大家好呀,我是小笙,我学习了韩老师VIP课程,以下是我的javascript学习笔记,和大家分享一下
javascript
基本概念
W3school教程
概念:JavaScript能改变 HTML内容,能改变 HTML属性,能改变 CSS 样式,能完成 页面的数据验证
特点:
-
JavaScript 是一种解释型的脚本语言,C、C++等语言先编译后执行,而 JavaScript 是在程序 的运行过程中逐行进行解释 -
JavaScript 是一种基于对象的脚本语言,可以创建对象,也能使用现有的对象(有对象) -
JavaScript 是弱类型的,对变量的数据类型不做严格的要求,变量的数据类型在运行过程可以变化  <!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
var age = 80;
console.log(typeof age);
age = "罗念笙";
console.log(typeof age);
</script>
</head>
<body>
</body>
</html>
-
跨平台性(只要是可以解释 JS 的浏览器都可以执行,和平台无关)
两种方式使用javascript语句
1.方式一:在script中书写
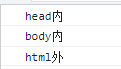
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
console.log("head内")
</script>
</head>
<body>
<script type="text/javascript">
console.log("body内")
</script>
</body>
</html>
<script type="text/javascript">
console.log("html外")
</script>
2.方式二:外部引入
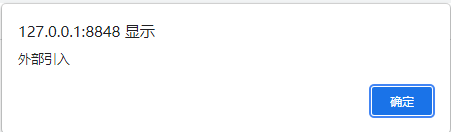
alert("外部引入");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript" src="js/demo.js"></script>
</head>
<body>
</body>
</html>
变量
概念:变量表示存储数据的容器
数据类型介绍
- 数值类型: number
- 字符串类型: string
- 对象类型:object
- 布尔类型: boolean
- 函数类型: function
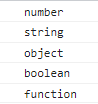
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
var test = 100;
console.log(typeof test);
test = "100";
console.log(typeof test);
test = document.getElementById("body");
console.log(typeof test);
test = true;
console.log(typeof test);
test = new Function();
console.log(typeof test);
</script>
</head>
<body id="body">
</body>
</html>
特殊值
- undefined 变量未赋初始值时,默认 undefined
- null 空值
- NaN Not a Number 非数值
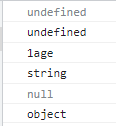
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
var test;
console.log(test);
console.log(typeof test);
test = 1 + 'age';
console.log(test);
console.log(typeof test);
test = null;
console.log(test);
console.log(typeof test);
</script>
</head>
<body>
</body>
</html>
运算符
算术运算符
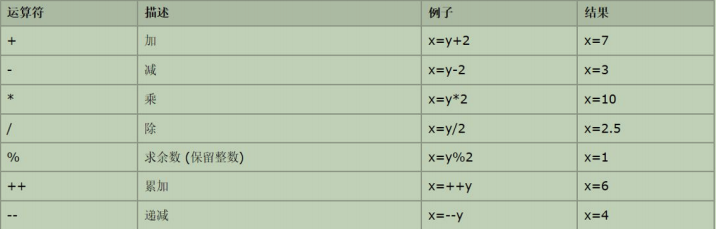
赋值运算符
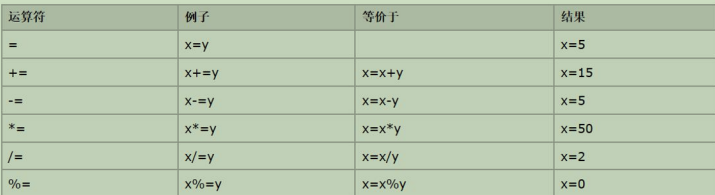
关系运算符
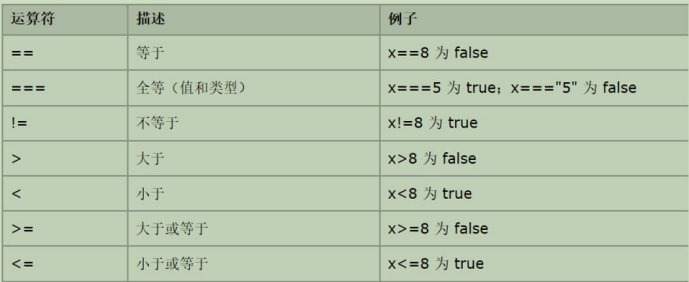
注意区别 == 和 ===

<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
a = "100";
b = 100;
console.log(a == b);
console.log(a === b)
</script>
</head>
<body>
</body>
</html>
逻辑运算符
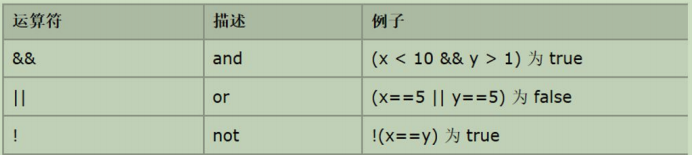
注意 && 和 | | 的使用
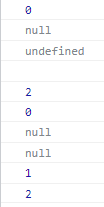
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
if(!0) console.log(0);
if(!null) console.log(null);
if(!undefined) console.log(undefined);
if(!"") console.log("");
console.log(1 && 2);
console.log(0 && null);
console.log(1 && null);
console.log(0 || null);
console.log(1 || 2);
console.log(0 || 2);
</script>
</head>
<body>
</body>
</html>
条件符运算
类似Java的三元运算符
<script type="text/javascript">
varres = 5 > 0?"hello":"ok";
alert(res);
</script>
数组
javascript数组定义使用的4种方式
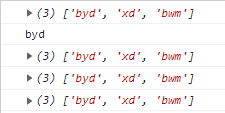
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
var cars = ["byd","xd","bwm"];
console.log(cars);
console.log(cars[0]);
var cars2 = [];
cars2[0] = "byd";
cars2[1] = "xd";
cars2[2] = "bwm";
console.log(cars2);
var cars3 = new Array("byd","xd","bwm");
console.log(cars3)
var cars4 = new Array();
cars4[0] = "byd";
cars4[1] = "xd";
cars4[2] = "bwm";
console.log(cars4);
</script>
</head>
<body>
</body>
</html>
数组使用和遍历
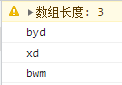
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
var cars = ["byd","xd","bwm"];
console.warn("数组长度: " + cars.length)
for(i = 0;i< cars.length;i++){
console.log(cars[i]);
}
</script>
</head>
<body>
</body>
</html>
函数
函数概念
概念:函数是由事件驱动的,或者当它被调用时,执行的可重复使用的代码
在 js 中如果要执行函数,有两种方式
- 主动调用函数
- 通过事件去触发该函数
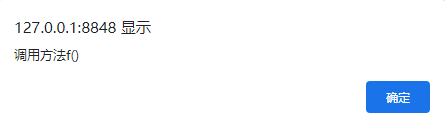
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
function f(){
alert("调用方法f()");
}
f();
</script>
</head>
<body>
<button type="button" onclick="f()">按钮</button>
</body>
</html>
函数定义方式
方式 1: function 关键字来定义函数
function 函数名(形参列表){
函数体
return 表达式
}
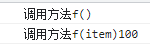
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
function f(){
console.log("调用方法f()");
}
function f2(item){
console.log("调用方法f(item)"+item);
}
f();
f2(100);
</script>
</head>
<body>
</body>
</html>
方式 2: 将函数赋给变量
var 函数名 = function(形参列表){
函数体
return 参数列表
}
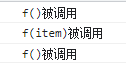
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
var f = function(){
console.log("f()被调用")
}
var f2 = function(item){
console.log("f(item)被调用")
}
f();
f2(100);
var f3 = f;
f3();
</script>
</head>
<body>
</body>
</html>
JavaScript 函数注意事项和细节
-
如果函数的函数名相同,形参列表个数最多的会覆盖掉其他相同名字的函数  <!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
function f(){
console.log("f()");
}
function f(item1){
console.log("f(item1)");
}
function f(item1,item2){
console.log("f(item1,item2)");
}
f();
f(1);
f(1,1);
</script>
</head>
<body>
</body>
</html>
-
函数的 arguments 隐形参数(作用域在 function 函数内)
- 隐形参数: 在 function 函数中不需要定义,可以直接用来获取所有参数的变量
- 隐形参数特别像 java 的可变参数一样(例如:void fun(int… args) )
 <!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
function f(){
var sum = 0;
for(i = 0;i < arguments.length;i++){
sum += arguments[i];
}
console.log(sum);
}
f(100,200,300);
</script>
</head>
<body>
</body>
</html>
函数课堂练习: 编写一个函数,用于计算所有参数相加的和并返回,如果实参不是number, 就过滤掉 <!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
function sum(){
var sum = 0;
for(i = 0;i < arguments.length;i++){
if(!isNaN(arguments[i])){
sum += arguments[i];
}
}
alert(sum);
}
sum(25,54,65,98,52,41,51,-5,0,"asd");
</script>
</head>
<body>
</body>
</html>
自定义对象
自定义对象方式 1:Object 形式
var 对象 = new Object();
对象名.属性名 = 值;
对象名.函数名 = function();
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
var person = new Object();
person.name = "罗念笙";
person.eat = function(){
console.log("eating");
}
person.eat();
</script>
</head>
<body>
</body>
</html>
自定义对象方式 2:{} 形式
var 对象名 = {
属性名:值,
函数名:function(){}
};
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
var person = {
name:"张洛融",
run:function(){
alert("running")
}
}
console.log(person.name);
person.run();
</script>
</head>
<body>
</body>
</html>
事件
概念:事件是电脑输入设备与页面进行交互的响应 (事件通常与函数配合使用)
常见的事件
事件 | 描述 |
---|
onchange | HTML 元素已被改变 | onclick | 用户点击了 HTML 元素 | onmouseover | 用户把鼠标移动到 HTML 元素上 | onmouseout | 用户把鼠标移开 HTML 元素 | onkeydown | 用户按下键盘按键 | onload | 浏览器已经完成页面加载 | onblur | 元素失去焦点 |
事件分类
事件注册(绑定):当事件响应(触发)后要浏要览器执行哪些操作代码,叫 事件注册或事件绑定
静态注册事件: 通过 html 标签的事件属性直接赋于事件响应后的代码,这种方式叫 静态注册
’
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
function f(){
console.log("f()被调用")
}
</script>
</head>
<body>
<button type="button" onclick="f()">按钮</button>
</body>
</html>
动态注册事件: 通过 js 代码得到标签的 dom 对象,然后再通过 dom 对象.事件名 = function(){} 这种形式叫 动态注册
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
window.onload = function(){
var btn01 = document.getElementById("btn");
btn01.onclick = function(){
alert("点击按钮");
}
}
</script>
</head>
<body>
<button type="button" id="btn">按钮</button>
</body>
</html>
练习表单提交事件
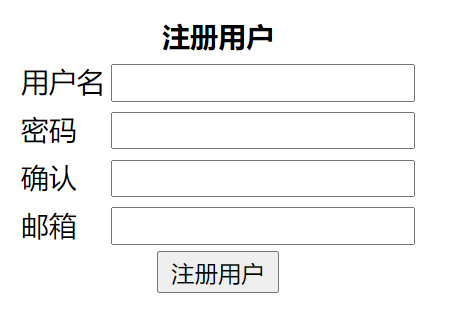
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script type="text/javascript">
window.onload = function(){
var from = document.getElementById(form);
form.onsubmit = function(){
if(form.text.value.length < 4 || form.text.value.length > 6){
alert("用户名不正确");
return false;
}
if(form.pwd1.value.length != 6){
alert("密码不正确");
return false;
}
if(form.pwd2.value.length != 6){
alert("确认密码不正确");
return false;
}
if(form.pwd1.value != form.pwd2.value){
alert("密码和确认密码不相同");
return false;
}
var emailPattern = /^[\w-]+@([a-zA-Z]+\.)+[a-zA-Z]+$/;
if(!(emailPattern.test(form.email.value))){
alert("电子邮件格式不匹配");
return false;
}
}
}
</script>
</head>
<body>
<table align="center">
<form action="404.html" method="get" id="form">
<tr><th colspan="2">注册用户</th></tr>
<tr>
<td>用户名</td>
<td><input type="text" name="text"/></td>
</tr>
<tr>
<td>密码</td>
<td><input type="password" name="pwd1"/></td>
</tr>
<tr>
<td>确认</td>
<td><input type="password" name="pwd2"/></td>
</tr>
<tr>
<td>邮箱</td>
<td><input type="text" name="email"/></td>
</tr>
<tr align="center">
<td colspan="2"><input type="submit" value="注册用户" /></td>
</tr>
</form>
</table>
</body>
</html>
|