Es6循环 五种方式
使用 foreach 点击显示数组目标项索引
<button @click="show1">使用 foreach 点击显示数组目标项索引</button>
var vm = new Vue({
el: '#app',
data: {
arr1: ['wlw', 'cyg', 'baby', ],
},
methods: {
show1() {
this.arr1.forEach((item, index) => {
if (item === '陈彦戈') {
console.log(index);
}
});
},
}
});
运行结果为: 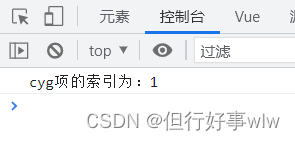
使用 some 点击显示数组目标项索引
<button @click="show2">使用 some 点击显示数组目标项索引</button>
var vm = new Vue({
el: '#app',
data: {
arr1: ['wlw', 'cyg', 'babyw', ],
},
methods: {
show2() {
this.arr1.some((item, index) => {
if (item === 'cyg') {
console.log(index);
return true
}
})
}
}
});
输出结果: 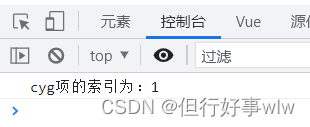 tips: some 方法相比较 foreach 能够在性能上 得到优化;
使用 every 点击显示数组目标项索引
<button @click="show3">使用 every 点击显示数组中 是否都符合 业务需求</button>
var vm = new Vue({
el: '#app',
data: {
arr2: [{
id: 1,
name: 'wlw',
age: '11',
status: true
}, {
id: 1,
name: 'cyg',
age: '11',
status: true
}, {
id: 1,
name: 'babyw',
age: '11',
status: true
}]
},
methods: {
show3() {
const status = this.arr2.every(item =>
item.status === true
)
return console.log(status);
}
}
});
输出结果: 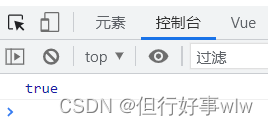 tips : every 方法 常用于判断 数组的状态是都全选,或者全不选(购物车全选按钮);
使用 filter 点击过滤出需要的项
<button @click="show4">使用 filter 点击过滤出需要的项</button>
var vm = new Vue({
el: '#app',
data: {
allCount: 0,
arr3: [{
id: 1,
name: 'banana',
status: true,
price: 10,
count: 1
}, {
id: 1,
name: 'apple',
status: true,
price: 10,
count: 2
}, {
id: 1,
name: 'orange',
status: true,
price: 10,
count: 1
}]
},
methods: {
show4() {
this.arr3.filter(item => item.status).forEach(item => {
this.allCount += item.price * item.count
})
console.log(this.allCount);
}
}
});
输出结果: 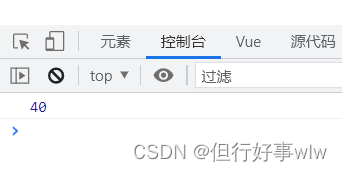
使用 reduce 累加器 来循环累加
show5() {
const result = arr.filter(item => item.status).reduce((allPrice, item) => {
return allPrice += item.price * item.count
}, 0)
console.log(result)
}
tips : 最后累加器reduce 循环累加结束以后 会把 allPrice return 出去 给 result
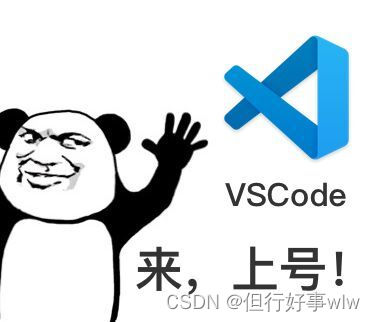
|