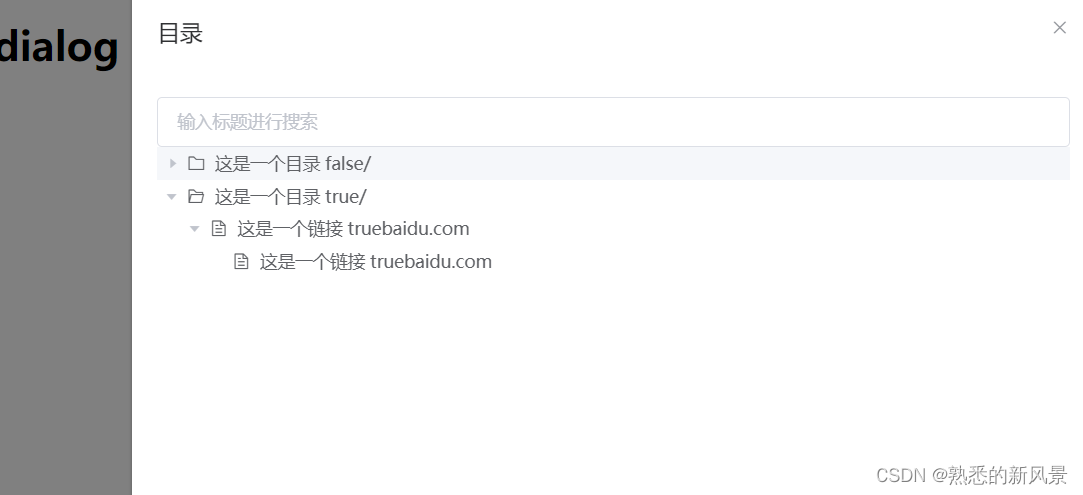 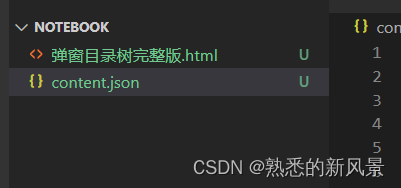
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<style>
.el-dialog__body {
overflow-y: auto;
height: 80vh;
}
.el-dialog {
margin: 0 auto !important;
min-width: 600px;
}
</style>
</head>
<body>
<h1 onclick="show()">外部dom点击开启dialog</h1>
<div id="app">
<el-button type="text" @click="dialogVisible = true">点击打开 Dialog</el-button>
<el-dialog title="目录" :visible.sync="dialogVisible">
<el-input placeholder="输入标题进行搜索" v-model="filterText"></el-input>
<el-tree :data="data" :props="defaultProps" default-expand-all :filter-node-method="filterNode" ref="tree"
@node-click="handleNodeClick">
<span slot-scope="{ node, data }">
<i :class="{
'el-icon-document': data.type == 1 || !data.type,
'el-icon-folder': data.type == 2 && !node.expanded,
'el-icon-folder-opened': data.type == 2 && node.expanded
}"></i>
<span style="padding-left: 4px;">{{node.label}}</span>
{{node.expanded}}{{data.url}}
</span>
</el-tree>
</el-dialog>
</div>
</body>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<link rel="stylesheet" href="https://unpkg.com/element-ui/lib/theme-chalk/index.css">
<script src="https://unpkg.com/element-ui@2.15.8/lib/index.js"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.js"></script>
<script>
const request = (url, params, method = "get") => {
return new Promise((resolve, reject) => {
$.ajax({
url,
method,
params,
success: function (res) {
resolve(res)
}
})
})
}
var app = new Vue({
el: '#app',
data() {
return {
dialogVisible: false,
filterText: '',
data: [],
defaultProps: {
children: 'children',
label: 'label'
}
};
},
watch: {
filterText(val) {
this.$refs.tree.filter(val);
}
},
methods: {
filterNode(value, data) {
if (!value) return true;
return data.label.indexOf(value) !== -1;
},
handleNodeClick(data, a, b) {
var {
type
} = a.data;
var {
url
} = a.data;
if (type == 1 || !type) {
this.$confirm('是否跳转到:' + url + "?").then(_ => {
this.saveDialogVisible()
window.location.href = url;
})
}
},
saveDialogVisible() {
this.dialogVisible ? this.dialogVisible = false : this.dialogVisible = true;
}
},
mounted() {
request("content.json", {}).then(res => {
this.data = res;
})
}
})
function show() {
app.saveDialogVisible()
}
</script>
</html>
[{
"label": "这是一个目录",
"url": "/",
"type": "2",
"children": [{
"label": "这是一个链接",
"url": "baidu.com",
"type": 1,
"children": [{
"label": "这是一个链接",
"url": "baidu.com",
"type": 1
}]
}]
},
{
"label": "这是一个目录",
"url": "/",
"type": "2",
"children": [{
"label": "这是一个链接",
"url": "baidu.com",
"type": 1,
"children": [{
"label": "这是一个链接",
"url": "baidu.com",
"type": 1
}]
}]
}
]
|