一、测试代理的服务器的配置和启动,等待React发起请求
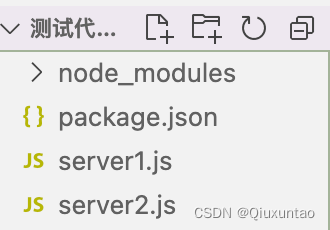
1.1、package.json
{
"name": "server",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"express": "^4.17.1"
}
}
1.2、server1.js
const express = require('express')
const app = express()
app.use((request,response,next)=>{
console.log('有人请求服务器1了');
console.log('请求来自于',request.get('Host'));
console.log('请求的地址',request.url);
next()
})
app.get('/students',(request,response)=>{
const students = [
{id:'001',name:'tom',age:18},
{id:'002',name:'jerry',age:19},
{id:'003',name:'tony',age:120},
]
response.send(students)
})
app.listen(5001,(err)=>{
if(!err) console.log('服务器1启动成功了,请求学生信息地址为:http://localhost:5001/students');
})
1.3、server2.js
const express = require('express')
const app = express()
app.use((request,response,next)=>{
console.log('有人请求服务器2了');
next()
})
app.get('/cars',(request,response)=>{
const cars = [
{id:'001',name:'奔驰',price:199},
{id:'002',name:'马自达',price:109},
{id:'003',name:'捷达',price:120},
]
response.send(cars)
})
app.listen(5002,(err)=>{
if(!err) console.log('服务器2启动成功了,请求汽车信息地址为:http://localhost:5002/cars');
})
1.4、node_modules
在终端里面执行 npm install
npm install
1.5、启动服务器(server1.js)

1.6、浏览器get请求数据
http://localhost:5001/students
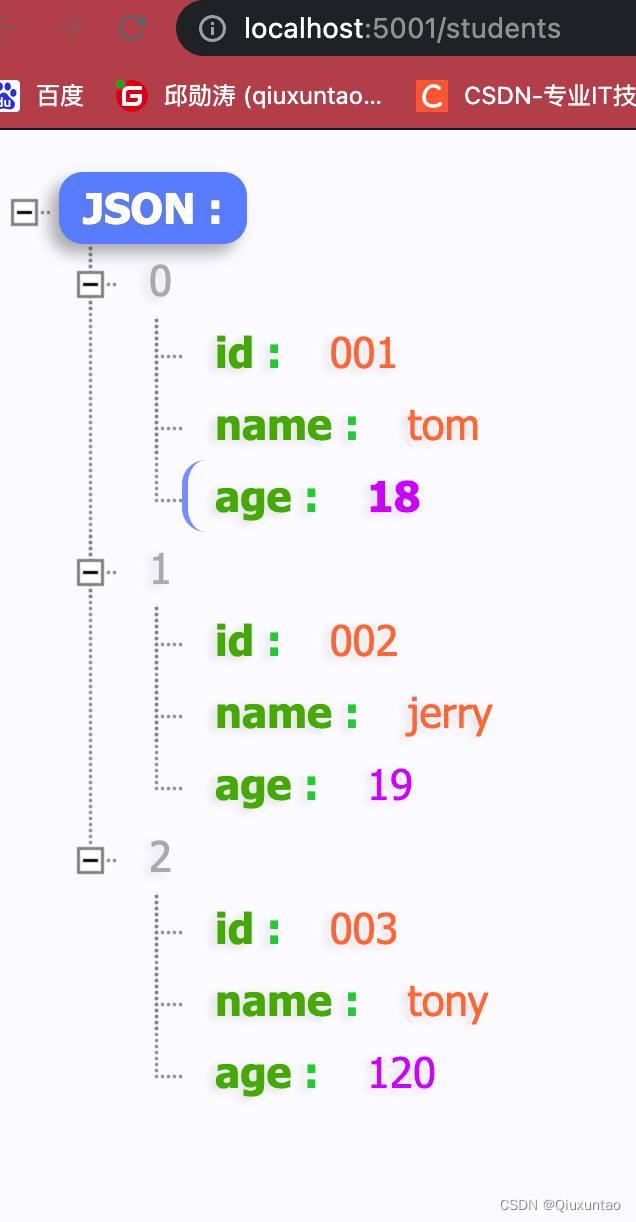
二、React中的配置代理解决跨域问题的两种方法
需求
2.1、方法1(不推荐)
在react脚手架的package.json 里面配置proxy
"proxy":"http://localhost:5001"
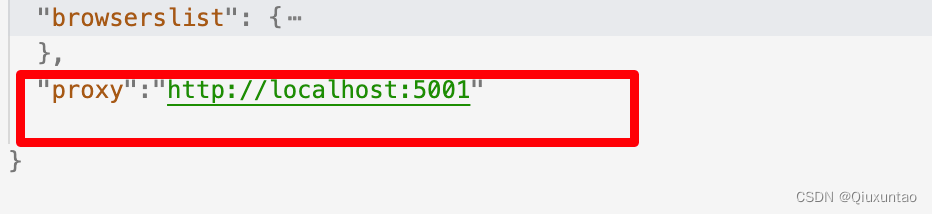
import React, { Component } from 'react'
import axios from 'axios'
export default class App extends Component {
getStudentData = ()=>{
axios.get('http://localhost:3000/students').then(
response => {console.log('成功了',response.data);},
error => {console.log('失败了',error);}
)
}
render() {
return (
<div>
<button onClick={this.getStudentData}>点我获取学生数据</button>
</div>
)
}
}
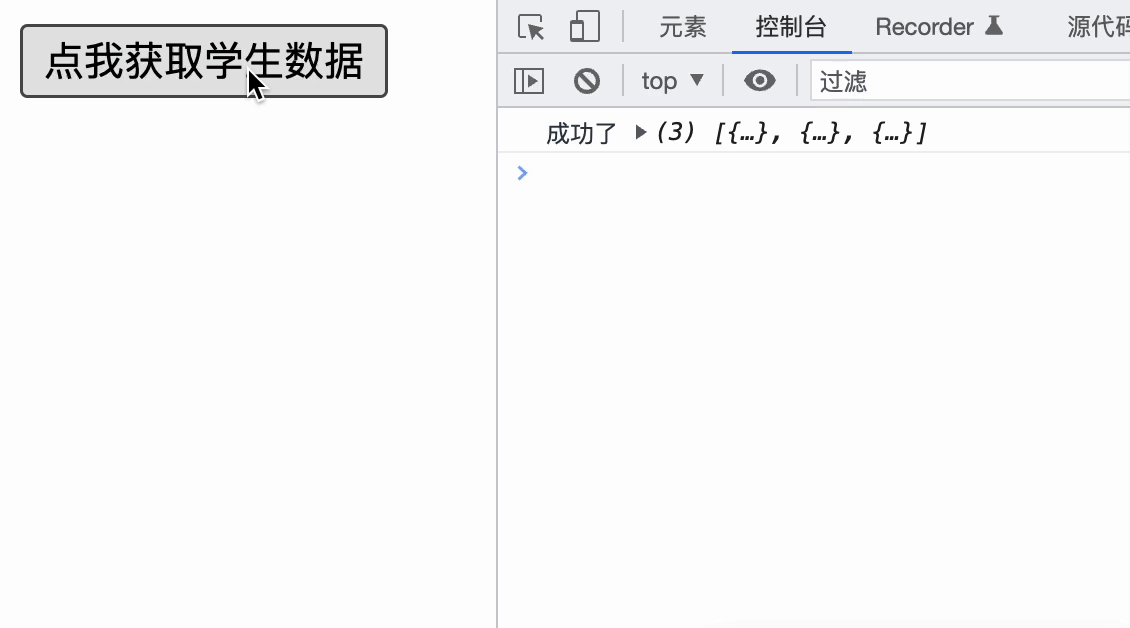
2.2.1、总结
优点 :配置简单,前端请求资源时可以不加任何前缀(api1) 缺点 :不能配置多个代理,满足不了多个代理同时执行 工作方式 :上述方式配置代理,当请求了3000不存在的资源时,那么该请求会转发给5001 (优先匹配react前端资源,如果有,那么返回前端有的,没有再去找服务器的)
|