1.实现加载服务器端excel文件
引入相关js
<link rel="stylesheet" href="./plugins/css/pluginsCss.css" />
<link rel="stylesheet" href="./plugins/plugins.css" />
<link rel="stylesheet" href="./css/luckysheet.css" />
<link rel="stylesheet" href="./assets/iconfont/iconfont.css" />
<script type="text/javascript" src="./jquery-2.1.1.min.js"></script>
<script type="text/javascript" src="./js/vue.js"></script>
<script type="text/javascript" src="./lib/layui-2.5.6/layui.js"></script>
<script type="text/javascript" src="https://www.xdocin.com/xdoc.js"></script>
<script src="xlsx.full.min.js"></script>
<script type="text/javascript" src="./luckyexcel.umd.js"></script>
<script type="text/javascript" src="./luckysheet.umd.js"></script>
<script src="./plugins/js/plugin.js"></script>
luckysheet容器
<div id="luckysheet" style="margin:0px;padding:0px;width:100%;height:95vh;"></div>
获取excel并渲染
$(function () {
let xhr = new XMLHttpRequest();
xhr.open('GET',"/file/excels/测试excel.xlsx");
xhr.responseType = 'blob';
xhr.onload = () => {
let content = xhr.response;
let blob = new Blob([content]);
let file = new File([blob],'测试excel.xlsx',{ type: 'excel/xlsx' });
console.log("file",file)
LuckyExcel.transformExcelToLucky(
file,
function (exportJson,luckysheetfile) {
luckysheet.create({
container: 'luckysheet',
showinfobar: false,
lang: 'zh',
allowEdit: true,
allowCopy: true,
showtoolbar: true,
showinfobar: true,
showsheetbar: true,
showstatisticBar: true,
pointEdit: false,
pointEditUpdate: null,
data: exportJson.sheets,
title: exportJson.info.name,
userInfo: exportJson.info.name.creator,
});
},
function (err) {
logger.error('Import failed. Is your fail a valid xlsx?');
}
);
let formData = new FormData();
formData.append('file',file);
}
xhr.send();
})
2.文件编辑后保存
提交按钮
<button onclick="downloadCurrent()">提交</button>
提交函数
function downloadCurrent() {
var fileName = $("#luckysheet_info_detail_input").val()
fileName = (fileName + "").trim();
var sumarr =luckysheet.find("SUM",{type:"f"});
if(sumarr && sumarr.length>0){
console.log("sumarr[0].v",sumarr[0].v);
}
$.ajax({
url: '/upload_luckysheet',
type: 'POST',
headers: { 'Content-Type': 'application/json;' },
data: JSON.stringify({
exceldatas: JSON.stringify(luckysheet.getAllSheets()),
fileName: fileName,
}),
success: function (response) {
alert("保存成功!")
}
})
}
后台接口
1)入参VO:LuckysheetParamsVo
import io.swagger.annotations.ApiModelProperty;
import lombok.Data;
import javax.validation.constraints.NotBlank;
@Data
public class LuckysheetParamsVo {
@NotBlank(message = "Luckysheet的表格数据不能为空")
@ApiModelProperty("Luckysheet的表格数据")
private String excelDatas;
@NotBlank(message = "表格名称不能为空")
@ApiModelProperty("表格名称")
private String fileName;
}
2)Controller
@PostMapping("/upload_luckysheet")
@ResponseBody
public String downData(@Valid @RequestBody LuckysheetParamsVo luckysheetParamsVo) {
String filePath = uploadFileDir+"excels/";
String fileName = luckysheetParamsVo.getFileName();
String excelDatas = luckysheetParamsVo.getExcelDatas();
try{
luckysheetService.exportLuckySheetXlsxByPOI("", filePath,fileName,excelDatas);
} catch (Exception e) {
e.printStackTrace();
return new ReturnData(ReturnData.OPERATE_FAIL, ReturnData.SERVICE_EXCEPTION);
}
return filePath+fileName;
}
3)LuckysheetService
public interface LuckysheetService {
String exportLuckySheetXlsxByPOI(String title, String newFileDir, String newFileName, String excelData);
}
4)LuckysheetServiceImpl
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import org.apache.poi.ss.usermodel.BorderStyle;
import org.apache.poi.ss.usermodel.FillPatternType;
import org.apache.poi.ss.usermodel.HorizontalAlignment;
import org.apache.poi.ss.usermodel.VerticalAlignment;
import org.apache.poi.ss.util.CellRangeAddress;
import org.apache.poi.xssf.usermodel.*;
import org.springframework.stereotype.Service;
import java.awt.*;
import java.io.*;
import java.util.HashMap;
import java.util.Map;
@Service
public class LuckysheetServiceImpl implements LuckysheetService {
@Override
public String exportLuckySheetXlsxByPOI(String title, String newFileDir, String newFileName, String excelData) {
excelData = excelData.replace("
", "\\r\\n");
JSONArray jsonArray = (JSONArray) JSONObject.parse(excelData);
XSSFWorkbook excel = new XSSFWorkbook();
for (int sheetIndex = 0; sheetIndex < jsonArray.size(); sheetIndex++) {
JSONObject jsonObject = (JSONObject) jsonArray.get(sheetIndex);
JSONArray celldataObjectList = jsonObject.getJSONArray("celldata");
JSONArray rowObjectList = jsonObject.getJSONArray("visibledatarow");
JSONArray colObjectList = jsonObject.getJSONArray("visibledatacolumn");
JSONArray dataObjectList = jsonObject.getJSONArray("data");
JSONObject mergeObject = jsonObject.getJSONObject("config").getJSONObject("merge");
JSONObject columnlenObject = jsonObject.getJSONObject("config").getJSONObject("columnlen");
JSONObject rowlenObject = jsonObject.getJSONObject("config").getJSONObject("rowlen");
JSONArray borderInfoObjectList = jsonObject.getJSONObject("config").getJSONArray("borderInfo");
XSSFCellStyle cellStyle = excel.createCellStyle();
XSSFSheet sheet = excel.createSheet(jsonObject.getString("name"));
sheet.setForceFormulaRecalculation(true);
if (rowObjectList != null && rowObjectList.size() > 0) {
for (int i = 0; i < rowObjectList.size(); i++) {
XSSFRow row = sheet.createRow(i);
try {
BigDecimal excleHei1=new BigDecimal(72);
BigDecimal excleHei2=new BigDecimal(96);
row.setHeightInPoints(new BigDecimal(rowlenObject.get(i)+ "").multiply(excleHei1).divide(excleHei2).floatValue());
} catch (Exception e) {
row.setHeightInPoints(20f);
}
if (colObjectList != null && colObjectList.size() > 0) {
for (int j = 0; j < colObjectList.size(); j++) {
if (columnlenObject != null && columnlenObject.getInteger(j + "") != null) {
BigDecimal excleWid=new BigDecimal(33);
sheet.setColumnWidth(j, new BigDecimal(rowlenObject.get(j)+ "").multiply(excleWid).setScale(0,BigDecimal.ROUND_HALF_UP).intValue());
}
row.createCell(j);
}
}
}
}
setCellValue(celldataObjectList, borderInfoObjectList, sheet, excel);
File dir = new File(newFileDir);
if (!dir.exists()) {
dir.mkdirs();
}
OutputStream out = null;
try {
out = new FileOutputStream(newFileDir + newFileName);
excel.write(out);
out.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
return newFileDir+newFileName;
}
private static void setMergeAndColorByObject(JSONObject jsonObjectValue, XSSFSheet sheet, XSSFCellStyle style) {
JSONObject mergeObject = (JSONObject) jsonObjectValue.get("mc");
if (mergeObject != null) {
int r = (int) (mergeObject.get("r"));
int c = (int) (mergeObject.get("c"));
if ((mergeObject.get("rs") != null && (mergeObject.get("cs") != null))) {
int rs = (int) (mergeObject.get("rs"));
int cs = (int) (mergeObject.get("cs"));
CellRangeAddress region = new CellRangeAddress(r, r + rs - 1, (short) (c), (short) (c + cs - 1));
sheet.addMergedRegion(region);
}
}
if (jsonObjectValue.getString("bg") != null) {
int bg = Integer.parseInt(jsonObjectValue.getString("bg").replace("#", ""), 16);
style.setFillPattern(FillPatternType.SOLID_FOREGROUND);
style.setFillForegroundColor(new XSSFColor(new Color(bg)));
}
}
private static void setBorder(JSONArray borderInfoObjectList, XSSFWorkbook workbook, XSSFSheet sheet) {
Map<Integer, BorderStyle> bordMap = new HashMap<>();
bordMap.put(1, BorderStyle.THIN);
bordMap.put(2, BorderStyle.HAIR);
bordMap.put(3, BorderStyle.DOTTED);
bordMap.put(4, BorderStyle.DASHED);
bordMap.put(5, BorderStyle.DASH_DOT);
bordMap.put(6, BorderStyle.DASH_DOT_DOT);
bordMap.put(7, BorderStyle.DOUBLE);
bordMap.put(8, BorderStyle.MEDIUM);
bordMap.put(9, BorderStyle.MEDIUM_DASHED);
bordMap.put(10, BorderStyle.MEDIUM_DASH_DOT);
bordMap.put(11, BorderStyle.SLANTED_DASH_DOT);
bordMap.put(12, BorderStyle.THICK);
if (borderInfoObjectList != null && borderInfoObjectList.size() > 0) {
for (int i = 0; i < borderInfoObjectList.size(); i++) {
JSONObject borderInfoObject = (JSONObject) borderInfoObjectList.get(i);
if (borderInfoObject.get("rangeType").equals("cell")) {
JSONObject borderValueObject = borderInfoObject.getJSONObject("value");
JSONObject l = borderValueObject.getJSONObject("l");
JSONObject r = borderValueObject.getJSONObject("r");
JSONObject t = borderValueObject.getJSONObject("t");
JSONObject b = borderValueObject.getJSONObject("b");
int row = borderValueObject.getInteger("row_index");
int col = borderValueObject.getInteger("col_index");
XSSFCell cell = sheet.getRow(row).getCell(col);
if (l != null) {
cell.getCellStyle().setBorderLeft(bordMap.get((int) l.get("style")));
int bg = Integer.parseInt(l.getString("color").replace("#", ""), 16);
cell.getCellStyle().setLeftBorderColor(new XSSFColor(new Color(bg)));
}
if (r != null) {
cell.getCellStyle().setBorderRight(bordMap.get((int) r.get("style")));
int bg = Integer.parseInt(r.getString("color").replace("#", ""), 16);
cell.getCellStyle().setRightBorderColor(new XSSFColor(new Color(bg)));
}
if (t != null) {
cell.getCellStyle().setBorderTop(bordMap.get((int) t.get("style")));
int bg = Integer.parseInt(t.getString("color").replace("#", ""), 16);
cell.getCellStyle().setTopBorderColor(new XSSFColor(new Color(bg)));
}
if (b != null) {
cell.getCellStyle().setBorderBottom(bordMap.get((int) b.get("style")));
int bg = Integer.parseInt(b.getString("color").replace("#", ""), 16);
cell.getCellStyle().setBottomBorderColor(new XSSFColor(new Color(bg)));
}
} else if (borderInfoObject.get("rangeType").equals("range")) {
int bg_ = Integer.parseInt(borderInfoObject.getString("color").replace("#", ""), 16);
int style_ = borderInfoObject.getInteger("style");
JSONObject rangObject = (JSONObject) ((JSONArray) (borderInfoObject.get("range"))).get(0);
JSONArray rowList = rangObject.getJSONArray("row");
JSONArray columnList = rangObject.getJSONArray("column");
for (int row_ = rowList.getInteger(0); row_ < rowList.getInteger(rowList.size() - 1) + 1; row_++) {
for (int col_ = columnList.getInteger(0); col_ < columnList.getInteger(columnList.size() - 1) + 1; col_++) {
XSSFCell cell = sheet.getRow(row_).getCell(col_);
cell.getCellStyle().setBorderLeft(bordMap.get(style_));
cell.getCellStyle().setLeftBorderColor(new XSSFColor(new Color(bg_)));
cell.getCellStyle().setBorderRight(bordMap.get(style_));
cell.getCellStyle().setRightBorderColor(new XSSFColor(new Color(bg_)));
cell.getCellStyle().setBorderTop(bordMap.get(style_));
cell.getCellStyle().setTopBorderColor(new XSSFColor(new Color(bg_)));
cell.getCellStyle().setBorderBottom(bordMap.get(style_));
cell.getCellStyle().setBottomBorderColor(new XSSFColor(new Color(bg_)));
}
}
}
}
}
}
private static void setCellValue(JSONArray jsonObjectList, JSONArray borderInfoObjectList, XSSFSheet
sheet, XSSFWorkbook workbook) {
for (int index = 0; index < jsonObjectList.size(); index++) {
XSSFCellStyle style = workbook.createCellStyle();
XSSFFont font = workbook.createFont();
JSONObject object = jsonObjectList.getJSONObject(index);
String str_ = (int) object.get("r") + "_" + object.get("c") + "=" + ((JSONObject) object.get("v")).get("v") + "\n";
JSONObject jsonObjectValue = ((JSONObject) object.get("v"));
String value = "";
String t = "";
if (jsonObjectValue != null ) {
if (jsonObjectValue.get("v") != null){
value = jsonObjectValue.getString("v");
}
if (jsonObjectValue.get("ct") != null){
JSONObject jsonObject = ((JSONObject) jsonObjectValue.get("ct"));
if (jsonObject.get("t") != null){
t = jsonObject.getString("t");
}
}
}
if (sheet.getRow((int) object.get("r")) != null && sheet.getRow((int) object.get("r")).getCell((int) object.get("c")) != null) {
XSSFCell cell = sheet.getRow((int) object.get("r")).getCell((int) object.get("c"));
if (jsonObjectValue != null && jsonObjectValue.get("f") != null) {
String f = jsonObjectValue.getString("f");
cell.setCellFormula(f.substring(1,f.length()));
cell.setCellValue(value);
}else {
switch(t){
case "n":
cell.setCellValue(Double.valueOf(value));
break;
default:
cell.setCellValue(value);
}
}
setMergeAndColorByObject(jsonObjectValue, sheet, style);
XSSFRow row = sheet.getRow((int) object.get("r"));
int vt = jsonObjectValue.getInteger("vt") == null ? 1 : jsonObjectValue.getInteger("vt");
int ht = jsonObjectValue.getInteger("ht") == null ? 1 : jsonObjectValue.getInteger("ht");
switch (vt) {
case 0:
style.setVerticalAlignment(VerticalAlignment.CENTER);
break;
case 1:
style.setVerticalAlignment(VerticalAlignment.TOP);
break;
case 2:
style.setVerticalAlignment(VerticalAlignment.BOTTOM);
break;
}
switch (ht) {
case 0:
style.setAlignment(HorizontalAlignment.CENTER);
break;
case 1:
style.setAlignment(HorizontalAlignment.LEFT);
break;
case 2:
style.setAlignment(HorizontalAlignment.RIGHT);
break;
}
String ff = jsonObjectValue.getString("ff");
int fs = jsonObjectValue.getInteger("fs") == null ? 14 : jsonObjectValue.getInteger("fs");
int bl = jsonObjectValue.getInteger("bl") == null ? 0 : jsonObjectValue.getInteger("bl");
int it = jsonObjectValue.getInteger("it") == null ? 0 : jsonObjectValue.getInteger("it");
String fc = jsonObjectValue.getString("fc") == null ? "" : jsonObjectValue.getString("fc");
if (fc.length() > 0) {
font.setColor(new XSSFColor(new Color(Integer.parseInt(fc.replace("#", ""), 16))));
}
font.setFontName(ff);
font.setFontHeightInPoints((short) fs);
if (bl == 1) {
font.setBold(true);
}
font.setItalic(it == 1 ? true : false);
style.setFont(font);
style.setWrapText(true);
cell.setCellStyle(style);
} else {
System.out.println("错误的=" + index + ">>>" + str_);
}
}
setBorder(borderInfoObjectList, workbook, sheet);
}
}
期间遇到的问题 1.修改后的excel文件打开发现sum函数未保存进去 解决方案:在上面exportLuckySheetXlsxByPOI方法中创建XSSFSheet对象之后设置sheet.setForceFormulaRecalculation(true);  2.保存之后的excel文件中所有的值均为字符串类型,导致sum函数为0 解决方案:在上面setCellValue方法中往excel对象写值时指定其格式 这里的“n”对应得Luckysheet单元格值格式的数字类型 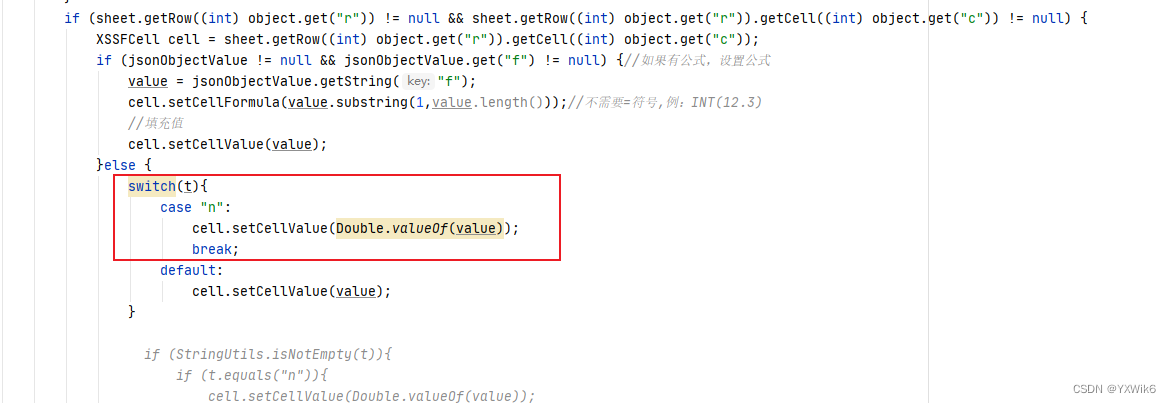 3.保存之后发现在excel文件中单元格的高度和宽度便大了,排查问题的时候也发现写入文件之前设置的高度和宽度值都没有发生变化,写入到excel中才会变大,网上寻找答案时无意中发现有个博客中记载着转换方式:博客地址 将创建excel的行和列代码更改后发现问题解决: 原创建行和列代码:
if (rowObjectList != null && rowObjectList.size() > 0) {
for (int i = 0; i < rowObjectList.size(); i++) {
XSSFRow row = sheet.createRow(i);
try {
row.setHeightInPoints(Float.parseFloat(rowlenObject.get(i) + ""));
} catch (Exception e) {
row.setHeightInPoints(20f);
}
if (colObjectList != null && colObjectList.size() > 0) {
for (int j = 0; j < colObjectList.size(); j++) {
if (columnlenObject != null && columnlenObject.getInteger(j + "") != null) {
sheet.setColumnWidth(j, columnlenObject.getInteger(j + "") * 42);
}
row.createCell(j);
}
}
}
}
通过转换创建行和列代码:
if (rowObjectList != null && rowObjectList.size() > 0) {
for (int i = 0; i < rowObjectList.size(); i++) {
XSSFRow row = sheet.createRow(i);
try {
BigDecimal excleHei1=new BigDecimal(72);
BigDecimal excleHei2=new BigDecimal(96);
row.setHeightInPoints(new BigDecimal(rowlenObject.get(i)+ "").multiply(excleHei1).divide(excleHei2).floatValue());
} catch (Exception e) {
row.setHeightInPoints(20f);
}
if (colObjectList != null && colObjectList.size() > 0) {
for (int j = 0; j < colObjectList.size(); j++) {
if (columnlenObject != null && columnlenObject.getInteger(j + "") != null) {
BigDecimal excleWid=new BigDecimal(33);
sheet.setColumnWidth(j, new BigDecimal(rowlenObject.get(j)+ "").multiply(excleWid).setScale(0,BigDecimal.ROUND_HALF_UP).intValue());
row.createCell(j);
}
row.createCell(j);
}
}
}
}
4.使用luckysheet.find(“SUM”,{type:“f”})函数查找返回空的问题 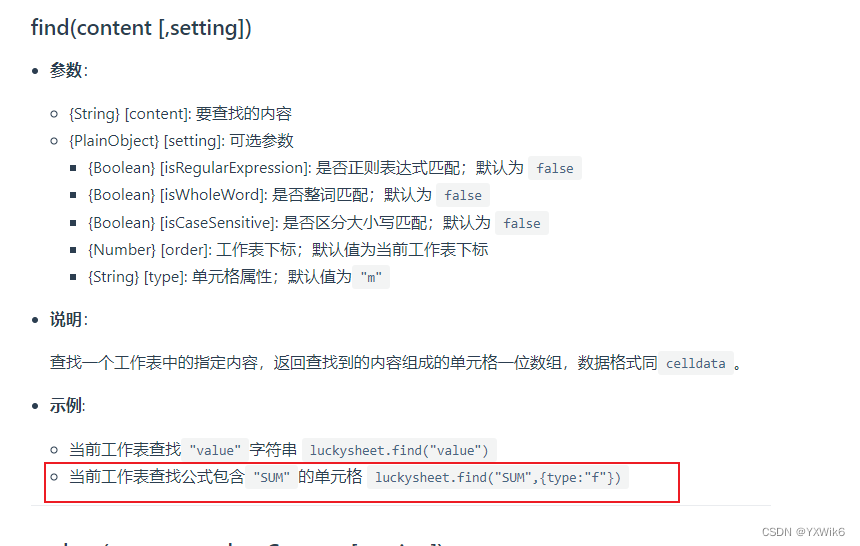 解决方案:我这里的原因是因为luckysheet.umd.js的版本不是最新版导致的,更换最新版发现问题解决 其他 Luckysheet中的一些函数:
hook: {
cellDragStop: function (cell, postion, sheetFile, ctx, event) {
},
rowTitleCellRenderBefore: function (rowNum, postion, ctx) {
},
rowTitleCellRenderAfter: function (rowNum, postion, ctx) {
},
columnTitleCellRenderBefore: function (columnAbc, postion, ctx) {
},
columnTitleCellRenderAfter: function (columnAbc, postion, ctx) {
},
cellRenderBefore: function (cell, postion, sheetFile, ctx) {
},
cellRenderAfter: function (cell, postion, sheetFile, ctx) {
},
cellMousedownBefore: function (cell, postion, sheetFile, ctx) {
},
cellMousedown: function (cell, postion, sheetFile, ctx) {
},
sheetMousemove: function (cell, postion, sheetFile, moveState, ctx) {
},
sheetMouseup: function (cell, postion, sheetFile, moveState, ctx) {
},
cellAllRenderBefore: function (data, sheetFile, ctx) {
},
updated: function (operate) {
},
cellUpdateBefore: function (r, c, value, isRefresh) {
},
cellUpdated: function (r, c, oldValue, newValue, isRefresh) {
},
sheetActivate: function (index, isPivotInitial, isNewSheet) {
},
rangeSelect: function (index, sheet) {
},
commentInsertBefore: function (r, c) {
},
commentInsertAfter: function (r, c, cell) {
},
commentDeleteBefore: function (r, c, cell) {
},
commentDeleteAfter: function (r, c, cell) {
},
commentUpdateBefore: function (r, c, value) {
},
commentUpdateAfter: function (r, c, oldCell, newCell) {
},
cellEditBefore: function (range) {
},
workbookCreateAfter: function (json) {
},
rangePasteBefore: function (range, data) {
},
}
例如: 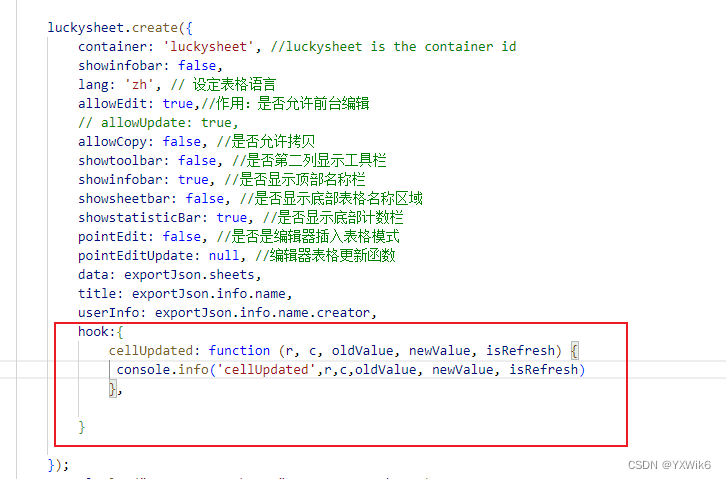
DEMO地址: 链接:https://pan.baidu.com/s/1fa_4mGnKiH_TOXi0OlKR6g?pwd=7pcy 提取码:7pcy
下载demo然后解压,放置D盘根目录下 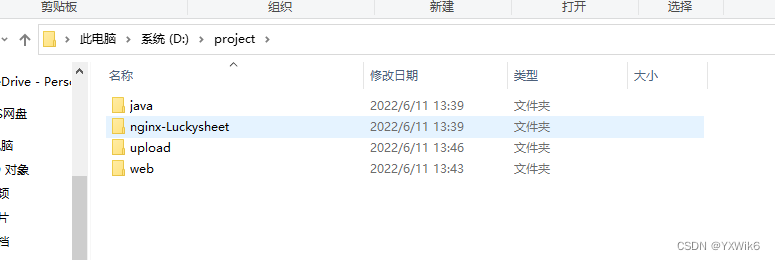
启动其中的Java代码 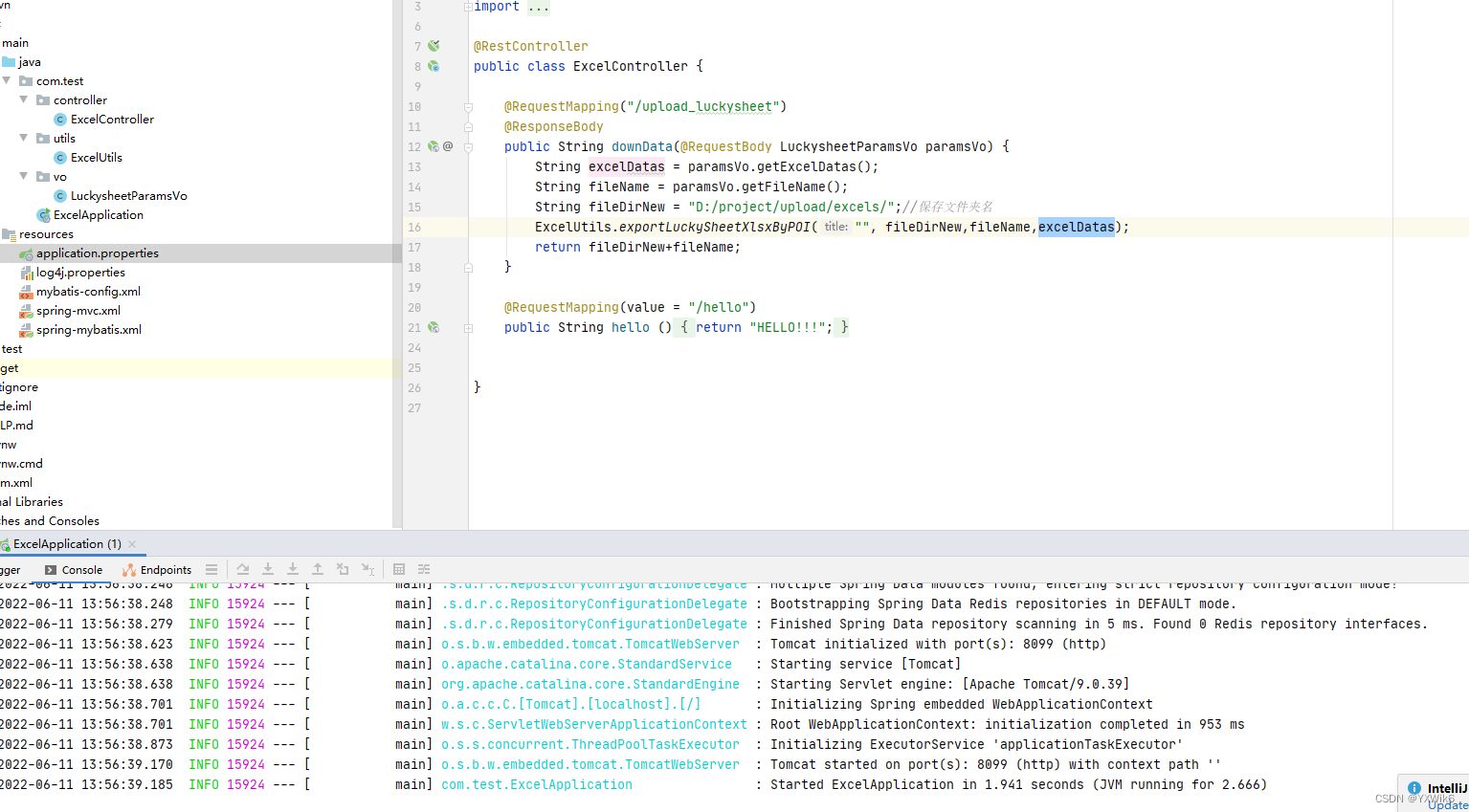 运行nginx-Luckysheet文件夹下的luckysheet.exe 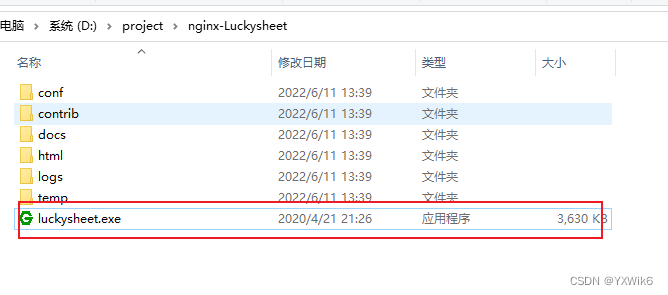 访问 http://localhost:8088/web/ 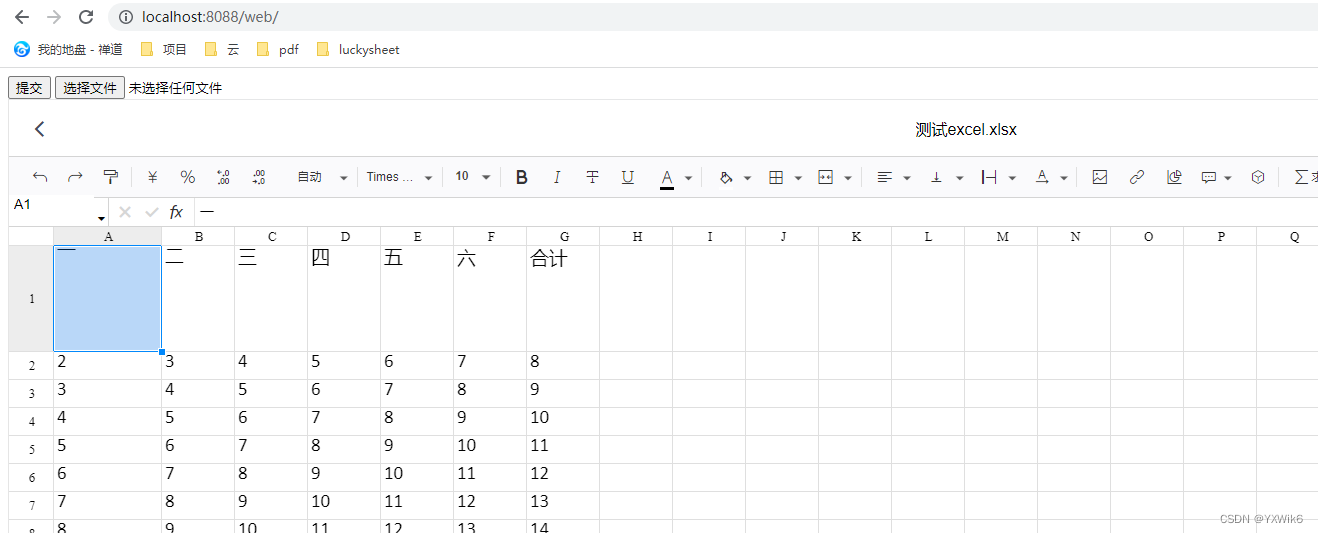
|