基础学习
在学习本篇小案例之前,请确保你已经学习了react基础知识,如果没有学,可以进行基础学习后再来这里,基础学习传送门
todolist
包含展示任务,添加任务和删除任务功能这几个小功能 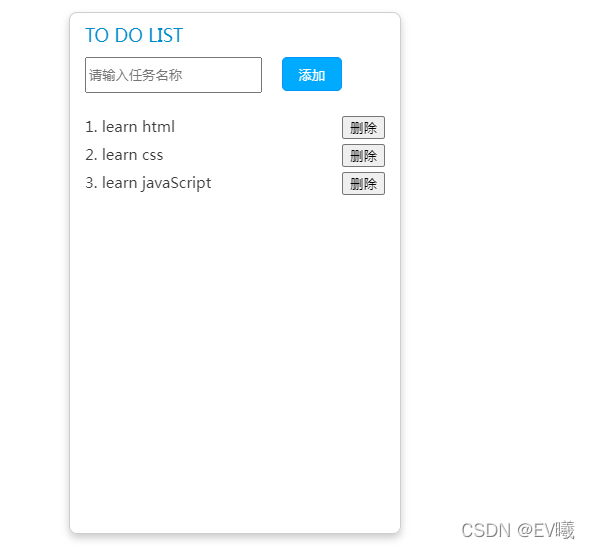
分析:任务清单的展示需要用到数组,因此我们需要在state中定义一个清单数组,添加功能需要取输入框中的值,因此需要在state中定义变量去存输入框中的值,添加完毕需要更新展示清单列表,因此需要用到setState去设置清单数组的值,删除对应的任务,需要传任务的下标值进行对应任务的删除。
初始赋值
输入框value绑定值,以及list清单数组
this.state={
inputValue:'',
doList:[
'learn html',
'learn css',
'learn javaScript',
]
}
列表展示
使用数组方法map进行数组遍历,并在每个任务后添加删除按钮
<ul>
{
this.state.doList.map((item,index)=>{
return <li key={index}>
<div>
<span>{index+1}. {item}</span>
<button onClick={this.delete.bind(this,index)}>删除</button>
</div>
</li>
})
}
</ul>
添加功能
输入框value绑定state中定义的inputValue变量,onChange事件绑定getValue方法用于实时获取输入框中的值,点击事件绑定add方法设置state中的变量值,去更新清单数组以及添加后清空输入框
<input type="text" value={this.state.inputValue} onChange={this.getValue.bind(this)} placeholder="请输入任务名称"/>
<button id="addbnt" onClick={this.add.bind(this)}>添加</button>
add(){
this.setState({
doList:[...this.state.doList,this.state.inputValue],
inputValue:''
})
}
删除功能
点击事件绑定delete方法并传入需删除任务的下标值,使用数组splice进行删除,并重置清单数组
delete(index){
this.state.doList.splice(index,1);
this.setState({
doList: this.state.doList
})
}
完整代码
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script src="https://cdn.staticfile.org/react/16.4.0/umd/react.development.js"></script>
<script src="https://cdn.staticfile.org/react-dom/16.4.0/umd/react-dom.development.js"></script>
<script src="https://cdn.staticfile.org/babel-standalone/6.26.0/babel.min.js"></script>
<style type="text/css">
#container{
width: 300px;
min-height: 500px;
margin: 50px 100px;
padding: 10px 15px;
border: #cecece 1px solid;
border-radius: 8px;
box-shadow: #CECECE;
box-shadow: 0 4px 8px 0 rgba(0,0,0,0.2);
}
#title{
color: #008bcc;
font-size: 18px;
}
input{
height: 30px;
}
input:hover,input:active,input:focus{
border: #0296ff 0.5px solid;
outline-color: #00AAFF;
}
#addbnt{
width: 60px;
height: 34px;
background-color: #00aaff;
color: #ffffff;
border: #0296ff 1px solid;
margin: 10px 20px;
border-radius: 5px;
}
ul{
padding: 0 !important;
list-style: none;
font-size: 15px;
color: #3d3d3d;
}
li div{
display: flex;
justify-content: space-between;
margin-bottom: 5px;
}
</style>
</head>
<body>
<div id="example"></div>
<script type="text/babel">
class Todolist extends React.Component{
constructor(props){
super(props);
this.state={
inputValue:'',
doList:[
'learn html',
'learn css',
'learn javaScript',
]
}
}
getValue(event){
this.setState({
inputValue: event.target.value
})
}
add(){
this.setState({
doList:[...this.state.doList,this.state.inputValue],
inputValue:''
})
}
delete(index){
this.state.doList.splice(index,1);
this.setState({
doList: this.state.doList
})
}
render(){
return(
<div id="container">
<div id="title">TO DO LIST</div>
<input type="text" value={this.state.inputValue} onChange={this.getValue.bind(this)} placeholder="请输入任务名称"/>
<button id="addbnt" onClick={this.add.bind(this)}>添加</button>
<ul>
{
this.state.doList.map((item,index)=>{
return <li key={index}>
<div>
<span>{index+1}. {item}</span>
<button onClick={this.delete.bind(this,index)}>删除</button>
</div>
</li>
})
}
</ul>
</div>
)
}
}
ReactDOM.render(
<Todolist/>,
document.getElementById('example')
)
</script>
</body>
</html>
|