目录
一.JQuery入门
二.JQuery选择器
三.设置样式
1.利用css()?
2.设置类样式
3.JQuery的排他思想
4.链式编程
三.jQuery操作属性
四.jQuery事件对象
五.案例
1.下拉菜单
2.淘宝服饰案例
3.TAB栏切换案例
六.其他
1.原生JS中的className和jQuery的类操作的区别?
JQuery是JavaScript库,封装了JavaScript许多函数,JQuery主要来操作DOM。
JQuery:JavaScript Query 查询JS,把JS中的DOM操作封装,可以快速查询使用里面的功能。
一.JQuery入门
$
?$是JQuery的别称,在代码中可以使用JQuery代替$。$是jQuery的顶级对象
<script>
jQuery(document).ready(function () {
jQuery('div').hide();
})
</script>
? DOM对象和JQuery对象
DOM对象:通过原生js获得的对象
var myDiv = document.querySelector('div');
//div就是DOM对象
jQuery对象:用jQuery方式获取的对象
$('div')
//$('div')就是Jquery对象
jQuery对象本质是利用$对DOM对象包装后产生的对象,用伪数组的形式存储。
注意:jQuery对象只能用jQuery方法,DOM对象只能使用js的属性和方法
例如
myDiv要是想隐藏的话只能myDiv.style.display = 'none'? 而不能用hide
$('div').style.display = 'none' 也是不允许的。
?DOM对象和JQuery对象的相互转换
原生JS的范围要比JQuery的范围大,原生JS中有一些没有被JQuery封装。
DOM对象转为JQuery对象
<script>
$(function () {
var myDiv = document.querySelector('div');
$(myDiv).hide();
})
</script>
JQuery对象转为DOM对象
<script>
$(function () {
$('div')[0].hide();
$('div').get(0).hide();
})
</script>
JQuery入口函数
<script>
$(document).ready(function () {
$('div').hide();
})
</script>
<script>
$(function () {
$('div').hide();
})
</script>
两种方式都等价于
document.addEventListener('DOMContentLoaded',function(){})
不必等到js外部文件,css文件,图片全部加载完毕再去执行
二.JQuery选择器
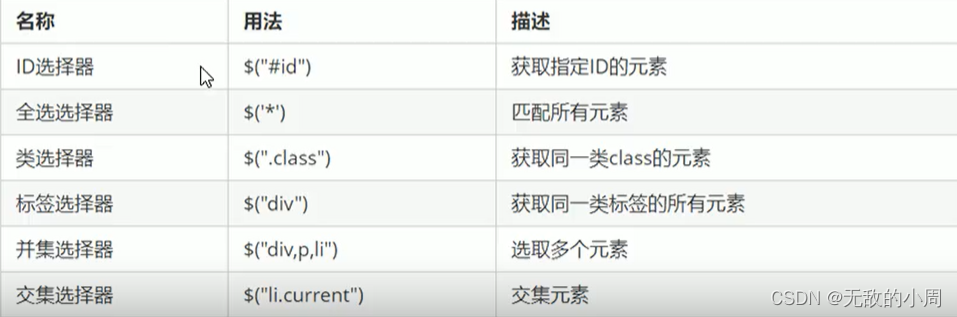

筛选选择器
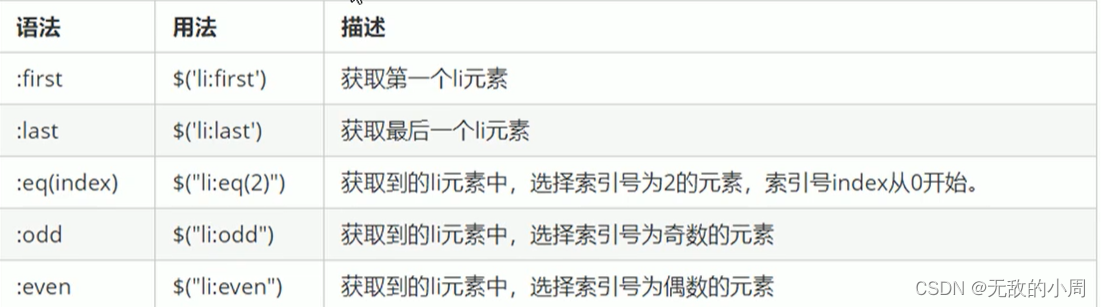
?选取父子元素
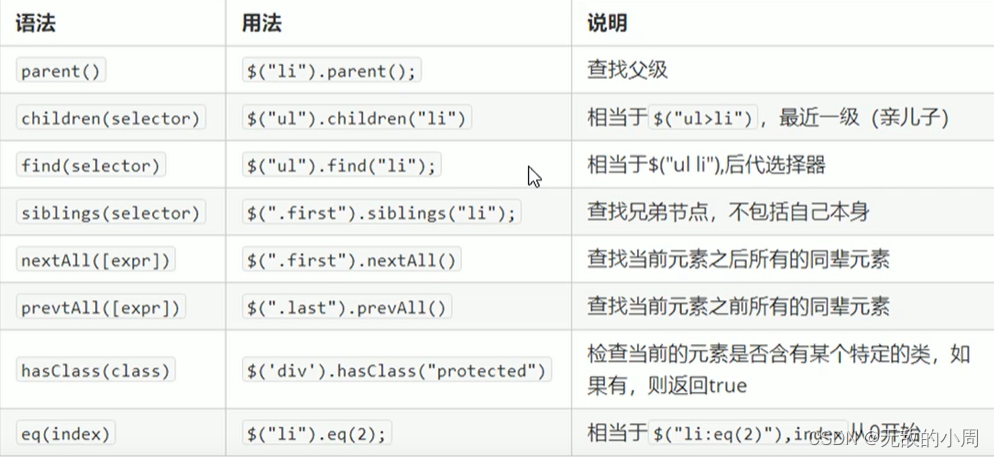
JQuery - 选择器 - 伪类选择器_梨花炖海棠的博客-CSDN博客_jquery伪类选择器
三.设置样式
?1.利用css()?
<script>
$(function () {
$('div').css('background','pink');
})
</script>
<body>
<div></div>
<div></div>
<div></div>
<div></div>
</body>
?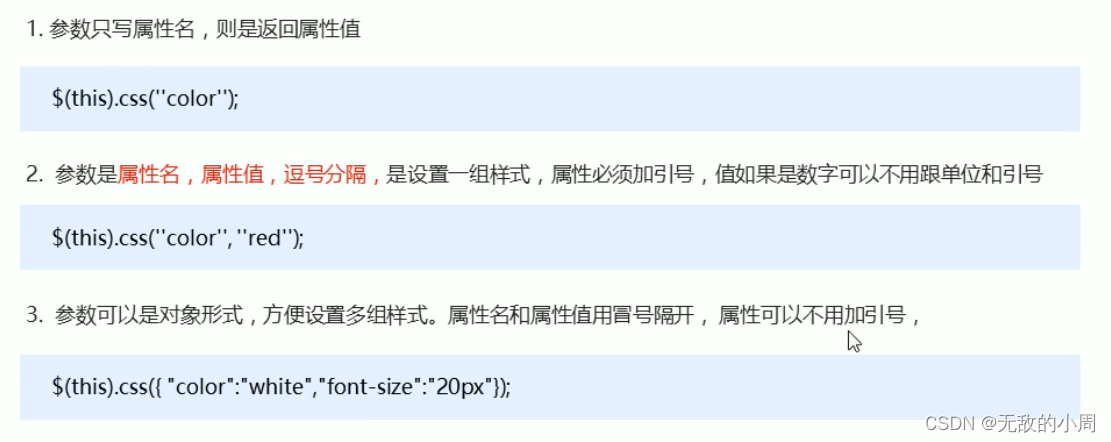
?设置多组样式时,复合属性要采取驼峰命名法,比如background-color要写成backgroundColor
隐式迭代
上面四个div都改变了样式,原生js中,只有通过for循环,给每个元素设置style,才能全部改变样式。这里用到了JQuery的隐式迭代。

?2.设置类样式
上面那种操作添加多个样式会显得繁琐。
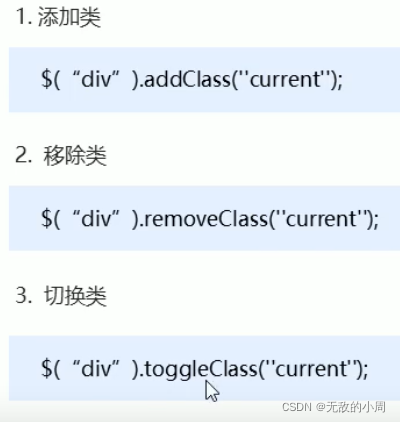
??切换类,如果这个元素有这个类名就去掉,没有这个类名即加上。
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="jquery-3.4.1.min.js"></script>
<script>
$(function () {
$('button').eq(0).click(function () {
$(this).addClass('change');
})
$('button').eq(1).click(function () {
$($('button').eq(0)).removeClass('change');
})
$('button').eq(0).click(function (){
$(this).toggleClass('toggle');
})
})
</script>
<style>
.change{
width: 100px;
height: 100px;
background-color: red;
}
.remove{
background-color: whitesmoke;
width: auto;
height: auto;
}
.toggle{
background-color: pink;
}
</style>
</head>
<body>
<button>change</button>
<button>remove</button>
</body>
</html>
3.JQuery的排他思想
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="jquery-3.4.1.min.js"></script>
<script>
$(function () {
$('button').click(function () {
$(this).css('background','pink');
$(this).siblings('button').css('background','');
})
})
</script>
<style>
</style>
</head>
<body>
<button>按钮</button>
<button>按钮</button>
<button>按钮</button>
<button>按钮</button>
<button>按钮</button>
<button>按钮</button>
</body>
</html>
4.链式编程
上面的排他思想中的代码,this出现了两次,可以使用链式编程让代码更简洁。
$(function () {
$('button').click(function () {
$(this).css('background','pink').siblings('button').css('background','');
})
})
三.jQuery操作属性?
设置或获取元素固有属性值prop()
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="js/jquery-3.4.1.min.js"></script>
<script>
$(function () {
$('a').prop('title','ccc');//更改a标签的title属性值
console.log($('a').prop('title'));//打印a标签的title属性值
$('input').change(function () {
console.log($(this).prop('checked'))
})
})
</script>
</head>
<body>
<a href="www.baidu.com" title="dddd">aa</a>
<input type="checkbox">
</body>
?设置或获取元素自定义属性值attr()
?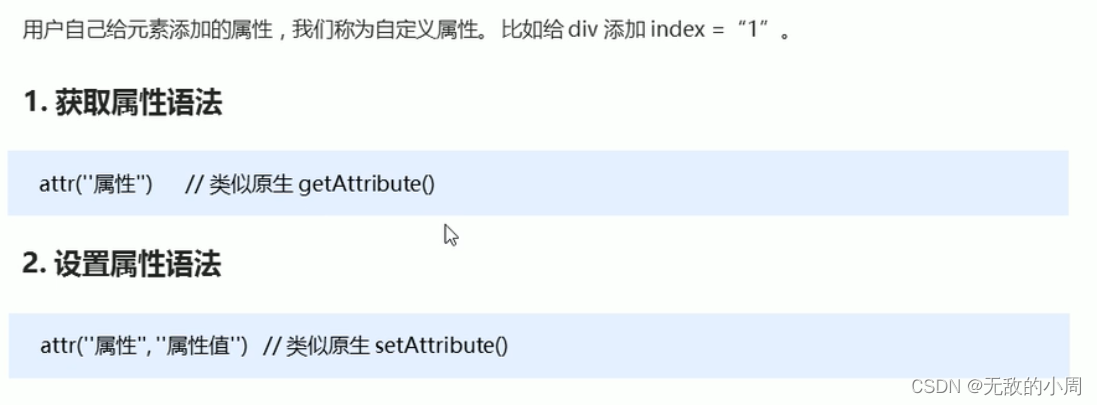
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="js/jquery-3.4.1.min.js"></script>
<script>
$(function () {
$('a').attr('index','2');
console.log($('a').attr('index'));
})
</script>
</head>
<body>
<a href="www.baidu.com" title="dddd" index="1">aa</a>
</body>
设置更改元素内容
相当于原生innerHTML
html()? ? ? 获取元素的内容
html("内容")? ? ? ? 设置元素的内容
<script>
$(function () {
console.log($('div').html());
})
</script>
<div>
<span>我是span</span>
</div>
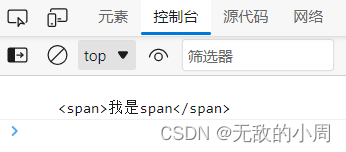
设置更改元素的文本内容
相当于原生innerText
text()? ? ? ?获取元素的文本内容
text("内容")? ? ? 设置元素的文本内容
<script>
$(function () {
console.log($('div').text());
})
</script>
<div>
<span>我是span</span>
</div>
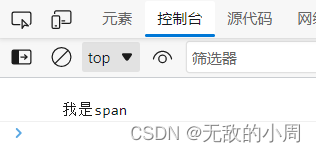
设置更改表单的值
<script>
$(function () {
$('input').val('123');
})
</script>
<input type="text">
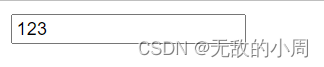
??
四.jQuery事件对象
五.jQuery方法
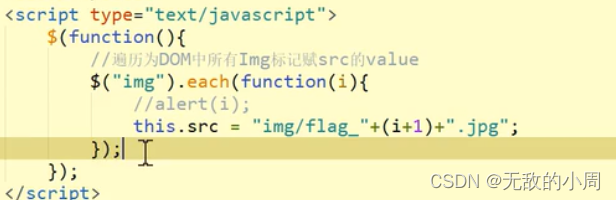
?each方法
append方法
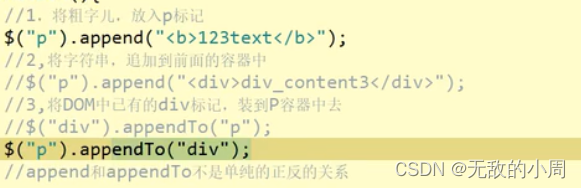
appendTo方法
empty
删除其子节点
remove
删除此节点
六.案例
1.下拉菜单
?在DOM博客中有JS原生代码写的案例
$(function () {
$('.nav>li').mouseover(function (){
$(this).children('ul').show();
})
$('.nav>li').mouseout(function () {
$(this).children('ul').hide();
})
})
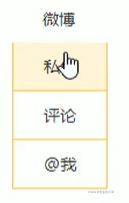
2.淘宝服饰案例
鼠标经过哪个链接,右侧就显示哪个图片
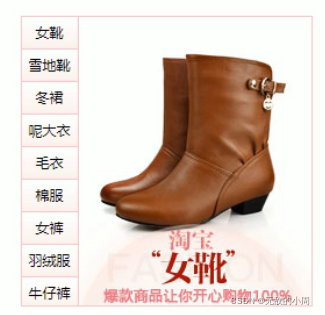
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="jquery-3.4.1.min.js"></script>
<script>
$(function () {
$('.list li').mouseover(function () {
var index = $(this).index();//获取当前li的下标
console.log($('.content div'));
$('.content div').eq(index).show();
$('.content div').eq(index).siblings().hide();
})
})
</script>
<style>
*{
margin: 0;
padding: 0;
}
ul{
list-style: none;
float: left;
}
button{
background-color: pink;
}
.content div{
float: left;
display: none;
}
</style>
</head>
<body>
<div class="list">
<ul>
<li><button>跑鞋</button></li>
<li><button>短裤</button></li>
<li><button>蜂蜜</button></li>
</ul>
</div>
<div class="content">
<div>
<img src="../images/跑鞋.png" alt="">
</div>
<div>
<img src="../images/短裤.png" alt="">
</div>
<div>
<img src="../images/蜂蜜.png" alt="">
</div>
</div>
</body>
</html>
3.TAB栏切换案例?
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="js/jquery-3.4.1.min.js"></script>
<script>
$(function () {
$('.item').hide();
$('button').click(function () {
$(this).css({'background':'black','color':'white'})
.siblings().css({'background':'white','color':'black'})
var index = $(this).index();
$('.item').eq(index).show().siblings().hide();
})
})
</script>
</head>
<body>
<button>按钮1</button>
<button>按钮2</button>
<button>按钮3</button>
<div>
<div class="item">
我是按钮1
</div>
<div class="item">
我是按钮2
</div>
<div class="item">
我是按钮3
</div>
</div>
</body>
</html>
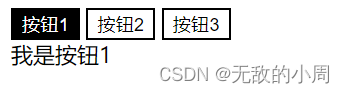
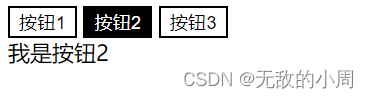
4.购物车
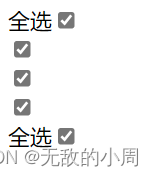
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="js/jquery-3.4.1.min.js"></script>
<script>
$(function () {
//点击全选,所有复选框被选中
$('.all').click(function () {
$('.single,.all').prop('checked',$(this).prop('checked'));
})
//当所有复选框被选中,全选框自动选中
$('.single').change(function () {
if ($('.single:checked').length === 3){
$('.all').prop('checked',true);
}else{
$('.all').prop('checked',false);
}
})
})
</script>
</head>
<body>
全选<input type="checkbox" class="all"><br>
<input type="checkbox" class="single"><br>
<input type="checkbox" class="single"><br>
<input type="checkbox" class="single"><br>
全选<input type="checkbox" class="all">
</body>
</html>
六.其他
1.原生JS中的className和jQuery的类操作的区别?

<script>
var one = document.querySelector("one");
one.className = "two";
</scirpt>
会覆盖掉之前的类
$('.one').addClass('two')
不会覆盖掉之前的类,只是增加新的类
|