-
替换object对象的键名: 答: JSON.parse(JSON.stringify(data).replace(/keyName/g, ‘name’)) 注:data为数组,keyName为修改前的键名,name为修改后的键名 1、JSON.stringify()把json对象转成json字符串; 2、使用正则的replace()方法替换属性名; 3、JSON.parse()把json字符串又转成json对象。 -
时间匹配: 例: 2021-01-21 11:17:33 正则匹配成只要 2021-01-21 11:17 答: ‘2021-01-21 11:17:33’.replace(/:\d+$/, ‘’)
例: 0:0:15 替换成 0时0分15秒
方法一: '0:0:15'.replace(/(\d+):(\d+):(\d+)/, '$1时$2分$3秒')
方法二: '0:0:15'.split(':').map((x, i) => `${x}${['时', '分', '秒'][i]}`).join('')
年月同理,' 2021-06'..split('-').map((x, i) => `${x}${['年', '月'][i]}`).join(''))
- 截取想要字符:
如果数据格式不会更改可以用substring() 例: 2021-01-21 11:17:33 匹配 2021年01月
let time= '2021-01-21 11:17:33'
let newTime= `${ time.substring(0,4)} 年${time.substring(5,7)}月`
答: 2021-01-21 11:17:33.substring(0,4)年2021-01-21 11:17:33.substring(5,7)月
- 将数据相同的分类为一个object,如以月为一类,月里面有很多小数据
原数据:
arr:[
{ month: "2020年5月", date: "06日", name: "分享1:"},
{month: "2020年5月", date: "26日", name: "分享2:"},
{month: "2020年5月", date: "28日", name: "分享3:"},
{month: "2020年4月", date: "06日", name: "分享4:"},
{month: "2020年4月", date: "26日", name: "分享5:"},
{month: "2020年4月", date: "27日", name: "分享6:"}
]
const groupBy=(list, key = 'id')=> {
if (!list) return [];
const recordMap = new Map();
list.forEach(x => {
if (recordMap.has(x[key])) {
recordMap.set(x[key], [...recordMap.get(x[key]), x]);
} else {
recordMap.set(x[key], [x]);
}
});
return [...recordMap.values()];
},
this.groupBy(beforeData, 'month').map(list => {
return {
month: list[0].month,
date_arr: list,
}
})
5 数字转换成数组
let num= 13452
num.toString().split('')
function numberToArray(num) {
const arr = [];
while (num !== 0) {
const digit = num % 10;
num = Math.floor(num / 10);
arr.unshift(digit);
}
return arr;
}
console.log( numberToArray(156))
6 判断电话号码格式是否匹配的正则:
!/^1[3456789]\d{9}$/.test(phone)
function isIdCard(str) {
return /^[1-9]\d{5}(18|19|([23]\d))\d{2}((0[1-9])|(10|11|12))(([0-2][1-9])|10|20|30|31)\d{3}[0-9Xx]$/.test(str);
}
function isHttpUrl(str) {
return /^(http|https):\/\/[^\s]+$/.test(str);
}
7 时间转换成时间戳
let date = new Date('2021-01-01');
let str = date.getTime() / 1000;
console.log(str,"时间戳-------")
8 时间戳转时间
let date= new Date(1609430400);
console.log(date);
console.log(date.toLocaleDateString().replace(/\//g, "-") + " " + date.toTimeString().substr(0, 8));
getdate(time, type) {
let now = new Date(time),
y = now.getFullYear(),
m = now.getMonth() + 1,
d = now.getDate();
if (type === 'birthday') {
return `${y}-${(m < 10 ? "0" + m : m)}-${(d < 10 ? "0" + d : d)}`
} else {
return `${y}-${(m < 10 ? "0" + m : m)}-${(d < 10 ? "0" + d : d)} ${ now.toTimeString().substr(0, 5)}`
}
},
9 电话号码加密,中间四位加星号
"19919203800".replace(/(\d{3})\d{4}(\d{4})/, '$1****$2');
10 获取字符串中的参数值 在做项目时,可能会遇见值为"storeid%3D1%26tablesid%3D1%26type%3D1"这种,url通过UTF-8编码的转义后的值,首先url要解析成正常字符串,然后再获取参数值
let str="storeid%3D1%26tablesid%3D1%26type%3D1"
let newStr=decodeURIComponent(str)
console.log(newStr)
let obj=newStr.reduce( (res, keyvalue) => {
const [key, value] = keyvalue.split('=')
return {
...res,
[key]: value
}
}, {} )
console.log(obj)
URLSearchParams 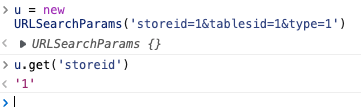 11 二维数组,根据数据内部的某条数据来进行分类,外层数据不重复
goods=[
{
title:"短发",
goods:[{sex:0,name:'短发一'},{sex:1,name:'短发二'},{sex:1,name:'短发三'}]
},
{title:"中长发"},
{
title:"长发",
goods:[{sex:0,name:长发一'},{sex:1,name:'长发二'},{sex:1,name:'长发三'}]
},
]
manList=[
{title:'短发',goods:[{sex:0,name:'短发一'},]},
{title:'长发',goods:[{sex:0,name:长发一'},]},
]
womanList:=[
{title:'短发',goods:[{sex:1,name:'短发二'},{sex:1,name:'短发三'}]},
{title:'长发',goods:[{sex:1,name:'长发二'},{sex:1,name:'长发三'}]},
]
const isEmptyArray = x => !(Array.isArray(x) && x.length > 0)
const deepClone = x => JSON.parse(JSON.stringify(x));
const getSexGoods = (classify) => {
const m = [];
const wm = [];
classify.goods.forEach(good => (String(good.sex) === '0' ? m : wm).push(good));
return [{
...deepClone(classify),
goods: m,
}, {
...deepClone(classify),
goods: wm,
}];
};
const [manList, womanList] = data.goods.reduce(([m, wm], classify) => {
if (!classify.goods) return [m, wm];
const [mItem, wmItem] = getSexGoods(classify)
return [
m.concat(isEmptyArray(mItem.goods) ? [] : mItem),
wm.concat(isEmptyArray(wmItem.goods) ? [] : wmItem),
]
}, [[], []]);
console.log({manList, womanList})
12 js获取页面url,获取想要的参数值
const url = window.location.href;
例:url='https://www.service_muban/edit?id=21&ref=addtabs'
getQueryData(arg) {
let query = window.location.search.substring(1);
let vars = query.split("&");
for (let i = 0; i < vars.length; i++) {
let arr= vars[i].split("=");
if (arr[0] === arg) {
return arr[1];
}
}
return false;
},
let id= this.getQueryData("id");
13 字符串拼接,前面加特殊符号,第一位不添加
str = str=== ' ' ? str + item.name : str + ' , ' + item.name
14 获取对象下某个键的键值
const objs={'name':'张三',''age': 24}
console.log(objs[Object.keys(objs)[0]])
15 字符串转obj 当后端返回数据有时成了字符串,我们可以这样转换
方法1:
res={errMsg: 'uploadFile:ok', statusCode: 200, data: '{"code":1,"msg":"上传成功","time":"1649390547","data":…20220408\\/2bc2e0ac4f89cea7f0c88dc40642e253.jpg"}}'}
eval('('+res.data+')')
方法二:
JSON.parse(res.data)
|