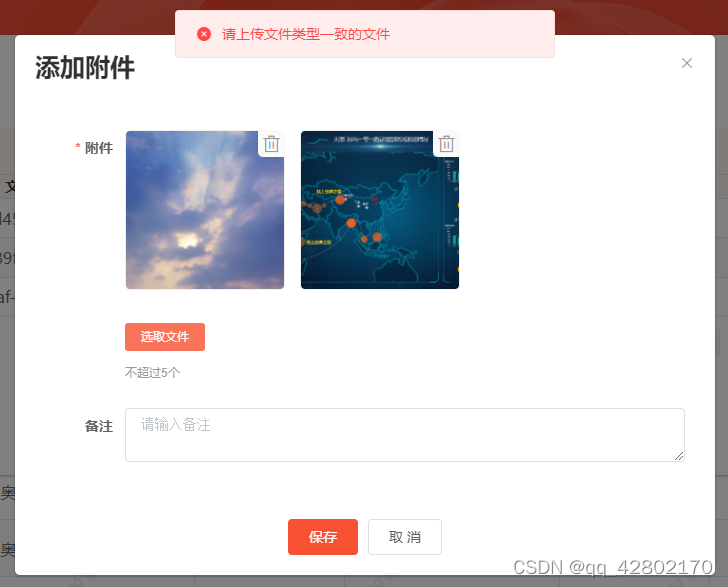
data() {
return {
IsImgOrFile: null,
isImage: null,
};
},
handleBeforeUpload(file) {
if(file && file.name){
var fileName = file.name;
var fileType = fileName.substring(fileName.lastIndexOf('.')+1);
var allFileType = ["pdf", "bmp", "webp", "dib", "gif", "jfif", "jpe", "jpeg", "jpg", "png", "tif", "tiff",
"ico", "pcx", "tga", "exif", "fpx", "svg", "psd", "cdr", "pcd", "dxf", "ufo", "eps", "ai", "raw", "WMF", "avif", "apng"]
this.isImage = allFileType.includes(fileType)
if(this.IsImgOrFile == null){
this.isIMGOrfileFUN()
}else if(this.IsImgOrFile && this.isImage){
this.isIMGOrfileFUN()
}else{
this.$message.error("请上传文件类型一致的文件")
return false
}
}
isIMGOrfileFUN(){
if(this.isImage){
this.isMultiple = true
this.showImage = true
this.limit = 5
this.IsImgOrFile = true
}else{
this.isMultiple = false
this.showImage = false
this.limit = 1
this.IsImgOrFile = false
}
},
图片和文件上传组件完整代码:
<template>
<div class="upload-file">
<!-- 图片列表 -->
<draggable class="row_wrap" v-if="showImage" @update="dragEvent" animation="300" draggable=".draggable-item">
<div v-for="(item,imgIndex) in list" :key="imgIndex" class="draggable-item upload-image mr15 mb15">
<el-image :src="item" :preview-src-list="list" fit="cover" :class="[isSquare?'square-image':'rectangle-image']"></el-image>
<li class="el-icon-delete" @click.stop="removeImage(imgIndex)"></li>
</div>
</draggable>
<!-- 文件列表 -->
<transition-group class="upload-file-list el-upload-list el-upload-list--text" name="el-fade-in-linear" tag="ul" v-else>
<li :key="index" class="el-upload-list__item ele-upload-list__item-content" v-for="(file, index) in list">
<el-link :href="file" target="_blank">
<span class="el-icon-document"> {{(file) }} </span>
</el-link>
<div class="ele-upload-list__item-content-action">
<el-link @click="handleDelete(index)" type="danger">删除</el-link>
</div>
</li>
</transition-group>
<el-upload :action="uploadFileUrl" :before-upload="handleBeforeUpload" :limit="limit" :on-error="handleUploadError" :on-exceed="handleExceed" :on-success="handleUploadSuccess" :show-file-list="false" :headers="headers" class="upload-file-uploader" ref="upload" v-if="list.length < limit" :multiple="isMultiple">
<!-- 上传按钮 -->
<el-button size="mini" type="primary">选取文件</el-button>
</el-upload>
<span class="font-xs text-grey">不超过{{limit}}{{isImage ? "张":"个"}}</span>
</div>
</template>
<script>
import { getToken, getTenantId } from "@/utils/auth";
import draggable from "vuedraggable"
export default {
components: {
draggable
},
props: {
value: [String, Object, Array],
fileSize: {
type: Number,
default: 5,
},
isShowTip: {
type: Boolean,
default: true,
},
teacherFileList: {
type: Array,
},
isSquare: {
type: Boolean,
default: true,
},
},
data() {
return {
uploadFileUrl: process.env.VUE_APP_BASE_API + "/file/uploadDocument",
headers: {
Authorization: "Bearer " + getToken(),
'tenantid': getTenantId(),
'waterMark': 0,
},
limit:5,
isMultiple:false,
showImage:true,
list: [],
IsImgOrFile: null,
isImage: null,
};
},
methods: {
dragEvent(item) {
let oldIndexImg = this.list[item.oldDraggableIndex]
let navIndexImg = this.list[item.newDraggableIndex]
this.list[item.oldDraggableIndex] = navIndexImg;
this.list[item.newDraggableIndex] = oldIndexImg;
},
handleBeforeUpload(file) {
if(file && file.name){
var fileName = file.name;
var fileType = fileName.substring(fileName.lastIndexOf('.')+1);
var allFileType = ["pdf", "bmp", "webp", "dib", "gif", "jfif", "jpe", "jpeg", "jpg", "png", "tif", "tiff",
"ico", "pcx", "tga", "exif", "fpx", "svg", "psd", "cdr", "pcd", "dxf", "ufo", "eps", "ai", "raw", "WMF", "avif", "apng"]
this.isImage = allFileType.includes(fileType)
if(this.IsImgOrFile == null){
this.isIMGOrfileFUN()
}else if(this.IsImgOrFile && this.isImage){
this.isIMGOrfileFUN()
}else{
this.$message.error("请上传文件类型一致的文件")
return false
}
}
if (this.fileSize) {
const isLt = file.size / 1024 / 1024 < this.fileSize;
if (!isLt) {
this.$message.error(`上传文件大小不能超过 ${this.fileSize} MB!`);
return false;
}
}
return true;
},
isIMGOrfileFUN(){
if(this.isImage){
this.isMultiple = true
this.showImage = true
this.limit = 5
this.IsImgOrFile = true
}else{
this.isMultiple = false
this.showImage = false
this.limit = 1
this.IsImgOrFile = false
}
},
handleExceed() {
this.$message.error("上传文件个数最多不超过"+this.limit+"个");
},
handleUploadError(err) {
this.$message.error("上传失败, 请重试");
},
handleUploadSuccess(res, file) {
if (res.code !== 200) {
this.$message.error(`${res.msg}`);
} else {
this.list.push(res.data.url);
this.$emit("input", this.list);
this.$message.success("上传成功");
}
},
handleDelete(index) {
this.list.splice(index, 1);
this.IsImgOrFileStart()
},
removeImage(i) {
this.list.splice(i, 1);
this.IsImgOrFileStart()
},
IsImgOrFileStart(){
if(this.list.length == 0){
this.IsImgOrFile = null
}
}
},
watch: {
value(newVal, oldVal) {
this.list = newVal;
},
waterMark(newVal, oldVal) {
this.headers.waterMark = newVal;
}
},
};
</script>
<style scoped lang="scss">
.upload-file-list .el-upload-list__item {
border: 1px solid #e4e7ed;
line-height: 2;
margin: 2px 0 8px 0px;
position: relative;
}
.upload-file-list .ele-upload-list__item-content {
display: flex;
justify-content: space-between;
align-items: center;
color: inherit;
}
.ele-upload-list__item-content-action .el-link {
margin-right: 10px;
}
.square-image {
width: 160px;
height: 160px;
}
.rectangle-image {
width: 160px;
height: auto;
}
.upload-image {
position: relative;
img {
border: 1px solid #efefef;
border-radius: 5px;
}
li {
position: absolute;
padding: 4px;
top: 0px;
right: 0px;
font-size: 19px;
border-top-right-radius: 5px;
border-bottom-left-radius: 5px;
color: #999;
background-color: #fafafa;
cursor: pointer;
}
}
.draggable-item {
background: transparent !important;
}
</style>
|