json
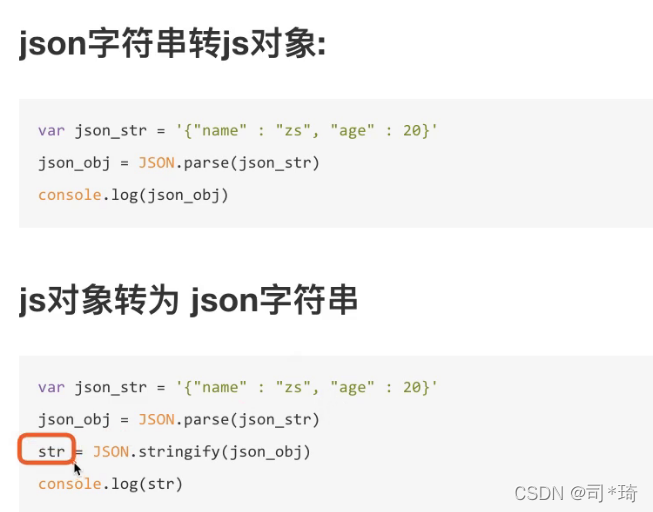
vue-事件修饰符
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.box {
width: 300px;
height: 300px;
background-color: aqua;
}
.minbox {
width: 100px;
height: 100px;
background-color: blue;
}
</style>
<script src="./jquery/vue.js"></script>
<script>
window.onload=function(){
new Vue ({
el:'#app',
data:{
},
methods:{
firstClicked:function(){
alert('box')
},
secondClicked:function(){
alert('minbox')
}
}
})
}
</script>
</head>
<body>
<div id="app">
<div class="box" @click="firstClicked">
<div class="minbox" @click.stop.prevent="secondClicked">
</div>
</div>
</div>
</body>
</html>
练习题-弹窗
第一种–jquery:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="./main.css">
<script src="./jquery/jquery-1.12.4.min.js"></script>
<script>
$(function(){
$('#btn01').click(function(){
$('#pop').show()
})
$('#shutoff').click(function(){
$('#pop').hide()
})
$('.mask').click(function(){
$('#pop').hide()
})
})
</script>
</head>
<body>
<input type="button" value="弹出弹窗" id="btn01">
<div class="pop_main" id="pop">
<div class="pop_con">
<div class="pop_title">
<h3>系统提示</h3>
<a href="#" id="shutoff">x</a>
</div>
<div class="pop_detail">
<p class="pop_text">亲!请关注近期的优惠活动!</p>
</div>
<div class="pop_footer"></div>
</div>
<div class="mask"></div>
</div>
</body>
</html>
第二种–vue:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>聊天</title>
<style>
.outBox {
width: 322px;
height: 360px;
border: 1px solid black;
}
.box {
width: 300px;
height: 300px;
border: 1px solid black;
margin: 10px;
overflow:auto
}
select {
margin-left: 10px;
}
.bTalk {
text-align: left;
}
.aTalk span{
background-color: blue;
color: aliceblue;
}
.bTalk {
text-align: right;
}
.bTalk span{
background-color: gold;
color: aliceblue;
}
</style>
<script src="../jquery/vue.js"></script>
<script>
window.onload=function(){
new Vue({
el:"#app",
data:{
inputValue:'',
sayValue:'0',
array:[
{who:"A",said:"还没吃吗?"},
{who:"B",said:"吃了,你呢?"}
]
},
methods:{
clicked:function(){
if (this.inputValue==''){
alert('输入内容为空,请重新输入')
return
}
this.array.push({who:(this.sayValue=='0'?'A':'B'),said:this.inputValue})
this.inputValue=''
}
}
})
}
</script>
</head>
<body>
<div id="app">
<div class="outBox">
<div class="box" id="words">
<div v-for="item in array" :class="[item.who=='A'?'aTalk':'bTalk']"><span>{{item.who}}说:{{item.said}}</span></div>
</div>
<div>
<select name="" id="input02" v-model="sayValue">
<option value="0" id="aSay">A说</option>
<option value="1" id="bSay">B说</option>
</select>
<input type="text" id="input01" v-model="inputValue">
<input @click="clicked" type="button" value="提交" id="btn">
</div>
</div>
</div>
</body>
</html>
过滤器
内置过滤器
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./jquery/vue.js"></script>
<script>
window.onload=function(){
new Vue({
el:'#app',
data:{
msg:"hello word"
},
filters:{
reverse:function(value){
return value.split('').reverse().join('')
}
}
})
}
</script>
</head>
<body>
<div id="app">
{{msg}}
<br>
{{msg | reverse}}
</div>
</body>
</html>
外置过滤器
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./jquery/vue.js"></script>
<script>
window.onload =function(){
Vue.filter('upper',function(value){
return value.toUpperCase()
})
Vue.filter('lower',function(value){
return value.toLowerCase()
})
new Vue({
el:"#app",
data:{
msg:"hello word"
}
})
}
</script>
</head>
<body>
<div id="app">
{{msg|upper}}
<br>
{{msg|upper|lower}}
</div>
</body>
</html>
自定义指令
外部
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./jquery/vue.js"></script>
<script>
window.onload=function(){
Vue.directive('style',{
inserted:function(el){
el.style.width='300px',
el.style.height='300px',
el.style.background='blue'
}
}
)
new Vue({
el:"#app",
})
}
</script>
</head>
<body>
<div id="app">
<div v-style></div>
</div>
</body>
</html>
内部
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./jquery/vue.js"></script>
<script>
window.onload=function(){
Vue.directive('style',{
inserted:function(el){
el.style.width='300px',
el.style.height='300px',
el.style.background='blue'
}
}
)
new Vue({
el:"#app",
directives:{
'focus':{
inserted:function(el){
el.focus()
el.style.background='pink'
}
}}
})
}
</script>
</head>
<body>
<div id="app">
<input type="text" v-focus>
</div>
</body>
</html>
定时器之箭头函数
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script>
setInterval(()=>{
alert('func')},3000)
</script>
</head>
<body>
</body>
</html>
|