学习目标:
提示:React生命周期
例如:
学习内容:
提示:学会React生命周期
6、生命周期
(1)什么是生命周期
生命周期LifeCycle ,描述了一个组件从创建、挂载、运行到销毁的全过程
项目开发中,每个组件在不同的运行阶段需要执行不同的操作,需要针对组件的生命周期有比较深入的理解
回顾Vue 生命周期
- 创建:
beforeCreate() | created() - 加载:
beforeMount() | mounted() - 运行:
beforeUpdate() | updated() - 销毁:
beforeDestroy | destroyed() - 缓存:
activated() | deactivated()
(2)常见生命周期
挂载阶段:
constructor() :构造static getDerivedStateFromProps() :更新,不常用UNSAFE_comopnentWillMount() :声明过时~V18.+ 不再支持render() :渲染componentDidMount() :渲染完成
更新阶段:
static getDerivedStateFromProps() :判断数据更新UNSAFE_comoponentWillRecieveProps() :处理属性数据~声明过时V18.+ 版本不再支持’’shouldComponentUpdate() :判断组件数据更新前后是否一致,用于性能提升~组件继承PureComponnet UNSAFE_componentWillUpdate() :声明过时~V18.+ 不再支持render() :渲染,重新渲染页面数据getSnapshotBeforeUpdate() :执行预处理dom 操作,使用较少componentDidUpdate() :数据更新完成
卸载阶段:
componentWillUnmount() :组件即将卸载
总结:常用的声明周期
- constructor() 构造方法,一般用于初始化状态数据
- componentDidMount() 加载方法,一般用于执行DOM操作
- render() 渲染方法,数据的刷新、获取、执行业务逻辑,渲染展示
- componentWillUnmount() 卸载方法,一般资源回收,请求取消...
-- shouldComponentUpdate() 判断方法,用于按照条件判断是否需要更新视图,特殊业务逻辑中常用
import React, {Component} from 'react';
class Life extends Component {
constructor() {
super();
console.log("constructor--构造方法,一般用于初始化状态数据")
}
componentWillUnmount() {
console.log("componentWillUnmount--卸载方法,一般资源回收,请求取消...")
}
render() {
console.log("render-- 渲染方法,数据的刷新、获取、执行业务逻辑,渲染展示")
return (
<div>
<h2>Life 生命周期组件</h2>
</div>
);
}
componentDidMount() {
console.log("componentDidMount--加载方法,一般用于执行DOM操作")
}
shouldComponentUpdate(nextProps, nextState, nextContext) {
console.log(nextProps + nextState + nextContext)
console.log("shouldComponentUpdate--判断方法,用于按照条件判断是否需要更新视图,特殊业务逻辑中常用")
}
}
export default Life;
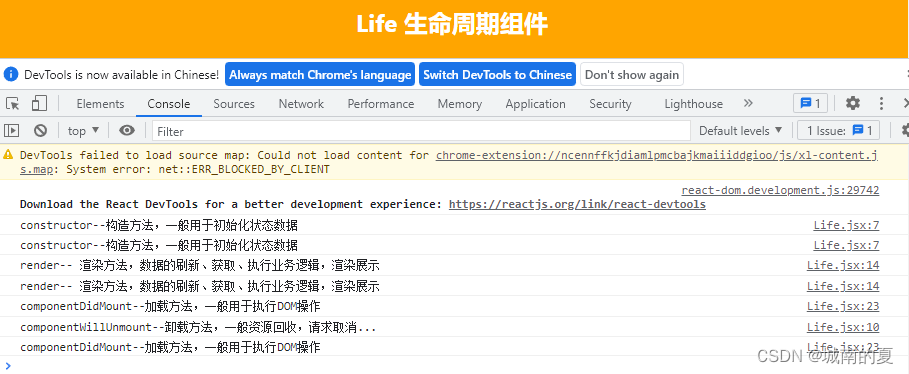
7、核心API
React 提供了两个核心API ,用于简化开发
(1) 虚拟根节点:Fragment
组件中返回的UI/JSX 片段,必须包含一个唯一的根节点,某些操作(封装功能组件)中不太友好
① 以前的组件
render() {
console.log("3.render 执行....")
return (
<div>
<h2>LifeCycle</h2>
<p>生命周期介绍</p>
</div>
)
}
② 建议的操作:React.Fragment 是一个虚拟组件节点,解释的时候置空不会影响页面结构
render() {
console.log("3.render 执行....")
return (
<React.Fragment>
<h2>LifeCycle</h2>
<p>生命周期介绍</p>
</React.Fragment>
)
}
③ 简化写法
render() {
console.log("3.render 执行....")
return (
<>
<h2>LifeCycle</h2>
<p>生命周期介绍</p>
</>
)
}
(2) 性能提升: PureComponent
操作过程中,如果某个组件中的数据需要频繁的更新,可以建议使用PureComponent 提升性能
- 注意:
PureComponent 实现了shouldComponentUpdate() 生命周期,会比普通Component 更加消耗资源
import React, { PureComponent } from 'react'
export default class LifeCycle extends PureComponent {
....
}
|