一、新增的用于声明变量的关键字。
(1)let
- 声明的变量只在所处于的块级有效
- 不存在变量提升
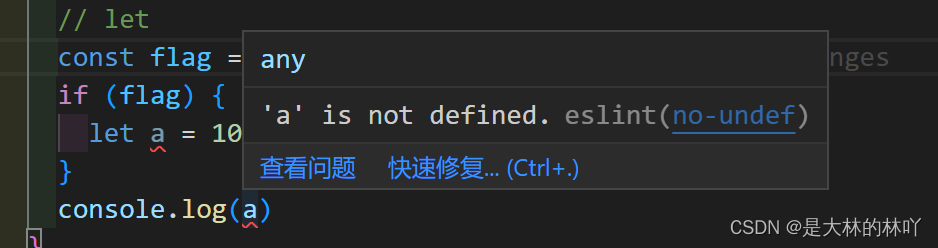
(2)const
- 声明的变量只在所处于的块级有效
- 声明常量时必须赋值
- 常量赋值后,值不能修改
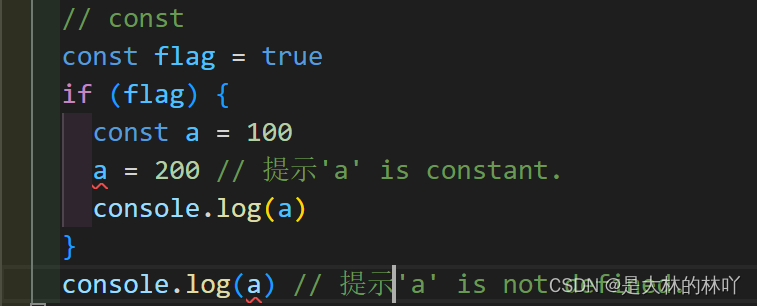
(3)var、let、const的区别
- 使用 var 声明的变量,其作用域在该语句所在的函数内,且存在变量提升现象,值可更改。
- 使用 let 声明的变量,其作用域在该语句所在的代码块内,不存在变量提升,值不可更改。
- 使用 const 声明的变量,其作用域在该语句所在的代码块内,不存在变量提升,值不可更改。
二、解构赋值
(1)数组解构
const [a, b, c] = [1, 2, 3]
console.log(a, b, c)
const [d, [[e]], [f]] = [11, [[42]], [35]]
console.log(d, e, f)
const [g = 1, h] = []
console.log(g, h)
const [i, ...j] = [323, 121, 32, 98]
console.log(i, j)
const [k = 3] = [1]
console.log(k)
const [o, p, q] = 'ljc'
console.log(o, p, q)
(2)对象解构
const { name, age } = { name: 'ljc', age: 24 }
console.log(name, age)
const { addr: val } = { addr: '深圳市' }
console.log(val)
const obj = { p: ['hello', { y: 'world' }] }
const {
p: [x, {}]
} = obj
console.log(x)
const aa = { f: [{ b: 'world' }] }
const {
f: [{ b }, a]
} = aa
console.log(b, a)
const { name1, age1, ...objs } = {
name1: 'ljc',
age1: 24,
addr: '深圳市',
love: 'xlt'
}
console.log(name1)
console.log(age1)
console.log(objs)
const { val1 = 10, val2 = 5 } = { val1: 3 }
console.log(val1, val2)
const { a: val11 = 10, b: val22 = 10 } = { a: 99 }
console.log(val11, val22)
三、箭头函数
- 如果函数只有一个语句,并且该语句返回一个值,则可以去掉括号和 return 关键字:
- 如果形参只有一个,可以省略小括号
const hello1 = () => {
return 'Hello World!'
}
console.log(hello1())
const hello2 = () => 'Hello World!'
console.log(hello2())
const hello3 = val => 'Hello' + val
console.log(hello3(' world!'))
- 箭头函数不绑定this关键字,箭头函数中的this,指向的是函数定义位置的上下文this
function fn() {
console.log(this)
return () => {
console.log(this)
}
}
const Fn = fn.call(obj)
Fn()
四、剩余参数
void (function(val1, ...val2) {
console.log(val1)
console.log(val2)
})(10, 20, 30)

- 剩余参数语法允许我们将一个不定数量的参数表示为一个数组。
- 剩余参数和解构配合使用
五、ES6 的内置对象扩展
1、Array 的扩展方法
(1)扩展运算符可以将数组或者对象转为用逗号分隔的参数序列
const ary = [1, 2, 3]
console.log(...ary)
(2)可以使用扩展运算符进行数组的合并
const ary = [1, 2, 3]
console.log(...ary)
const ary1 = [1, 2, 3]
const ary2 = [4, 5, 6]
const ary3 = [...ary1, ...ary2]
ary1.push(...ary2)
console.log(ary3)
console.log(ary1)
(3)使用Array.from()方法可以使伪数组转为真正的数组
const arrLike = {
'0': 'a',
'1': 'b',
'2': 'c',
length: 3
}
const newArr = Array.from(arrLike, item => item + item)
console.log(newArr)
- 方法还可以接受第二个参数,作用类似于数组的map方法,用来对每个元素进行处理,将处理后的值放入返回的数组。
(4)find()方法
- 用于找出第一个 符合条件的数组成员,如果没有找到返回undefined
const arr = [
{
id: 1,
name: 'ljc',
age: 24
},
{
id: 2,
name: 'xlt',
age: 23
},
{
id: 3,
name: 'lhw',
age: 25
}
]
const data = arr.find(item => item.id === 2)
console.log(data)
(5)findIndex()方法
- 用于找出第一个符合条件的数组成员的位置,如果没有找到返回-1
const arr = [
{
id: 1,
name: 'ljc',
age: 24
},
{
id: 2,
name: 'xlt',
age: 23
},
{
id: 3,
name: 'lhw',
age: 25
}
]
const data = arr.findIndex(item => item.id === 2)
console.log(data)
(6)includes()方法
const arr = [1, 2, 3]
const data = arr.includes(2)
console.log(data)
2、String的扩展方法
(1)模板字符串
const name = 'ljc'
const say = `
hello!
my name is ${name}
`
console.log(say)

const fn = () => {
return 'world'
}
const s = `hello ${fn()}`
console.log(s)
(2)startsWith()和endsWith()
- startsWith():表示参数字符串是否在原字符串的开头,返回布尔值
- endsWith():表示参数字符串是否在原字符串的尾部,返回布尔值
let s = 'hello world!'
console.log( s.startsWith('hello') )
console.log( s.startsWith('wo') )
console.log( s.endsWith( '!' ) )
console.log( s.endsWith( 'd' ) )
(3)repeat()
- repeat(n)方法表示将原字符串重复n次,返回一个新字符串
const s = 'hello world\n'
const newS = s.repeat(3)
console.log(newS)

六、Set 数据结构
ES6 提供了新的数据结构 Set。它类似于数组,但是成员的值都是唯一的,没有重复的值。
const set = new Set()
const set = new Set( [1,1,1,2,2,2,3,3,3,4,5,6] )
console.log(set)

Set数据结构的实例方法
- add(value):添加某个值,返回 Set 结构本身
- delete(value):删除某个值,返回一个布尔值,表示删除是否成功
- has(value):返回一个布尔值,表示该值是否为Set的成员
- clear():清除所有成员,没有返回值
const set = new Set()
set
.add(1)
.add(2)
.add(3)
console.log(set)
const d = set.delete(1)
const h = set.has(1)
console.log(d)
console.log(h)
console.log(set)
set.clear()
console.log(set)
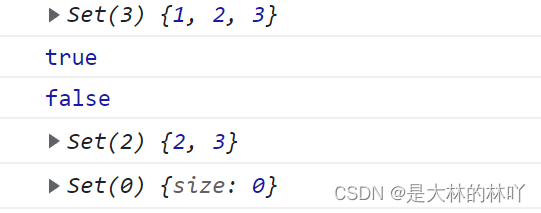
set遍历(foreach())
- Set 结构的实例与数组一样,也拥有forEach方法,用于对每个成员执行某种操作,没有返回值。
const set = new Set()
set
.add(1)
.add(2)
.add(3)
set.forEach(item => {
console.log(item)
})
|