陈丹青:看过的东西走过的路,还有你所经历的一切,都会经历一个开眼界的过程。 但眼界开了并不是一件好事情,反而顿悟之后从此你就会知道,你在社会之中是完全没有位置可言的。我算个屁,什么事都有人做过,都有人做得那么好了。 年轻时候有过的那一股傻劲,慢慢地被磨得全没有了,开眼界仅仅只是让你活得更痛苦,我宁愿傻一点活着,也不愿意做一个开眼界的聪明人。
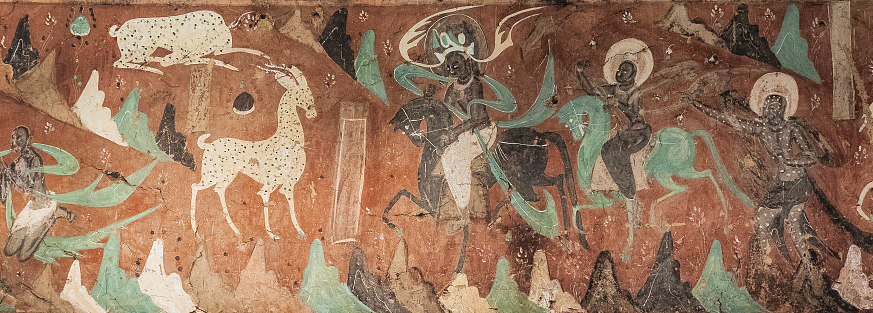
一、Vue 基础回顾
1. 基础结构
el 结构:
<template>
<div id="app">
<p>公司名称:{{ company.name }}</p>
<p>公司地址:{{ company.address }}</p>
</div>
</template>
<script>
new Vue({
el: '#app',
data: {
company: {
name: 'title',
address: '中国中关村'
}
}
})
</script>
render 结构:
<div id="app">
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
new Vue({
data: {
company: {
name: 'title',
address: '中国中关村'
}
},
render(h) {
return h('div', [
h('p', '公司名称:' + this.company.name),
h('p', '公司地址:' + this.company.address)
])
}
}).$mount('#app')
</script>
2. Vue 的声明周期
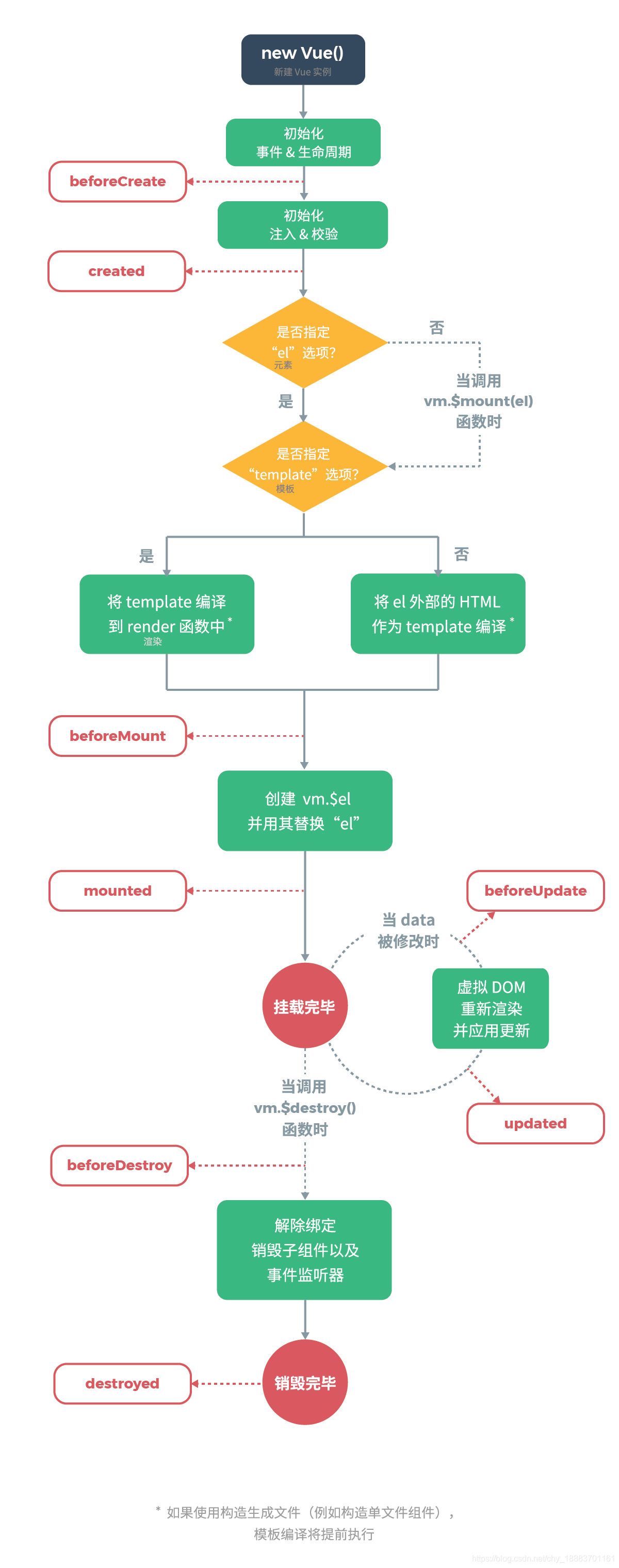
3. Vue 的语法和概念
- 插值表达式
- 指令(内置指令 14 个)
- 计算属性和侦听器
- class 和 style
- 条件渲染/列表渲染
- 表单输入绑定
- 组件
- 插槽
- 插件
- 混入 mixin
- 响应式
- 不同构建版本的 vue
二、Vue Router 基础
1. 基础使用
1. 创建路由页面 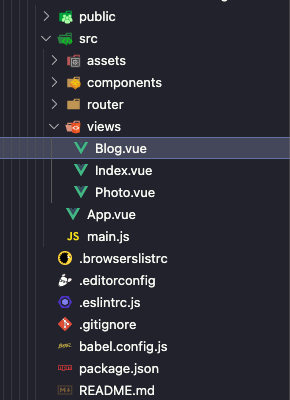
2. 注册路由插件 - 配置路由规则 - 创建 router 对象
编辑 index.js 文件(src/router/index.js):
import Vue from 'vue'
import VueRouter from 'vue-router'
import Index from '../views/Index.vue'
Vue.use(VueRouter)
const routes = [
{
path: '/',
name: 'Index',
component: Index
},
{
path: '/blog',
name: 'Blog',
component: () => import( '../views/Blog.vue')
},
{
path: '/photo',
name: 'Photo',
component: () => import( '../views/Photo.vue')
}
]
const router = new VueRouter({
routes
})
export default router
3. 注册 router 对象
编辑 main.js 文件(src/main.js):
import Vue from 'vue'
import App from './App.vue'
import router from './router'
Vue.config.productionTip = false
new Vue({
router,
render: h => h(App)
}).$mount('#app')
4. 创建路由组建的占位 - 创建链接:
<template>
<div id="app">
<div id="nav">
<router-link to="/">Index</router-link> |
<router-link to="/blog">Blog</router-link> |
<router-link to="/photo">Photo</router-link>
</div>
<router-view/>
</div>
</template>
2. 动态路由
1. 配置路由规则:
const routes = [
{
path: '/',
name: 'Index',
component: Index
},
{
path: '/detail/:id',
name: 'Detail',
props: true,
component: () => import( '../views/Detail.vue')
}
]
2. 路由页面:
<template>
<div>
通过当前路由规则获取:{{ $route.params.id }}
<br>
通过开启 props 获取:{{ id }}
</div>
</template>
<script>
export default {
name: 'Detail',
props: ['id']
}
</script>
3. 嵌套路由
1. 配置路由规则:
import Vue from 'vue'
import VueRouter from 'vue-router'
import Layout from '@/components/Layout.vue'
import Index from '@/views/Index.vue'
import Login from '@/views/Login.vue'
Vue.use(VueRouter)
const routes = [
{
name: 'login',
path: '/login',
component: Login
},
{
path: '/',
component: Layout,
children: [
{
name: 'index',
path: '',
component: Index
},
{
name: 'detail',
path: 'detail/:id',
props: true,
component: () => import('@/views/Detail.vue')
}
]
}
]
const router = new VueRouter({
routes
})
export default router
2. 嵌套路由页面:
<template>
<div>
<div>
<img width="25%" src="@/assets/logo.png">
</div>
<div>
<router-view></router-view>
</div>
<div>
Footer
</div>
</div>
</template>
<script>
export default {
name: 'layout'
}
</script>
4. 编程式导航
路由跳转的三种方式:
this.$router.replace('/login')
this.$router.push('/')
this.$router.go(-2)
三. Hash哈希 VS History
1. 区别
表现形式:
- Hash模式: http://localhost/#/detail?id=1234
- History模式: http://localhost/detail/1234
原理:
- Hash模式是基于锚点,以及
onHashChange 事件。(通过锚点的值作为路由地址,当地址变化后,触发 onHashChange 事件,根据路由呈现页面内容) - History模式是基于HTML5中的History API
History.pushState() IE10以后才支持(路径发生变化,像服务器发送请求)History.replaceState() (不会像服务器发送请求,只会改变 url 地址,并且保留历史记录)
2. History模式
⑴. 基本使用
- History需要服务器的支持
- 单页应用中,服务端不存在http://www.test.com/login这样的地址会返回找不到该页面
- 在服务端应该除了静态资源外都返回单页应用的index.html
配置路由规则:
const routes = [
{
path: '/',
name: 'Home',
component: Home
},
{
path: '/about',
name: 'About',
component: () => import( '../views/About.vue')
},
{
path: '*',
name: '404',
component: () => import( '../views/404.vue')
}
]
const router = new VueRouter({
mode: 'history',
routes
})
export default router
⑵. 刷新 404 问题
因为 History刷新会像服务端发送请求,而服务端未设置关于路由的请求相应,所以会展示默认 404 内容
const path = require('path')
const history = require('connect-history-api-fallback')
const express = require('express')
const app = express()
app.use(history())
app.use(express.static(path.join(__dirname, '../web')))
app.listen(3000, () => {
console.log('服务器开启,端口:3000')
})
- Nginx服务器配置:
-
下载nginx -
把压缩包解压到 C 盘根目录 -
启动命令行工具
start nginx
nginx -s reload
nginx -s stop
-
将打包项目拷贝到 nginx 项目 -
在浏览器中输入 localhost 地址访问 nginx 项目 -
如果没有处理history模式,刷新未配置的路径会报404的错误 -
修改 nginx.conf 文件
http {
server{
...
location / {
...
index index.html index.htm;
try_files $uri $uri/ /index.html;
?
}
}
}
执行流程: 服务器接收当前路径请求地址,如果没有当前 url 对应的资源,服务端会返回 index.html 首页; 当浏览器接收到服务端返回的是首页时,并有着对应的子路由名,将自动解析路由组件,从而显示对应的子路由组件
四. 手撕一个Vue Router
1. Vue 前置知识
- 插件
- 混入
- Vue.observable()
- 插槽
- render 函数
- 运行时和完整版的 Vue
2. Vue Router 的路由模式
Vue Router 是前端路由,在浏览器端判断当前的路径,并加载当前路径对应的组件
Hash 模式:
URL 中 # 后面的内容作为路径地址,不会请求服务器,但是会记录历史- 监听
hashchange 事件,路径改变后进行对应的组件渲染 - 根据当前路由地址找到对应组件重新渲染
History 模式:
- 通过
History.pushState() 方法改变地址栏(不会向服务器发送请求,但会将这次 URL 记录到历史中) - 监听
popstate 事件
- 监听浏览器历史的变化,可以记录改变后的历史地址
- 调用
pushState 或者 replaceState 并不会触发该事件 - 点击前进后退按钮或者调用
back 或者 forward 方法时才触发 - 根据当前路由地址找到对应组件重新渲染
3. Vue Router 的实现原理
⑴. 核心代码:
?
Vue.use(VueRouter)
?
const router = new VueRouter({
routes: [
{ name: 'home', path: '/', component: homeComponent }
]
})
new Vue({
router,
render: h => h(App)
}).$mount('#app')
⑵. 核心要点:
- 需要
Vue.use(VueRouter) 注册,所以 VueRouter 是个具有 install 方法的类 - 类中需要定义路由规则
⑶. 类图:
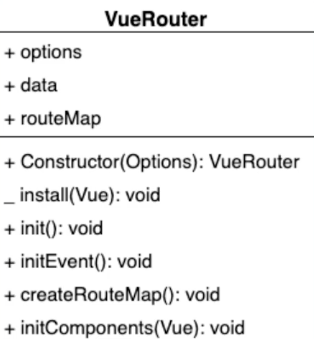
-
类名: VueRouter -
属性:
- options 对象: 记录构造函数中传入的对象
- data 对象: 有一个属性
current 记录当前路由地址;目的是让该对象是响应式的,地址发生变化后对应组件会进行更新 - routeMap 对象: 记录路由地址和组件的对应关系,将路由规则解析到routerMap中来
-
方法: -
+ 是对外公开的方法,_ 是静态方法 -
Coustructor(Options): 构造函数,初始化属性 -
install(Vue): 静态方法,实现vue的插件机制 -
init(): 调用下面三个方法,将不同代码分隔到不同方法实现
- initEvent(): 注册
popstate 事件,监听浏览器历史的变化 - creatRouteMap(): 初始化
routeMap 属性,把构造函数中传入的路由规则转换成键值对的形式存储到 RouterMap 对象。(路由地址:对应组件) - initComponents(Vue): 创建
router-link 和 router-vue 两个组件
4. Vue Router - Coustructor(构造函数)
创建 index.js 文件(vuerouter/index.js):
class VueRouter {
constructor (options) {
this.options = options
this.routeMap = {}
this.data = _Vue.observable({
current: '/'
})
}
}
5. Vue Router - install(注册插件)
let _Vue = null
class VueRouter {
constructor (options) { ... }
static install(Vue){
if(VueRouter.install.installed) return
VueRouter.install.installed = true
_Vue = Vue
_Vue.mixin({
beforeCreate(){
if(this.$options.router){
_Vue.prototype.$router = this.$options.router
}
}
})
}
}
6. Vue Router - creatRouteMap
- 功能: 遍历所有的路由规则,形成路径和组件的映射关系,存储到
routerMap 中 - routerMap: 存储的键就是路由地址,值就是地址对应的组件(将来路由变化就可以根据键值对的对应关系找到对应的组件)
createRouteMap () {
this.options.routes.forEach(route => {
this.routeMap[route.path] = route.component
})
}
7. Vue Router - initComponents
- router-link 组件:
功能: 将 router-link 标签中的内容渲染到 a 标签中去
initComponents (Vue) {
_Vue.component('router-link', {
props: {
to: String
},
template: '<a :href="to"><slot></slot></a>'
}
- router-view 组件
- 相当于一个占位符,要根据当前路由地址,获取对应的路由组件
- 调用h函数,将获取到的当前路由地址对应的组件渲染到Router-view的位置
initComponents (Vue) {
_Vue.component('router-link', {
...
})
const self = this
_Vue.component('router-view', {
render (h) {
const component = self.routeMap[self.data.current]
return h(component)
}
})
}
8. Vue Router - init
功能: 包装 createRouteMap () 、 initComponents (Vue) 和 initEvent方便调用
init () {
this.createRouteMap()
this.initComponents(_Vue)
this.initEvent()
}
并且在 install() 方法中的最后调用初始化方法
static install (Vue) {
...
if (this.$options.router) {
_Vue.prototype.$router = this.$options.router
this.$options.router.init()
}
}
9. Vue 版本
⑴. Vue 的构建版本
- 运行时版: 不支持 template 模板,需要打包的时候提前编译(render函数创建虚拟DOM渲染到视图)
- 完整版: 包含运行时和编译器,体积比运行时版大 10K 左右编译器在程序运行的时候把模板转换成 render 函数,所以性能不如运行时版本的提前编译
⑵. Vue 完整版本
vue-cli 创建的项目默认使用的是 运行时版本的 Vue - 如果想切换成带编译器版本的
Vue.js ,需要修改 vue-cli 配置 - 如果想要改配置需要在项目根目录下创建一个
vue.config.js 并导出一个模块
?
module.exports = {
runtimeCompiler: true
}
⑶. Vue 的运行时版本
采用 render 函数的形式来进行提前渲染,而不是程序执行时渲染
删除 vue.config.js ,编辑 vuerouter/index.js :
initComponents (Vue) {
_Vue.component('router-link', {
props: {
to: String
},
render (h) {
return h('a', {
attrs: {
href: this.to
},
on:{
click:this.clickhander
}
}, [this.$slots.default])
},
methods:{
clickhander(e){
history.pushState({}, "", this.to)
this.$router.data.current = this.to
e.preventDefault()
}
}
})
...
}
10. Vue Router - initEvent
- 用来实现前进后退功能
- 如果没有此方法,点击前进后退后地址改变但是组件页面不会重载,因为没有重新加载地址对应的组件
- 需要在
init 方法中进行调用
initEvent(){
window.addEventListener("popstate", () => {
this.data.current = window.location.pathname
})
}
11. Vue Router - 完整代码
console.dir(Vue)
let _Vue = null
class VueRouter {
static install(Vue){
if(VueRouter.install.installed){
return;
}
VueRouter.install.installed = true
_Vue = Vue
_Vue.mixin({
beforeCreate(){
if(this.$options.router){
_Vue.prototype.$router = this.$options.router
}
}
})
}
constructor(options){
this.options = options
this.routeMap = {}
this.data = _Vue.observable({
current:"/"
})
this.init()
}
init(){
this.createRouteMap()
this.initComponent(_Vue)
this.initEvent()
}
createRouteMap(){
this.options.routes.forEach(route => {
this.routeMap[route.path] = route.component
});
}
initComponent(Vue){
Vue.component("router-link",{
props:{
to:String
},
render(h){
return h("a",{
attrs:{
href:this.to
},
on:{
click:this.clickhander
}
},[this.$slots.default])
},
methods:{
clickhander(e){
history.pushState({},"",this.to)
this.$router.data.current=this.to
e.preventDefault()
}
}
})
const self = this
Vue.component("router-view",{
render(h){
const cm=self.routeMap[self.data.current]
return h(cm)
}
})
}
initEvent(){
window.addEventListener("popstate",()=>{
this.data.current = window.location.pathname
})
}
}
|