页面引入redux
首先创建store.ts。此处主要用于仓库的入口,redux-thunk处理异步函数!
import { createStore, applyMiddleware } from 'redux';
import rootReducer from './reducers';
import thunk from 'redux-thunk';
export default createStore(
rootReducer,
applyMiddleware(thunk)
);
redux 中创建reducers、actions、types文件夹
reducer 主要是数据的纯函数
actions 主要是数据的处理,以及公共接口(axios)处理
目录如下
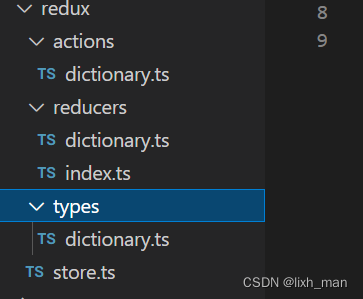
reducer
首先创建index.ts文件
import { combineReducers } from 'redux';
import dictionary from './dictionary';
const rootReducer = combineReducers({
dictionary
});
export default rootReducer;
依次拆分模块
import { SECURITY_LEVEL, METADATA_TYPE, DATA_TYPE, VALUE_TYPE } from '../types/dictionary';
const init_state = {
levelType: [], //安全级别
metaType: [], //元数据类型
dataType: [], //来源类型-类型
valueType: [] //取值范围类型
};
export default function dictionary(state = init_state, action) {
const { type, data } = action;
switch (type) {
case SECURITY_LEVEL:
return Object.assign({}, state, { levelType: data });
case METADATA_TYPE:
return Object.assign({}, state, { metaType: data });
case DATA_TYPE:
return Object.assign({}, state, { dataType: data });
case VALUE_TYPE:
return Object.assign({}, state, { valueType: data });
default:
return state;
}
}
actions 主要处理数据,及接口
import { SECURITY_LEVEL, INIT_LANG, } from '../types/dictionary';
import { getSecurityLevel } from '@/service/metadata/dictionary';
/**
* 示例
*/
export const initRoutes = (data) => {
return {
type: INIT_LANG,
data
};
};
/**
示例
* 获取安全级别
*/
export const getSecurityLevelList = () => {
return async (dispatch) => {
const res: CommonObjectType = await getSecurityLevel();
dispatch({
type: SECURITY_LEVEL,
data: res.data
});
};
};
types 主要是依赖的type标识
export const INIT_LANG = 'INIT_LANG'; //示例
export const SECURITY_LEVEL = 'SECURITY_LEVEL';
页面调用 – 示例
useSelector获取数据,useDispatch调取dispatch的方法
import React, { FC ,useEffect} from "react";
import { getSecurityLevelList, getMetadataTypeList } from '@/redux/actions/dictionary';
import { useSelector, useDispatch } from 'react-redux';
const App: FC = () => {
const dispatch = useDispatch();
const levelType = useSelector((state) => state.dictionary.levelType); // 示例
const metaType = useSelector((state) => state.dictionary.metaType); //
useEffect(() => {
initDict();
}, []);
//初始化字典表
const initDict = () => {
dispatch(getSecurityLevelList());
dispatch(getMetadataTypeList());
};
return <div>1111</div>;
};
export default App;
这是一个简易版的,符合大众化的redux使用,
像vue有vuex,以及新出的pinia 新一代的vue状态管理 都是为了方便而生
react 中也有dva 、mobx、及@reduxjs/toolkit
@reduxjs/toolkit 可以推荐使用一下
|