简介
学习了一下关于如何自定义一个日历表。 参考文章:Vue写一个日历 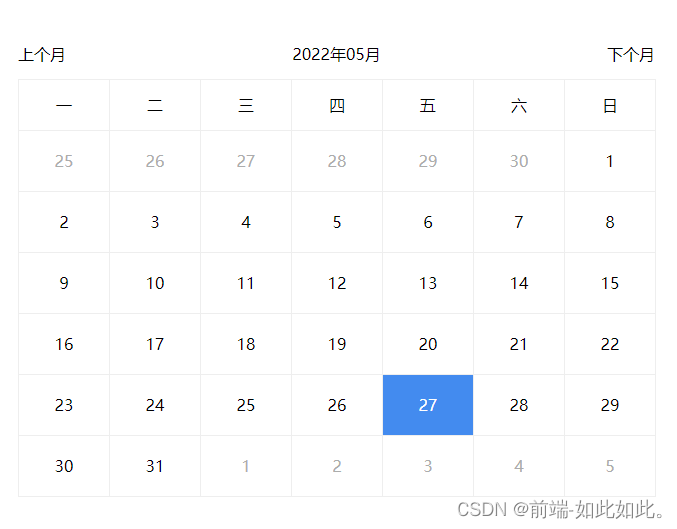
具体实现
第一步:打开windows的日历
可以看到,有如下关键点(暂时忽略农历、节气、节日的备注信息): ①年月的信息; ②上一个月、下一个月的快捷切换按钮; ③周一到周天的行; ④深颜色的当前月日期; ⑤填充空白的灰色日期(其他月的日期信息); 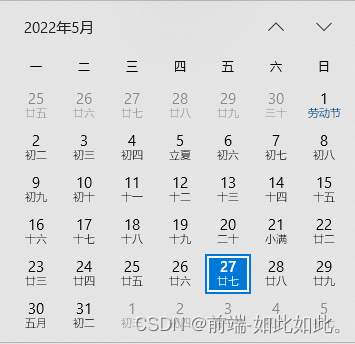
第二步:分析
①计算出显示月份的天,并显示; ②切换月份时,重新执行①; ③月初、月末的星期几的空白日,需要上一个月下一个月的对应天数补齐; ④第一次显示时,今天日期的选中; ⑤注意2月的天数差距:
a、通常能被4整除的年份是闰年,不能被4整除的年份是平年。如:1988年2008年是闰年;2005年2006年2007年是平年。
b、如果是世纪年,即整百年能被400整除是闰年,否则是平年。如:2000年就是闰年,1900年就是平年。
c、闰年的2月有29天,平年的2月只有28天。
第三步:了解需要使用到的日期API
getFulleYear();
getMonth();
getDate();
getDay();
new Date(2022,2,10);
new Date(2022,2,0);
new Date(2022,2,-1);
var d = new Date();
d.setTime(new Date(2022,1,1).getTime());
d.getFullYear();
第四步:代码实现
<template>
<div class="calendar-box">
<div class="calendar-wrapper">
<div class="calendar-toolbar">
<div class="prev" @click="prevMonth">上个月</div>
<div class="current">{{ currentDateStr }}</div>
<div class="next" @click="nextMonth">下个月</div>
</div>
<div class="calendar-week">
<div
class="week-item calendarBorder"
v-for="item of weekList"
:key="item"
>
{{ item }}
</div>
</div>
<div class="calendar-inner">
<div
class="calendar-item calendarBorder"
v-for="(item, index) of calendarList"
:key="index"
:class="{
'calendar-item': true,
calendarBorder: true,
'calendar-item-hover': !item.disable,
'calendar-item-disabled': item.disable,
'calendar-item-checked':
dayChecked && dayChecked.value == item.value,
}"
@click="handleClickDay(item)"
>
{{ item.date }}
</div>
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
showYearMonth: {},
calendarList: [],
shareDate: new Date(),
dayChecked: {},
weekList: ["一", "二", "三", "四", "五", "六", "日"],
};
},
created() {
this.initDataFun();
},
computed: {
currentDateStr() {
let { year, month } = this.showYearMonth;
return `${year}年${this.pad(month + 1)}月`;
},
},
methods: {
initDataFun() {
this.setCurrentYearMonth();
this.createCalendar();
this.getCurrentDay();
},
setCurrentYearMonth(d = new Date()) {
let year = d.getFullYear();
let month = d.getMonth();
let date = d.getDate();
this.showYearMonth = {
year,
month,
date,
};
},
getCurrentDay(d = new Date()) {
let year = d.getFullYear();
let month = d.getMonth();
let date = d.getDate();
this.dayChecked = {
year,
month,
date,
value: this.stringify(year, month, date),
disable: false
};
},
createCalendar() {
const oneDayMS = 24 * 60 * 60 * 1000;
let list = [];
let { year, month } = this.showYearMonth;
let firstDay = this.getFirstDayByMonths(year, month);
let prefixDaysLen = firstDay === 0 ? 6 : firstDay - 1;
let begin = new Date(year, month, 1).getTime() - oneDayMS * prefixDaysLen;
let lastDay = this.getLastDayByMonth(year, month);
let suffixDaysLen = lastDay === 0 ? 0 : 7 - lastDay;
let end = new Date(year, month + 1, 0).getTime() + oneDayMS * suffixDaysLen;
while (begin <= end) {
this.shareDate.setTime(begin);
let year = this.shareDate.getFullYear();
let curMonth = this.shareDate.getMonth();
let date = this.shareDate.getDate();
list.push({
year: year,
month: curMonth + 1,
date: date,
value: this.stringify(year, curMonth, date),
disable: curMonth !== month,
});
begin += oneDayMS;
}
this.calendarList = list;
},
stringify(year, month, date) {
let str = [year, this.pad(month + 1), this.pad(date)].join("-");
return str;
},
pad(str) {
return str < 10 ? `0${str}` : str;
},
prevMonth() {
this.showYearMonth.month--;
this.recalculateYearMonth();
this.createCalendar();
},
nextMonth() {
this.showYearMonth.month++;
this.recalculateYearMonth();
this.createCalendar();
},
recalculateYearMonth() {
let { year, month, date } = this.showYearMonth;
let maxDate = this.getDaysByMonth(year, month);
date = Math.min(maxDate, date);
let instance = new Date(year, month, date);
this.setCurrentYearMonth(instance);
},
getDaysByMonth(year, month) {
return new Date(year, month + 1, 0).getDate();
},
getFirstDayByMonths(year, month) {
return new Date(year, month, 1).getDay();
},
getLastDayByMonth(year, month) {
return new Date(year, month + 1, 0).getDay();
},
handleClickDay(item) {
if (!item || item.disable) return;
console.log(item);
this.dayChecked = item;
},
},
};
</script>
<style lang="less" scoped>
@calendarWidth: 637px;
.calendar-box {
width: 100vw;
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
.calendar-wrapper {
.calendar-toolbar {
width: @calendarWidth;
height: 50px;
display: flex;
justify-content: space-between;
align-items: center;
.prev,
.next,
.current {
cursor: pointer;
&:hover {
color: #438bef;
}
}
}
.calendar-week {
width: @calendarWidth;
border-left: 1px solid #eee;
display: flex;
flex-wrap: wrap;
.week-item {
width: 90px;
height: 50px;
border-top: 1px solid #eee;
}
}
.calendar-inner {
width: @calendarWidth;
border-left: 1px solid #eee;
display: flex;
flex-wrap: wrap;
.calendar-item {
width: 90px;
height: 60px;
}
.calendar-item-hover {
cursor: pointer;
&:hover {
color: #fff;
background-color: #438bef;
}
}
.calendar-item-disabled {
color: #acacac;
cursor: not-allowed;
}
.calendar-item-checked {
color: #fff;
background-color: #438bef;
}
}
.calendarBorder {
display: flex;
justify-content: center;
align-items: center;
border-bottom: 1px solid #eee;
border-right: 1px solid #eee;
}
}
}
</style>
|