leaflet地图在React中的使用
关于Leaflet
leaflet是一款开源且易用于web开发的地图库。它拥有大多数开发者所需要的所有地图功能。,之前使用基本是在vue中使用,踩在巨人的肩膀上使用别人基于**leaflet 二次封装过的vue-leaflet **,该插件封装使用起来非常简单,直接参考 官方文档 和教程使用即可。
React中使用
显然react中是不可以使用vue-leaflet插件去绘制地图的,这是我们就是要直接去啃leaflet这个骨头
leaftlet官网
https://leafletjs.com/
我们需要的API文档以及教程都可以参照官网
下载leaflet
yarn add leaflet
引入css
import "leaflet/dist/leaflet.css";
创建地图容器组件
<Box id="latest-location-history-map-container"> </Box>
初始化地图
import L from "leaflet";
const { id, center = [30.636088, 180.990693], zoom = 2 } = props;
const myMap = L.map(id).setView(center, zoom);
L.tileLayer("http://{s}.tile.osm.org/{z}/{x}/{y}.png", {
attribution: 'Map data © <a href="https://www.openstreetmap.org/copyright">OpenStreetMap</a> contributors, Imagery ? <a href="https://www.mapbox.com/">Mapbox</a>',
maxZoom: 18,
minZoom: 2,
}).addTo(myMap);
myMap.setMaxBounds(L.latLngBounds(L.latLng(-90, -360), L.latLng(90, 180)));
此时地图已经可以正常显示在我们的容器组件里面了
其他操作
添加标记点
const icon = L.icon({ iconUrl: marker, popupAnchor: [0, -36], iconAnchor: [24, 48] });
L.marker([res.latitude, res.longitude], { icon }).bindPopup(`<div style="font-size: 16px">${res.locationName}</div>`).addTo(myMap).openPopup();
注: 上面的popupAnchor和iconAnchor都是用来调整popup和icon的位置的参数,icon也并非一定要自定义
聚合marker
在vue-leaflet中我们可以使用v-marker-cluster 组件来添加自动聚合的marker
例如:
<v-marker-cluster :options="clusterOptions">
<v-marker v-for="(item, index) in locations" :key="index" :lat-lng="[item.latitude, item.longitude]" dblclick.stop :icon="$map.Icon.default">
<v-tooltip>
<div style="padding: 5px">
<p>{{ item.locationName }}</p>
<div>
<span>{{ $tr("common.latestReportedTime") }}</span>
<span>{{ $timeFormat(item.latestReportedTime) }}</span>
</div>
<p v-if="showTotalCheckins">
<span>{{ $tr("common.totalCheckins") }}</span>
<span>{{ item.deviceLocationPoints.length }}</span>
</p>
<div v-if="showDeviceName">{{ item.deviceFriendlyName }}</div>
</div>
</v-tooltip>
</v-marker>
</v-marker-cluster>
现在我们需要在leaflet插件库中下载leaflet.markercluster 这个插件并引入插件以及对应的css文件
下载
yarn add leaflet.markercluster
引入
import "leaflet.markercluster";
import "leaflet.markercluster/dist/MarkerCluster.css";
import "leaflet.markercluster/dist/MarkerCluster.Default.css";
使用
本身在引入leaflet这个库的时候leaflet会将自己的核心对象L 挂载在window 对象上,在引入leaflet.markercluster 后,该插件会向L 对象添加markerClusterGroup 方法,然后我们直接使用(按照文档)
useEffect(() => {
if (!map) {
return;
}
const icon = L.icon({ iconUrl: marker, tooltipAnchor: [0, -36], iconAnchor: [24, 48] });
deviceController
.getDeviceLocationHistories(id, pageLink)
.then((res) => {
setLocationsData(res);
var markers = L.markerClusterGroup();
res.data.forEach((data) => {
markers.addLayer(L.marker([data.latitude, data.longitude], { icon }).bindTooltip(data.locationName));
});
// @ts-ignore
map.addLayer(markers);
})
.catch((err) => {});
}, [id, pageLink, map]);
这样地图就有了自动聚合marker的功能。 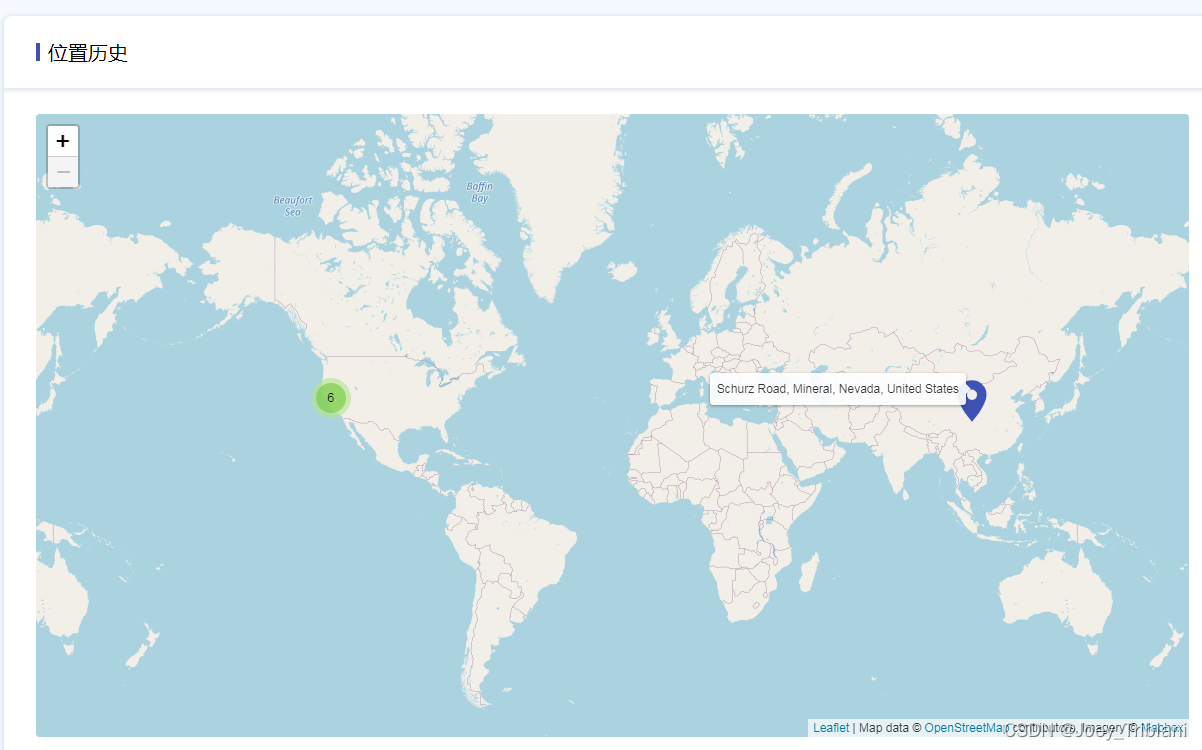
|