鼠标移入效果采用css实现。
删除todo,代码变更涉及的文件有:
- App.vue,即App组件
- List.vue,即List组件
- Item.vue,即Item组件
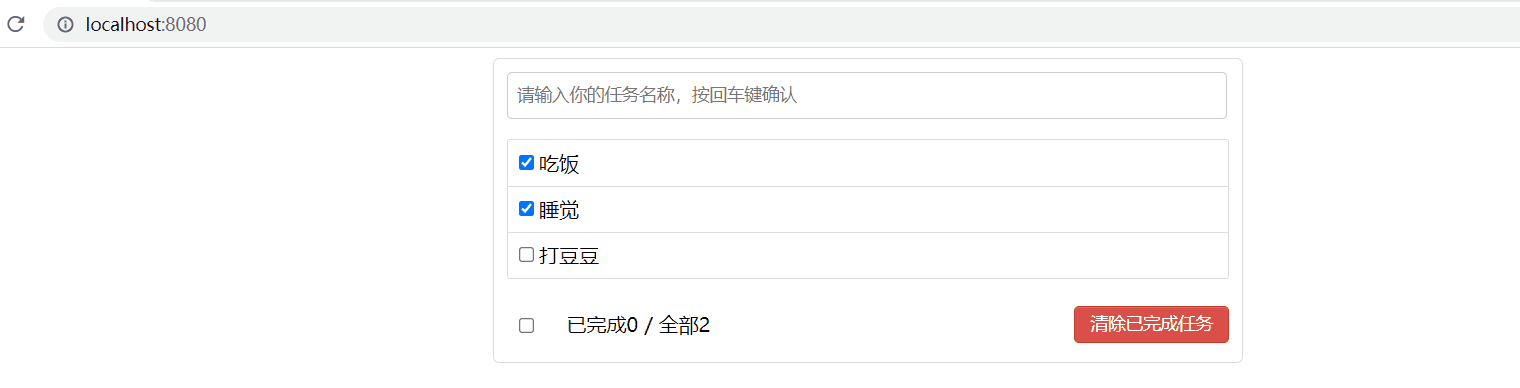
App.vue(App组件)
<template>
<div id="app">
<div class="todo-container">
<div class="todo-wrap">
<Header :addTodo="addTodo"/>
<List :todos="todos" :checkTodo="checkTodo" :deleteTodo="deleteTodo"/>
<Footer/>
</div>
</div>
</div>
</template>
<script>
import Header from './components/Header.vue';
import List from "./components/List.vue";
import Footer from "./components/Footer.vue";
export default {
name: 'App',
components: {
Header,
List,
Footer
},
data(){
return {
todos:[
{id:"001",title:"吃饭",done:true},
{id:"002",title:"睡觉",done:true},
{id:"003",title:"打豆豆",done:false}
]
}
},
methods:{
addTodo(todoObj){
this.todos.unshift(todoObj);
},
checkTodo(id){
this.todos.forEach(todo => {
if(todo.id === id) todo.done = !todo.done;
})
},
deleteTodo(id){
this.todos = this.todos.filter(todo => todo.id!==id)
}
}
}
</script>
<style>
body {
background: #fff;
}
.btn {
display: inline-block;
padding: 4px 12px;
margin-bottom: 0;
font-size: 14px;
line-height: 20px;
text-align: center;
vertical-align: middle;
cursor: pointer;
box-shadow: inset 0 1px 0 rgba(255, 255, 255, 0.2), 0 1px 2px rgba(0, 0, 0, 0.05);
border-radius: 4px;
}
.btn-danger {
color: #fff;
background-color: #da4f49;
border: 1px solid #bd362f;
}
.btn-danger:hover {
color: #fff;
background-color: #bd362f;
}
.btn:focus {
outline: none;
}
.todo-container {
width: 600px;
margin: 0 auto;
}
.todo-container .todo-wrap {
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
</style>
List.vue(List组件)
<template>
<ul class="todo-main">
<Item v-for="todoObj in todos"
:key="todoObj.id"
:todo="todoObj"
:checkTodo="checkTodo"
:deleteTodo="deleteTodo"
/>
</ul>
</template>
<script>
import Item from "./Item.vue";
export default {
name:"List",
components:{Item},
props:["todos","checkTodo","deleteTodo"]
}
</script>
<style scoped>
.todo-main {
margin-left: 0px;
border: 1px solid #ddd;
border-radius: 2px;
padding: 0px;
}
.todo-empty {
height: 40px;
line-height: 40px;
border: 1px solid #ddd;
border-radius: 2px;
padding-left: 5px;
margin-top: 10px;
}
</style>
Item.vue(Item组件)
<template>
<li>
<label>
<input type="checkbox" :checked="todo.done" @change="handleChange(todo.id)"/>
<span>{{todo.title}}</span>
</label>
<button class="btn btn-danger" @click="handleClick(todo.id)">删除</button>
</li>
</template>
<script>
export default {
name:"Item",
props:["todo","checkTodo","deleteTodo"],
methods:{
handleChange(id){
this.checkTodo(id);
},
handleClick(id){
if(confirm("确定删除吗?")){
this.deleteTodo(id);
}
}
}
}
</script>
<style scoped>
li {
list-style: none;
height: 36px;
line-height: 36px;
padding: 0 5px;
border-bottom: 1px solid #ddd;
}
li label {
float: left;
cursor: pointer;
}
li label li input {
vertical-align: middle;
margin-right: 6px;
position: relative;
top: -1px;
}
li button {
float: right;
display: none;
margin-top: 3px;
}
li:before {
content: initial;
}
li:last-child {
border-bottom: none;
}
li:hover{
background: #ddd;
}
li:hover button{
display: block;
}
</style>
相关链接
todoList案例(vue版本)之搭建静态页面 todoList案例(vue版本)之初始化列表 todoList案例(vue版本)之添加todo todoList案例(vue版本)之勾选/去勾选todo todoList案例(vue版本)之鼠标移入效果
|