<template>
<div v-for="(item, i) in programmeList" :key="item" class="special-l-one">
<span
class="special-l-one-name"
:ref="
(el) => {
if (el) specialNameArray[i] = el;
}
"
>
{{ item.name }}
</span>
</div>
</template>
<script setup lang="ts" name="componentA">
import { computed, onMounted, onBeforeUpdate, ref } from 'vue';
const props = defineProps({
programmeLists: {
type: Array,
required: true,
},
qualificationLists: {
type: Array,
required: true,
},
activityLists: {
type: Array,
required: true,
},
});
const specialNameArray = ref([]);
onBeforeUpdate(() => {
specialNameArray.value = [];
});
const programmeList = computed(() => props.programmeLists);
const qualificationList = computed(() => props.qualificationLists);
const activityList = computed(() => props.activityLists);
onMounted(() => {
for (var i = 0; i < specialNameArray.value.length; i++) {
programmeList.value.clientHeight = specialNameArray.value[i].clientHeight;
}
programmeList.value.map((item, index) => {
item.clientHeight = specialNameArray.value[index].clientHeight;
});
});
</script>
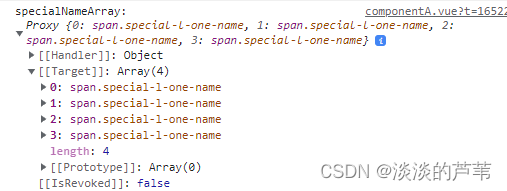 这里我是想找到该节点的clientHeight高度,然后判断一行还是两行文字,不用行数,展示的背景图不同。所以这里把高度再遍历到数组里 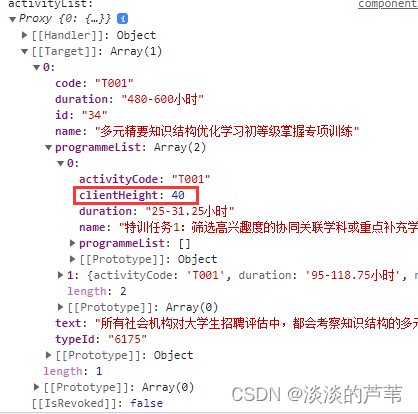
上面为一维数组 找节点的方法。
然后如果是二维数组,也是同理
<template>
<div
class="special-box"
:style="{
height: specialHValue
? activityList.length <= 1
? specialHValue + 60 + 'px'
: specialHValue + 'px'
: specialRHeight + 'px',
}"
>
<div
class="special-l"
ref="specialH"
:style="{
height: specialHValue + 'px',
width:
activityList[1].clientWidth > 443
? activityList[1].clientWidth + 20 + `px`
: `443px`,
}"
>
<div v-for="(item, i) in activityList" :key="item" class="special-l-one">
<div class="special-task-comb-inner-box-middle-top">
<div
class="special-task-comb-inner-box-middle-top-one"
:ref="
(el) => {
if (el) activityNameWidthArr[i] = el;
}
"
>
<div class="special-task-comb-inner-box-middle-top-l"></div>
<div class="special-task-comb-inner-box-middle-top-m">
<img
class="icon_l"
src="/src/assets/svg/careerMap/special_l.svg"
alt=""
/>
<span>{{ item?.name }}</span>
<img
class="icon_r"
src="/src/assets/svg/careerMap/icon_tip.svg"
alt=""
@click="showModal(item)"
/>
</div>
<div class="special-task-comb-inner-box-middle-top-r"></div>
</div>
<div
class="special-task-comb-inner-box-middle-top-two"
:ref="
(el) => {
if (el) activityNameWidthArrTwo[i] = el;
}
"
>
<div class="special-task-comb-inner-box-middle-top-two-l"></div>
<div class="special-task-comb-inner-box-middle-top-two-m">
时长:{{ item?.duration }}
</div>
<div class="special-task-comb-inner-box-middle-top-two-r"></div>
</div>
</div>
<template
v-for="(itemSpecial, idx) in item.programmeList"
:key="itemSpecial"
>
<div
:class="
itemSpecial.clientHeight1 <= 30
? `special-l-one-sm`
: `special-l-one-big`
"
:style="{
marginBottom: idx + 1 === programmeList.length ? `0` : `20px`,
}"
>
<span
class="special-l-one-name"
:ref="
(el) => {
if (el) specialNameArray1[[i, idx]] = el;
}
"
>
{{ itemSpecial.name }}{{ itemSpecial.clientHeight1 }}
</span>
<span class="special-l-one-time">
({{ itemSpecial.duration }}) {{ idx % 4 }}{{ 4 % 4 }}{{ 4 / 4
}}{{ 0 / 4 }}
</span>
<div
class="special-l-one-icon"
:style="{
top: itemSpecial.clientHeight1 <= 30 ? `-11px` : `1px`,
}"
></div>
</div>
</template>
</div>
</div>
</div>
</template>
<script setup lang="ts" name="componentA">
import { computed, onMounted, onBeforeUpdate, ref } from "vue";
const props = defineProps({
programmeLists: {
type: Array,
required: true,
},
qualificationLists: {
type: Array,
required: true,
},
activityLists: {
type: Array,
required: true,
},
});
const specialH = ref();
const specialHValue = ref();
const specialNameArray = ref([]);
const specialNameArray1 = ref([]);
onBeforeUpdate(() => {
specialNameArray.value = [];
});
const programmeList = computed(() => props.programmeLists);
const qualificationList = computed(() => props.qualificationLists);
const activityList = computed(() => props.activityLists);
const specialR = ref();
const specialRHeight = ref();
const activityNameWidthArr = ref([]);
const activityNameWidthArrTwo = ref([]);
onMounted(() => {
specialHValue.value =
activityList.value.length <= 1
? specialH.value.clientHeight - 60
: specialH.value.clientHeight - 90;
specialRHeight.value = specialR.value.clientHeight;
activityList.value.map((item, index) => {
item.clientWidth =
activityNameWidthArr.value[index].clientWidth +
activityNameWidthArrTwo.value[index].clientWidth;
item.programmeList.map((item1, idx) => {
item1.clientHeight1 =
specialNameArray1.value[[index, idx]].clientHeight;
});
});
});
</script>
|