一、语言:vue 二、实现效果:1、左侧框选择后在右侧有显示,右侧框内点击每一条后面的图标可以删除在右侧显示的,同时左侧的多选框取消选中。2、左侧有一个本地的模糊搜索框。3、完成组件效果,要求适配多处 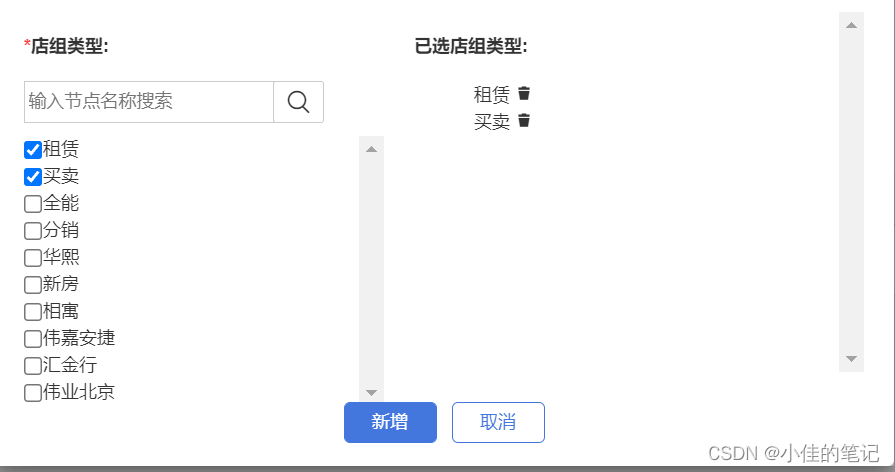 三、HTML+css 样式
<div class="transfer-box">
<div class="transfer-box-left">
<div class="box-title">
<h5><span class="box-title-icon">*</span>{{ render.leftTitle }}:</h5>
<search @search="handleSearch" />
<div class="box-left-check mt-10">
<div v-for="item in listData" :key="item.valueCode">
<input :id="item.valueCode" v-model="item.checked" type="checkbox" /><label
:for="item.valueCode"
>{{ item.valueName }}</label
>
</div>
</div>
</div>
</div>
<div class="transfer-box-right">
<div class="box-title">
<h5>{{ render.rightTitle }}:</h5>
</div>
<div>
<ul class="box-ul">
<li v-for="item in dataSource.filter((i) => i.checked)" :key="item.valueCode">
{{ item.valueName }} <span class="icon-delete" @click="delFn(item)"></span>
</li>
</ul>
</div>
</div>
</div>
<style lang="less" scoped>
.transfer-box {
display: flex;
.transfer-box-left {
flex: 1;
height: 240px;
.box-left-check {
height: 180px;
overflow-y: scroll;
font-size: 12px;
line-height: 1.5em;
}
}
.transfer-box-right {
width: 300px;
height: 240px;
overflow-y: scroll;
margin-left: 20px;
.box-ul {
list-style: none;
line-height: 1.5em;
}
}
.box-title-icon {
color: #f0463f;
}
}
</style>
四、需要传入的数据
- dataSource–选项的数据,数据格式为[{valueCode:1,valueName:1}]
- render–需要渲染的文字 ,格式为render: { leftTitle: “岗位”, rightTitle: “已选岗位” },
<script>
import { ref } from "vue";
import search from "./search.vue";
export default {
components: {
search,
},
props: {
dataSource: Object,
render: Object,
},
setup(props, { emit }) {
const listData = ref(props.dataSource);
const delFn = (item) => {
item.checked = false;
};
const handleSearch = (e) => {
if (e.value) {
listData.value = listData.value.filter((item) => {
if (item.valueName.indexOf(e.value) != -1) {
return item;
}
});
} else {
listData.value = props.dataSource;
}
};
return { listData, delFn, handleSearch };
},
};
</script>
五、搜索框组件:
<template>
<div class="search-box">
<span class="input-group">
<input v-model="searchValue" class="search-box-input" type="text" :placeholder="placeholder" />
<span v-if="searchValue" class="icon-close" @click="clearFn"></span>
</span>
<button type="button" class="icon-search search-box-icon btn" @click="handleSearch"></button>
</div>
</template>
<script>
import { ref } from "vue";
export default {
props: {
placeholder: { type: String, default: "输入节点名称搜索" },
},
emits: ["search"],
setup(props, { emit }) {
const searchValue = ref();
const handleSearch = () => {
emit("search", searchValue);
};
const clearFn = () => {
searchValue.value = "";
};
return { handleSearch, searchValue, clearFn };
},
};
</script>
<style lang="less">
.search-box {
display: flex;
align-items: center;
height: 26px;
line-height: 26px;
width: 200px;
position: relative;
.input-group {
border: 1px solid #ccc;
height: 26px;
padding: 0;
margin: 0;
width: 165px;
}
.search-box-input {
outline: none;
border: none;
}
.search-box-icon {
position: absolute;
right: 0;
text-align: center;
font-size: 16px;
height: 28px;
border-radius: 2px;
border: 1px solid #ccc;
width: 34px;
padding: 0;
}
}
</style>
六、简易粗糙版穿梭框完成,想起再完善。或者大家提提建议
|