场景: 产品扔出一个需求说:图片随便用户选择不限制但是必须要按照一定的大小对图片进行剪裁
方案:为了快速解决这个问题,并且避免重复造轮子直接就使用了vue-cropper
效果图如下:
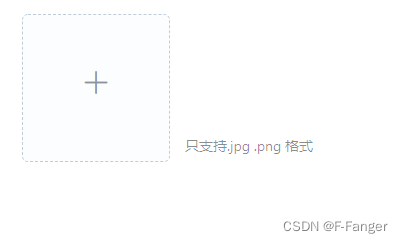 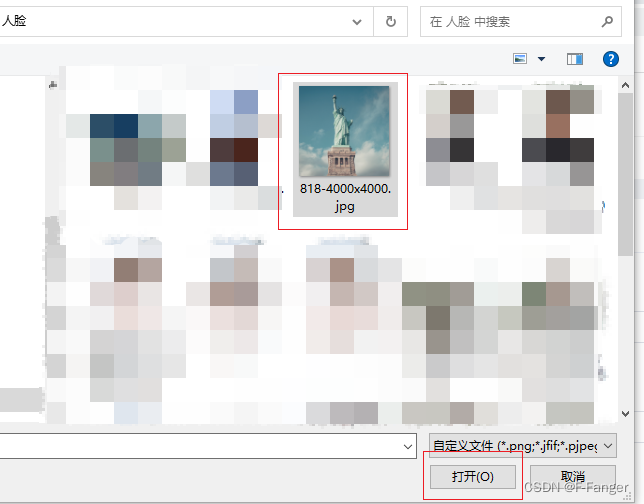
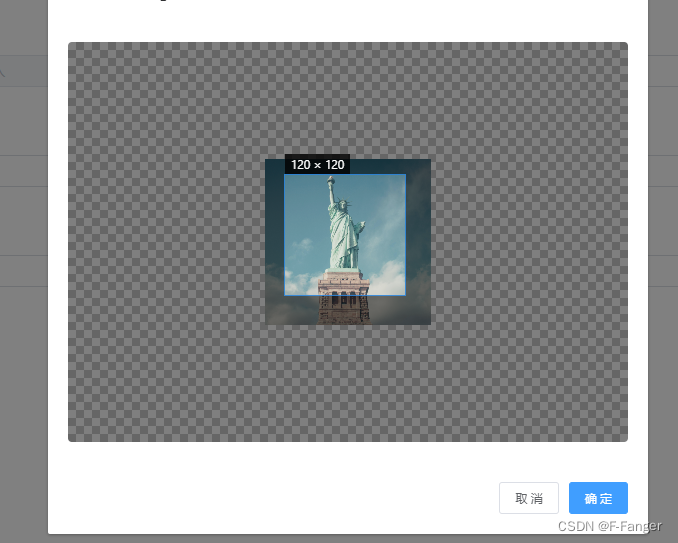 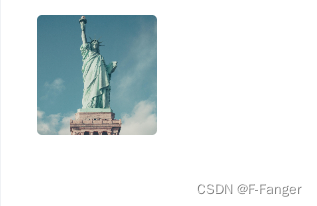
第一步:npm install vue-cropper
npm install vue-cropper
第二步: 使用(按需引入)
<template>
<el-dialog
title='裁剪Logo'
:visible.sync='visible'
:show-close='false'
:close-on-click-modal='false'
:close-on-press-escape='false'
@close='closeDialog'
width='600px'
>
<div class='avatar-container'>
<div class='avatar-crop'>
<VueCropper
class='crop-box'
ref='cropper'
:img='currOptions.img'
:autoCrop='currOptions.autoCrop'
:fixedBox='currOptions.fixedBox'
:canMoveBox='currOptions.canMoveBox'
:autoCropWidth='currOptions.autoCropWidth'
:autoCropHeight='currOptions.autoCropHeight'
:centerBox='currOptions.centerBox'
:fixed='currOptions.fixed'
:fixedNumber='currOptions.fixedNumber'
:canMove='currOptions.canMove'
:canScale='currOptions.canScale'
></VueCropper>
</div>
</div>
<template slot='footer' class='dialog-footer'>
<div>
<el-button :size='$btnSize' @click='closeDialog'>取 消</el-button>
<el-button :size='$btnSize' type='primary' @click='getCrop'>确 定</el-button>
</div>
</template>
</el-dialog>
</template>
<script>
import { VueCropper } from 'vue-cropper'
export default {
name: 'cropperDialog',
components: {
VueCropper
},
props: {
visible: {
type: Boolean,
default: false,
required: true
},
options: {
type: Object,
default: () => {
return {
img: '',
autoCrop: true,
fixedBox: true,
canMoveBox: true,
autoCropWidth: 120,
autoCropHeight: 120,
fixed: true,
fixedNumber: [1, 1],
centerBox: true,
canMove: true,
canScale: true
}
},
required: true
}
},
data() {
return {
currOptions: {}
}
},
watch: {
options: {
handler(newVal) {
this.currOptions = newVal
},
deep: true,
immediate: true
}
},
methods: {
closeDialog() {
this.$emit('update:visible', false)
},
getCrop() {
this.$refs.cropper.getCropBlob(data => {
this.$emit('imgDataResultBlob', data)
this.closeDialog()
})
}
}
}
</script>
<style scoped lang='scss'>
.dialog-footer {
display: flex;
justify-content: space-between;
align-items: center;
font-size: 14px;
.reupload {
color: #409eff;
cursor: pointer;
}
}
.avatar-container {
display: flex;
justify-content: center;
align-items: center;
width: 560px;
height: 400px;
background-color: #f0f2f5;
margin-right: 10px;
border-radius: 4px;
.upload {
text-align: center;
margin-bottom: 24px;
}
.avatar-crop {
width: 560px;
height: 400px;
position: relative;
.crop-box {
width: 100%;
height: 100%;
border-radius: 4px;
overflow: hidden;
}
}
}
</style>
在使用剪裁弹窗的页面引入弹窗组件,使用如下:
...
<cropperDialog
:visible.sync='cropperDialogVisible'
:options='options'
@imgDataResultBlob='imgDataResultBlob'
/>
...
import cropperDialog from '../../cropperDialog
export default {
components: { cropperDialog },
data() {
return {
cropperDialogVisible: false,
fileImg: '',
options: {
img: '',
autoCrop: true,
fixedBox: true,
canMoveBox: true,
autoCropWidth: 120,
autoCropHeight: 120,
fixed: true,
fixedNumber: [1, 1],
centerBox: true,
canMove: true,
canScale: true
},
}
},
methods: {
async imgDataResultBlob(data) {
const myBlob = new window.Blob([data], {type: 'image/jpeg'})
this.fileImg = window.URL.createObjectURL(myBlob)
...
},
}
}
vue-cropper 部分文档:
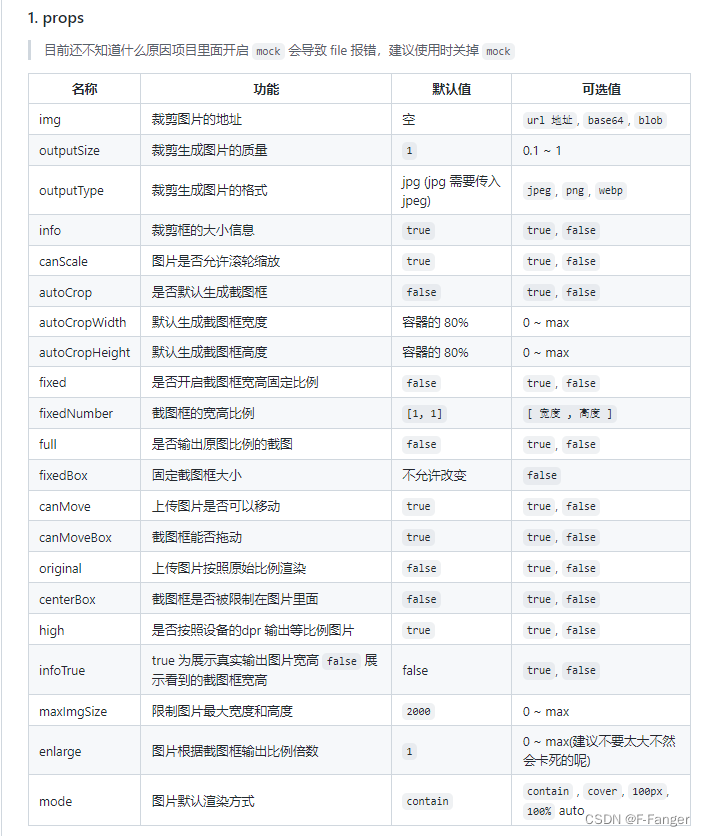 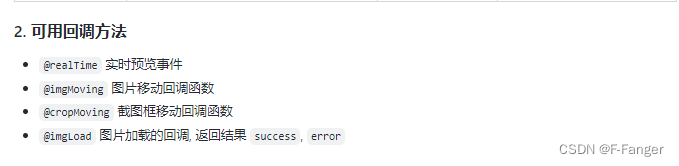 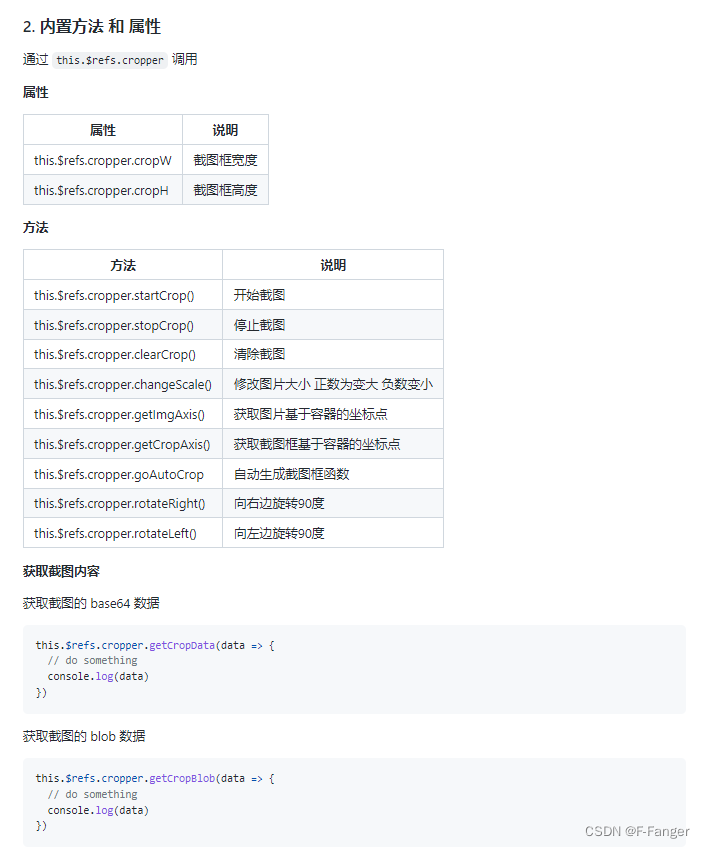
|