pc 选项卡组件 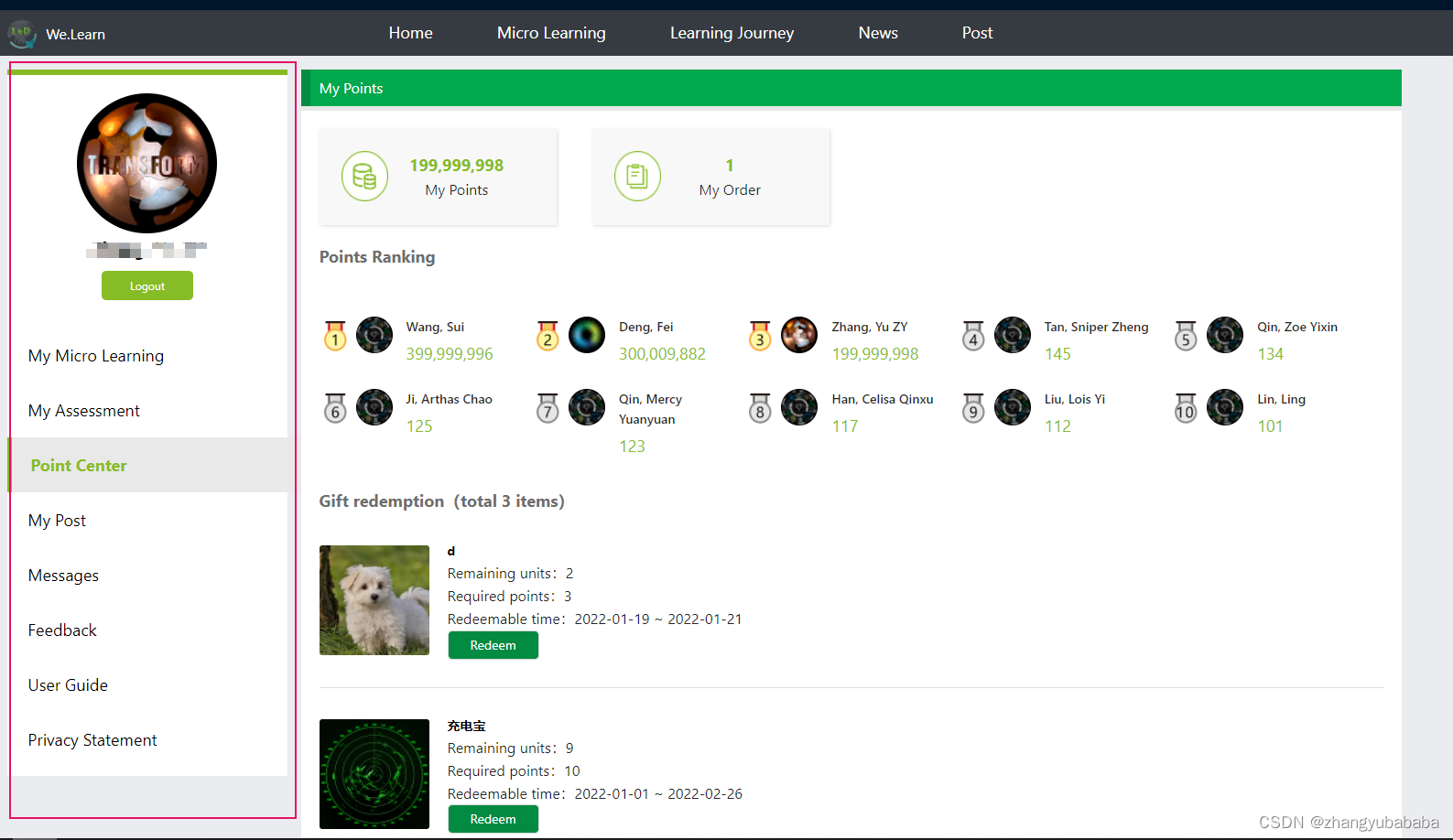
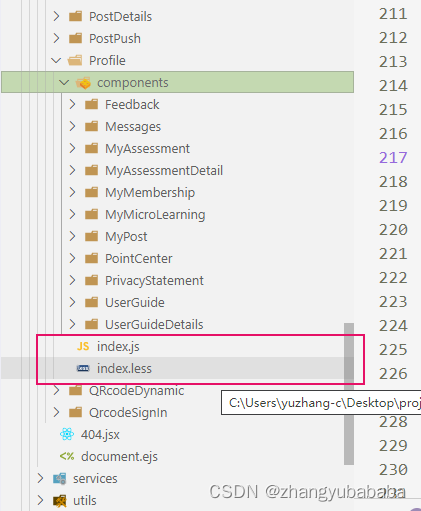
import React, { useState, useEffect, ReactElement } from 'react';
import { baseUrl, MessageSuccess, MessageError } from '@/utils/utils';
import Header from '@/components/UserHeader';
import { connect, history, useIntl } from 'umi';
import { Row, Col, Tooltip, message, Modal } from 'antd';
import { authContext } from '@/utils/msalConfig';
import { debounce } from 'lodash';
import {
GetUserPersonAvatarDetail,
GetUserPersonAvatarList,
UserEditPersonAvatar,
} from '@/services/personalCenter';
import defaultImg from '@/assets/default.png';
import selectedIcon from '@/assets/profile/selectedIcon.jpg';
import styles from './index.less';
export default (props) => {
const intl = useIntl();
const { children, user, dispatch } = props;
const [visible, setVisible] = useState(false);
const [isPc, setPc] = useState(true);
const [optionKey, setOptionKey] = useState('myMicroLearning');
const lists = [
{ name: intl.formatMessage({ id: 'homePage.MyMicroLearning' }), id: 0, key: 'myMicroLearning' },
{ name: intl.formatMessage({ id: 'homePage.MyAssessment' }), id: 1, key: 'myAssessment' },
{ name: intl.formatMessage({ id: 'homePage.IntegralCenter' }), id: 2, key: 'pointCenter' },
{ name: intl.formatMessage({ id: 'homePage.MyInteraction' }), id: 3, key: 'myPost' },
{ name: intl.formatMessage({ id: 'homePage.MyMessage' }), id: 5, key: 'messages' },
{ name: intl.formatMessage({ id: 'homePage.Feedback' }), id: 6, key: 'feedback' },
{ name: intl.formatMessage({ id: 'homePage.UserGuide' }), id: 7, key: 'userGuide' },
{ name: intl.formatMessage({ id: 'homePage.PrivacyStatement' }), id: 8, key: 'PrivacyStatement' },
];
const [userAwater, setUserAwater] = useState(defaultImg);
const [avaterList, setAvaterList] = useState([]);
const [selectAvatarId, setSelectAvatarId] = useState('');
const onTabsChange = (obj) => {
history.replace(`/userInterface/profile/${obj.key}`);
setOptionKey(obj.key);
};
const onLogOut = () => {
authContext.logOut();
localStorage.removeItem('at');
localStorage.removeItem('sk');
localStorage.removeItem('userName');
localStorage.removeItem('userEmail');
localStorage.removeItem('auth');
localStorage.removeItem('antd-pro-authority');
localStorage.setItem('loadingStatus', '');
};
const getUserPersonAvatarDetail = () => {
GetUserPersonAvatarDetail({}).then((res) => {
if (res && res.ReturnCode == 1001) {
setSelectAvatarId(res.DocumentId);
if (res.ImageUrl) {
setUserAwater(`${ATTACHMENT_URL}${res.ImageUrl}`);
}
}
});
};
const userEditPersonAvatar = debounce(() => {
if (!selectAvatarId) {
message.error(intl.formatMessage({ id: 'userCenter.changeAvatarTips' }));
return;
}
UserEditPersonAvatar({
DocumentId: selectAvatarId,
}).then((res) => {
if (res && res.ReturnCode == 1001) {
getUserPersonAvatarDetail();
MessageSuccess(res);
setVisible(false);
location.reload();
} else {
MessageError(res);
}
});
}, 300);
const getUserPersonAvatarList = () => {
GetUserPersonAvatarList({
Page: 1,
ItemsPerPage: 9999,
}).then((res) => {
if (res && res.ReturnCode === 1001) {
setAvaterList(res.Data || []);
}
});
};
useEffect(() => {
getUserPersonAvatarDetail();
getUserPersonAvatarList();
window.addEventListener('resize', () => {
if (document.body.clientWidth < 1200) {
setPc(false);
} else {
setPc(true);
}
});
}, []);
const showAvatarModal = () => {
setVisible(true);
};
useEffect(() => {
const { pathname } = location;
if (pathname.includes('myMicroLearning')) {
setOptionKey('myMicroLearning');
} else if (pathname.includes('myAssessment')) {
setOptionKey('myAssessment');
} else if (pathname.includes('pointCenter')) {
setOptionKey('pointCenter');
} else if (pathname.includes('myPost')) {
setOptionKey('myPost');
} else if (pathname.includes('myMembership')) {
setOptionKey('myMembership');
} else if (pathname.includes('messages')) {
setOptionKey('messages');
} else if (pathname.includes('feedback')) {
setOptionKey('feedback');
} else if (pathname.includes('userGuide')) {
setOptionKey('userGuide');
}
}, [location.pathname]);
const renderPcLeftMenu = () => {
return (
<div className={styles.container}>
<Row gutter={15}>
<Col span={5}>
<Row gutter={[0, 15]}>
<Col span={24}>
<div className={styles.userInfo}>
<div>
<img className={styles.userAwater} src={userAwater} onClick={showAvatarModal} />
</div>
<div className={styles.userName}>{localStorage.getItem('userName')}</div>
<div className={styles.userInfoButton} onClick={onLogOut}>
{intl.formatMessage({ id: 'userCenter.logout' })}
</div>
</div>
</Col>
</Row>
<Row>
<Col span={24}>
<div className={styles.userMenuList}>
{lists.map((item) => {
return (
<div
className={`${styles.menuItem} ${
item.key === optionKey ? styles.active : ''
}`}
key={item.id}
onClick={() => {
onTabsChange(item);
}}
>
{item.name}
</div>
);
})}
</div>
</Col>
</Row>
</Col>
<Col span={19}>{children}</Col>
</Row>
</div>
);
};
return (
<div>
<Header isActive={false} tabOr={true} />
{renderPcLeftMenu()}
<Modal
title={intl.formatMessage({ id: 'userCenter.changeAvatar' })}
visible={visible}
footer={null}
width="632px"
onCancel={() => {
setVisible(!visible);
setSelectAvatarId('');
}}
>
<Row className={styles.awaterList}>
{avaterList.map((item) => {
return (
<Col
span={8}
onClick={() => {
setSelectAvatarId(item.AvatarId);
}}
className={styles.awaterItem}
key={item.AvatarId}
>
<div className={styles.awaterImgBox}>
<img
className={styles.awaterImg}
src={`${ATTACHMENT_URL}${item.AvatarUrl}`}
alt=""
/>
{item.AvatarId == selectAvatarId ? (
<img className={styles.selectedIcon} src={selectedIcon} alt="" />
) : null}
</div>
<Tooltip title={item.AvatarTitle}>
<p className={styles.awaterTitle}>{item.AvatarTitle}</p>
</Tooltip>
</Col>
);
})}
</Row>
<div className={styles.modifyBtn} onClick={userEditPersonAvatar}>
{intl.formatMessage({ id: 'userCenter.sureChange' })}
</div>
</Modal>
</div>
);
};
.textOverflow(@line: 2) {
overflow: hidden;
text-overflow: ellipsis;
display: -webkit-box;
-webkit-line-clamp: @line;
-webkit-box-orient: vertical;
}
@themeColor: #86BC25;
@btnColor: #038B41;
.container {
margin: 15px auto 250px;
width: 80%;
}
.userInfo {
width: 100%;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
padding: 20px;
background: #ffffff;
border-top: 6px solid hsl(81, 67%, 44%);
.userAwater {
border-radius: 50%;
width: 153px;
cursor: pointer;
}
.userName {
font-size: 20px;
font-weight: bold;
color: #030303;
}
.avatar {
display: flex;
justify-content: center;
}
:global {
.ant-upload.ant-upload-select-picture-card>.ant-upload {
padding: 0
}
.ant-upload {
border-radius: 50%;
}
}
.avatarImg {
width: 100px;
height: 100px;
border-radius: 50%;
}
.userName {
width: 100%;
text-align: center;
display: inline-block;
color: #000000;
font-weight: bold;
overflow: hidden;
white-space: normal;
text-overflow: ellipsis;
}
.userInfoButton {
display: flex;
justify-content: center;
align-items: center;
width: 100px;
height: 32px;
margin-top: 10px;
color: #ffffff;
background: #86BC25;
border-radius: 5px;
cursor: pointer;
font-size: 12px;
}
}
.awaterList {
display: flex;
flex-wrap: wrap;
align-items: center;
text-align: center;
padding: 0 50px;
.awaterItem {
cursor: pointer;
.awaterImgBox {
position: relative;
.selectedIcon {
position: absolute;
width: 36px;
height: 36px;
bottom: 0;
right: 27px;
}
.awaterImg {
width: 120px;
height: 120px;
border-radius: 50%;
}
}
.awaterTitle {
font-size: 16px;
color: #000000;
line-height: 26px;
height: 52px;
.textOverflow(2)
}
}
}
.modifyBtn {
display: block;
width: 100px;
height: 32px;
line-height: 32px;
margin: 82px auto 20px;
font-size: 12px;
color: #fff;
background: @btnColor;
text-align: center;
border-radius: 5px;
cursor: pointer;
}
.userMenuList {
background: #ffffff;
padding: 10px 0;
.menuItem {
padding: 0px 0 0px 20px;
font-size: 18px;
color: #000;
border-left: 2px solid #ffffff;
cursor: pointer;
transition: all .5s;
height: 60px;
line-height: 60px;
width: 330px;
}
.active {
font-weight: bold;
color: #86bc25;
border-left: 5px solid #86bc25;
background: #E9E9E9;
transition: all .5s;
}
}
.customDrawer {
:global {
.ant-drawer-body {
padding: 0;
}
}
}
.navbar {
display: flex;
justify-content: center;
align-items: center;
position: fixed;
top: 100px;
right: 5px;
width: 50px;
height: 50px;
border-radius: 50%;
text-align: center;
font-size: 14px;
font-weight: bold;
background: #86bc25;
opacity: .6;
}
@media (max-width: 1200px) {
.container {
width: 98%;
}
}
实际上是自己手写的选项卡,点击的时候添加一个样式,过渡效果,背景色; 主要看下 布局,切换样式 active的 的传值
|