
</head>
<body>
<script>
function getName(){
console.log(this.name);
}
let getName2 = () => {
console.log(this.name);
}
window.name = '尚硅谷';
const school = {
name: "ATGUIGU"
}
let pow = n => n * n;
console.log(pow(8));
</script>
</body>
</html>
5 函数参数默认值
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>函数参数默认值</title>
</head>
<body>
<script>
function connect({host="127.0.0.1", username,password, port}){
console.log(host)
console.log(username)
console.log(password)
console.log(port)
}
connect({
host: 'atguigu.com',
username: 'root',
password: 'root',
port: 3306
})
</script>
</body>
</html>
6 模块化
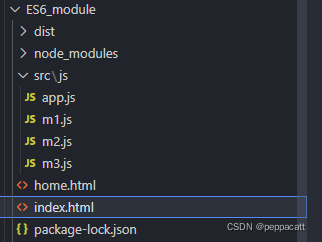
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>ES6 模块化</title>
</head>
<body>
<script type="module">
</script>
</body>
</html>
m1.js
export let school = '尚硅谷';
export function teach() {
console.log("我们可以教给你开发技能");
}
m2.js
let school = '尚硅谷';
function findJob(){
console.log("我们可以帮助你找工作!!");
}
export {school, findJob};
m3.js
export default {
school: 'ATGUIGU',
change: function(){
console.log("我们可以改变你!!");
}
}
|