说明
前面搜索了几个关于springboot+vue+elementui上传下载的文章,感觉写的都不尽如人意。要么是功能不完善,不好用。再者就是源码提供的实在差劲,都不完整。一气之下,自己搞了一个实用的完整版DEMO,有需要的朋友拿走稍加改动就能使用。
项目源码
源码已经整理好了,如何运行直接看根路径下的README.md 。
https://gitee.com/indexman/springbootdemo
效果展示
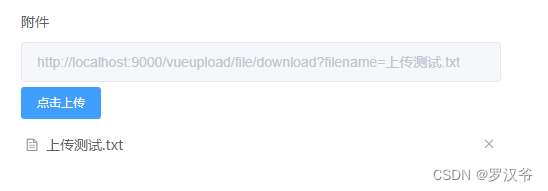 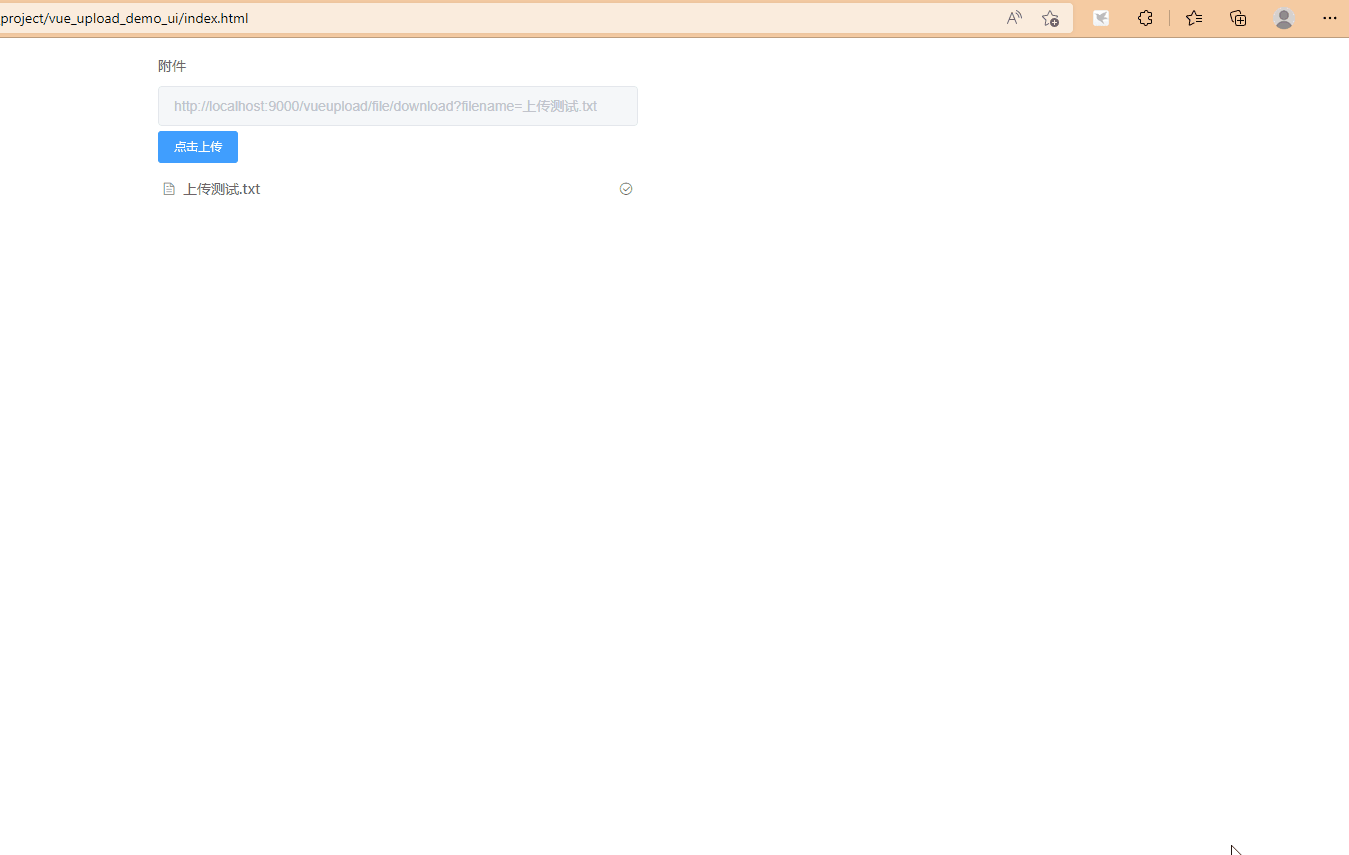
工程结构
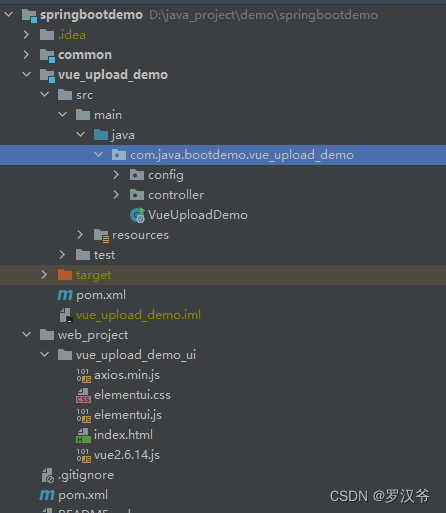
前端代码
<!DOCTYPE html>
<html lang="en" xmlns:th="http:www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<link rel="stylesheet" href="https://unpkg.com/element-ui/lib/theme-chalk/index.css">
<title>Vue文件上传下载删除DEMO</title>
<style>
#app {
z-index: 1;
left: 0;
right: 0;
top: 0;
bottom: 0;
display: flex;
justify-content: center;
align-items: center;
color: #ffffff;
}
</style>
</head>
<body>
<div id="app">
<el-form :label-width="120">
<el-form-item label="附件" prop="fileUrl">
<el-input v-model="fileUrl" :disabled="true"></el-input>
<el-upload style="width:480px;" class="upload-demo" :action="uploadUrl" :limit="1"
:show-file-list="true" :file-list="fileList" :on-remove="handleRemove" :before-remove="beforeRemove"
:on-success="uploadSuccess" :on-preview="handlePreview">
<el-button size="small" type="primary">点击上传</el-button>
</el-upload>
</el-form-item>
</el-form>
</div>
<script src="vue2.6.14.js"></script>
<script src="elementui.js"></script>
<script src="axios.min.js"></script>
<script>
var baseUrl = 'http://localhost:9000/vueupload/file/';
var app = new Vue({
el: '#app',
data: {
uploadUrl: baseUrl + 'upload',
attach: '',
fileUrl: '',
fileList: []
},
methods: {
handlePreview(file) {
let a = document.createElement('a')
a.target = '_blank';
a.href = this.fileUrl;
a.click();
},
handleExceed(files, fileList) {
this.$message.warning(`当前限制选择 5 个文件,本次选择了 ${files.length} 个文件,共选择了 ${files.length + fileList.length} 个文件`);
},
handleRemove(file, fileList) {
axios.post(baseUrl + 'remove?filename=' + this.attach).then((res) => {
console.log(res);
this.attach = '';
this.fileUrl = '';
}).catch(err => {
console.log(err);
});
console.log('删除文件:' + file.name)
},
beforeRemove(file, fileList) {
return this.$confirm(`确定移除 ${file.name}?`);
},
handleUploadError(error, file) {
console.log("文件上传出错:" + error)
},
uploadSuccess: function (response, file, fileList) {
console.log(response);
this.attach = response.data.filename;
this.fileUrl = baseUrl + 'download?filename=' + file.name
}
}
});
</script>
</body>
</html>
后端代码
package com.java.bootdemo.vue_upload_demo.controller;
import com.java.bootdemo.common.util.Result;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.*;
import java.net.URLEncoder;
import java.text.SimpleDateFormat;
import java.util.HashMap;
import java.util.Map;
@RestController
@RequestMapping("/file")
public class FileController {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
@Value("${app.uploadPath}")
private String uploadPath;
private Logger log = LoggerFactory.getLogger("FileController");
@PostMapping("/upload")
public Result fileUploads(HttpServletRequest request, @RequestParam("file") MultipartFile file) throws IOException {
String fileName = file.getOriginalFilename();
File dest = new File(uploadPath + fileName);
if (!dest.getParentFile().exists()) {
dest.getParentFile().mkdir();
}
try {
file.transferTo(dest);
log.info("上传成功,当前上传的文件保存在 {}",uploadPath + fileName);
Map map = new HashMap<>();
map.put("filename",fileName);
return new Result<>().ok(map);
} catch (IOException e) {
log.error(e.toString());
}
return new Result().error("上传失败");
}
@PostMapping("/remove")
public Result remove(@RequestParam String filename){
File dest = new File(uploadPath + filename);
if(dest.exists()){
dest.delete();
log.info("{}删除成功",filename);
return new Result<>().ok("删除成功");
}
return new Result().error("删除失败");
}
@GetMapping("/download")
public void download(@RequestParam String filename, HttpServletResponse response) throws UnsupportedEncodingException {
File dest = new File(uploadPath + filename);
if(dest.exists()){
response.reset();
response.setContentType("application/octet-stream");
response.setCharacterEncoding("utf-8");
response.setContentLength((int) dest.length());
response.setHeader("Content-Disposition", "attachment;filename="+ URLEncoder.encode( filename , "UTF-8"));
try(BufferedInputStream bis = new BufferedInputStream(new FileInputStream(dest));) {
byte[] buff = new byte[1024];
OutputStream os = response.getOutputStream();
int i = 0;
while ((i = bis.read(buff)) != -1) {
os.write(buff, 0, i);
os.flush();
}
} catch (IOException e) {
log.error("{}",e);
}
}
}
}
|