04. Vue组件
全局组件
01.Vue.extend()构造全局组件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./js/vue.js"></script>
</head>
<body>
<div id="app">
<my-component></my-component>
</div>
<script>
let myComponent = Vue.extend({
template: ` <h2> 我们注册的全局组件 </h2> `
});
Vue.component("my-component", myComponent);
let vm = new Vue({
el: "#app",
data: {
}
});
</script>
</body>
</html>
02.Vue.component()构造的时候直接传入配置对象(Vue推荐使用的方式)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./js/vue.js"></script>
</head>
<body>
<div id="app">
<my-component></my-component>
</div>
<script>
Vue.component("my-component", {
template: ` <h2> 我们注册的全局组件 </h2> `
});
let vm = new Vue({
el: "#app",
data: {
}
});
</script>
</body>
</html>
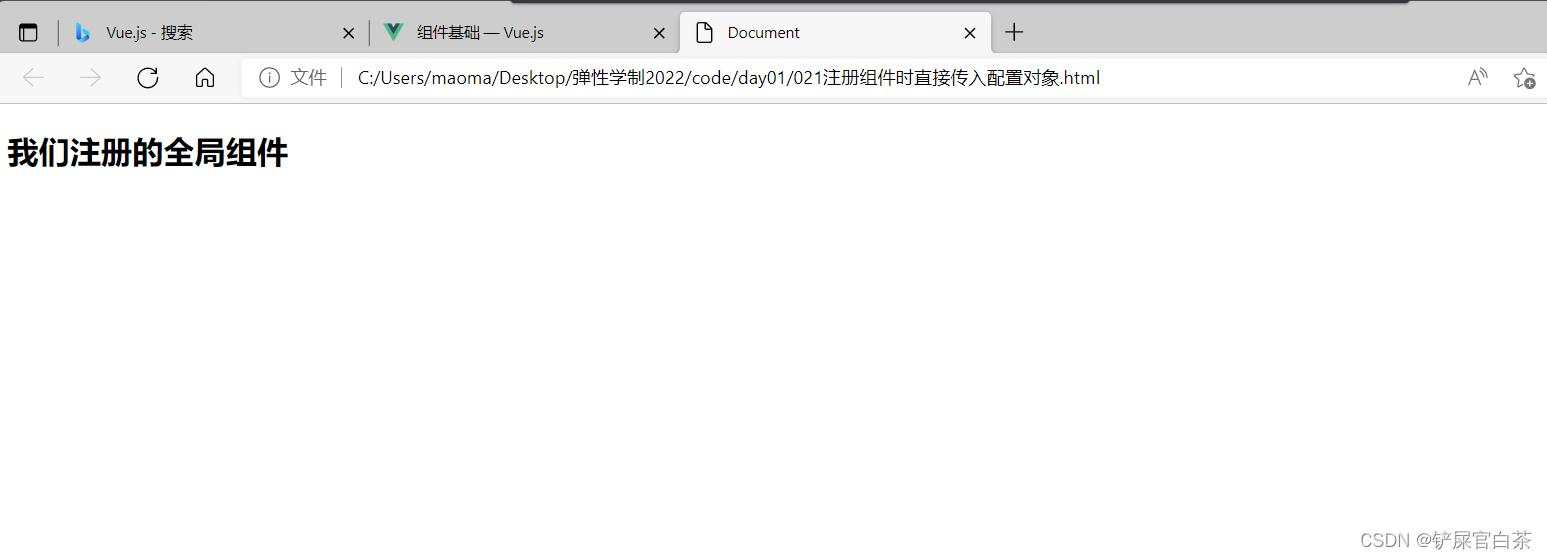
03.组件模板有且仅有一个根节点
错误演示
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./js/vue.js"></script>
</head>
<body>
<div id="app">
<my-component></my-component>
</div>
<script>
Vue.component("my-component", {
template: ` <h2> 我们注册的全局组件 </h2> <h2> 我们注册的全局组件 </h2> `
});
let vm = new Vue({
el: "#app",
data: {
}
});
</script>
</body>
</html>
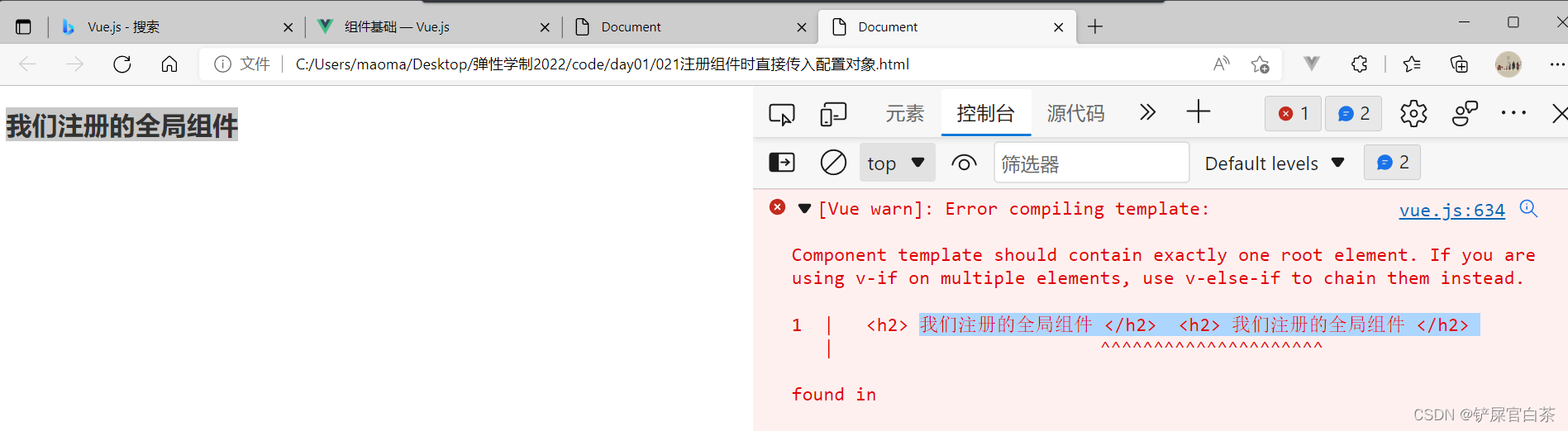
正确演示
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./js/vue.js"></script>
</head>
<body>
<div id="app">
<my-component></my-component>
</div>
<script>
Vue.component("my-component", {
template: `
<div>
<h2> 我们注册的全局组件 </h2>
<h2> 我们注册的全局组件 </h2>
</div>
`
});
let vm = new Vue({
el: "#app",
data: {
}
});
</script>
</body>
</html>
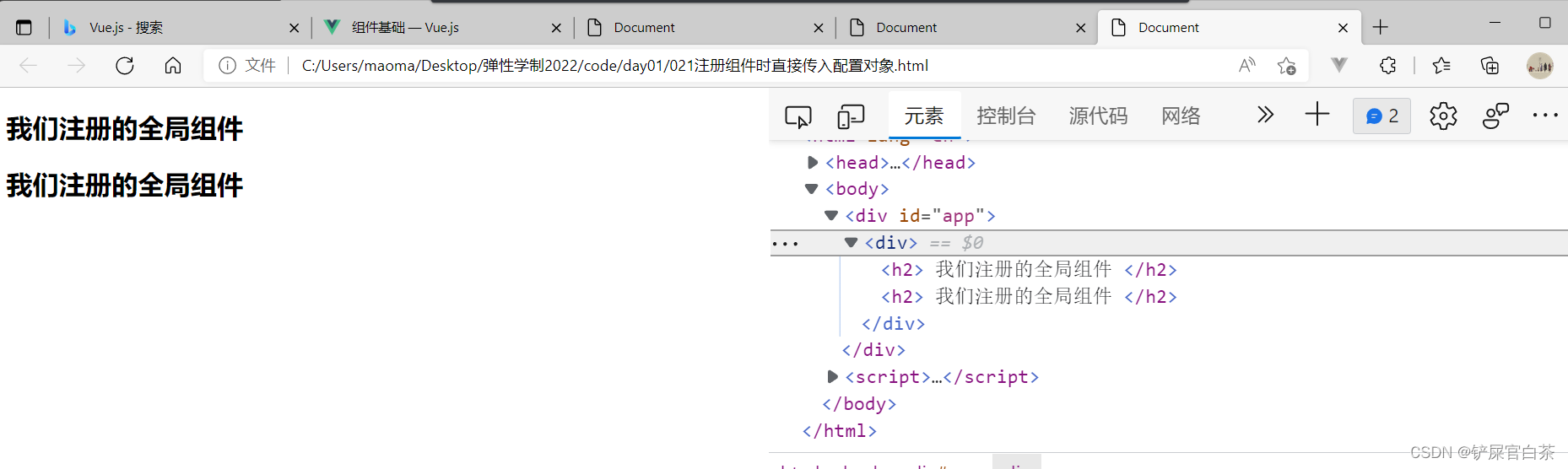
04.组件对象中的data和Vue实例选项中的data的不同
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./js/vue.js"></script>
</head>
<body>
<div id="app">
<button-count></button-count>
</div>
<script>
Vue.component("button-count",{
data: function(){
return {
count: 0
}
},
template: ` <button @click="count++">单击了{{count}}次的按钮</button> `
})
let vm = new Vue({
el: "#app",
})
</script>
</body>
</html>
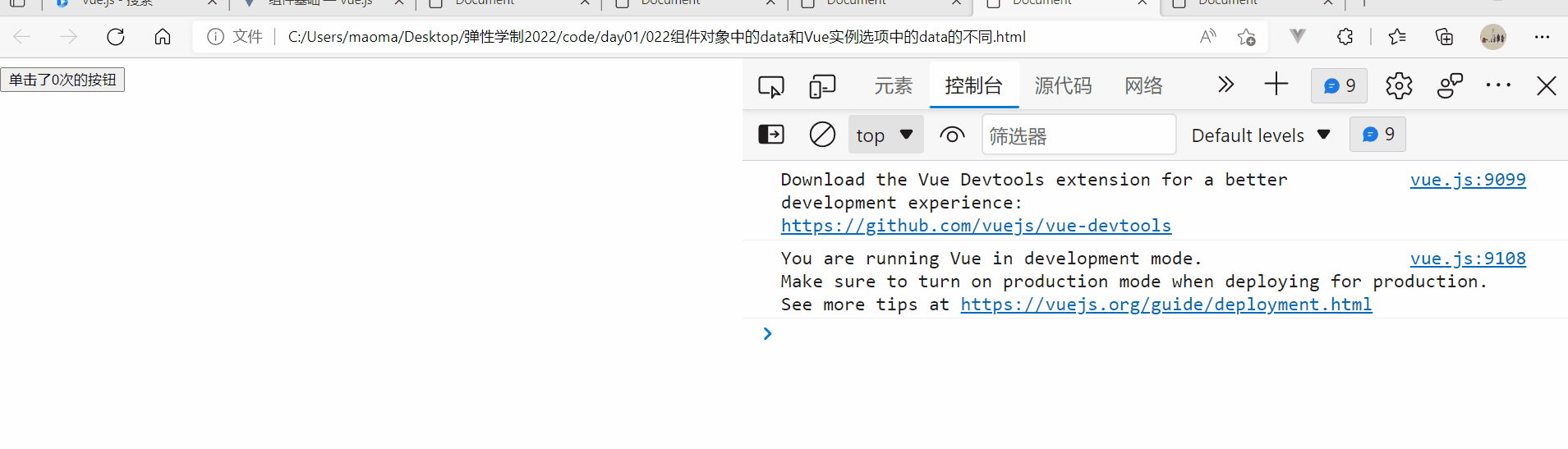
错误演示 当以对象的形式给组件中data赋值
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./js/vue.js"></script>
</head>
<body>
<div id="app">
<button-count></button-count>
<button-count1></button-count1>
</div>
<script>
Vue.component("button-count",{
data: function(){
return {
count: 0
}
},
template: ` <button @click="count++">单击了{{count}}次的按钮</button> `
})
Vue.component("button-count1",{
data: {
count: 0
},
template: ` <button @click="count++">单击了{{count}}次的按钮</button> `
})
let vm = new Vue({
el: "#app",
})
</script>
</body>
</html>
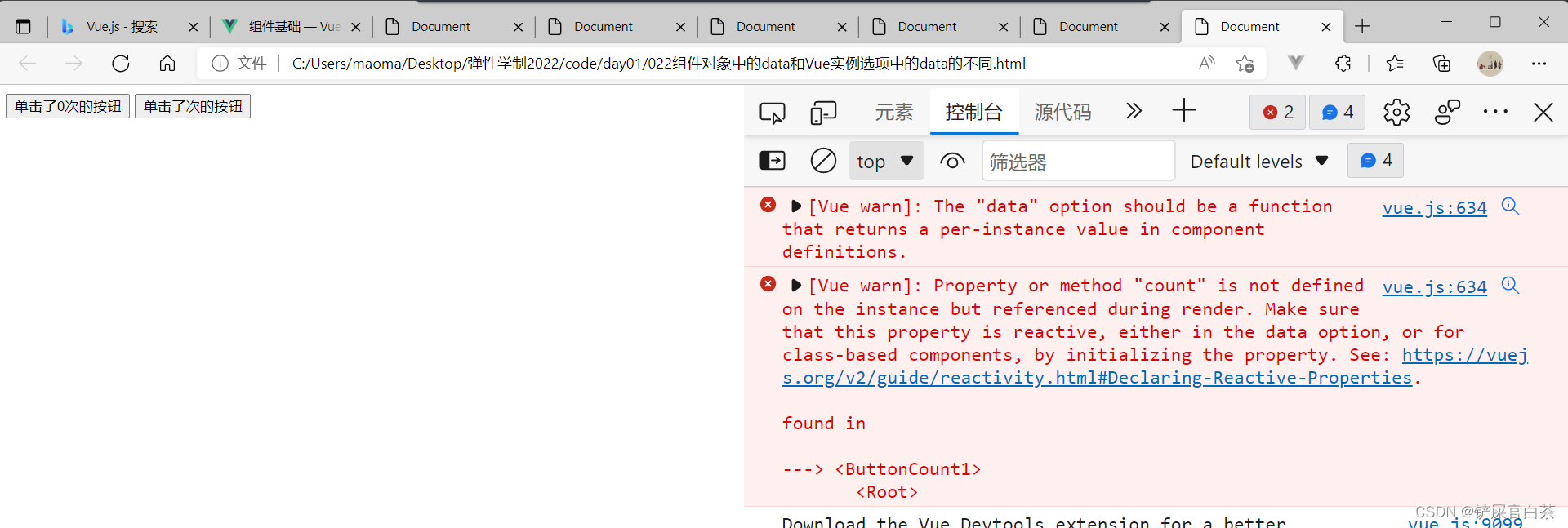
为什么非得用函数的形式给组件中data赋值-----是为了让组件被引用是时都有自己独立的数据域
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./js/vue.js"></script>
</head>
<body>
<div id="app">
<p> 引用3次相同的组件,组件中的数据相互不影响 </p>
<button-count></button-count>
<button-count></button-count>
<button-count></button-count>
</div>
<script>
Vue.component("button-count",{
data: function(){
return {
count: 0
}
},
template: ` <button @click="count++">单击了{{count}}次的按钮</button> `
})
let vm = new Vue({
el: "#app",
})
</script>
</body>
</html>
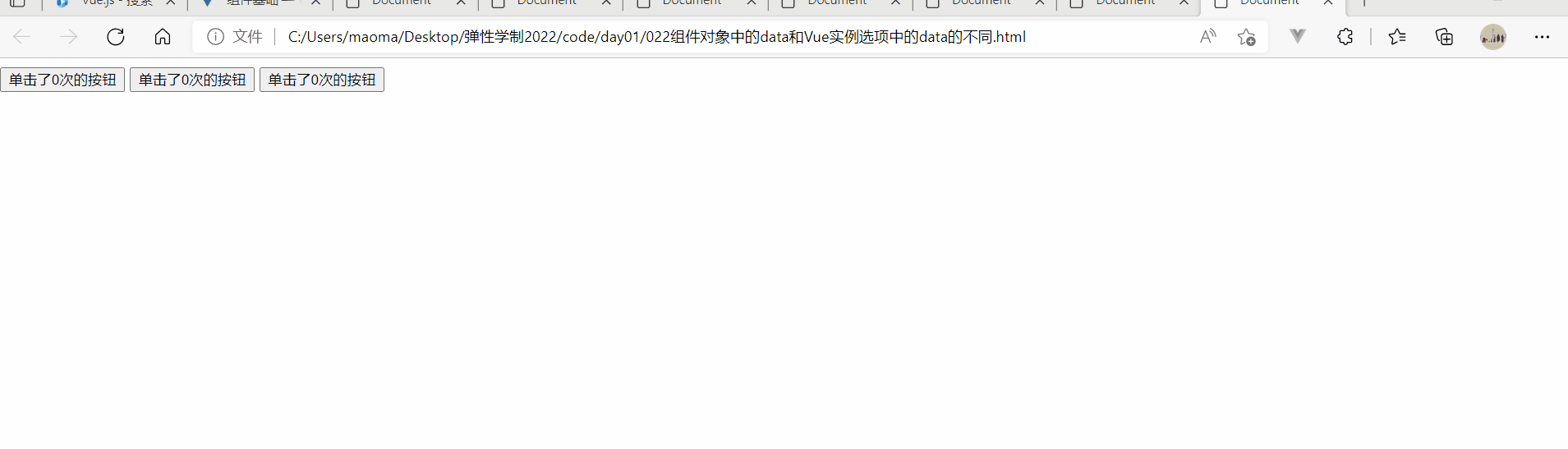
局部组件
01.定义局部组件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./js/vue.js"></script>
</head>
<body>
<div id="app">
<my-component></my-component>
</div>
<script>
let vm = new Vue({
el: "#app",
components: {
"my-component": {
template: ` <h2> 这是我的局部组件 </h2> `
}
}
})
</script>
</body>
</html>
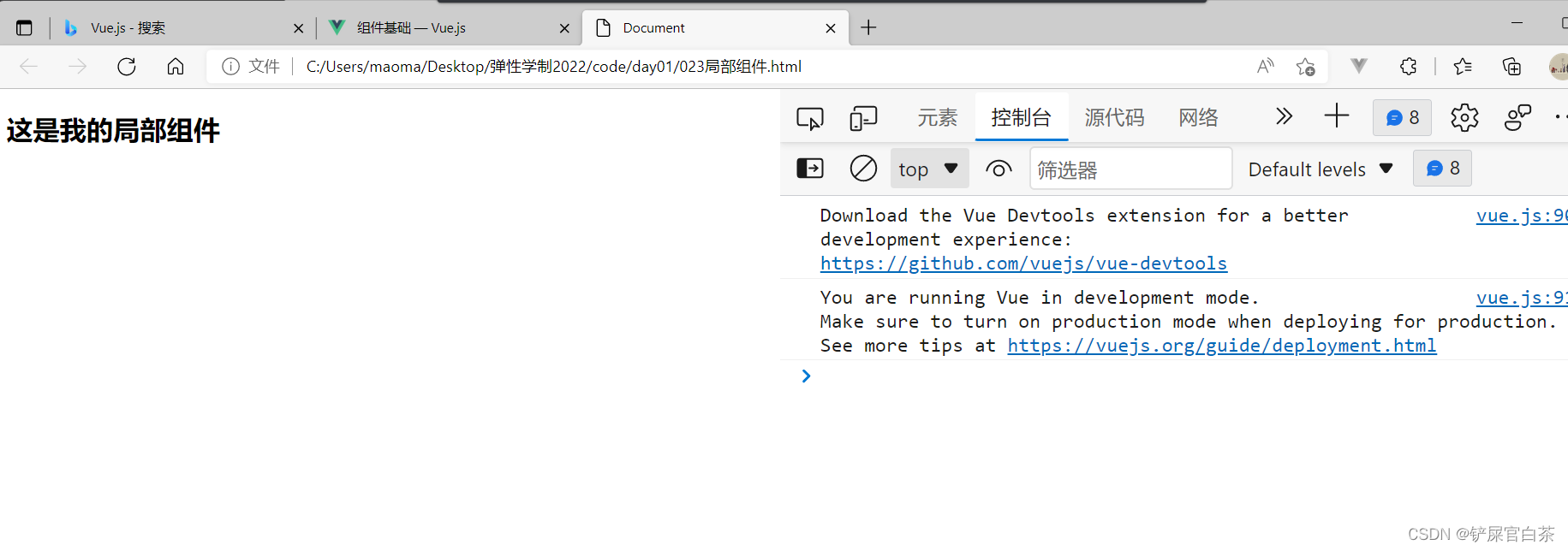
写法二
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./js/vue.js"></script>
</head>
<body>
<div id="app">
<my-component></my-component>
</div>
<script>
let child = {
template: ` <h2> 这是我的局部组件 </h2> `
}
let vm = new Vue({
el: "#app",
components: {
"my-component": child
}
})
</script>
</body>
</html>
写法三
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./js/vue.js"></script>
</head>
<body>
<div id="app">
<my-component></my-component>
</div>
<script>
let child = {
template: ` <h2> 这是我的局部组件 </h2> `
}
let mycom = {
"my-component": child
}
let vm = new Vue({
el: "#app",
components: mycom
})
</script>
</body>
</html>
02.子组件只能在父组件中使用
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./js/vue.js"></script>
</head>
<body>
<div id="app">
<parent></parent>
</div>
<script>
let child = {
template: ` <p> 这厮是子组件 </p> `
}
let parent = {
template: `
<div>
<p> 这厮是父组件 </p>
<child></child>
</div>
`,
components: {
"child": child
}
}
let vm = new Vue({
el: "#app",
components: {
"parent": parent
}
})
</script>
</body>
</html>
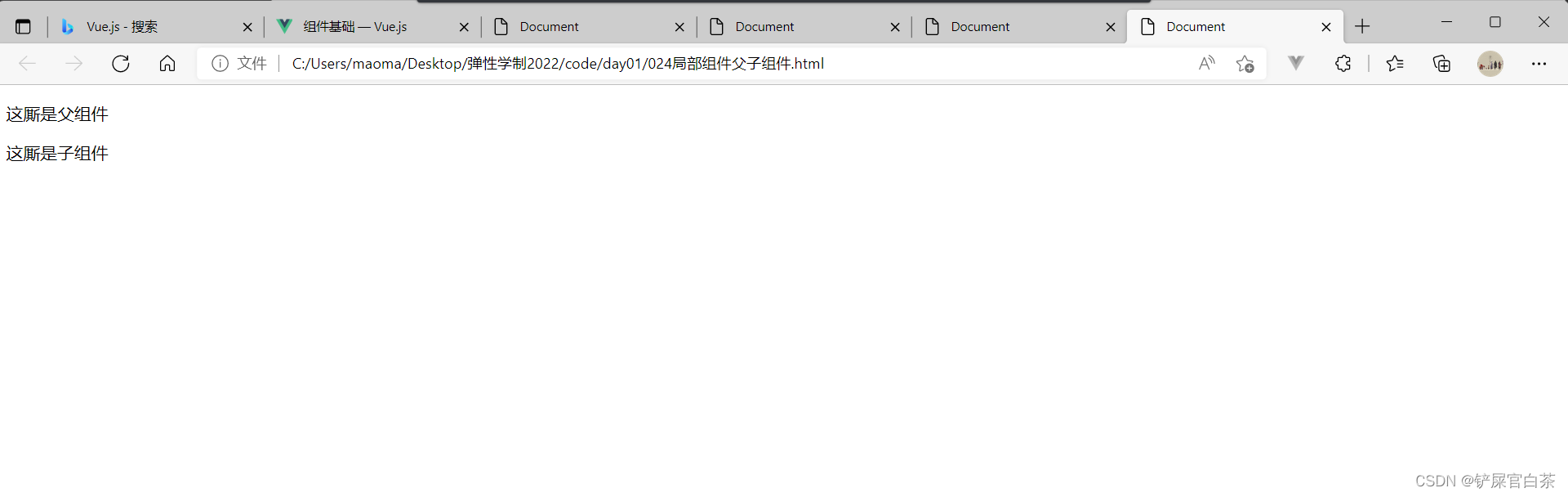
错误演示
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./js/vue.js"></script>
</head>
<body>
<div id="app">
<parent></parent>
<child></child>
</div>
<script>
let child = {
template: ` <p> 这厮是子组件 </p> `
}
let parent = {
template: `
<div>
<p> 这厮是父组件 </p>
<child></child>
</div>
`,
components: {
"child": child
}
}
let vm = new Vue({
el: "#app",
components: {
"parent": parent
}
})
</script>
</body>
</html>
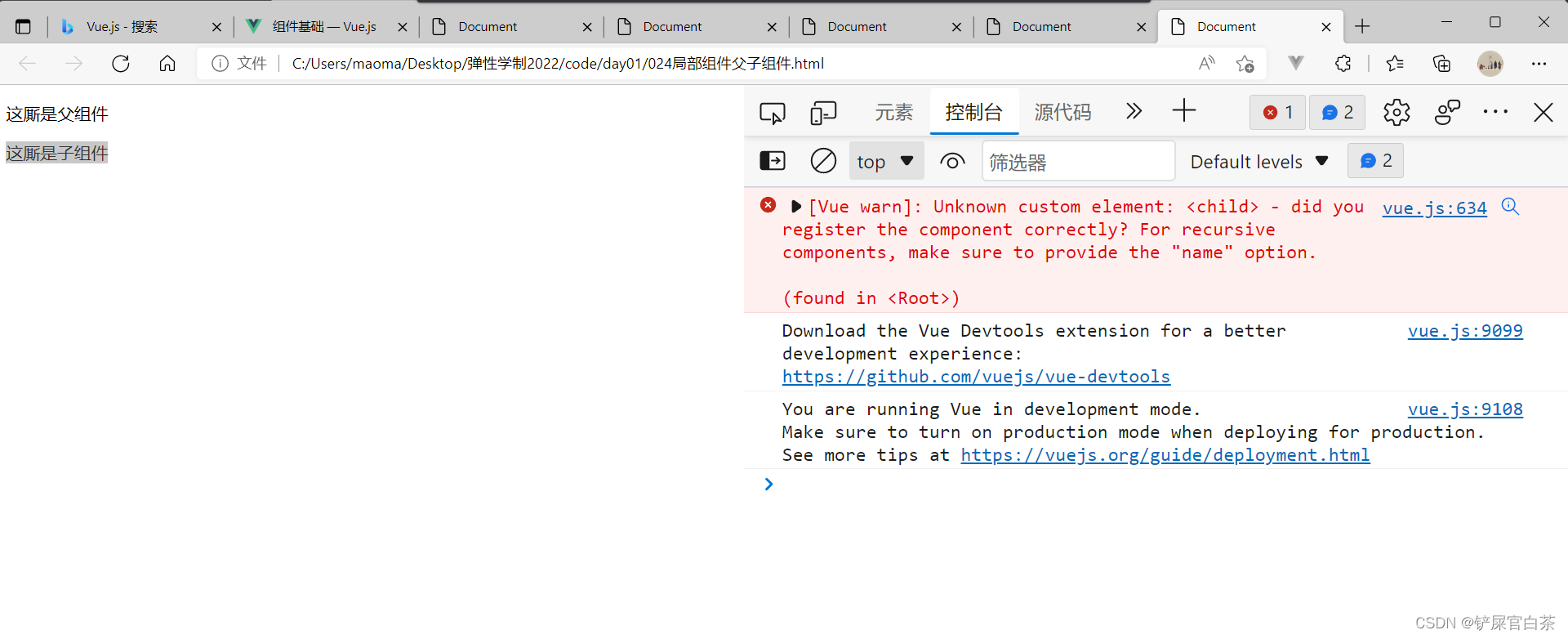
|