React 组件
创建组件
组件分为两类,分别是函数式组件,以及类组件
函数式组件
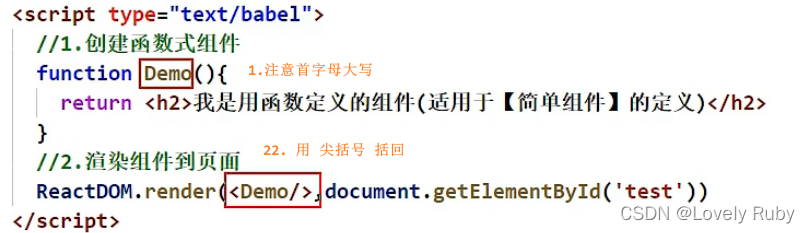 函数式组件中的 this 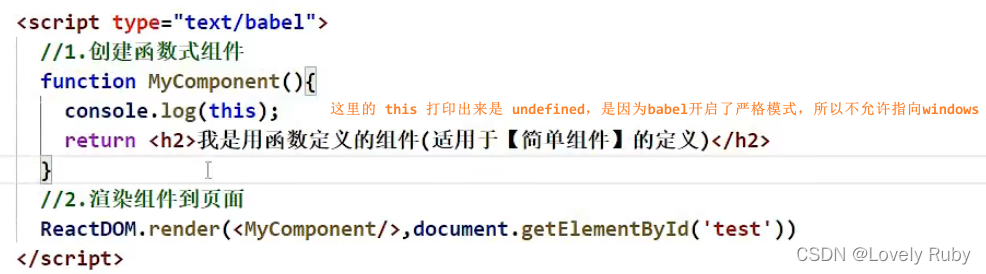 babel 官网的试一试,可以把函数复制进来看一看
类组件
类中的一般方法放到了类的原型对象上
<script type="text/babel">
class MyComponent extends React.Component {
render() {
console.log("render中的this:", this);
return <h2>我是用类定义的组件(适用于【复杂组件】的定义)</h2>;
}
}
ReactDOM.render(<MyComponent />, document.getElementById("test"));
</script>
组件三大特征
state
首先设置组件的 state ,进行状态的初始化:
<script type="text/babel">
class Weather extends React.Component {
constructor(props) {
super(props);
this.state = {
isHot: true,
};
}
render() {
const { isHot } = this.state;
return <h1>今天天气很{isHot ? "炎热" : "凉爽"}</h1>;
}
}
ReactDOM.render(<Weather />, document.querySelector("#test"));
</script>
添加点击事件:
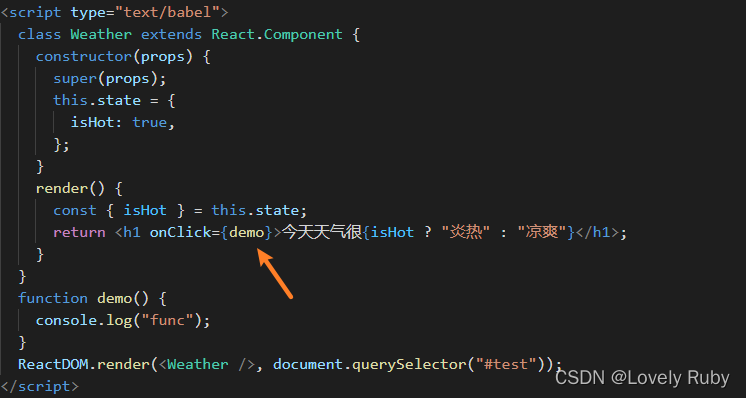
类中的方法默认开启了局部的严格模式
setState
状态不可以直接更改,必须要借助一个内置的 API:setState({}) ,要求必须传递一个对象,而且是合并操作
复杂模式的修改,理解一下this 和其他的概念
<script type="text/babel">
class Weather extends React.Component{
constructor(props){
console.log('constructor');
super(props)
this.state = {isHot:false,wind:'微风'}
this.changeWeather = this.changeWeather.bind(this)
}
render(){
console.log('render');
const {isHot,wind} = this.state
return <h1 onClick={this.changeWeather}>今天天气很{isHot ? '炎热' : '凉爽'},{wind}</h1>
}
changeWeather(){
console.log('changeWeather');
const isHot = this.state.isHot
this.setState({isHot:!isHot})
console.log(this);
}
}
ReactDOM.render(<Weather/>,document.getElementById('test'))
</script>
在开发中很少使用到构造函数,所以,属性和方法的设置,类似下面的代码块
<script type="text/babel">
class Weather extends React.Component{
state = {isHot:false,wind:'微风'}
render(){
const {isHot,wind} = this.state
return <h1 onClick={this.changeWeather}>今天天气很{isHot ? '炎热' : '凉爽'},{wind}</h1>
}
changeWeather = () => {
const isHot = this.state.isHot
this.setState({isHot:!isHot})
}
}
ReactDOM.render(<Weather/>,document.getElementById('test'))
</script>
总结一下:
- 组件中
render 方法中的this 为组件实例对象 - 组件自定义的方法中
this 为undefined ,如何解决? a). 强制绑定this: 通过函数对象的bind() b). 箭头函数 - 状态数据,不能直接修改或更新
props
基本传递
外部往组件中带东西 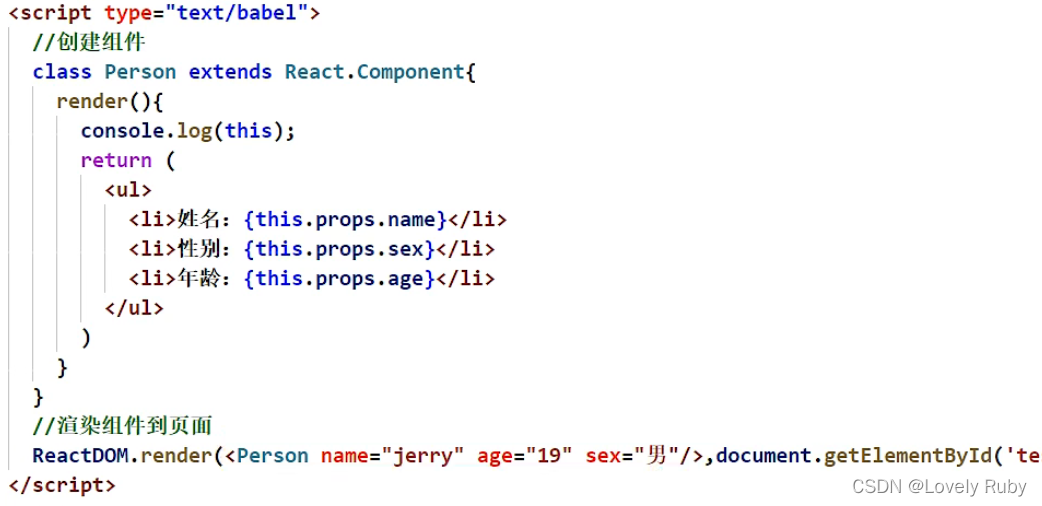
批量传递
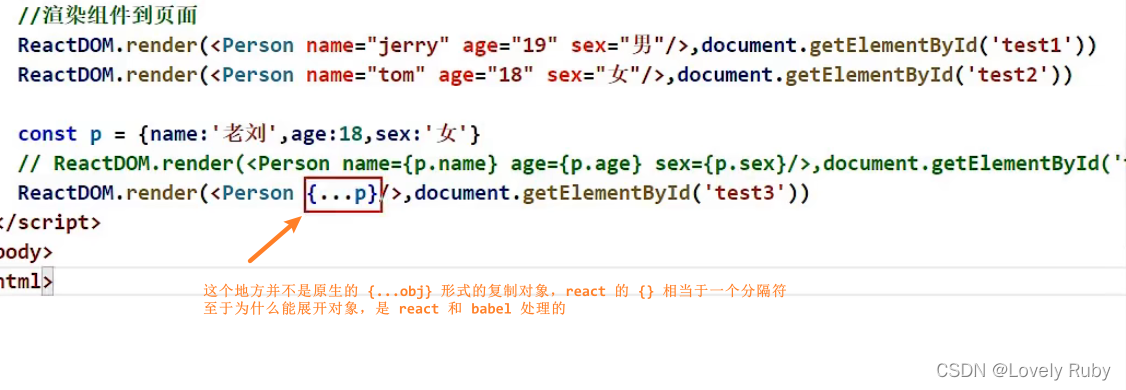 而且 ...obj 在 babel 和 react 环境 下,只有在标签上才可以,如果你想输出的话是不可以的。
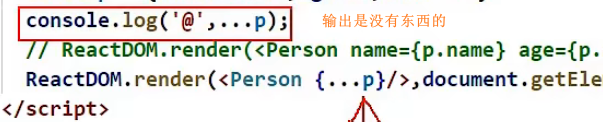
函数式组件的传值
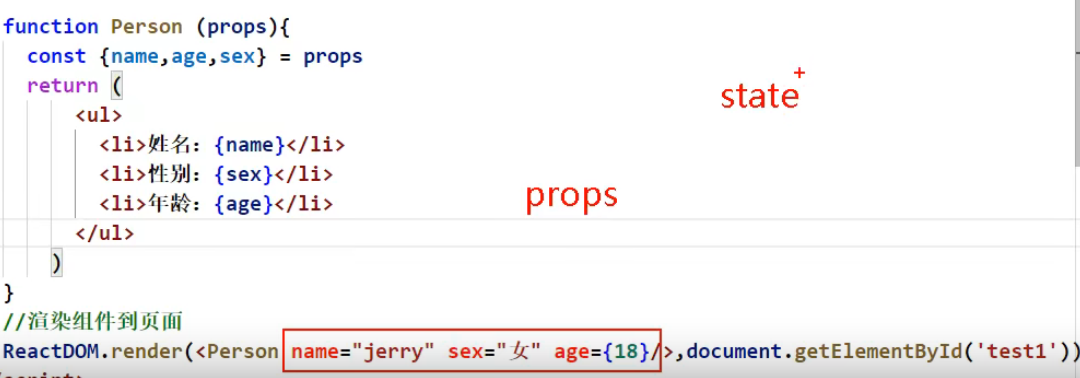
对 Props 进行限制【类式组件】
通过引入新的库 prop-types.js ,引入完之后,全局中会多出个新的对象,叫做:PropTypes
class Person extends React.Component {
render() {
const { name, age, sex } = this.props;
return (
<ul>
<li>姓名:{name}</li>
<li>性别:{sex}</li>
<li>年龄:{age + 1}</li>
</ul>
);
}
}
Person.propTypes = {
name: PropTypes.string.isRequired,
sex: PropTypes.string,
age: PropTypes.number,
speak: PropTypes.func,
};
Person.defaultProps = {
sex: "男",
age: 18,
};
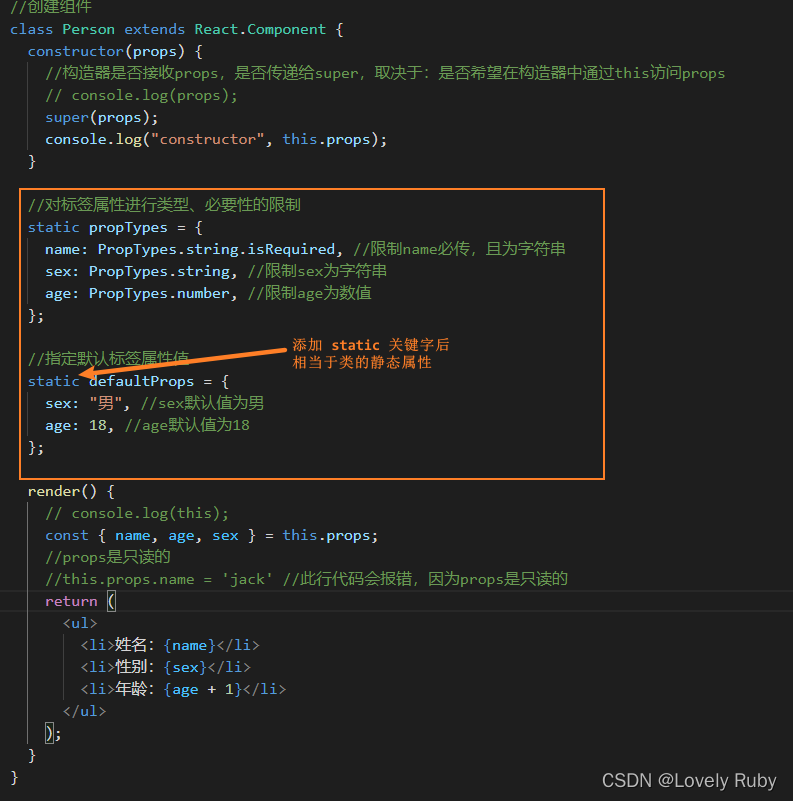
对 Props 进行限制【函数式组件】
对 prop 的限制,也就是给这个类填上些属性 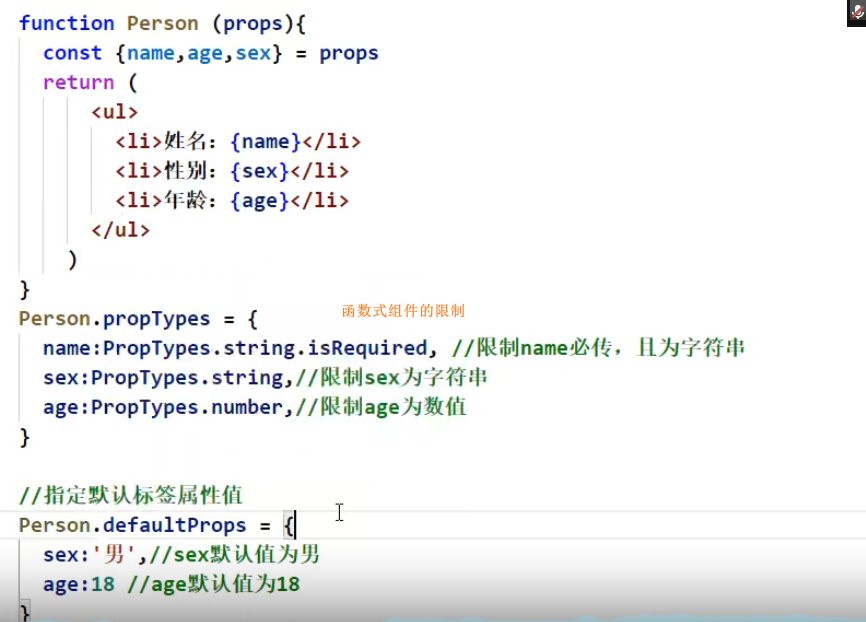
|