前言
本系列主要整理前端面试中需要掌握的知识点。本节介绍ajax的原理和实现方法。
一、ajax是什么
AJAX全称是 Async Javascript and XML,即异步的JS和XML,是一种创建交互式网页应用开发技术,可以在不重新加载整个网页的情况下,与服务器交换数据,并且更新部分网页。 原理:通过XmlHttpRequest对象来向服务器发异步请求,从服务器获得数据,然后用JavaScript来操作DOM而更新页面。
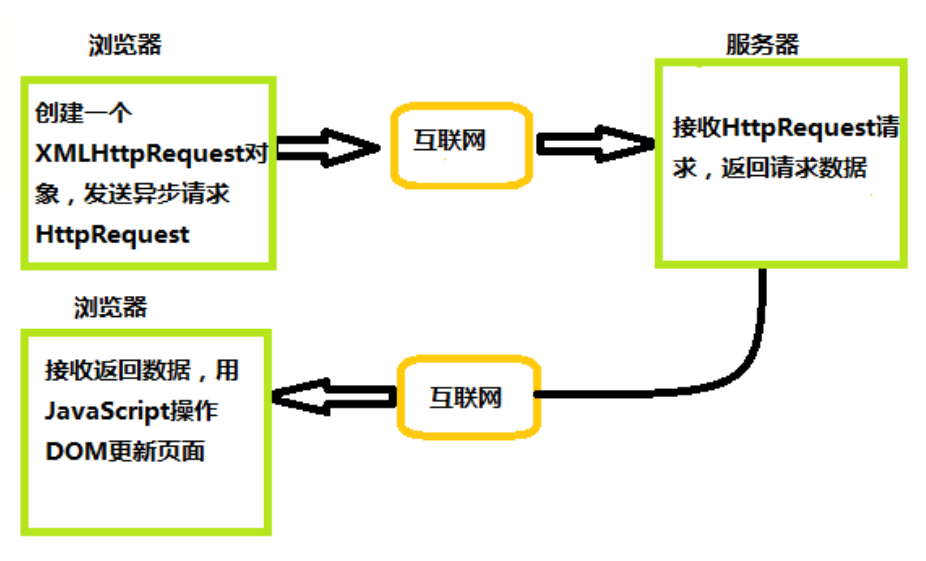
二、实现过程
- 创建Ajax的核心对象XMLHttpRequest对象;
- 通过XMLHttpRequest对象的open()方法与服务器建立连接;
- 构建请求所需的结构数据内容,并通过XMLHttpRequest对象的send()方法发送给服务器端;
- 通过XMLHttpRequest对象提供的onreadystatechange事件监听服务器端你的通信状态;
- 接受并处理服务器端向客户端响应的数据结果;
- 将处理结果更新到HTML页面中。
1.创建XMLHttpRequest对象;
const xhr = new XMLHttpRequest();
2.与服务器建立连接;
xhr.open('GET',url,true);
参数说明: method:表示当前的请求方式,常见的有GET、POST url:服务端地址 async:布尔值,表示是否异步执行操作,默认为true user: 可选的用户名用于认证用途;默认为’null’ password: 可选的密码用于认证用途,默认为’null’
3.send()发送给服务器端;
xhr.send(null)
body: 在 XHR 请求中要发送的数据体,如果不传递数据则为 null
4.onreadystatechange事件监听通信状态;
xhr.onreadystatechange = function(){
console.log('xhr.readyState',xhr.readyState);
if(xhr.readyState === 4){
if(xhr.status === 200){
reslove(JSON.parse(xhr.responseText));
}else{
reject(new Error('请求失败'))
}
}
}
关于XMLHttpRequest.readyState属性有五个状态:
值 | 状态 | 描述 |
---|
0 | UNSENT(未打开) | open()方法还未被调用 | 1 | OPENED(未发送) | send()方法还未被调用 | 2 | HEADERS_RECEIVED(以获取响应头) | send()方法已经被调用,响应头和响应状态已经返回 | 3 | LOADING(正在下载响应体) | 响应体下载中;responseText已经获取部分数据 | 4 | DONE(请求完成) | 整个请求过程已完毕 |
function myAjax(url){
const promise = new Promise((reslove,reject) => {
const xhr = new XMLHttpRequest();
xhr.open('GET',url,true);
xhr.onreadystatechange = function(){
console.log('xhr.readyState',xhr.readyState);
if(xhr.readyState === 4){
if(xhr.status === 200){
reslove(JSON.parse(xhr.responseText));
}else{
reject(new Error('请求失败'))
}
}
}
xhr.send(null)
})
return promise;
}
const url = '/mock/data.json'
myAjax(url).then(data=>{
console.log('data',data);
}).catch(err=>{
console.error(err);
})
三、封装
function myAjax(options){
const xhr = new XMLHttpRequest()
options = options||{}
options.type = (options.type || 'GET').toUpperCase()
options.dataType = options.dataType || 'json'
const params = options.data
if(options.type === 'GET'){
xhr.open('GET',options.url+'?'+params,true)
xhr.send(null)
}else if(options.type === 'POST'){
xhr.open('POST',options.url,true)
xhr.send(params)
}
xhr.onreadystatechange = function(){
if(xhr.readyState === 4){
let status = xhr.status
if(status >= 200 && status < 300){
options.success && options.success(xhr.responseText,xhr.responseXML)
}else{
options.fail && options.fail(status)
}
}
}
}
myAjax({
type:'get',
dataType:'json',
data:{},
url:'/mock/data.json',
success:function(text,xml){
console.log(text);
},
fail:function(status){
console.log(status);
}
})
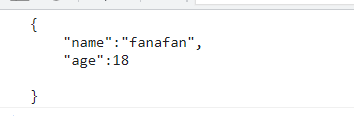
|