DOM-获取DOM元素,修改属性
web API 基本认知
- 作用和分类
作用:就是使用JS去操作html和浏览器 分类:DOM(文档对象模型),BOM(浏览器对象模型) - 什么是DOM
(文档对象模型)是用来呈现以及与任意HTML或XML文档交互的API DOM作用:开发网页内容特效和实现用户交互 - DOM树
获取DOM对象
- 根据CSS选择器来获取DOM元素
选择匹配单个元素(可以直接修改) 语法  参数: 包含一个或多个有效的css选择器字符串 返回值: css选择器匹配的第一个元素,一个HTMLElement对象 如果没有匹配到,则返回null
选择匹配多个元素(不可以直接修改) 语法:  参数: 包含一个或多个有效的CSS选择器 字符串 返回值: CSS选择器匹配的NodeList对象集合 注意 得到的是一个 伪数组 有长度有索引号的数组 但没有pop() push()等数组方法 想要得到每一个数组,需要进行循环遍历
- 其他获取DOM元素方法
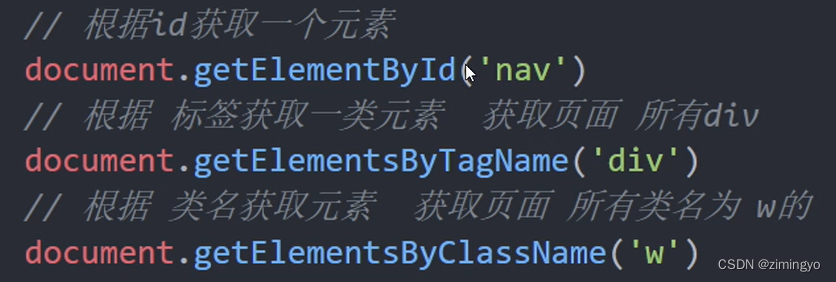
设置/修改DOM元素内容
- document.write() 方法
只能将文本内容追加到前面的位置 文本中包含的标签会被解析 - 对象.innerText属性
将文本内容添加/更新到任意标签位置 文本中包含的标签不会被解析 - 对象.innerHTML属性
将文本内容添加/更新到任意标签位置 文本中包含的标签会被解析
设置/修改DOM元素属性
操作类名(className)操作CSS   
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div {
width: 200px;
height: 200px;
background-color: pink;
}
.active {
width: 300px;
height: 300px;
background-color: hotpink;
margin-left: 100px;
}
</style>
</head>
<body>
<div class="one"></div>
<script>
let box = document.querySelector('div')
box.className = 'one active'
</script>
</body>
</html>
通过classLIst  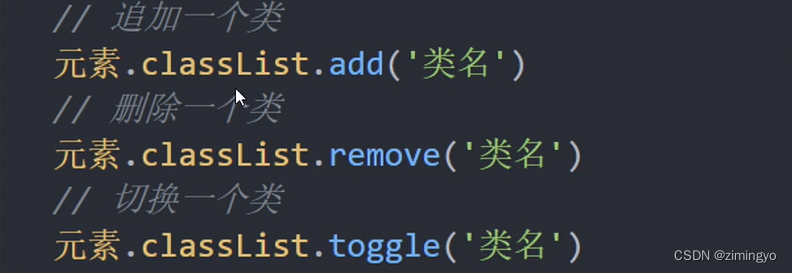 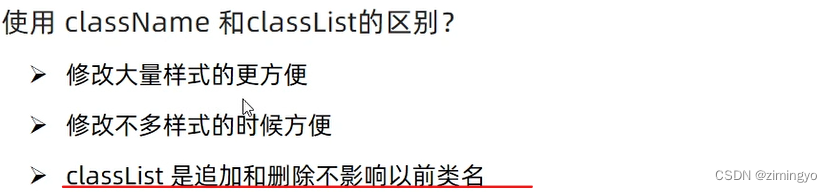
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div {
width: 200px;
height: 200px;
background-color: pink;
}
.active {
width: 300px;
height: 300px;
background-color: hotpink;
margin-left: 100px;
}
</style>
</head>
<body>
<div class="one"></div>
<script>
let box = document.querySelector('div')
box.classList.toggle('one')
</script>
</body>
</html>
- 设置/修改表单元素属性
获取:DOM对象.属性名 设置:DOM对象.属性名=新值 
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<input type="text" value="请输入">
<button disabled>按钮</button>
<input type="checkbox" name="" id="" class="agree">
<script>
let input = document.querySelector('input')
input.value = '小米手机'
input.type = 'password'
let btn = document.querySelector('button')
btn.disabled = false
let checkbox = document.querySelector('.agree')
checkbox.checked = false
</script>
</body>
</html>
定时器-间歇函数
- 开启定时器

<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
function show() {
console.log('2512')
}
let timer = setInterval(show, 1000)
clearInterval(timer)
</script>
</body>
</html>
function show() {
console.log('2512')
}
let timer = setInterval(show, 1000)
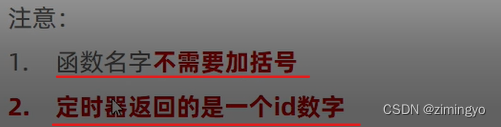
- 关闭定时器

function show() {
console.log('2512')
}
let timer = setInterval(show, 1000)
// let timer1 = setInterval(show, 1000)
// 清除定时器
clearInterval(timer)
|