一、src/pages/postman/Task.vue文件
<template>
<div>
<a-card :bordered="false">
<div style="display: flex; flex-wrap: wrap">
<head-info title="我的待办" content="8个任务" :bordered="true"/>
<head-info title="本周任务平均处理时间" content="32分钟" :bordered="true"/>
<head-info title="本周完成任务数" content="24个"/>
</div>
</a-card>
<br />
<a-form
:model="formState"
name="horizontal_login"
layout="inline"
autocomplete="off"
@finish="onFinish"
@finishFailed="onFinishFailed"
>
<a-form-item
label="任务id"
name="task_id"
:rules="[{ required: true, message: 'Please input your task_id!' }]"
>
<a-input v-model="formState.task_id" />
</a-form-item>
<a-form-item
label="创建人"
name="creator"
:rules="[{ required: true, message: 'Please input your creator!' }]"
>
<a-select v-model="UserType" style="width:150px" allowClear>
<a-select-option :value="item.id" v-for="(item, i) in UserArr" :key="i">
{{ item.name }}
</a-select-option>
</a-select>
</a-form-item>
<a-form-item>
<a-button type="primary" html-type="submit" @click="onSearchData">查询</a-button>
<a-button :style="{ marginLeft: '8px' }" @click="reset">重置</a-button>
<a-button :style="{ marginLeft: '8px' }" @click="showModal">+任务</a-button>
<a-popconfirm
title="task执行后不可修改,你确定要执行吗?"
ok-text="确定"
cancel-text="取消"
@confirm="confirm"
@cancel="cancel"
>
<a-button :style="{ marginLeft: '8px' }" :disabled="!hasSelected" @click="start">批量执行</a-button>
</a-popconfirm>
</a-form-item>
<a-modal v-model="visible" width="500px" title="添加用例" @ok="handleOk">
<p>Some contents...</p>
</a-modal>
</a-form>
<a-table :columns="columns" :data-source="data" class="components-table-demo-nested" @change="onChanged" rowKey="id"
:row-selection="{ selectedRowKeys: selectedRowKeys, onChange: onSelectChange }">
<template #bodyCell="{ column }">
<template v-if="column.key === 'operation'">
<a>编辑</a>
</template>
</template>
<template #expandedRowRender>
<a-table :columns="innerColumns" :data-source="innerData" :pagination="false">
<template #bodyCell="{ column }">
<template v-if="column.key === 'operation'">
<span class="table-operation">
<a>Pause</a>
<a>Stop</a>
<a-dropdown>
<template #overlay>
<a-menu>
<a-menu-item>Action 1</a-menu-item>
<a-menu-item>Action 2</a-menu-item>
</a-menu>
</template>
<a>
More
<down-outlined />
</a>
</a-dropdown>
</span>
</template>
</template>
</a-table>
</template>
</a-table>
</div>
</template>
<script>
import HeadInfo from "@/components/tool/HeadInfo";
import { get_case_list, get_task_list } from "@/services/build_history";
import {getUserList} from "@/services/postman";
import {message} from "ant-design-vue";
const columns = [
{ title: '任务ID', dataIndex: 'id', key: 'id' },
{ title: '任务名称', dataIndex: 'task_name', key: 'task_name' },
{ title: '关联case数', dataIndex: 'bind_case', key: 'bind_case',
sorter: (a, b) => a.dataIndex - b.dataIndex,
defaultSortOrder: 'descend',
sortDirections: ['ascend', 'descend'] },
{ title: '所属需求', dataIndex: 'demand', key: 'demand'},
{ title: '状态', dataIndex: 'status', key: 'status' },
{ title: '创建人', dataIndex: 'creator', key: 'creator' },
{ title: '创建时间', dataIndex: 'createdAt', key: 'createdAt',
sorter: (a, b) => Date.parse(a.createdAt) - Date.parse(b.createdAt),
defaultSortOrder: 'descend',
sortDirections: ['ascend', 'descend'] },
{ title: '更新时间', dataIndex: 'updatedAt', key: 'updatedAt',
sorter: (a, b) => Date.parse(a.updatedAt) - Date.parse(b.updatedAt),
defaultSortOrder: 'descend',
sortDirections: ['ascend', 'descend'] },
{ title: '操作', key: 'operation' },
];
const innerColumns = [
{
title : '用例名称',
dataIndex: 'case_name',
resizable: true,
width: 20,
},
{
title : '优先级',
dataIndex: 'priority',
resizable: true,
width: 20,
},
{
title: '用例状态',
dataIndex: 'status',
resizable: true,
width: 20,
},
{
title: '创建人',
dataIndex: 'creator',
resizable: true,
width: 20,
},
{
title: '更新时间',
dataIndex: 'update_time',
resizable: true,
width: 50,
},
{
title: '操作',
dataIndex: 'operation',
resizable: true,
width: 50,
},
];
export default {
name: "Task",
data() {
return {
case_list: "http://localhost:7777/auth/case_list",
task_list:"http://localhost:7777/auth/task_list",
data:[],
columns,
innerColumns,
innerData:[],
formState: {
creator: '',
task_id: '',
},
form: this.$form.createForm(this, {name: 'task'}),
UserArr:[],
UserType:'',
visible:false,
hasSelected:false,
selectedRowKeys:[],
}
},
components: {HeadInfo},
methods: {
onSearchData() {
this.loading = true;
const task_id = this.formState.task_id
const creator = this.UserType
console.log(task_id,creator);
get_task_list(this.task_list, {task_id, creator})
.then((result) => {
this.loading = false;
this.data = result.data.data;
})
.catch((err) => {
this.data = err;
});
},
onSelectChange(selectedRowKeys){
this.selectedRowKeys = selectedRowKeys;
if (this.selectedRowKeys.length > 0) {
this.hasSelected = true
}
},
onChanged(pagination, filters, sorter) {
console.log('params', pagination, filters, sorter);
},
reset() {
this.UserType = ''
this.formState = []
this.data = []
},
start(){
this.loading = true;
setTimeout(() => {
this.loading = false;
this.selectedRowKeys = [];
}, 1000);
},
cancel(e) {
console.log(e);
message.error('Click on No');
},
confirm(e) {
console.log(e);
message.error('Click on Yes');
},
onFinish(val) {
console.log('endValue', val);
},
onFinishFailed(val) {
console.log('endValue', val);
},
showModal(){
this.visible = true;
},
handleOk(e){
console.log(e);
this.visible = false;
},
},
mounted() {
const task_id = 1
getUserList().then(res => {
this.UserArr = res.data.data
console.log('搜索条件',this.UserArr);
}),
get_case_list(this.case_list,{task_id}).then(res => {
this.loading = false;
this.innerData = res.data.data
if( this.innerData.length > 0) {
console.log('res:--- ', this.innerData);
}
})
},
}
</script>
<style scoped>
</style>
二、src/services/postman.js文件添加方法
export async function get_task_list(url, params) {
return request(url, METHOD.GET, params)
}
三、效果
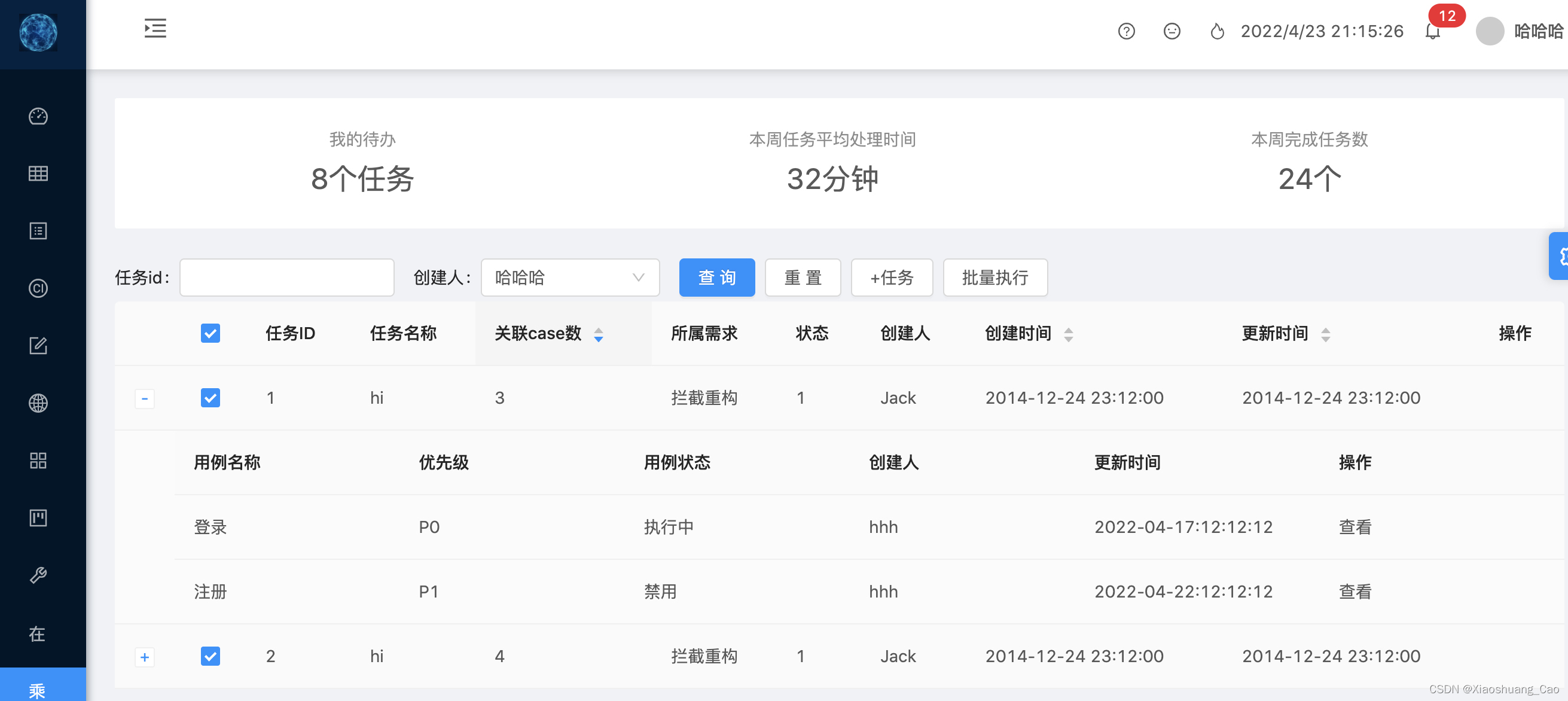
四、待解决问题
1、排序功能未生效 2、一些方法只是控制台打印,具体功能没实现
|