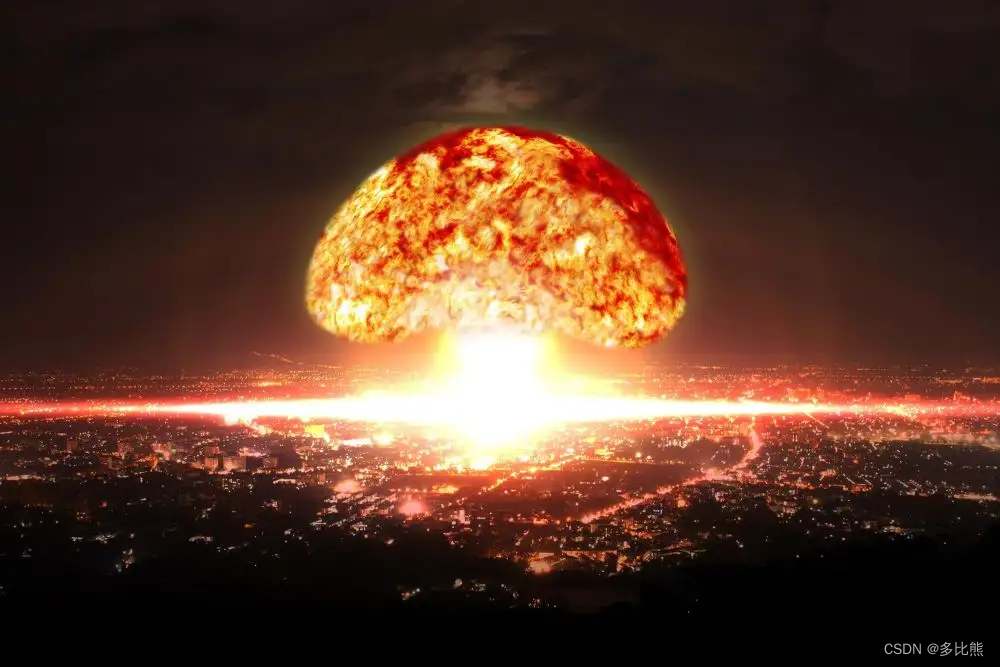
const slog = require('single-line-log').stdout;
class ProgressBar {
constructor(description, barLength) {
this.description = description || 'Progress';
this.barLength = barLength || 25;
}
render (options) {
const percent = (options.completed / options.total).toFixed(4);
const cell_num = Math.floor(percent * this.barLength);
let cell = '';
for (let i = 0; i < cell_num; i++) {
cell += '█';
}
let empty = '';
for (let j = 0; j < this.barLength - cell_num; j++) {
empty += '?';
}
const cmdText = this.description + ': ' + (100 * percent).toFixed(2) + '% ' + cell + empty;
slog(cmdText);
}
}
const awaitTime = async (time) => {
return new Promise((resolve) => {
setTimeout(() => {
resolve();
}, time)
});
};
const start = async () => {
let progressBar = null;
let num = 0;
let total = 100;
const handle = async (data, beforeMessage) => {
if (num <= total) {
data.render({ completed: num, total: total });
num++;
return new Promise((resolve) => {
setTimeout(async () => {
await handle(data, beforeMessage)
resolve();
}, 30)
});
}
await awaitTime(300);
slog(beforeMessage);
}
progressBar = new ProgressBar('检查发射环境', 50);
await handle(progressBar, '发射环境检查完毕... 开始启动发射程序...');
await awaitTime(1000);
total = 100;
num = 0;
progressBar = new ProgressBar('启动发射程序', 50);
await handle(progressBar, '启动发射程序完毕... 核弹即将发射...');
setTimeout(() => { return }, 1000)
launch();
}
start();
|