前言:
使用echarts遇到过的坑:
- 一定要给图表容器添加宽度与高度。
- 图表在容器中可以调整位置,让图表显示的更完整。
今日分享重点:画饼状图。 1.引入相关js
<script type="text/javascript" src="../js/jquery-2.1.1.js"></script>
<script type="text/javascript" src="../echarts-4.2.0/echarts.min.js"></script>
2.确定容器
<div id="pie" style="width: 600px; height: 325px; margin-top: 100px; margin-left: 200px;">
</div>
3.定义画饼状图的方法,配置图表参数
/**
* 画饼图,主要用来画入郑、出郑行程时间统计
* @param container 容器
* @param legendData 菜单
* @param seriesData 图表数据
*/
function drawPie(container, legendData, seriesData) {
var pieChart = echarts.init(document.getElementById(container));
pieChartOption = {
tooltip : {
trigger : 'item',
formatter : "{a} <br/>{b} : {c} ({d}%)"
},
legend : {
show : true,
type : 'scroll',
orient : 'horizontal',
left : 120,
top : 20,
bottom : 20,
data : legendData,
textStyle : {
color : 'white'
}
},
//设置饼状图每个颜色块的颜色
color : [ 'red', 'orange', 'yellow', 'green', 'blue', 'indigo', 'purple' ],
series : [ {
name : '颜色',
type : 'pie',
radius : '55%',
center : [ '53%', '50%' ],
label : {
normal : {
//饼形图显示格式
formatter : '{b|{b}} {per|{d}%} ',
rich : {
b : {
color : 'white',
fontSize : 14,
lineHeight : 33
},
//设置百分比数字颜色
per : {
color : '#00B4FB',
fontSize : 14,
padding : [ 2, 4 ],
borderRadius : 2
}
}
}
},
data : seriesData,
itemStyle : {
emphasis : {
shadowBlur : 10,
shadowOffsetX : 0,
shadowColor : 'rgba(0, 0, 0, 0.5)'
}
}
} ]
};
pieChart.setOption(pieChartOption);
var app = {};
app.currentIndex = -1;
var myTimer = setInterval(
function() {
var dataLen = pieChartOption.series[0].data.length;
if ((app.currentIndex < dataLen - 1)
&& pieChartOption.series[0].data[app.currentIndex + 1].value == 0) {
pieChart.dispatchAction({
type : 'downplay',
seriesIndex : 0,
dataIndex : app.currentIndex
});
app.currentIndex = (app.currentIndex + 1) % dataLen;
} else {
// 取消之前高亮的图形
pieChart.dispatchAction({
type : 'downplay',
seriesIndex : 0,
dataIndex : app.currentIndex
});
app.currentIndex = (app.currentIndex + 1) % dataLen;
// 高亮当前图形
pieChart.dispatchAction({
type : 'highlight',
seriesIndex : 0,
dataIndex : app.currentIndex
});
// 显示 tooltip
pieChart.dispatchAction({
type : 'showTip',
seriesIndex : 0,
dataIndex : app.currentIndex
});
}
}, 3000);
}
4.调用方法,传递参数
var legendData = ["红色", "橙色", "黄色", "绿色", "蓝色", '靛色', '紫色'];
var seriesData = [
{name: "红色", value: 14},
{name: "橙色", value: 14},
{name: "黄色", value: 14},
{name: "绿色", value: 14},
{name: "蓝色", value: 14},
{name: "靛色", value: 14},
{name: "紫色", value: 16},
];
drawPie("pie", legendData, seriesData);
5.划重点
- 设置饼状图每个颜色块的颜色可以使用color属性,这样就可以避免在具体的数据中每条数据再加样式。
- 方法中有一个定时器,用来定时跳动每个颜色块。
*其它一些小细节,有注释可以参考。
6.上图
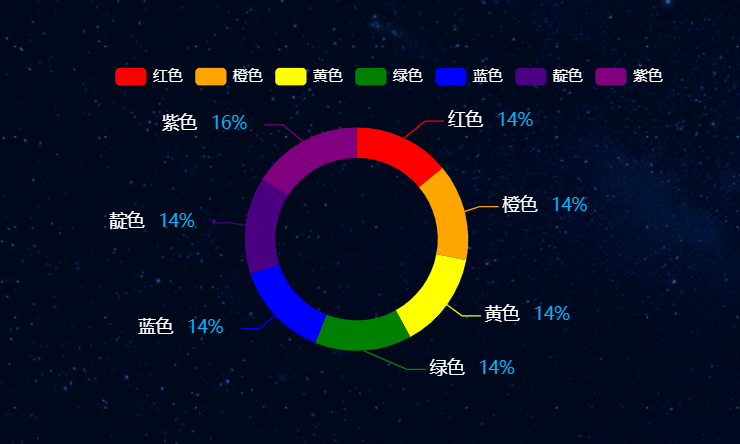
|