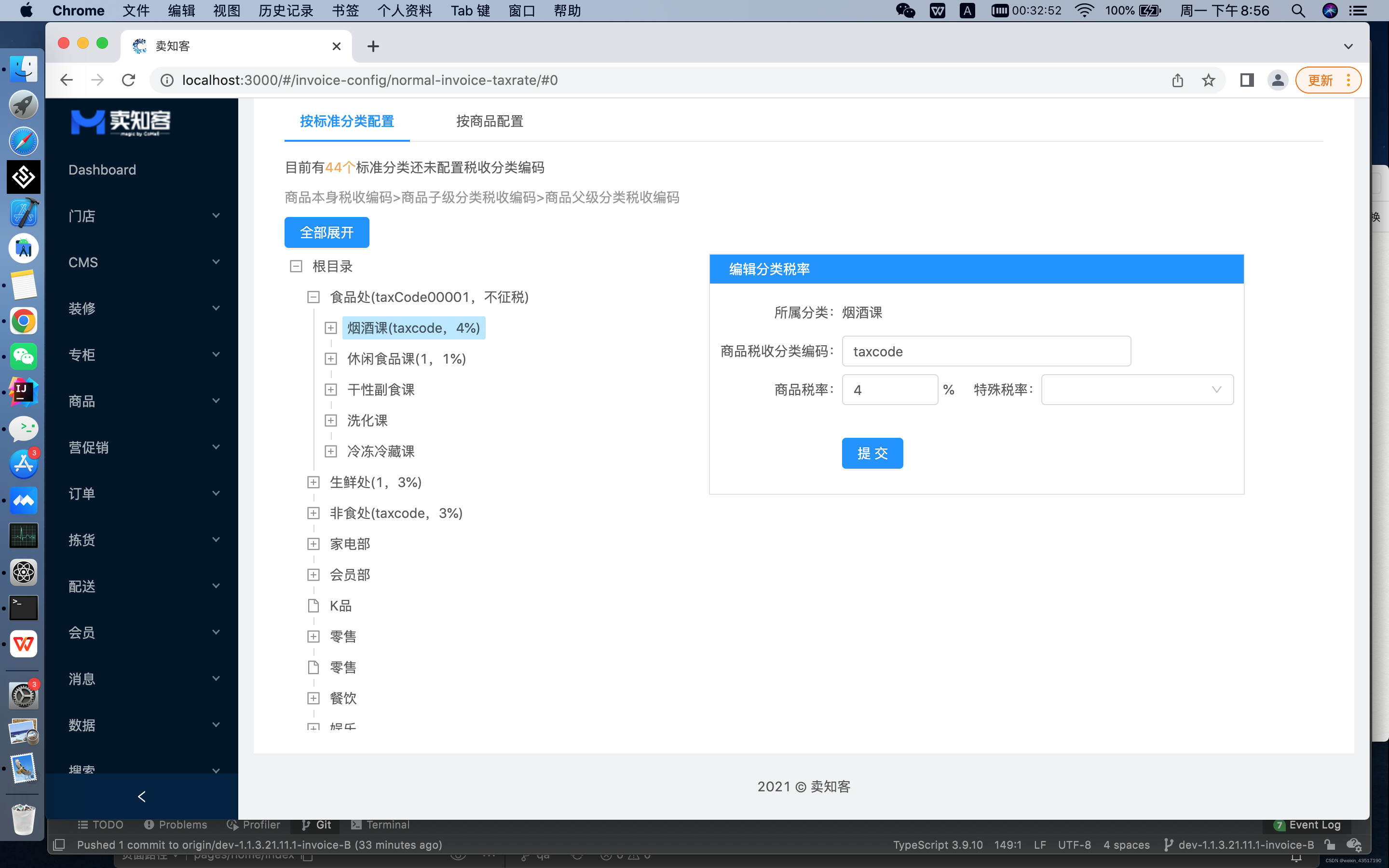
import React, { Component } from 'react';
import { services } from '@comall-backend-builder/core';
import { Tree, Button, Input, InputNumber, Select, message as AntMessage } from 'antd';
import { isEmpty, isArray } from 'lodash';
import './index.less';
const api = services.api;
enum CategoryRateType {
DUTY_FREE = 'DUTY_FREE',
NO_TAX = 'NO_TAX',
ZERO_RATE = 'ZERO_RATE',
EXPORT_TAX_REBATE = 'EXPORT_TAX_REBATE',
}
const CategoryRateTypeText: any = {
[CategoryRateType.DUTY_FREE]: 'DUTY_FREE',
[CategoryRateType.NO_TAX]: '不征税',
[CategoryRateType.ZERO_RATE]: '零税率',
[CategoryRateType.EXPORT_TAX_REBATE]: '出口退税',
};
export interface NormalInvoiceTaxrateCategoryEditFormStates {
taxRatesData: any | null;
categoriesData: Array<any>;
allKeys?: Array<string>;
expandedKeys?: Array<string>;
currentCategoriesKey: any;
currentCategories: any;
submitParams: any;
}
export class NormalInvoiceTaxrateCategoryEditForm extends Component<
{},
NormalInvoiceTaxrateCategoryEditFormStates
> {
constructor(props: any) {
super(props);
this.state = {
taxRatesData: null,
categoriesData: [],
allKeys: undefined,
expandedKeys: undefined,
currentCategoriesKey: '',
currentCategories: null,
submitParams: {
id: undefined,
taxCode: '',
taxRate: undefined,
taxRateType: '',
},
};
}
loadTaxRates = () => {
api.get(
{},
{
apiRoot: `${ENV.AUTH_API_ROOT}/MAGIC-PRODUCT`,
apiPath: `/admin/category_tax_rates/statistics`,
}
).then((response: any) => {
this.setState({
taxRatesData: response,
});
});
};
loadCategories = () => {
api.get(
{},
{
apiRoot: `${ENV.AUTH_API_ROOT}/MAGIC-PRODUCT`,
apiPath: `/admin/category_tax_rates`,
}
).then((response: any) => {
let categoriesData = [response];
const keys: string[] = [];
categoriesData.forEach((item, i) => {
this.format(item, [i], keys);
});
this.setState({
categoriesData: categoriesData,
allKeys: keys,
});
});
};
componentDidMount() {
this.loadTaxRates();
this.setState(
{
expandedKeys: ['0'],
},
() => {
this.loadCategories();
}
);
}
format = (data: any, indexs: number[], keys: string[]) => {
if (data.children && isArray(data.children) && data.children.length) {
data.children.forEach((item: any, i: number) => {
this.format(item, [...indexs, i], keys);
});
}
let title = data.name;
if (data.taxRate) {
if (data.taxRate.rate) {
title += `(${data.taxRate.code},${data.taxRate.rate}%)`;
}
if (data.taxRate.type) {
const typeText = CategoryRateTypeText[data.taxRate.type];
title += `(${data.taxRate.code},${typeText})`;
}
}
data.title = title;
if (indexs.length < 4) {
data.key = indexs.join('-');
keys.push(data.key);
}
};
onSelect = (selectedKeys: React.Key[], info: any) => {
const currentCategories = info.node.props;
this.setState({
currentCategoriesKey: selectedKeys,
currentCategories: currentCategories,
submitParams: {
id: currentCategories.id,
taxCode: currentCategories.taxRate && currentCategories.taxRate.code,
taxRate: currentCategories.taxRate && currentCategories.taxRate.rate,
taxRateType: currentCategories.taxRate && currentCategories.taxRate.type,
},
});
};
onExpand = (expandedKeys: any) => {
this.setState({
expandedKeys: expandedKeys,
});
};
selectAll = () => {
const { allKeys } = this.state;
this.setState({
expandedKeys: allKeys,
});
};
submit = () => {
const { submitParams, currentCategories } = this.state;
if (!submitParams.taxCode) {
AntMessage.warning('请输入商品税收分类编码');
return;
}
if (!submitParams.taxRate && !submitParams.taxRateType) {
AntMessage.warning('请输入商品税率或选择特殊税率');
return;
}
if (submitParams.id && currentCategories.taxRate) {
api.put(submitParams, {
apiRoot: `${ENV.AUTH_API_ROOT}/MAGIC-INVOICE`,
apiPath: '/admin/category_tax_rates',
}).then(() => {
AntMessage.success('保存成功');
});
} else {
api.post(submitParams, {
apiRoot: `${ENV.AUTH_API_ROOT}/MAGIC-INVOICE`,
apiPath: '/admin/category_tax_rates',
}).then(() => {
AntMessage.success('保存成功');
this.loadCategories();
});
}
};
onFiledChange(filed: string, e: any) {
const { submitParams } = this.state;
if (filed === 'taxCode') {
submitParams[filed] = e.target.value;
} else {
submitParams[filed] = e;
}
this.setState(
{
submitParams,
},
() => {
this.setTaxRateTypeDisabled();
}
);
}
setTaxRateTypeDisabled = () => {
const { submitParams } = this.state;
if (!isEmpty(submitParams.taxRateType)) {
submitParams.taxRate = undefined;
this.setState({
submitParams,
});
}
};
render() {
const {
taxRatesData,
categoriesData,
currentCategories,
submitParams,
expandedKeys,
} = this.state;
return (
<div className="normal-invoice-taxrate-category-edit-form">
<div>
{taxRatesData && taxRatesData.noTaxCodeCount && (
<div className="content">
<span>目前有</span>
<span className="content-value-red">
{taxRatesData.noTaxCodeCount}个
</span>
<span>标准分类还未配置税收分类编码</span>
</div>
)}
<div className="tip">
{`商品本身税收编码>商品子级分类税收编码>商品父级分类税收编码`}
</div>
<Button type="primary" onClick={this.selectAll}>
全部展开
</Button>
<div style={{ height: '500px', overflow: 'auto' }}>
<Tree
showLine
showIcon
onSelect={this.onSelect}
onExpand={this.onExpand}
expandedKeys={expandedKeys}
treeData={categoriesData}
/>
</div>
</div>
{currentCategories && currentCategories.id !== 1 && (
<div className="edit-wrap">
<div className="edit-title">编辑分类税率</div>
<div style={{ padding: '10px 10px 30px 10px' }}>
<div className="edit-input-wrap">
<div className="edit-input-title">所属分类:</div>
<span>{currentCategories.name}</span>
</div>
<div className="edit-input-wrap">
<div className="edit-input-title">商品税收分类编码:</div>
<Input
className="edit-input-no-width"
placeholder="请输入"
value={submitParams.taxCode}
onInput={this.onFiledChange.bind(this, 'taxCode')}
max={100}
/>
</div>
<div className="edit-input-wrap">
<div className="edit-input-title">商品税率:</div>
<InputNumber
className="edit-input-taxrate-width"
placeholder="请输入"
value={submitParams ? submitParams.taxRate : undefined}
disabled={submitParams.taxRateType}
min={0}
max={100}
onChange={(value: any) => {
this.onFiledChange('taxRate', value);
}}
/>
<span style={{ marginLeft: '5px' }}>%</span>
<span style={{ marginLeft: '20px' }}>特殊税率:</span>
<Select
placeholder="请选择"
style={{ width: 200 }}
value={submitParams.taxRateType}
onChange={this.onFiledChange.bind(this, 'taxRateType')}
>
<Select.Option value="">请选择</Select.Option>
<Select.Option value="DUTY_FREE">免税</Select.Option>
<Select.Option value="NO_TAX">不征税</Select.Option>
<Select.Option value="ZERO_RATE">零税率</Select.Option>
<Select.Option value="EXPORT_TAX_REBATE">
出口退税
</Select.Option>
</Select>
</div>
</div>
<Button className="edit-button" type="primary" onClick={this.submit}>
提交
</Button>
</div>
)}
</div>
);
}
}
主要用的是
<Tree
showLine
showIcon
onSelect={this.onSelect}
onExpand={this.onExpand}
expandedKeys={expandedKeys}
treeData={categoriesData}
/>
|